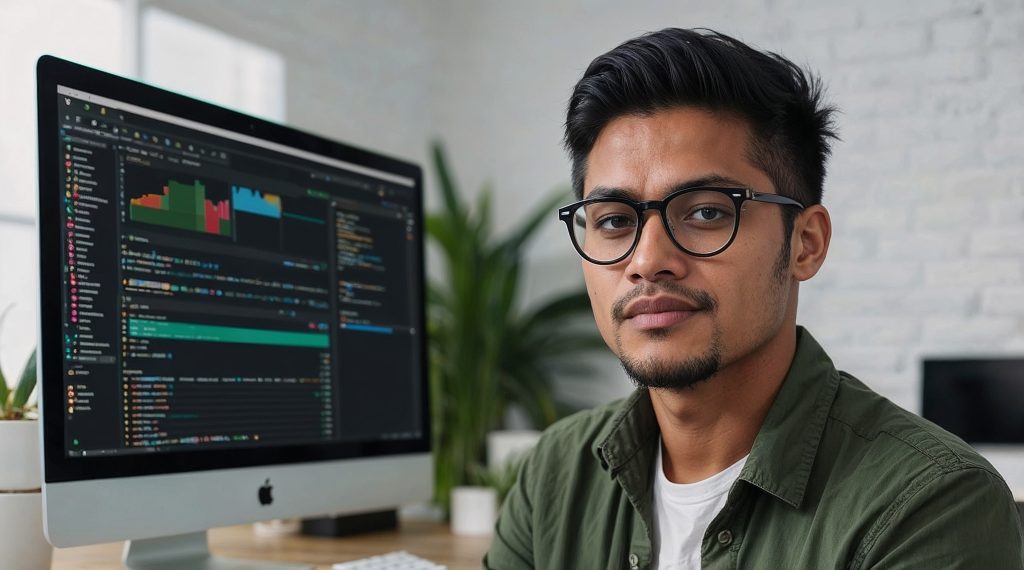
Building Web Applications using Spring Boot for Beginners
Welcome to the exciting world of Spring Boot! If you’re a beginner looking to dive into Java web development, you’ve come to the right place. Spring Boot has revolutionized the way we build web applications, making the process faster, easier, and more enjoyable than ever before. In this comprehensive guide, we’ll explore what Spring Boot is, why it’s become so popular, and how you can use it to create powerful web applications. Whether you’re a complete novice or have some experience with Java, this blog post will help you get up to speed with Spring Boot and set you on the path to becoming a proficient web developer.
What is Spring Boot?
Spring Boot is a powerful framework that simplifies the process of building production-ready applications using the Spring ecosystem. But what exactly does that mean? Let’s break it down. Spring Boot is an extension of the popular Spring Framework, which has been a cornerstone of Java development for years. While Spring itself is incredibly versatile and feature-rich, it can sometimes be complex to set up and configure, especially for beginners. This is where Spring Boot comes in โ it takes care of much of the boilerplate code and configuration, allowing you to focus on writing your application logic instead of worrying about how to wire everything together.
Key features of Spring Boot:
- Opinionated defaults: Spring Boot comes with sensible default configurations, reducing the need for extensive setup.
- Standalone applications: It allows you to create self-contained Java applications that can be run with a simple
java -jar
command. - Embedded servers: Spring Boot includes embedded web servers like Tomcat, making deployment a breeze.
- Auto-configuration: It automatically configures your application based on the dependencies you’ve added to your project.
- Production-ready features: Spring Boot includes built-in features for metrics, health checks, and externalized configuration.
These features combine to make Spring Boot an excellent choice for both beginners and experienced developers. It simplifies the development process without sacrificing the power and flexibility that the Spring ecosystem is known for.
Setting Up Your Development Environment
Before we dive into building web applications with Spring Boot, let’s make sure you have everything you need to get started. Setting up your development environment is crucial, and fortunately, it’s pretty straightforward with Spring Boot. Here’s what you’ll need:
- Java Development Kit (JDK): Spring Boot 3.x requires Java 17 or later. Download and install the latest version of the JDK from the official Oracle website or use an open-source alternative like OpenJDK.
- Integrated Development Environment (IDE): While you can use any text editor, an IDE will make your life much easier. Popular choices include IntelliJ IDEA, Eclipse, and Visual Studio Code. These IDEs offer excellent support for Spring Boot development.
- Build Tool: Spring Boot projects typically use either Maven or Gradle as a build tool. We’ll use Maven in our examples, but feel free to use Gradle if you prefer.
- Spring Initializr: This is a web-based tool that helps you generate a basic Spring Boot project structure. You can access it at https://start.spring.io/.
Once you have these tools installed, you’re ready to create your first Spring Boot project. Let’s walk through the process using Spring Initializr:
- Go to https://start.spring.io/ in your web browser.
- Choose “Maven Project” and “Java” as the language.
- Select the latest stable version of Spring Boot.
- Fill in the project metadata (Group, Artifact, Name, Description).
- Add the “Spring Web” dependency.
- Click “Generate” to download the project ZIP file.
Extract the ZIP file, and you’ll have a basic Spring Boot project ready to go. Open this project in your IDE, and you’re all set to start building your first web application with Spring Boot!
Your First Spring Boot Application
Now that we have our development environment set up and a basic project structure, let’s create a simple “Hello, World!” web application. This will help you understand the basics of how Spring Boot works and how easy it is to get a web application up and running.
First, let’s look at the main application class that Spring Initializr generated for us:
package com.example.demo;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
@SpringBootApplication
public class DemoApplication {
public static void main(String[] args) {
SpringApplication.run(DemoApplication.class, args);
}
}
This class is the entry point of our Spring Boot application. The @SpringBootApplication
annotation is a convenience annotation that adds all of the following:
@Configuration
: Tags the class as a source of bean definitions for the application context.@EnableAutoConfiguration
: Tells Spring Boot to start adding beans based on classpath settings, other beans, and various property settings.@ComponentScan
: Tells Spring to look for other components, configurations, and services in the current package.
Now, let’s add a simple controller to handle web requests. Create a new Java class called HelloController
in the same package as your main application class:
package com.example.demo;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RestController;
@RestController
public class HelloController {
@GetMapping("/")
public String hello() {
return "Hello, World! Welcome to Spring Boot!";
}
}
This controller is very simple:
- The
@RestController
annotation tells Spring that this class is a controller where every method returns a domain object instead of a view. - The
@GetMapping("/")
annotation tells Spring to use this method to handle GET requests to the root URL (“/”). - The
hello()
method returns a simple string that will be displayed in the browser.
That’s it! You now have a fully functional web application. To run it, you can either use your IDE’s run feature or open a terminal in your project directory and run:
./mvnw spring-boot:run
Once the application starts, open a web browser and go to http://localhost:8080
. You should see the message “Hello, World! Welcome to Spring Boot!” displayed.
Congratulations! You’ve just created and run your first Spring Boot web application. It’s amazing how little code we needed to write to get a web server up and running, isn’t it? This is the power of Spring Boot โ it takes care of all the configuration and setup behind the scenes, allowing you to focus on writing your application logic.
Understanding Spring Boot’s Magic
You might be wondering how Spring Boot manages to do so much with so little code. The secret lies in its auto-configuration feature and the concept of “convention over configuration.” Let’s dive a bit deeper into how this works.
Auto-configuration
When you start a Spring Boot application, it automatically configures your application based on the dependencies you’ve added to your project. For example, when you added the “Spring Web” dependency, Spring Boot automatically:
- Embedded a Tomcat web server in your application.
- Set up Spring MVC for handling web requests.
- Configured a DispatcherServlet to route requests to the appropriate controllers.
All of this happens without you having to write a single line of configuration code. Spring Boot examines your classpath and the beans you’ve defined, then makes reasonable guesses about how you want to configure your application.
Convention over Configuration
Spring Boot follows the principle of “convention over configuration.” This means it provides sensible defaults for most settings, which you can override if needed. For example:
- By default, Spring Boot looks for static resources (like HTML, CSS, and JavaScript files) in the
/static
,/public
,/resources
, and/META-INF/resources
directories of your classpath. - If you add a database dependency, Spring Boot will try to auto-configure a connection to the database using properties defined in your
application.properties
orapplication.yml
file.
This approach significantly reduces the amount of boilerplate configuration code you need to write, especially for common scenarios.
Building a RESTful API with Spring Boot
Now that we understand the basics, let’s build something a bit more complex โ a RESTful API. RESTful APIs are a common way to expose data and functionality over HTTP, and Spring Boot makes it incredibly easy to create them.
For this example, let’s create a simple API for managing a list of books. We’ll create endpoints to list all books, get a specific book, add a new book, update a book, and delete a book.
First, let’s create a Book
class to represent our data model:
package com.example.demo;
public class Book {
private Long id;
private String title;
private String author;
// Constructor
public Book(Long id, String title, String author) {
this.id = id;
this.title = title;
this.author = author;
}
// Getters and setters
public Long getId() { return id; }
public void setId(Long id) { this.id = id; }
public String getTitle() { return title; }
public void setTitle(String title) { this.title = title; }
public String getAuthor() { return author; }
public void setAuthor(String author) { this.author = author; }
}
Next, let’s create a BookController
to handle our API requests:
package com.example.demo;
import org.springframework.web.bind.annotation.*;
import java.util.ArrayList;
import java.util.List;
import java.util.Optional;
@RestController
@RequestMapping("/api/books")
public class BookController {
private List<Book> books = new ArrayList<>();
public BookController() {
books.add(new Book(1L, "To Kill a Mockingbird", "Harper Lee"));
books.add(new Book(2L, "1984", "George Orwell"));
books.add(new Book(3L, "Pride and Prejudice", "Jane Austen"));
}
@GetMapping
public List<Book> getAllBooks() {
return books;
}
@GetMapping("/{id}")
public Book getBook(@PathVariable Long id) {
return books.stream()
.filter(book -> book.getId().equals(id))
.findFirst()
.orElseThrow(() -> new RuntimeException("Book not found"));
}
@PostMapping
public Book addBook(@RequestBody Book book) {
book.setId((long) (books.size() + 1));
books.add(book);
return book;
}
@PutMapping("/{id}")
public Book updateBook(@PathVariable Long id, @RequestBody Book updatedBook) {
Book book = getBook(id);
book.setTitle(updatedBook.getTitle());
book.setAuthor(updatedBook.getAuthor());
return book;
}
@DeleteMapping("/{id}")
public void deleteBook(@PathVariable Long id) {
books.removeIf(book -> book.getId().equals(id));
}
}
Let’s break down what’s happening in this controller:
@RestController
indicates that this class is a controller where every method returns a domain object instead of a view.@RequestMapping("/api/books")
sets the base path for all endpoints in this controller.- We’re using a simple in-memory list to store our books. In a real application, you’d typically use a database.
@GetMapping
,@PostMapping
,@PutMapping
, and@DeleteMapping
specify which HTTP methods each endpoint should respond to.@PathVariable
is used to extract values from the URL path.@RequestBody
is used to automatically deserialize the request body into a Java object.
With this controller in place, you now have a fully functional RESTful API! You can test it using tools like cURL, Postman, or even your web browser (for GET requests).
Working with Databases in Spring Boot
While our in-memory book list works for demonstration purposes, most real-world applications need to persist data in a database. Spring Boot makes working with databases incredibly easy, thanks to its auto-configuration capabilities and the Spring Data project.
Let’s modify our book example to use a database. We’ll use H2, an in-memory database, for simplicity, but you can easily switch to other databases like MySQL or PostgreSQL by changing the dependencies and configuration.
First, add the following dependencies to your pom.xml
file:
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-jpa</artifactId>
</dependency>
<dependency>
<groupId>com.h2database</groupId>
<artifactId>h2</artifactId>
<scope>runtime</scope>
</dependency>
Now, let’s modify our Book
class to make it a JPA entity:
package com.example.demo;
import javax.persistence.Entity;
import javax.persistence.GeneratedValue;
import javax.persistence.GenerationType;
import javax.persistence.Id;
@Entity
public class Book {
@Id
@GeneratedValue(strategy = GenerationType.AUTO)
private Long id;
private String title;
private String author;
// Constructors, getters, and setters
}
Next, create a repository interface for handling database operations:
package com.example.demo;
import org.springframework.data.jpa.repository.JpaRepository;
public interface BookRepository extends JpaRepository<Book, Long> {
}
Finally, update the BookController
to use the repository:
package com.example.demo;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.web.bind.annotation.*;
import java.util.List;
@RestController
@RequestMapping("/api/books")
public class BookController {
@Autowired
private BookRepository bookRepository;
@GetMapping
public List<Book> getAllBooks() {
return bookRepository.findAll();
}
@GetMapping("/{id}")
public Book getBook(@PathVariable Long id) {
return bookRepository.findById(id)
.orElseThrow(() -> new RuntimeException("Book not found"));
}
@PostMapping
public Book addBook(@RequestBody Book book) {
return bookRepository.save(book);
}
@PutMapping("/{id}")
public Book updateBook(@PathVariable Long id, @RequestBody Book updatedBook) {
Book book = getBook(id);
book.setTitle(updatedBook.getTitle());
book.setAuthor(updatedBook.getAuthor());
return bookRepository.save(book);
}
@DeleteMapping("/{id}")
public void deleteBook(@PathVariable Long id) {
bookRepository.deleteById(id);
}
}
With these changes, your application is now using a database to store and retrieve books. Spring Boot automatically configures the database connection and creates the necessary tables based on your entity classes.
Adding Validation to Your Spring Boot Application
As your application grows, you’ll want to ensure that the data you’re working with is valid. Spring Boot integrates seamlessly with Java’s Bean Validation API, making it easy to add validation to your entities and controller methods.
First, add the validation dependency to your pom.xml
:
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-validation</artifactId>
</dependency>
Now, let’s add some validation to our Book
entity:
package com.example.demo;
import javax.persistence.Entity;
import javax.persistence.GeneratedValue;
import javax.persistence.GenerationType;
import javax.persistence.Id;
import javax.validation.constraints.NotBlank;
import javax.validation.constraints.Size;
@Entity
public class Book {
@Id
@GeneratedValue(strategy = GenerationType.AUTO)
private Long id;
@NotBlank(message = "Title is required")
@Size(min = 1, max = 100, message = "Title must be between 1 and 100 characters")
private String title;
@NotBlank(message = "Author is required")
@Size(min = 1, max = 100, message = "Author must be between 1 and 100 characters")
private String author;
// Constructors, getters, and setters
}
To use these validations in our controller, we need to add the @Valid
annotation to the method parameters we want to validate:
@PostMapping
public ResponseEntity addBook(@Valid @RequestBody Book book) {
Book savedBook = bookRepository.save(book);
return ResponseEntity.created(URI
return ResponseEntity.created(URI.create("/api/books/" + savedBook.getId())).body(savedBook);
}
@PutMapping("/{id}")
public ResponseEntity updateBook(@PathVariable Long id, @Valid @RequestBody Book updatedBook) {
Book book = bookRepository.findById(id)
.orElseThrow(() -> new RuntimeException("Book not found"));
book.setTitle(updatedBook.getTitle());
book.setAuthor(updatedBook.getAuthor());
Book savedBook = bookRepository.save(book);
return ResponseEntity.ok(savedBook);
}
Now, if a client tries to create or update a book with invalid data, Spring Boot will automatically return a 400 Bad Request response with details about the validation errors.
To handle these validation errors more gracefully, you can create a global exception handler:
java
package com.example.demo;
import org.springframework.http.HttpStatus;
import org.springframework.http.ResponseEntity;
import org.springframework.web.bind.MethodArgumentNotValidException;
import org.springframework.web.bind.annotation.ControllerAdvice;
import org.springframework.web.bind.annotation.ExceptionHandler;
import java.util.HashMap;
import java.util.Map;
@ControllerAdvice
public class GlobalExceptionHandler {
@ExceptionHandler(MethodArgumentNotValidException.class)
public ResponseEntity<Object> handleValidationExceptions(MethodArgumentNotValidException ex) {
Map<String, String> errors = new HashMap<>();
ex.getBindingResult().getAllErrors().forEach((error) -> {
String fieldName = ((FieldError) error).getField();
String errorMessage = error.getDefaultMessage();
errors.put(fieldName, errorMessage);
});
return new ResponseEntity<>(errors, HttpStatus.BAD_REQUEST);
}
}
This exception handler will catch validation errors and return a JSON response with details about each validation failure.
Securing Your Spring Boot Application
Security is a crucial aspect of any web application. Spring Boot makes it easy to add security features to your application using Spring Security. Let’s add basic authentication to our book API.
First, add the Spring Security dependency to your `pom.xml`:
org.springframework.boot spring-boot-starter-security
Now, create a simple security configuration:
package com.example.demo;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import org.springframework.security.config.annotation.web.builders.HttpSecurity;
import org.springframework.security.config.annotation.web.configuration.EnableWebSecurity;
import org.springframework.security.core.userdetails.User;
import org.springframework.security.core.userdetails.UserDetails;
import org.springframework.security.core.userdetails.UserDetailsService;
import org.springframework.security.provisioning.InMemoryUserDetailsManager;
import org.springframework.security.web.SecurityFilterChain;
@Configuration
@EnableWebSecurity
public class SecurityConfig {
@Bean
public SecurityFilterChain securityFilterChain(HttpSecurity http) throws Exception {
http
.authorizeRequests()
.antMatchers(HttpMethod.GET, "/api/books/**").permitAll()
.anyRequest().authenticated()
.and()
.httpBasic();
return http.build();
}
@Bean
public UserDetailsService userDetailsService() {
UserDetails user = User.withDefaultPasswordEncoder()
.username("user")
.password("password")
.roles("USER")
.build();
return new InMemoryUserDetailsManager(user);
}
}
This configuration does a few things: 1. It allows unauthenticated access to GET requests on the `/api/books` endpoints. 2. It requires authentication for all other requests. 3. It sets up HTTP Basic authentication. 4. It creates an in-memory user with the username “user” and password “password”. Now, if you try to create, update, or delete a book, you’ll be prompted for credentials. You can provide the username “user” and password “password” to authenticate. ### Testing Your Spring Boot Application Testing is an essential part of developing robust applications. Spring Boot provides excellent support for testing, making it easy to write unit tests and integration tests for your application. Let’s write a simple test for our `BookController`. First, add the test dependency to your `pom.xml` if it’s not already there:
org.springframework.boot spring-boot-starter-test test
Now, create a test class for the `BookController`:
package com.example.demo;
import org.junit.jupiter.api.Test;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.boot.test.autoconfigure.web.servlet.AutoConfigureMockMvc;
import org.springframework.boot.test.context.SpringBootTest;
import org.springframework.http.MediaType;
import org.springframework.test.web.servlet.MockMvc;
import static org.springframework.test.web.servlet.request.MockMvcRequestBuilders.*; import static org.springframework.test.web.servlet.result.MockMvcResultMatchers.*;
@SpringBootTest
@AutoConfigureMockMvc
public class BookControllerTest {
@Autowired
private MockMvc mockMvc;
@Test
public void testGetAllBooks() throws Exception {
mockMvc.perform(get("/api/books"))
.andExpect(status().isOk())
.andExpect(content().contentType(MediaType.APPLICATION_JSON));
}
@Test
public void testCreateBook() throws Exception {
String bookJson = "{\"title\":\"Test Book\",\"author\":\"Test Author\"}";
mockMvc.perform(post("/api/books")
.contentType(MediaType.APPLICATION_JSON)
.content(bookJson))
.andExpect(status().isCreated())
.andExpect(jsonPath("$.title").value("Test Book"))
.andExpect(jsonPath("$.author").value("Test Author"));
}
}
These tests use Spring’s MockMvc
to simulate HTTP requests to our controller. The testGetAllBooks
method tests the GET endpoint, while testCreateBook
tests the POST endpoint.
To run these tests, you can use your IDE’s test runner or run ./mvnw test
from the command line.
Conclusion
Congratulations! You’ve now created a fully functional web application with Spring Boot. We’ve covered a lot of ground, from setting up a basic application to working with databases, adding validation, implementing security, and writing tests.
Spring Boot’s power lies in its ability to simplify the development process without sacrificing flexibility. As you continue to explore Spring Boot, you’ll discover even more features that can help you build robust, scalable applications quickly and efficiently.
Remember, this is just the beginning. Spring Boot offers many more features, such as actuator endpoints for monitoring your application, support for caching, easy integration with message queues, and much more. As you become more comfortable with the basics we’ve covered here, don’t hesitate to dive deeper into Spring Boot’s documentation and explore its more advanced features.
Happy coding, and may your Spring Boot journey be filled with exciting discoveries and successful projects!
Disclaimer: This blog post is intended for educational purposes only. While we strive for accuracy, technologies and best practices in software development can change rapidly. Always refer to the official Spring Boot documentation for the most up-to-date information. If you notice any inaccuracies in this post, please report them so we can correct them promptly.