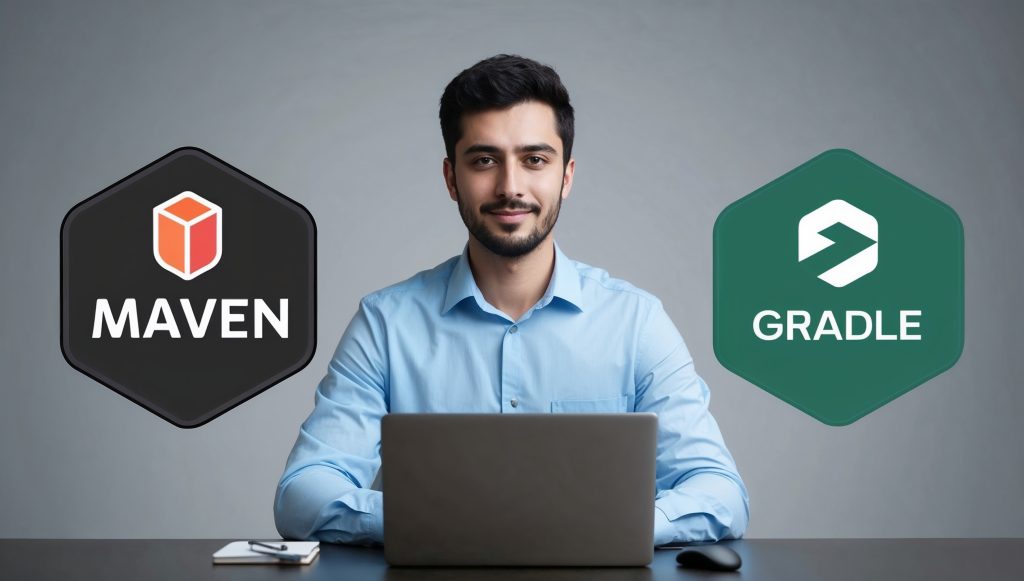
Build tools: Apache Maven and Gradle
Today, we’re diving into the exciting world of build tools – specifically, Apache Maven and Gradle. If you’ve been in the Java ecosystem for a while, you’ve probably heard these names tossed around. But what exactly are they, and why should you care? Well, buckle up, because we’re about to embark on a journey that’ll transform the way you manage your Java projects.
Imagine you’re building a house. You wouldn’t just start hammering away without a plan, right? You’d need blueprints, a list of materials, and a way to organize your work. That’s exactly what Maven and Gradle do for your Java projects. They’re like the master builders of the coding world, helping you manage dependencies, compile your code, run tests, and package your application – all with a few simple commands. But they’re not just about making your life easier (although they definitely do that). These tools are about bringing structure, consistency, and efficiency to your development process.
In this blog post, we’ll peel back the layers of both Maven and Gradle. We’ll explore their strengths, their quirks, and how they can supercharge your Java development workflow. Whether you’re a seasoned developer looking to optimize your build process or a newcomer trying to make sense of it all, there’s something here for everyone. So, let’s roll up our sleeves and get started!
Maven: The OG of Java Build Tools
What is Maven, anyway?
Apache Maven has been around since 2004, and it’s become something of a legend in the Java world. At its core, Maven is a project management and comprehension tool. But calling it just a “tool” is like calling a Swiss Army knife just a “blade.” Maven does so much more than simply build your project.
Think of Maven as your project’s personal assistant. It handles dependency management, so you don’t have to manually download and manage JAR files. It standardizes your project structure, making it easier for other developers to understand and contribute to your code. And it automates common tasks like compiling, testing, and packaging your application. All of this is done through a declarative approach using the Project Object Model (POM) file.
The POM: Maven’s Secret Sauce
The POM file is where the magic happens. It’s an XML file that contains information about your project and configuration details used by Maven to build it. Let’s take a look at a simple POM file:
<project xmlns="http://maven.apache.org/POM/4.0.0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>com.example</groupId>
<artifactId>my-awesome-project</artifactId>
<version>1.0-SNAPSHOT</version>
<dependencies>
<dependency>
<groupId>junit</groupId>
<artifactId>junit</artifactId>
<version>4.13.2</version>
<scope>test</scope>
</dependency>
</dependencies>
</project>
In this POM, we’re defining a project with a group ID, artifact ID, and version. We’re also declaring a dependency on JUnit for our tests. Maven uses this information to fetch the right dependencies, organize your project structure, and execute the build lifecycle.
Maven’s Build Lifecycle: A Well-Oiled Machine
One of Maven’s strengths is its standardized build lifecycle. This lifecycle consists of several phases, each responsible for a specific task in the build process. The main phases include:
- validate
- compile
- test
- package
- verify
- install
- deploy
When you run a Maven command like mvn package
, Maven executes all phases up to and including the “package” phase. This ensures that your code is compiled, tested, and packaged in a consistent manner every time.
Dependency Management: Say Goodbye to JAR Hell
Remember the days of manually downloading JAR files and adding them to your classpath? Maven makes those days a distant memory. With Maven’s dependency management system, you simply declare the libraries you need in your POM file, and Maven takes care of the rest. It downloads the required JARs (and their dependencies) from remote repositories, stores them in your local repository, and makes them available to your project.
This approach not only saves time but also helps prevent version conflicts and ensures that all developers on a project are using the same versions of dependencies. It’s like having a personal librarian who always knows exactly which books you need and where to find them.
Gradle: The New Kid on the Block
Introducing Gradle: Maven’s Hip Younger Sibling
While Maven has been the go-to build tool for Java projects for years, Gradle has been gaining popularity since its introduction in 2009. Gradle takes a different approach to build automation, offering more flexibility and power, especially for complex projects.
Gradle combines the best features of Apache Ant and Maven, while introducing a Groovy-based Domain Specific Language (DSL) for describing builds. This means you can write your build scripts in a more programmatic way, giving you greater control and flexibility over your build process.
Gradle Build Scripts: Where Code Meets Configuration
Instead of XML, Gradle uses a Groovy or Kotlin DSL for its build scripts. This allows for more expressive and concise build configurations. Let’s look at a simple Gradle build script:
plugins {
id 'java'
}
group 'com.example'
version '1.0-SNAPSHOT'
repositories {
mavenCentral()
}
dependencies {
testImplementation 'junit:junit:4.13.2'
}
test {
useJUnit()
}
This script does essentially the same thing as our Maven POM file earlier, but in a more readable and compact format. It applies the Java plugin, sets up the project details, declares a dependency on JUnit, and configures the test task to use JUnit.
Gradle’s Task-Based Model: Building Blocks of Automation
One of Gradle’s key features is its task-based model. Everything in Gradle is a task – compiling your code, running tests, generating documentation, you name it. These tasks can be customized, extended, and combined to create complex build processes tailored to your project’s needs.
For example, let’s say you want to add a custom task to your build:
task hello {
doLast {
println 'Hello, Gradle!'
}
}
Now you can run this task with gradle hello
, and it will print “Hello, Gradle!” to the console. This may seem simple, but it demonstrates the power and flexibility of Gradle’s task system. You can create tasks for anything from generating code to deploying your application to a server.
Incremental Builds: Gradle’s Speed Demon
One area where Gradle really shines is in its support for incremental builds. Gradle is smart enough to know which parts of your project have changed and only rebuilds what’s necessary. This can lead to significant time savings, especially on larger projects.
Gradle achieves this through its incremental task execution and build cache features. It tracks the inputs and outputs of each task and only re-executes a task if its inputs have changed. This means that if you’re working on a specific part of your project, you’re not wasting time rebuilding everything else.
Maven vs Gradle: Choosing Your Weapon
The Great Build Tool Showdown
Now that we’ve explored both Maven and Gradle, you might be wondering which one you should choose for your next project. The truth is, both tools are excellent choices, and the best one for you will depend on your specific needs and preferences.
Maven is like the reliable family sedan. It’s been around for a long time, has a huge ecosystem of plugins and integrations, and follows a “convention over configuration” approach that makes it easy to get started with. If you’re working on a straightforward Java project and value simplicity and standardization, Maven might be your best bet.
Gradle, on the other hand, is more like a high-performance sports car. It offers more flexibility and power, especially for complex multi-module projects or projects that require custom build logic. If you’re comfortable with a bit more complexity in exchange for greater control and faster build times, Gradle could be the way to go.
Performance: The Need for Speed
When it comes to performance, Gradle generally has the edge, especially for larger projects. Its incremental build feature and build cache can significantly reduce build times. Maven has made strides in this area with features like parallel builds, but Gradle still tends to be faster out of the box.
Here’s a simple Java class that we might use to benchmark our build tools:
public class BuildToolBenchmark {
public static void main(String[] args) {
long startTime = System.currentTimeMillis();
// Simulate some build tasks
for (int i = 0; i < 1000000; i++) {
Math.sqrt(i);
}
long endTime = System.currentTimeMillis();
System.out.println("Build time: " + (endTime - startTime) + " ms");
}
}
While this isn’t a real build process, it illustrates the point that build time can be a crucial factor in choosing your build tool, especially for larger projects.
Ecosystem and Community Support
Maven has been around longer and has a vast ecosystem of plugins and integrations. Almost any tool or framework you might want to use with Java has Maven support. Gradle is catching up quickly, but there are still some areas where Maven has more comprehensive support.
On the other hand, Gradle is the build tool of choice for Android development, which has led to a growing and vibrant community around it. Both tools have active communities and extensive documentation, so you’re unlikely to be left high and dry with either choice.
Learning Curve and Ease of Use
Maven’s XML-based configuration and convention-over-configuration approach make it relatively easy to get started with. If you’re new to build tools or prefer a more structured approach, Maven might feel more comfortable.
Gradle’s Groovy-based DSL can be more intuitive for those with programming experience, but it also means there’s more to learn. The flexibility Gradle offers comes at the cost of a steeper learning curve. However, once you’re comfortable with Gradle, you might find that it allows you to express your build logic more clearly and concisely.
Best Practices for Maven and Gradle
Keeping Your Builds Clean and Efficient
Regardless of whether you choose Maven or Gradle, there are some best practices that can help you get the most out of your build tool:
- Keep your dependencies up to date: Regularly review and update your project dependencies. Both Maven and Gradle have plugins that can help with this.
- Use version ranges carefully: While version ranges can help keep your dependencies up to date automatically, they can also lead to unexpected behavior if a dependency introduces breaking changes. Use them judiciously.
- Leverage the cache: Both Maven and Gradle use local caches to store dependencies. Ensure your CI/CD pipeline is configured to take advantage of this to speed up builds.
- Modularize your project: For larger projects, consider breaking your application into modules. This can help with maintainability and can speed up builds by allowing parallel execution.
- Write clean, maintainable build scripts: Just like your application code, your build scripts should be clean, well-documented, and maintainable. Avoid copy-pasting large blocks of configuration.
Optimizing Build Performance
Build performance can have a significant impact on developer productivity. Here are some tips to keep your builds fast:
- Use parallel execution: Both Maven and Gradle support parallel execution of tasks. Enable this feature to take advantage of multi-core processors.
- Minimize plugin usage: While plugins can add powerful features to your build, each one adds overhead. Use only the plugins you need.
- Profile your builds: Both Maven and Gradle offer ways to profile your build to identify bottlenecks. Use these tools to find and address performance issues.
- Optimize your test execution: Tests are often the most time-consuming part of a build. Consider using techniques like test parallelization or selectively running only affected tests.
Here’s an example of how you might configure parallel test execution in Gradle:
test {
maxParallelForks = Runtime.runtime.availableProcessors().intdiv(2) ?: 1
}
This configuration sets the number of parallel test processes to half the number of available processors (with a minimum of 1), which can significantly speed up test execution on multi-core machines.
Advanced Features and Integrations
Taking Your Build to the Next Level
Both Maven and Gradle offer advanced features that can help you tackle complex build scenarios and integrate with other tools in your development ecosystem.
Multi-Module Projects
For larger applications, you might want to split your project into multiple modules. Both Maven and Gradle excel at managing multi-module projects, but they approach it differently.
In Maven, you use a parent POM and child POMs to define the structure of your multi-module project. Here’s a simple example:
<!-- Parent POM -->
<project>
<modelVersion>4.0.0</modelVersion>
<groupId>com.example</groupId>
<artifactId>parent-project</artifactId>
<version>1.0</version>
<packaging>pom</packaging>
<modules>
<module>module1</module>
<module>module2</module>
</modules>
</project>
<!-- Child POM (in module1 directory) -->
<project>
<parent>
<groupId>com.example</groupId>
<artifactId>parent-project</artifactId>
<version>1.0</version>
</parent>
<artifactId>module1</artifactId>
</project>
Gradle, on the other hand, uses a settings.gradle file to define the structure of a multi-module project:
// settings.gradle
rootProject.name = 'parent-project'
include 'module1', 'module2'
// build.gradle in root directory
allprojects {
group = 'com.example'
version = '1.0'
}
// build.gradle in module1 directory
plugins {
id 'java'
}
Continuous Integration and Deployment
Both Maven and Gradle integrate well with popular CI/CD tools like Jenkins, Travis CI, and GitLab CI. They can be used to automate your entire build, test, and deployment pipeline.
For example, here’s a simple .gitlab-ci.yml file that uses Maven to build and test a project on GitLab CI:
image: maven:3.8.1-jdk-11
stages:
- build
- test
build:
stage: build
script:
- mvn compile
test:
stage: test
script:
- mvn test
And here’s a similar configuration using Gradle:
image: gradle:7.0-jdk11
stages:
- build
- test
build:
stage: build
script:
- gradle build -x test
test:
stage: test
script:
- gradle test
Custom Plugins and Extensions
Both Maven and Gradle allow you to create custom plugins to extend their functionality. This can be incredibly powerful for automating project-specific tasks or integrating with internal tools.
In Maven, you can create a custom plugin by implementing the Mojo interface. Here’s a simple example:
import org.apache.maven.plugin.AbstractMojo;
import org.apache.maven.plugin.MojoExecutionException;
import org.apache.maven.plugins.annotations.Mojo;
@Mojo(name = "say-hello")
public class HelloMojo extends AbstractMojo {
public void execute() throws MojoExecutionException {
getLog().info("Hello, Maven!");
}
}
In Gradle, you can create a custom task type or plugin. Here’s a simple custom task:
class HelloTask extends DefaultTask {
@TaskAction
def sayHello() {
println 'Hello, Gradle!'
}
}
// Use the custom task
task hello(type: HelloTask)
These examples barely scratch the surface of what’s possible with custom plugins and extensions. As you become more comfortable with your chosen build tool, exploring these advanced features can help you create more efficient and automated build processes.
Wrapping Up: The Future of Java Build Tools
As we’ve seen, both Maven and Gradle are powerful tools that can significantly improve your Java development workflow. They each have their strengths and weaknesses, and the choice between them often comes down to personal preference and project requirements.
Maven’s standardized approach and vast ecosystem make it a solid choice for many projects, especially those that follow conventional structures. Its learning curve is generally smoother, making it an excellent choice for teams new to build automation or those who prefer a more opinionated tool.
Gradle, with its flexibility and performance optimizations, shines in complex, custom build scenarios. Its programmable build scripts offer unparalleled customization options, and its incremental build feature can significantly speed up the development process for large projects.
The Road Ahead
As we look to the future, it’s clear that build tools will continue to play a crucial role in Java development. Both Maven and Gradle are actively developed, with new features and improvements being added regularly.
We’re likely to see continued focus on areas like:
- Build performance: As projects grow larger and more complex, the need for faster builds becomes even more critical. Expect to see further optimizations in areas like incremental builds, parallel execution, and caching.
- Cloud integration: With the increasing adoption of cloud technologies, build tools will likely offer better integration with cloud platforms for tasks like dependency resolution and artifact deployment.
- AI and machine learning: We might see the integration of AI to optimize build processes, predict build times, or even suggest improvements to build scripts.
- Enhanced dependency management: As the number of open-source libraries continues to grow, managing dependencies and ensuring security will become even more important. Build tools may offer more advanced features for dependency analysis and vulnerability detection.
- Support for modern Java features: As Java itself evolves, build tools will need to keep pace, supporting new language features and runtime optimizations.
Making Your Choice
So, which should you choose – Maven or Gradle? As with many things in software development, the answer is: it depends. Here are some guidelines:
- If you’re working on a relatively straightforward Java project and value convention and simplicity, Maven might be the way to go.
- If you’re building a complex, multi-module project, or if you need a high degree of build customization, Gradle could be the better choice.
- If you’re doing Android development, Gradle is the standard choice.
- If you’re joining an existing project, it’s usually best to stick with whatever build tool is already in use unless there’s a compelling reason to switch.
Remember, the most important thing is not which tool you choose, but how effectively you use it. Both Maven and Gradle are capable of handling most Java build scenarios you’re likely to encounter. The key is to invest time in learning your chosen tool well, understanding its best practices, and leveraging its features to create an efficient, reproducible build process.
As you embark on your next Java project, armed with the knowledge of these powerful build tools, remember that the goal is to spend less time fighting with your build process and more time writing great code. Whether you choose the tried-and-true Maven or the flexible Gradle, you’re setting yourself up for a smoother, more automated development workflow.
Happy building, and may your compiles always be clean and your tests always pass!
Disclaimer: This blog post is intended for informational purposes only. While we strive for accuracy, technologies and best practices in software development evolve rapidly. Always refer to the official documentation of Maven and Gradle for the most up-to-date information. If you notice any inaccuracies in this post, please report them so we can correct them promptly.