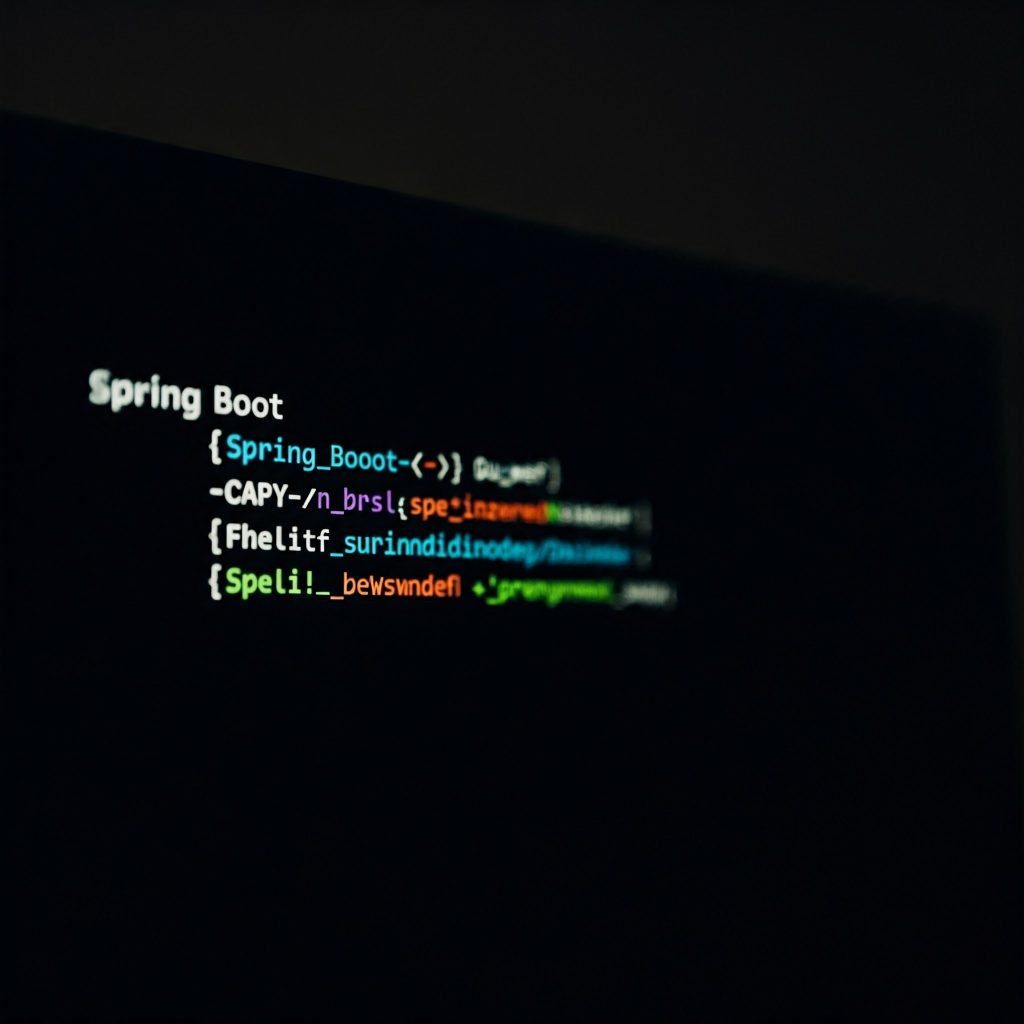
Boost Your Productivity Using Spring Boot CLI
Spring Boot is a powerful framework that simplifies the development of Java-based enterprise applications by offering a convention-over-configuration paradigm. Among its various tools, the Spring Boot Command Line Interface (CLI) stands out as an essential tool that can drastically improve productivity by enabling developers to create Spring applications without the need for complex setups. The Spring Boot CLI allows you to run, test, and deploy applications efficiently with minimal configuration, making it particularly useful for rapid development and prototyping.
In this comprehensive guide, we will delve into the details of how to master the Spring Boot CLI to enhance productivity. This blog will cover everything from installation and setup to advanced tips, with code examples to guide you along the way. By the end of this article, you’ll have a solid understanding of how to leverage the Spring Boot CLI to streamline your development process.
What is Spring Boot CLI?
The Spring Boot CLI is a command-line tool that allows developers to rapidly create, run, and manage Spring applications without having to write extensive configuration files. The CLI uses Groovy, a dynamic language for the Java platform, to write concise scripts that are automatically configured with Spring Boot’s default settings.
This flexibility enables developers to focus on business logic instead of boilerplate configuration, which significantly speeds up the development process. It is particularly useful for small prototypes and microservices that can be built quickly and expanded later if necessary.
Why Use Spring Boot CLI?
Using Spring Boot CLI comes with several advantages that make it a must-have tool for Java developers. Below are some of the key benefits:
Advantages | Description |
---|---|
Rapid Prototyping | Spring Boot CLI allows developers to create and run applications with minimal setup, enabling faster prototyping. |
Minimal Configuration | With built-in conventions and auto-configuration, the Spring Boot CLI reduces the need for manual configurations. |
Lightweight | As the CLI uses Groovy, scripts can be significantly shorter than traditional Java files, making it easier to write concise and readable code. |
Integrated Testing | You can quickly test applications via the CLI without setting up complex testing frameworks. |
Reduced Boilerplate Code | By leveraging Groovy and the CLI, developers can avoid writing repetitive boilerplate code, improving productivity. |
Flexible Deployment | The CLI enables quick deployment options, either locally or to cloud services, making it easier to go from development to production. |
Installing Spring Boot CLI
The first step to mastering Spring Boot CLI is installing it on your system. It is available on multiple platforms, including macOS, Linux, and Windows. Below are the steps to install Spring Boot CLI on different operating systems.
For macOS/Linux: Using SDKMAN!
SDKMAN! is the recommended tool for installing Spring Boot CLI on macOS and Linux. SDKMAN! simplifies the installation and version management of multiple SDKs and Java versions.
- Install SDKMAN! by running the following command in your terminal:
curl -s "https://get.sdkman.io" | bash
- After the installation is complete, run the following command to load SDKMAN!:
source "$HOME/.sdkman/bin/sdkman-init.sh"
- Install Spring Boot CLI using SDKMAN!:
sdk install springboot
- Verify the installation by checking the version:
spring --version
For Windows: Using the Spring Installer
- Download the Spring CLI Windows Installer from the official Spring website.
- Follow the instructions on the installer wizard to install the CLI.
- After installation, open the command prompt and verify the installation:
spring --version
Manual Installation
Alternatively, you can manually install the Spring Boot CLI by downloading the appropriate binaries from the Spring Boot GitHub repository and configuring your PATH
environment variable to include the Spring Boot CLI binary location.
Getting Started with Spring Boot CLI
Once installed, the Spring Boot CLI allows you to start developing applications right away. You can write Groovy scripts to create Spring Boot applications, which eliminates much of the configuration typically required.
Creating a Simple Spring Boot Application
Here’s a basic example of how to create a simple RESTful web service using the Spring Boot CLI:
- Create a new Groovy script file named
app.groovy
:
@RestController
class HelloController {
@RequestMapping("/")
String home() {
"Hello, Spring Boot CLI!"
}
}
- Run the application using the CLI:
spring run app.groovy
- The application will start, and you can navigate to
http://localhost:8080
in your browser to see the output:Hello, Spring Boot CLI!
This basic application demonstrates how simple it is to create a fully functioning Spring Boot application with just a few lines of code. The CLI takes care of setting up the necessary configuration, so you can focus on writing the business logic.
Key Spring Boot CLI Commands
The Spring Boot CLI provides several commands that are useful for running, testing, and deploying applications. Below are some of the most frequently used commands:
Command | Description |
---|---|
spring run <file> | Runs a Spring Groovy script. |
spring test <file> | Runs the tests for the provided Spring application. |
spring jar <jar-name> <file> | Packages the application into a JAR file. |
spring help | Displays a list of available commands and their descriptions. |
spring version | Shows the installed version of the Spring Boot CLI. |
spring init <project-name> | Initializes a new Spring Boot project using default settings or specified parameters. |
Example: Running an Application from Groovy Script
spring run myapp.groovy
This command starts the application by automatically configuring the embedded Tomcat server and running your code, all without needing additional configuration.
Creating a Spring Boot Project with CLI
In addition to running Groovy scripts, you can also use the Spring Boot CLI to initialize full Spring Boot projects. The spring init
command is particularly useful for setting up new projects with predefined dependencies.
Generating a Basic Spring Boot Project
To generate a new Spring Boot project, use the following command:
spring init --build=maven --dependencies=web,data-jpa,devtools my-spring-app
This command creates a Maven-based project with dependencies for Spring Web, JPA, and Spring Boot DevTools, stored in the my-spring-app
directory.
Explanation of Flags:
--build=maven
: Specifies Maven as the build system (can also begradle
).--dependencies=web,data-jpa,devtools
: Adds dependencies for Spring Web, JPA, and Spring Boot DevTools.my-spring-app
: The name of the generated project folder.
Directory Structure of the Generated Project
Folder | Description |
---|---|
/src/main/java | Contains the application’s source code. |
/src/main/resources | Holds configuration files like application.properties . |
/src/test/java | Contains the application’s test code. |
/pom.xml or build.gradle | The build configuration file, depending on whether Maven or Gradle was selected. |
The project can then be opened in any Integrated Development Environment (IDE) such as IntelliJ IDEA or Eclipse for further development.
Testing with Spring Boot CLI
Testing is a critical part of the software development lifecycle, and the Spring Boot CLI makes it easy to run tests for your application. You can either include test cases in your Groovy scripts or run tests from a preconfigured Spring Boot project.
Running Tests in a Spring Boot CLI Application
Let’s assume you have the following Groovy script that contains a basic unit test:
import org.junit.Test
import static org.junit.Assert.*
class SampleTest {
@Test
void testHome() {
def result = new HelloController().home()
assertEquals("Hello, Spring Boot CLI!", result)
}
}
You can run the test using the following command:
spring test app.groovy
The Spring Boot CLI will execute the test and display the results, giving you instant feedback on the correctness of your application.
Advanced Tips for Boosting Productivity
To truly master the Spring Boot CLI, here are some advanced tips that can help you work even faster and more efficiently.
1. Using Custom Starters
Spring Boot starters simplify dependency management. You can create custom starters to include frequently used dependencies, making project initialization faster.
spring init --build=maven --dependencies=my-custom-starter my-app
2. Automating Rebuilds
Spring Boot DevTools automatically restarts your application whenever you make code changes, reducing the time spent manually restarting it. This can be activated by including the devtools
dependency in your project.
3. Debugging with the CLI
The Spring Boot CLI supports debugging by passing additional JVM options. You can enable debugging by running:
spring run --
debug myapp.groovy
This allows you to step through the application and examine its execution more thoroughly.
Conclusion
Mastering the Spring Boot CLI is a crucial skill for any Java developer looking to improve productivity and streamline the development process. Whether you’re building prototypes, microservices, or full-scale enterprise applications, the Spring Boot CLI offers numerous benefits, from reducing boilerplate code to enabling rapid prototyping with minimal setup. By learning the commands, best practices, and advanced tips outlined in this guide, you can take full advantage of the Spring Boot CLI to boost your productivity and make your development workflow more efficient.
Disclaimer: The information provided in this blog post is accurate to the best of our knowledge at the time of writing. However, technologies and tools evolve rapidly. Please report any inaccuracies so we can correct them promptly.