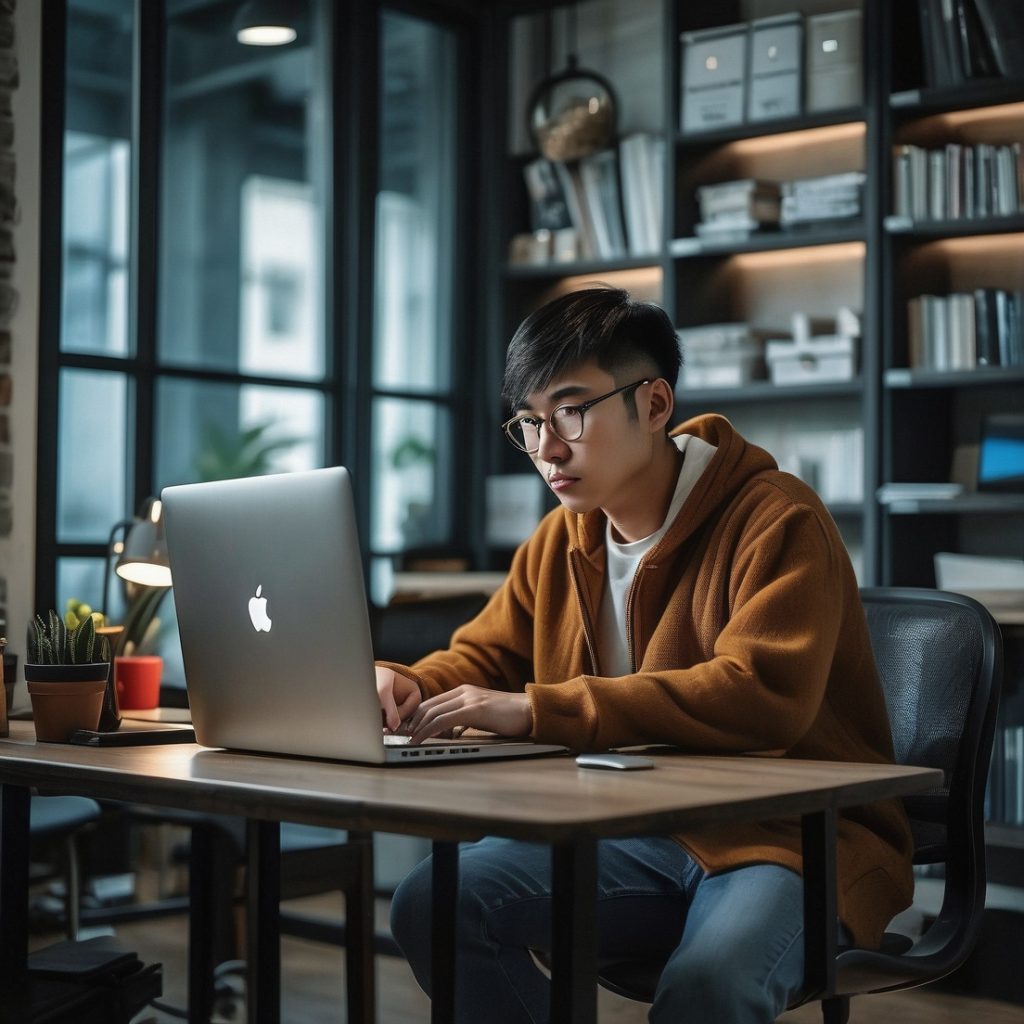
Beyond Loops and Lists: Unlocking Python’s Hidden Gems
Python is a language beloved by many for its simplicity and readability. But beyond the basics of loops and lists lies a treasure trove of advanced features and hidden gems that can make your code more efficient, readable, and powerful. In this blog, we’ll explore some of these lesser-known but highly valuable Python features, delving into the world of metaprogramming, context managers, decorators, and more.
Exploring Python’s Magic Methods
Python’s magic methods, also known as dunder (double underscore) methods, allow you to define how objects of your classes behave with built-in operations. These methods are a powerful tool for creating more intuitive and Pythonic classes.
The __init__
and __repr__
Methods
The __init__
method is one of the most commonly used magic methods, responsible for initializing an object’s attributes. The __repr__
method provides a string representation of the object, useful for debugging.
class Point:
def __init__(self, x, y):
self.x = x
self.y = y
def __repr__(self):
return f"Point({self.x}, {self.y})"
p = Point(1, 2)
print(p) # Output: Point(1, 2)
Operator Overloading
Magic methods can also be used to overload operators, allowing you to define custom behavior for standard operations.
class Vector:
def __init__(self, x, y):
self.x = x
self.y = y
def __add__(self, other):
return Vector(self.x + other.x, self.y + other.y)
def __repr__(self):
return f"Vector({self.x}, {self.y})"
v1 = Vector(2, 3)
v2 = Vector(5, 7)
print(v1 + v2) # Output: Vector(7, 10)
Custom Iterable Objects
By implementing the __iter__
and __next__
methods, you can create custom iterable objects that work with Python’s iteration protocols.
class Countdown:
def __init__(self, start):
self.current = start
def __iter__(self):
return self
def __next__(self):
if self.current <= 0:
raise StopIteration
self.current -= 1
return self.current
for count in Countdown(5):
print(count) # Output: 4 3 2 1 0
Leveraging Python’s Decorators
Decorators are a powerful and expressive tool for modifying the behavior of functions or methods. They allow you to wrap another function and extend its behavior without permanently modifying it.
Basic Function Decorators
A simple decorator can log the execution of a function.
def log_decorator(func):
def wrapper(*args, **kwargs):
print(f"Calling {func.__name__} with args: {args}, kwargs: {kwargs}")
result = func(*args, **kwargs)
print(f"{func.__name__} returned {result}")
return result
return wrapper
@log_decorator
def add(a, b):
return a + b
print(add(5, 3)) # Output: Calling add with args: (5, 3), kwargs: {} \n add returned 8 \n 8
Class-Based Decorators
For more complex scenarios, you can use class-based decorators.
class RepeatDecorator:
def __init__(self, times):
self.times = times
def __call__(self, func):
def wrapper(*args, **kwargs):
result = None
for _ in range(self.times):
result = func(*args, **kwargs)
return result
return wrapper
@RepeatDecorator(3)
def greet(name):
print(f"Hello, {name}!")
greet("Alice") # Output: Hello, Alice! \n Hello, Alice! \n Hello, Alice!
Decorating Methods
Decorators can also be used with class methods to extend their functionality.
def uppercase_decorator(func):
def wrapper(*args, **kwargs):
result = func(*args, **kwargs)
return result.upper()
return wrapper
class Person:
def __init__(self, name):
self.name = name
@uppercase_decorator
def greet(self):
return f"Hello, my name is {self.name}"
p = Person("Alice")
print(p.greet()) # Output: HELLO, MY NAME IS ALICE
Context Managers: Ensuring Resource Management
Context managers are used to manage resources, ensuring that they are properly acquired and released. The most common way to create a context manager is by using the with
statement.
The with
Statement
Using a context manager with the with
statement ensures that resources are released, even if an exception occurs.
with open("example.txt", "w") as file:
file.write("Hello, World!")
# The file is automatically closed here
Creating Custom Context Managers
You can create your own context managers using the contextlib
module.
from contextlib import contextmanager
@contextmanager
def open_file(file, mode):
f = open(file, mode)
try:
yield f
finally:
f.close()
with open_file("example.txt", "w") as f:
f.write("Hello, again!")
# The file is automatically closed here
Class-Based Context Managers
You can also create context managers using classes with the __enter__
and __exit__
methods.
class OpenFile:
def __init__(self, filename, mode):
self.filename = filename
self.mode = mode
def __enter__(self):
self.file = open(self.filename, self.mode)
return self.file
def __exit__(self, exc_type, exc_val, exc_tb):
self.file.close()
with OpenFile("example.txt", "w") as f:
f.write("Hello, once more!")
# The file is automatically closed here
Metaprogramming with Metaclasses
Metaclasses allow you to modify or create classes dynamically. They are a powerful feature for advanced Python users who need to control the creation and behavior of classes.
Basic Metaclass Example
A metaclass is a class of a class that defines how a class behaves. A class is an instance of a metaclass.
class MyMeta(type):
def __new__(cls, name, bases, dct):
print(f"Creating class {name}")
return super().__new__(cls, name, bases, dct)
class MyClass(metaclass=MyMeta):
pass
# Output: Creating class MyClass
Customizing Class Creation
You can customize class creation by overriding the __new__
and __init__
methods in the metaclass.
class SingletonMeta(type):
_instances = {}
def __call__(cls, *args, **kwargs):
if cls not in cls._instances:
instance = super().__call__(*args, **kwargs)
cls._instances[cls] = instance
return cls._instances[cls]
class Singleton(metaclass=SingletonMeta):
def __init__(self):
print("Creating a new instance")
s1 = Singleton()
s2 = Singleton()
print(s1 is s2) # Output: True
Utilizing Python’s Standard Library
Python’s standard library is a goldmine of functionality. Let’s explore some of its hidden gems that can simplify your code and boost productivity.
The collections
Module
The collections
module provides specialized container datatypes.
from collections import namedtuple, deque, Counter, defaultdict
# namedtuple
Point = namedtuple("Point", "x y")
p = Point(10, 20)
print(p.x, p.y) # Output: 10 20
# deque
d = deque(["a", "b", "c"])
d.append("d")
d.appendleft("z")
print(d) # Output: deque(['z', 'a', 'b', 'c', 'd'])
# Counter
counter = Counter("mississippi")
print(counter) # Output: Counter({'s': 4, 'i': 4, 'p': 2, 'm': 1})
# defaultdict
dd = defaultdict(int)
dd["a"] += 1
print(dd) # Output: defaultdict(<class 'int'>, {'a': 1})
The itertools
Module
The itertools
module provides functions that create iterators for efficient looping.
import itertools
# count
for i in itertools.count(10, 2):
if i > 20:
break
print(i) # Output: 10 12 14 16 18 20
# cycle
colors = itertools.cycle(["red", "green", "blue"])
for _ in range(6):
print(next(colors)) # Output: red green blue red green blue
# permutations
perms = itertools.permutations([1, 2, 3])
for perm in perms:
print(perm) # Output: (1, 2, 3) (1, 3, 2) (2, 1, 3) (2, 3, 1) (3, 1, 2) (3,
2, 1)
The functools
Module
The functools
module provides higher-order functions that act on or return other functions.
from functools import lru_cache, partial, reduce
# lru_cache
@lru_cache(maxsize=None)
def fibonacci(n):
if n < 2:
return n
return fibonacci(n - 1) + fibonacci(n - 2)
print(fibonacci(10)) # Output: 55
# partial
def multiply(x, y):
return x * y
double = partial(multiply, 2)
print(double(5)) # Output: 10
# reduce
numbers = [1, 2, 3, 4, 5]
sum = reduce(lambda x, y: x + y, numbers)
print(sum) # Output: 15
Asynchronous Programming with asyncio
Asynchronous programming can greatly improve the performance of your code, especially when dealing with I/O-bound tasks. Python’s asyncio
module provides a framework for writing asynchronous code.
Basic asyncio
Example
The asyncio
module allows you to write asynchronous code using async
and await
.
import asyncio
async def say_hello():
print("Hello")
await asyncio.sleep(1)
print("World")
asyncio.run(say_hello())
# Output: Hello \n (after 1 second) World
Running Multiple Coroutines
You can run multiple coroutines concurrently using asyncio.gather
.
async def greet(name):
print(f"Hello, {name}")
await asyncio.sleep(1)
print(f"Goodbye, {name}")
async def main():
await asyncio.gather(
greet("Alice"),
greet("Bob")
)
asyncio.run(main())
# Output: Hello, Alice \n Hello, Bob \n (after 1 second) Goodbye, Alice \n Goodbye, Bob
Creating an Asynchronous Context Manager
You can create asynchronous context managers using the async with
statement.
class AsyncFile:
def __init__(self, filename, mode):
self.filename = filename
self.mode = mode
async def __aenter__(self):
self.file = open(self.filename, self.mode)
return self.file
async def __aexit__(self, exc_type, exc_val, exc_tb):
self.file.close()
async def write_file():
async with AsyncFile("example.txt", "w") as f:
f.write("Hello, World!")
asyncio.run(write_file())
Data Classes: Simplifying Class Definitions
Introduced in Python 3.7, data classes provide a decorator and functions for automatically adding special methods to user-defined classes.
Basic Data Class Example
Data classes reduce boilerplate code for class definitions.
from dataclasses import dataclass
@dataclass
class Point:
x: int
y: int
p = Point(10, 20)
print(p) # Output: Point(x=10, y=20)
Default Values and Type Annotations
Data classes support default values and type annotations.
@dataclass
class Person:
name: str
age: int = 25
p = Person("Alice")
print(p) # Output: Person(name='Alice', age=25)
Immutable Data Classes
You can create immutable data classes by setting frozen=True
.
@dataclass(frozen=True)
class ImmutablePoint:
x: int
y: int
p = ImmutablePoint(10, 20)
# p.x = 15 # This will raise a FrozenInstanceError
Python’s hidden gems extend far beyond basic loops and lists, offering advanced features and tools that can elevate your coding skills. From magic methods and decorators to context managers and asynchronous programming, Python provides a rich ecosystem for writing clean, efficient, and expressive code. By exploring these hidden gems, you’ll unlock new possibilities and deepen your understanding of this versatile language. So, dive in, experiment, and let Python’s hidden treasures transform your coding journey. Happy coding!