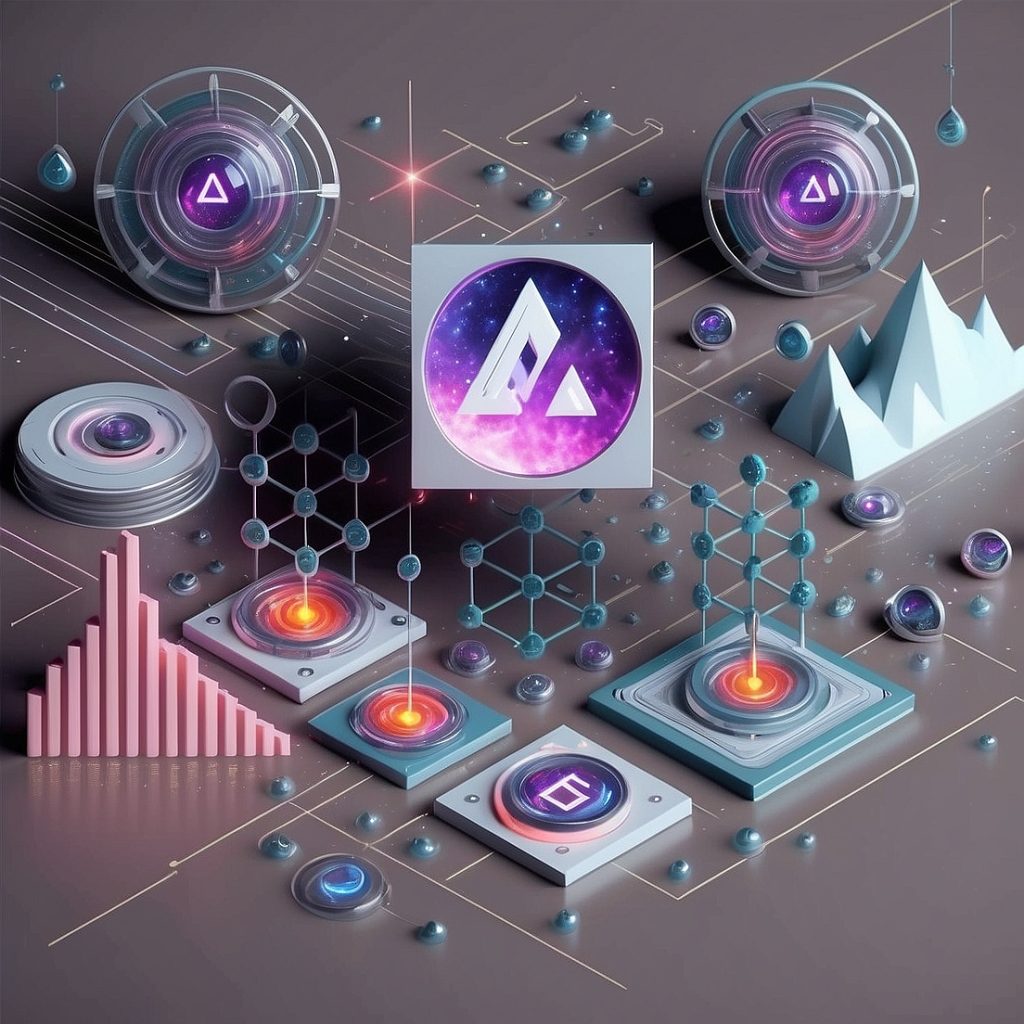
Beyond the Basics: Unveiling the Advanced Features of Avalanche
Avalanche, a revolutionary platform for decentralized applications and enterprise blockchain deployments, has gained significant traction since its inception. While many are familiar with its basic functionalities, there’s a treasure trove of advanced features that can take your blockchain projects to the next level. In this blog, we will dive deep into these advanced features, providing you with a comprehensive understanding and practical insights. Get ready for a fun, conversational journey through the intricate landscape of Avalanche’s capabilities!
Understanding the Avalanche Consensus Protocol
The heart of Avalanche is its consensus protocol, a novel approach that provides unparalleled speed and security. Unlike traditional consensus mechanisms, Avalanche uses a unique approach that ensures faster finality and scalability.
Snowball Sampling and Subsampling
The Avalanche consensus protocol employs a process known as Snowball sampling. Instead of requiring all nodes to agree simultaneously, it leverages a smaller subset of nodes, known as subsampling. This method reduces the communication overhead and speeds up the consensus process.
Benefits of Avalanche Consensus
- Speed: Transactions on Avalanche achieve finality in just a few seconds.
- Scalability: The network can handle thousands of transactions per second.
- Security: The probabilistic approach ensures a high level of security against 51% attacks.
Here’s a simple code snippet to demonstrate how you can interact with the Avalanche network using its API:
from avalanche import Avalanche
# Connect to Avalanche network
avax = Avalanche("https://api.avax.network")
# Check network status
status = avax.get_status()
print(f"Network Status: {status}")
This script connects to the Avalanche network and checks its status, showcasing the simplicity of interacting with Avalanche.
Exploring Avalanche’s Subnets
Avalanche’s architecture is designed to support multiple, interoperable blockchains called subnets. These subnets allow for customization and optimization based on specific use cases.
Creating and Managing Subnets
Creating a subnet in Avalanche involves defining the rules and parameters that govern it. This can include consensus mechanisms, virtual machines, and transaction fees.
Benefits of Subnets
- Customization: Tailor the blockchain to specific application needs.
- Interoperability: Seamlessly interact with other subnets and the primary Avalanche network.
- Performance Optimization: Adjust parameters to optimize for speed, security, or decentralization.
Here’s an example of how to create a new subnet using Avalanche’s API:
from avalanche import Avalanche
# Initialize Avalanche connection
avax = Avalanche("https://api.avax.network")
# Create a new subnet
subnet_params = {
"consensus": "snowman++",
"virtual_machine": "EVM",
"transaction_fee": 1000000
}
subnet_id = avax.create_subnet(subnet_params)
print(f"New Subnet ID: {subnet_id}")
This script demonstrates the process of creating a subnet with specific parameters, illustrating the flexibility and power of Avalanche’s subnet architecture.
Leveraging Avalanche’s Cross-Chain Functionality
One of the standout features of Avalanche is its ability to facilitate cross-chain transactions seamlessly. This capability is vital for building complex, interoperable blockchain ecosystems.
Atomic Swaps and Cross-Chain Transactions
Atomic swaps enable the exchange of assets between different blockchains without the need for a centralized intermediary. This is crucial for decentralized finance (DeFi) applications and other cross-chain use cases.
Benefits of Cross-Chain Functionality
- Interoperability: Easily move assets between different chains.
- Security: Atomic swaps ensure that transactions are either completed fully or not at all, reducing the risk of partial failures.
- Efficiency: Streamlined processes for asset transfers and smart contract interactions.
Here’s a code snippet to perform a cross-chain transaction on Avalanche:
from avalanche import Avalanche
# Initialize Avalanche connection
avax = Avalanche("https://api.avax.network")
# Perform a cross-chain transaction
tx_params = {
"source_chain": "X-Chain",
"destination_chain": "C-Chain",
"amount": 1000,
"asset": "AVAX"
}
tx_id = avax.cross_chain_transfer(tx_params)
print(f"Transaction ID: {tx_id}")
This script showcases the ease with which you can perform cross-chain transactions on Avalanche, highlighting the platform’s advanced functionality.
Advanced Smart Contract Capabilities
Smart contracts are the backbone of decentralized applications (dApps). Avalanche offers advanced capabilities that enhance the development and deployment of smart contracts.
Avalanche Contract Chain (C-Chain)
The C-Chain is specifically designed for creating and managing smart contracts. It uses the Ethereum Virtual Machine (EVM), allowing developers to leverage existing tools and knowledge from the Ethereum ecosystem.
Benefits of Advanced Smart Contracts
- Compatibility: Use existing Ethereum tools and libraries.
- Performance: Optimized for high throughput and low latency.
- Flexibility: Support for complex smart contract logic and interactions.
Here’s a simple example of deploying a smart contract on the Avalanche C-Chain:
// SPDX-License-Identifier: MIT
pragma solidity ^0.8.0;
contract SimpleStorage {
uint256 private storedData;
function set(uint256 data) public {
storedData = data;
}
function get() public view returns (uint256) {
return storedData;
}
}
To deploy this contract on the C-Chain, you can use tools like Remix or Truffle, following similar steps as you would on Ethereum.
Utilizing Avalanche’s Governance Mechanisms
Governance is a crucial aspect of any decentralized platform. Avalanche provides robust mechanisms for decentralized governance, allowing stakeholders to have a say in the network’s evolution.
Proposal and Voting System
Avalanche’s governance system enables the creation of proposals for network upgrades, parameter changes, and other important decisions. Stakeholders can vote on these proposals, ensuring a democratic process.
Benefits of Decentralized Governance
- Inclusivity: All stakeholders have a voice in the decision-making process.
- Transparency: Proposals and voting results are publicly available.
- Flexibility: The network can evolve and adapt based on the community’s needs.
Here’s a script to create a governance proposal on Avalanche:
from avalanche import Avalanche
# Initialize Avalanche connection
avax = Avalanche("https://api.avax.network")
# Create a governance proposal
proposal_params = {
"title": "Increase block size",
"description": "Proposal to increase the block size to 2MB",
"voting_period": 7
}
proposal_id = avax.create_proposal(proposal_params)
print(f"Proposal ID: {proposal_id}")
This example highlights how you can participate in Avalanche’s governance, showcasing the platform’s commitment to decentralization and community involvement.
Harnessing Avalanche’s Developer Tools
Avalanche offers a rich set of developer tools that streamline the development process, making it easier to build and deploy applications on the network.
AvalancheJS
AvalancheJS is a comprehensive JavaScript library that simplifies interactions with the Avalanche network. It provides functions for managing assets, creating transactions, and interacting with smart contracts.
Benefits of AvalancheJS
- Ease of Use: Intuitive API design for quick integration.
- Comprehensive: Support for a wide range of functionalities.
- Community Support: Active community and extensive documentation.
Here’s an example of using AvalancheJS to create a transaction:
const avalanche = require("avalanche");
const { Avalanche, BinTools, Buffer, AVMAPI, KeyChain } = avalanche;
const myNetworkID = 1; // default is 1, we change it to 2 to work with our local network
const avalanche = new Avalanche("localhost", 9650, "http", myNetworkID);
const xchain = avalanche.XChain(); // returns a reference to the X-Chain used by AvalancheJS
const bintools = BinTools.getInstance();
const myKeychain = xchain.keyChain();
const myKeypair = myKeychain.makeKey();
const tx = {
sourceChain: "X-Chain",
destinationChain: "C-Chain",
amount: 1000,
asset: "AVAX"
};
async function main() {
const unsignedTx = await xchain.buildBaseTx(tx);
const signedTx = xchain.signTx(unsignedTx);
const txid = await xchain.issueTx(signedTx);
console.log(`Transaction ID: ${txid}`);
}
main().catch(console.error);
This script demonstrates how to create and issue a transaction using AvalancheJS, showcasing the simplicity and power of Avalanche’s developer tools.
Implementing Custom Virtual Machines
Avalanche’s architecture allows for the implementation of custom virtual machines (VMs), enabling developers to create tailored solutions for specific use cases.
Creating a Custom VM
To create a custom VM, developers can define the execution environment, consensus rules, and state management processes. This flexibility allows for the creation of highly specialized blockchains.
Benefits of Custom VMs
- Tailored Solutions: Create VMs optimized for specific applications.
- Innovation: Experiment with new consensus mechanisms and features.
- Integration: Seamlessly integrate custom VMs with the broader Avalanche network.
Here’s an outline of how to create a simple custom VM on Avalanche:
package main
import (
"fmt"
"github.com/ava-labs/avalanchego/snow/engine/snowman"
"github.com/ava-labs/avalanchego/vms/components/verify"
)
type CustomVM struct {
// Define VM state and variables
}
func (vm *CustomVM) Initialize(ctx *snow.Context, db database.Database, genesisBytes []byte, upgradeBytes []byte, configBytes []byte) error
// Initialize VM state
fmt.Println("Initializing Custom VM")
return nil
}
func (vm *CustomVM) Shutdown() error {
// Clean up resources
fmt.Println("Shutting down Custom VM")
return nil
}
func (vm *CustomVM) CreateBlock() (snowman.Block, error) {
// Logic to create a new block
fmt.Println("Creating a new block")
return nil, nil
}
func (vm *CustomVM) VerifyBlock(block snowman.Block) error {
// Logic to verify a block
fmt.Println("Verifying block")
return nil
}
func (vm *CustomVM) LastAccepted() (ids.ID, error) {
// Logic to return the last accepted block ID
fmt.Println("Fetching last accepted block ID")
return ids.ID{}, nil
}
// Main function to run the Custom VM
func main() {
// Register the custom VM with Avalanche
fmt.Println("Registering Custom VM")
vm := &CustomVM{}
if err := vm.Initialize(nil, nil, nil, nil, nil); err != nil {
fmt.Println("Failed to initialize VM:", err)
}
// Further implementation details would go here
}
This basic outline demonstrates the fundamental steps involved in creating a custom VM. You can expand this to include more detailed logic for block creation, verification, and state management.
Utilizing Avalanche’s Advanced APIs
Avalanche offers a comprehensive set of APIs that enable developers to interact with the network at a granular level. These APIs provide functionalities for asset management, transaction creation, network monitoring, and more.
Advanced API Features
- Asset Management: Create and manage digital assets with fine-grained control.
- Transaction APIs: Build, sign, and issue transactions programmatically.
- Network Monitoring: Access real-time data on network status, performance, and metrics.
Benefits of Advanced APIs
- Control: Fine-tune your applications with detailed API functionalities.
- Automation: Automate complex workflows and processes.
- Insights: Gain deep insights into network performance and behavior.
Here’s a more advanced example using Avalanche’s API to manage assets:
from avalanche import Avalanche
# Initialize Avalanche connection
avax = Avalanche("https://api.avax.network")
# Create a new asset
asset_params = {
"name": "MyToken",
"symbol": "MTK",
"initial_supply": 1000000,
"decimals": 18
}
asset_id = avax.create_asset(asset_params)
print(f"New Asset ID: {asset_id}")
# Check asset balance
balance = avax.get_balance("your-address", asset_id)
print(f"Balance of MyToken: {balance}")
This script showcases how to create a new asset and check its balance, illustrating the power and flexibility of Avalanche’s advanced APIs.
Integrating with Decentralized Finance (DeFi) Protocols
Avalanche’s robust infrastructure makes it an ideal platform for building and integrating with decentralized finance (DeFi) protocols. From lending and borrowing to decentralized exchanges, Avalanche supports a wide range of DeFi applications.
Building DeFi Protocols on Avalanche
Developers can leverage Avalanche’s smart contract capabilities and high throughput to build innovative DeFi solutions. The platform’s interoperability allows for seamless integration with other blockchains and DeFi ecosystems.
Benefits of DeFi on Avalanche
- Performance: High transaction throughput and low latency.
- Interoperability: Cross-chain capabilities for interacting with other DeFi platforms.
- Security: Robust consensus mechanism ensuring the integrity and security of DeFi protocols.
Here’s a simplified example of a DeFi lending contract in Solidity for the Avalanche C-Chain:
// SPDX-License-Identifier: MIT
pragma solidity ^0.8.0;
contract DeFiLending {
mapping(address => uint256) public balances;
mapping(address => uint256) public loans;
function deposit() public payable {
balances[msg.sender] += msg.value;
}
function borrow(uint256 amount) public {
require(balances[msg.sender] >= amount, "Insufficient balance");
loans[msg.sender] += amount;
balances[msg.sender] -= amount;
payable(msg.sender).transfer(amount);
}
function repay() public payable {
require(loans[msg.sender] >= msg.value, "Overpayment not allowed");
loans[msg.sender] -= msg.value;
}
function checkBalance() public view returns (uint256) {
return balances[msg.sender];
}
function checkLoan() public view returns (uint256) {
return loans[msg.sender];
}
}
This contract allows users to deposit funds, borrow against their deposits, and repay loans. It’s a simple example of how DeFi protocols can be implemented on Avalanche.
Enhancing Security with Avalanche’s Features
Security is paramount in any blockchain platform, and Avalanche provides several advanced features to ensure the integrity and safety of the network and its applications.
Security Features
- Threshold Signatures: Enhance the security of transactions and smart contracts.
- Native Support for Hardware Wallets: Secure your assets with hardware wallets like Ledger and Trezor.
- Dynamic Validator Set: The validator set can change dynamically, ensuring decentralization and security.
Benefits of Enhanced Security
- Protection: Safeguard against common attack vectors.
- Trust: Build confidence among users and stakeholders.
- Compliance: Meet regulatory requirements for security and data integrity.
Here’s a sample script to use a hardware wallet for signing transactions on Avalanche:
from avalanche import Avalanche
from hardware_wallet import HardwareWallet
# Initialize Avalanche connection
avax = Avalanche("https://api.avax.network")
# Connect to hardware wallet
wallet = HardwareWallet("Ledger")
# Sign transaction using hardware wallet
tx_params = {
"source_chain": "X-Chain",
"destination_chain": "C-Chain",
"amount": 1000,
"asset": "AVAX"
}
unsigned_tx = avax.build_tx(tx_params)
signed_tx = wallet.sign_tx(unsigned_tx)
tx_id = avax.issue_tx(signed_tx)
print(f"Transaction ID: {tx_id}")
This script demonstrates how to securely sign transactions using a hardware wallet, highlighting Avalanche’s commitment to security.
Conclusion
Avalanche is a powerful and versatile platform for building decentralized applications and enterprise blockchain solutions. Its advanced features, including the unique consensus protocol, customizable subnets, cross-chain functionality, and robust security measures, make it an ideal choice for developers and businesses alike.
By exploring these advanced capabilities, you can unlock the full potential of Avalanche and create innovative, high-performance blockchain applications. Whether you’re building DeFi protocols, deploying smart contracts, or creating custom virtual machines, Avalanche provides the tools and infrastructure to support your vision.
Disclaimer: The information provided in this article is for educational purposes only. It is not intended to be, nor should it be construed as, investment, financial, or legal advice. Always do your own research and consult with professionals before making any decisions related to blockchain and cryptocurrency projects.