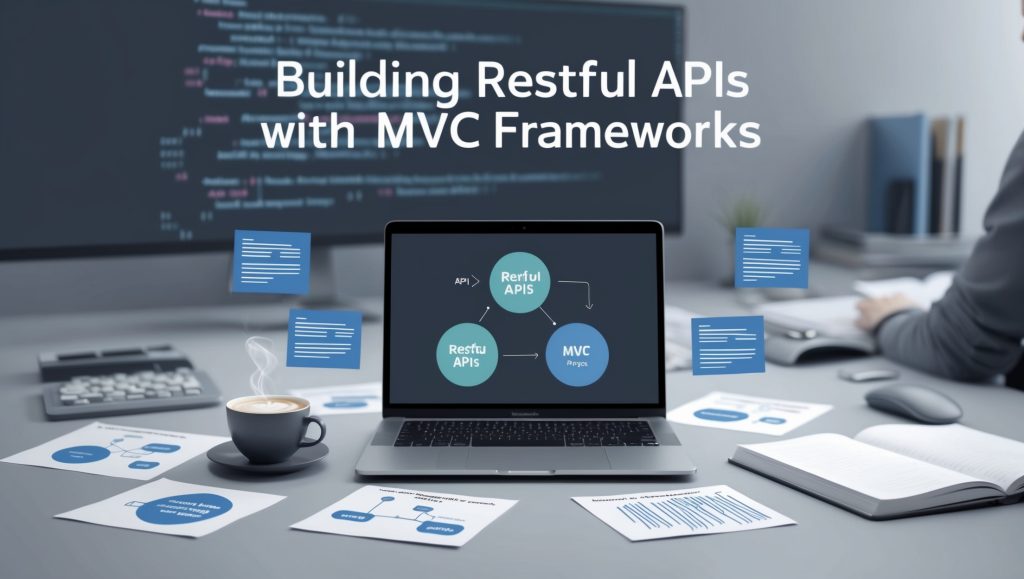
Building RESTful APIs with MVC Frameworks
The modern web development landscape demands robust, scalable, and maintainable APIs that can effectively serve diverse client applications. REST (Representational State Transfer) has emerged as the de facto standard for building web APIs, while the Model-View-Controller (MVC) architectural pattern continues to provide a solid foundation for organizing code in a structured and maintainable way. This comprehensive guide explores the intersection of RESTful APIs and MVC frameworks, offering practical insights into designing and implementing APIs that leverage MVC principles. We’ll examine best practices, common patterns, and real-world examples using popular frameworks in both Python and Java, providing you with the knowledge needed to build professional-grade APIs that stand the test of time.
Understanding RESTful APIs and MVC Architecture
REST architecture, introduced by Roy Fielding in 2000, has revolutionized how we design and implement web services. It emphasizes stateless communication, standardized interfaces, and resource-based interactions. The MVC pattern, on the other hand, provides a clear separation of concerns by dividing application logic into three distinct components: Models (data and business logic), Views (presentation layer), and Controllers (request handling and flow control). When combined, these architectural approaches create a powerful foundation for building scalable and maintainable APIs.
Key REST Principles
- Resource Identification Through URIs
- Uniform Interface
- Stateless Communication
- Client-Server Architecture
- Cacheable Responses
- Layered System
MVC Components in API Context
- Models: Handle data persistence, validation, and business logic
- Controllers: Process HTTP requests, coordinate with models, and prepare responses
- Views: Transform data into appropriate formats (JSON, XML, etc.)
Setting Up the Development Environment
Before diving into implementation details, let’s establish our development environment. We’ll explore two popular technology stacks: Python with Flask/Django and Java with Spring Boot. Both ecosystems offer robust MVC implementations and excellent support for building RESTful APIs.
Python Setup (Flask)
flask==2.0.1
flask-restful==0.3.9
flask-sqlalchemy==2.5.1
marshmallow==3.13.0
Java Setup (Spring Boot)
<!-- pom.xml -->
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
<version>2.6.3</version>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-jpa</artifactId>
<version>2.6.3</version>
</dependency>
</dependencies>
Designing the API Structure
A well-designed API structure is crucial for maintaining code organization and ensuring scalability. Let’s examine how to structure our API using MVC principles.
Project Structure (Python/Flask)
/api
/models
__init__.py
user.py
product.py
/controllers
__init__.py
user_controller.py
product_controller.py
/views
__init__.py
schemas.py
/services
__init__.py
auth_service.py
config.py
app.py
Project Structure (Java/Spring Boot)
/src/main/java/com/example/api
/model
User.java
Product.java
/controller
UserController.java
ProductController.java
/repository
UserRepository.java
ProductRepository.java
/service
UserService.java
ProductService.java
/dto
UserDTO.java
ProductDTO.java
Application.java
Implementing the Model Layer
The model layer forms the foundation of our API, representing the business entities and their relationships. Let’s implement a simple user management system to demonstrate model implementation in both Python and Java.
Python Model Implementation
from flask_sqlalchemy import SQLAlchemy
from datetime import datetime
db = SQLAlchemy()
class User(db.Model):
__tablename__ = 'users'
id = db.Column(db.Integer, primary_key=True)
username = db.Column(db.String(80), unique=True, nullable=False)
email = db.Column(db.String(120), unique=True, nullable=False)
created_at = db.Column(db.DateTime, default=datetime.utcnow)
def __init__(self, username, email):
self.username = username
self.email = email
def to_dict(self):
return {
'id': self.id,
'username': self.username,
'email': self.email,
'created_at': self.created_at.isoformat()
}
Java Model Implementation
// model/User.java
import javax.persistence.*;
import java.time.LocalDateTime;
@Entity
@Table(name = "users")
public class User {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
@Column(unique = true, nullable = false)
private String username;
@Column(unique = true, nullable = false)
private String email;
@Column(name = "created_at")
private LocalDateTime createdAt;
@PrePersist
protected void onCreate() {
createdAt = LocalDateTime.now();
}
// Getters and setters
}
Building the Controller Layer
Controllers handle HTTP requests, process input data, coordinate with services, and prepare responses. They serve as the entry point for API requests and implement the RESTful endpoint definitions.
Python Controller Implementation
from flask import Blueprint, request, jsonify
from models.user import User, db
user_bp = Blueprint('users', __name__)
@user_bp.route('/users', methods=['GET'])
def get_users():
users = User.query.all()
return jsonify([user.to_dict() for user in users])
@user_bp.route('/users', methods=['POST'])
def create_user():
data = request.get_json()
if not all(k in data for k in ('username', 'email')):
return jsonify({'error': 'Missing required fields'}), 400
user = User(username=data['username'], email=data['email'])
try:
db.session.add(user)
db.session.commit()
return jsonify(user.to_dict()), 201
except Exception as e:
db.session.rollback()
return jsonify({'error': str(e)}), 400
@user_bp.route('/users/<int:user_id>', methods=['GET'])
def get_user(user_id):
user = User.query.get_or_404(user_id)
return jsonify(user.to_dict())
Java Controller Implementation
// controller/UserController.java
import org.springframework.web.bind.annotation.*;
import org.springframework.http.ResponseEntity;
import java.util.List;
@RestController
@RequestMapping("/api/users")
public class UserController {
private final UserService userService;
public UserController(UserService userService) {
this.userService = userService;
}
@GetMapping
public ResponseEntity<List<UserDTO>> getAllUsers() {
return ResponseEntity.ok(userService.getAllUsers());
}
@PostMapping
public ResponseEntity<UserDTO> createUser(@RequestBody UserDTO userDTO) {
return ResponseEntity.status(201)
.body(userService.createUser(userDTO));
}
@GetMapping("/{id}")
public ResponseEntity<UserDTO> getUser(@PathVariable Long id) {
return ResponseEntity.ok(userService.getUserById(id));
}
}
Implementing Data Transfer Objects (DTOs) and Views
DTOs and views handle data transformation between the internal model representation and the external API format. They provide a clean separation between the data storage layer and the API contract.
Python Schema Implementation
from marshmallow import Schema, fields
class UserSchema(Schema):
id = fields.Int(dump_only=True)
username = fields.Str(required=True)
email = fields.Email(required=True)
created_at = fields.DateTime(dump_only=True)
user_schema = UserSchema()
users_schema = UserSchema(many=True)
Java DTO Implementation
// dto/UserDTO.java
import java.time.LocalDateTime;
public class UserDTO {
private Long id;
private String username;
private String email;
private LocalDateTime createdAt;
// Getters and setters
public static UserDTO fromEntity(User user) {
UserDTO dto = new UserDTO();
dto.setId(user.getId());
dto.setUsername(user.getUsername());
dto.setEmail(user.getEmail());
dto.setCreatedAt(user.getCreatedAt());
return dto;
}
}
Implementing Service Layer
The service layer encapsulates business logic and provides a clean interface between controllers and models. This separation helps maintain clean code and enables easy unit testing.
Python Service Implementation
from models.user import User, db
from views.schemas import user_schema
class UserService:
@staticmethod
def get_all_users():
return User.query.all()
@staticmethod
def create_user(data):
user = User(
username=data['username'],
email=data['email']
)
db.session.add(user)
db.session.commit()
return user
@staticmethod
def get_user_by_id(user_id):
return User.query.get_or_404(user_id)
Java Service Implementation
// service/UserService.java
import org.springframework.stereotype.Service;
import org.springframework.transaction.annotation.Transactional;
@Service
public class UserService {
private final UserRepository userRepository;
public UserService(UserRepository userRepository) {
this.userRepository = userRepository;
}
public List<UserDTO> getAllUsers() {
return userRepository.findAll()
.stream()
.map(UserDTO::fromEntity)
.collect(Collectors.toList());
}
@Transactional
public UserDTO createUser(UserDTO userDTO) {
User user = new User();
user.setUsername(userDTO.getUsername());
user.setEmail(userDTO.getEmail());
User savedUser = userRepository.save(user);
return UserDTO.fromEntity(savedUser);
}
}
API Documentation and Testing
Proper documentation and testing are crucial for maintaining and scaling your API. Here’s how to implement both using popular tools.
API Documentation with Swagger/OpenAPI
openapi: 3.0.0
info:
title: User Management API
version: 1.0.0
paths:
/api/users:
get:
summary: Get all users
responses:
'200':
description: Successful operation
content:
application/json:
schema:
type: array
items:
$ref: '#/components/schemas/User'
post:
summary: Create a new user
requestBody:
required: true
content:
application/json:
schema:
$ref: '#/components/schemas/UserInput'
responses:
'201':
description: User created successfully
Testing Implementation (Python)
import pytest
from app import create_app
from models.user import db, User
@pytest.fixture
def client():
app = create_app('testing')
with app.test_client() as client:
with app.app_context():
db.create_all()
yield client
db.session.remove()
db.drop_all()
def test_create_user(client):
response = client.post('/api/users', json={
'username': 'testuser',
'email': 'test@example.com'
})
assert response.status_code == 201
assert response.json['username'] == 'testuser'
Best Practices and Common Pitfalls
When building RESTful APIs with MVC frameworks, following best practices and avoiding common pitfalls is crucial for long-term success.
Best Practices
- Use appropriate HTTP methods for operations
- Implement proper error handling and validation
- Version your API
- Use meaningful HTTP status codes
- Implement rate limiting and security measures
- Cache responses when appropriate
- Follow consistent naming conventions
Common Pitfalls to Avoid
- Breaking REST principles
- Mixing concerns across layers
- Inadequate error handling
- Poor documentation
- Lack of input validation
- Insufficient testing
- Ignoring security considerations
Security Considerations
Implementing proper security measures is crucial for protecting your API and its users. Here’s a basic implementation of authentication and authorization.
Python Security Implementation
from functools import wraps
from flask import request, jsonify
import jwt
def token_required(f):
@wraps(f)
def decorated(*args, **kwargs):
token = request.headers.get('Authorization')
if not token:
return jsonify({'error': 'Token is missing'}), 401
try:
data = jwt.decode(token, app.config['SECRET_KEY'])
current_user = User.query.get(data['user_id'])
except:
return jsonify({'error': 'Token is invalid'}), 401
return f(current_user, *args, **kwargs)
return decorated
Java Security Implementation
// config/SecurityConfig.java
import org.springframework.security.config.annotation.web.builders.HttpSecurity;
import org.springframework.security.config.annotation.web.configuration.EnableWebSecurity;
@EnableWebSecurity
public class SecurityConfig extends WebSecurityConfigurerAdapter {
@Override
protected void configure(HttpSecurity http) throws Exception {
http
.csrf().disable()
.authorizeRequests()
.antMatchers("/api/public/**").permitAll()
.anyRequest().authenticated()
.and()
.addFilter(new JWTAuthenticationFilter(authenticationManager()))
.addFilter(new JWTAuthorizationFilter(authenticationManager()));
}
}
Conclusion
Building RESTful APIs using MVC frameworks requires careful planning, proper implementation of architectural patterns, and adherence to best practices. By following the guidelines and examples provided in this guide, you can create robust, maintainable, and secure APIs that serve your application’s needs effectively. Remember to regularly review and update your implementation as new best practices emerge and your application’s requirements evolve.
Disclaimer: The code examples and implementations provided in this blog post are for educational purposes and may require additional modification for production use. While we strive for accuracy, technology evolves rapidly, and some information may become outdated. Please review the latest documentation for the frameworks and libraries mentioned. If you find any inaccuracies or have suggestions for improvements, please report them to our editorial team at editorialteam@felixrante.com.