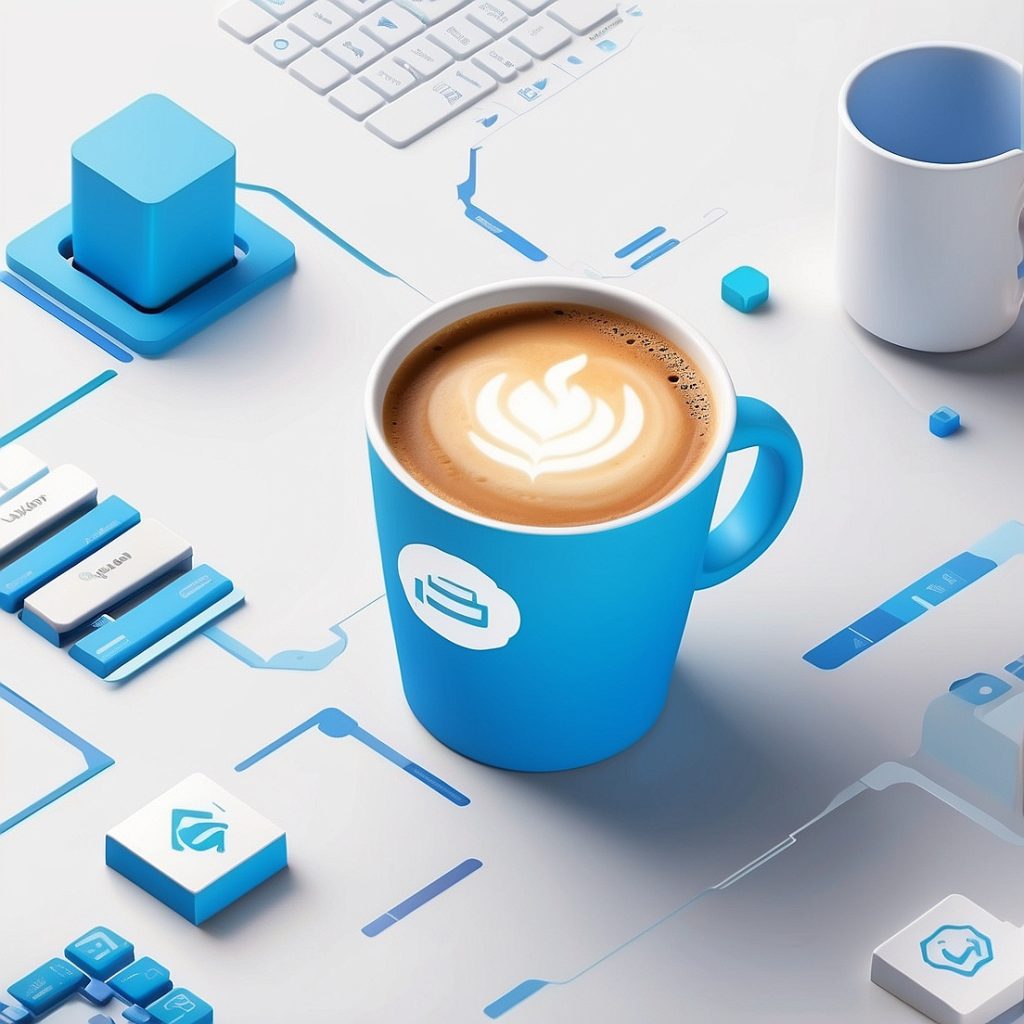
Beyond GC: Demystifying Memory Management in Java
Java is known for its developer-friendly features, and automatic memory management through Garbage Collection (GC) is a prime example. While GC is a powerful tool, a deeper understanding of memory management in Java empowers developers to write more efficient and performant applications. This blog delves beyond the basics of GC, exploring the intricacies of the Java memory model, heap generations, and advanced techniques for memory optimization.
Understanding the Java Memory Model
Java enforces a strict memory model that dictates how objects are created, accessed, and manipulated. This model separates the programmer’s view of memory from the physical memory layout. Objects reside in the heap, a managed memory space, while references to those objects live on the stack, a thread-local storage area.
Sample Code:
public class MemoryModelDemo {
public static void main(String[] args) {
String name = "Alice"; // reference on stack
Object obj = new Object(); // object in heap
}
}
In this example, the name
variable is a reference on the stack that points to a String object allocated in the heap. The obj
variable directly holds a reference to an object in the heap.
Demystifying the Heap and Garbage Collection
The heap is the primary battleground for memory management. Objects are allocated memory in the heap as needed, and GC automatically reclaims memory occupied by unreachable objects. However, GC is not instantaneous, and understanding its behavior is crucial.
Generational Garbage Collection:
The Java heap is typically divided into generations based on object lifespans. The Young Generation, where most short-lived objects reside, undergoes frequent, minor collections. The Old Generation, for longer-lived objects, is collected less often but with potentially longer pauses.
Code Snippet:
// Assuming a generational GC with Young and Old generations
public class ObjectLifespan {
public static void main(String[] args) throws InterruptedException {
for (int i = 0; i < 10000; i++) {
Object temp = new Object(); // Young generation object
}
Thread.sleep(1000); // Allow some objects to become unreachable
System.gc(); // Force a full GC (not recommended in practice)
}
}
This code snippet demonstrates the Young generation. As temporary objects are created and go out of scope, they become unreachable and are candidates for minor collections.
Advanced Techniques for Memory Optimization
While GC automates memory management, developers can influence its behavior and optimize memory usage. Here are some key techniques:
- Finalization: The
finalize()
method allows objects to clean up resources before being garbage collected. However, it’s generally discouraged due to unpredictable execution timing. - Weak References: Weak references allow objects to be collected even if they are still referenced by a weak reference. This is useful for caching scenarios where entries can be expired automatically.
Sample Code (Weak References):
public class WeakReferenceDemo {
public static void main(String[] args) {
ReferenceQueue<MyClass> queue = new ReferenceQueue<>();
WeakReference<MyClass> ref = new WeakReference<>(new MyClass(), queue);
// Use the object referenced by ref
// ...
if (queue.poll() != null) {
System.out.println("Object referenced by ref is garbage collected");
}
}
}
class MyClass {
// ...
}
In this example, the WeakReference
allows the MyClass
object to be collected even though ref
holds a weak reference to it. The ReferenceQueue
helps identify when the object is collected.
- Profiling and Monitoring: Tools like VisualVM or JVisualVM can be used to monitor memory usage and identify potential memory leaks. By analyzing heap dumps, developers can pinpoint objects that are not being garbage collected as expected.
Remember: Understanding memory management in Java goes beyond GC. By delving into the memory model, heap generations, and advanced techniques, developers can write more efficient and performant applications. This empowers them to create applications that utilize memory resources effectively and avoid potential pitfalls.
Further Exploration:
This blog has provided a foundational understanding of memory management in Java. There’s a wealth of information available for further exploration, including the official Java documentation on memory management and GC https://blogs.oracle.com/javamagazine/post/understanding-garbage-collectors, as well as in-depth articles on specific GC algorithms and memory optimization strategies.