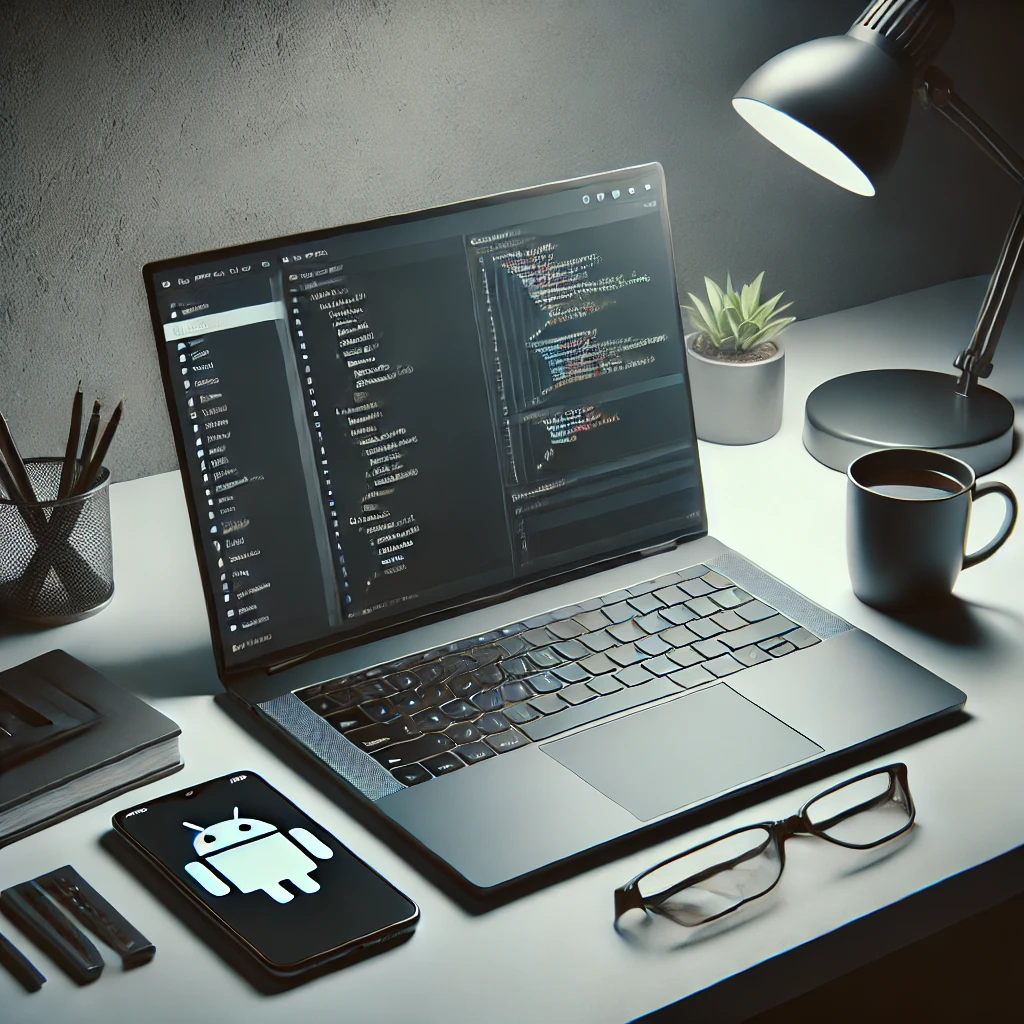
Building Your First Android App with Java: A Beginner’s Guide
Are you ready to dive into the exciting world of Android app development? Whether you’re a coding novice or an experienced programmer looking to expand your skills, creating your first Android app with Java can be an incredibly rewarding experience. In this comprehensive guide, we’ll walk you through the process step-by-step, from setting up your development environment to publishing your app on the Google Play Store. So, grab a cup of coffee, fire up your computer, and let’s embark on this thrilling journey together!
Why Choose Android and Java?
Before we jump into the nitty-gritty of app development, let’s take a moment to understand why Android and Java make such a powerful combination. Android is the world’s most popular mobile operating system, powering billions of devices worldwide. This massive user base translates to incredible opportunities for developers like you. Java, on the other hand, has been the primary language for Android development since the platform’s inception. Its robust ecosystem, extensive libraries, and strong community support make it an excellent choice for beginners and experienced developers alike.
Android’s open-source nature and flexibility allow developers to create a wide range of applications, from simple utilities to complex, feature-rich apps. Java’s object-oriented programming paradigm aligns perfectly with Android’s architecture, making it easier to create modular, maintainable code. Moreover, the wealth of resources available for Java developers, including tutorials, forums, and third-party libraries, can significantly accelerate your learning curve and help you overcome challenges along the way.
Setting Up Your Development Environment
Installing Android Studio
The first step in your Android development journey is setting up Android Studio, the official Integrated Development Environment (IDE) for Android app development. Android Studio provides a comprehensive set of tools and features specifically designed to streamline the app creation process. To get started, follow these steps:
- Visit the official Android Studio website (developer.android.com/studio) and download the latest version for your operating system.
- Run the installer and follow the on-screen instructions to complete the installation process.
- During the installation, make sure to select the option to install the Android SDK (Software Development Kit) as well.
- Once the installation is complete, launch Android Studio and follow the initial setup wizard to configure your development environment.
Android Studio’s intuitive interface and powerful features will become your best friends as you embark on your app development journey. Take some time to familiarize yourself with the layout, including the project explorer, code editor, and various tool windows. Don’t worry if it feels overwhelming at first – we’ll guide you through the essential components as we build our app.
Configuring the Android SDK
With Android Studio installed, it’s time to ensure you have the necessary SDK components for your target Android versions. The SDK Manager allows you to download and manage these components:
- In Android Studio, go to Tools > SDK Manager.
- In the SDK Platforms tab, select the Android versions you want to target (e.g., Android 11, Android 12).
- Switch to the SDK Tools tab and make sure you have the latest versions of essential tools like Android SDK Build-Tools and Android Emulator.
- Click “Apply” to download and install the selected components.
Having the right SDK components installed ensures that you can develop and test your app for various Android versions, maximizing its compatibility and reach.
Creating Your First Android Project
Starting a New Project
Now that your development environment is set up, it’s time to create your first Android project. Android Studio makes this process straightforward:
- Launch Android Studio and click on “New Project” from the welcome screen.
- Choose “Empty Activity” as your project template.
- Give your project a name (e.g., “MyFirstAndroidApp”) and select a package name (e.g., “com.example.myfirstandroidapp”).
- Choose Java as the programming language and select your minimum SDK version (e.g., API 21: Android 5.0).
- Click “Finish” to create the project.
Android Studio will generate the basic project structure for you, including essential files like MainActivity.java and activity_main.xml. These files will serve as the foundation for your app’s functionality and user interface.
Understanding the Project Structure
Take a moment to explore the project structure in the Project view. You’ll notice several key directories:
- app/manifests: Contains the AndroidManifest.xml file, which defines your app’s package name, components, and permissions.
- app/java: Houses your Java source code files.
- app/res: Contains resources like layouts, images, and strings.
Familiarizing yourself with this structure will help you navigate your project more efficiently as it grows in complexity.
Building the User Interface
Designing Your App’s Layout
The user interface is the face of your app, so let’s start by creating a simple yet functional layout. We’ll use XML to define our UI components in the activity_main.xml file:
- Open the activity_main.xml file in the layout editor.
- Switch to the “Code” view to edit the XML directly.
- Replace the existing code with the following:
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical"
android:padding="16dp">
<EditText
android:id="@+id/editTextName"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:hint="Enter your name" />
<Button
android:id="@+id/buttonGreet"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginTop="16dp"
android:text="Greet" />
<TextView
android:id="@+id/textViewGreeting"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginTop="16dp"
android:textSize="18sp" />
</LinearLayout>
This layout creates a simple form with an input field for the user’s name, a button to trigger the greeting, and a text view to display the greeting message.
Understanding Layout Components
Let’s break down the key components of our layout:
- LinearLayout: A container that arranges its child views in a single column or row.
- EditText: An input field for user text entry.
- Button: A clickable element that triggers an action.
- TextView: A view that displays text to the user.
Each component has attributes like android:layout_width and android:layout_height that define its size and position within the parent layout. The android:id attribute assigns a unique identifier to each view, which we’ll use to interact with them in our Java code.
Adding Functionality with Java
Implementing the Greeting Logic
Now that we have our user interface in place, let’s add some functionality to make our app interactive. We’ll implement a simple greeting feature that displays a personalized message when the user enters their name and clicks the “Greet” button.
Open the MainActivity.java file and replace its contents with the following code:
package com.example.myfirstandroidapp;
import android.os.Bundle;
import android.view.View;
import android.widget.Button;
import android.widget.EditText;
import android.widget.TextView;
import androidx.appcompat.app.AppCompatActivity;
public class MainActivity extends AppCompatActivity {
private EditText editTextName;
private Button buttonGreet;
private TextView textViewGreeting;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
// Initialize views
editTextName = findViewById(R.id.editTextName);
buttonGreet = findViewById(R.id.buttonGreet);
textViewGreeting = findViewById(R.id.textViewGreeting);
// Set click listener for the button
buttonGreet.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
greetUser();
}
});
}
private void greetUser() {
String name = editTextName.getText().toString().trim();
if (!name.isEmpty()) {
String greeting = "Hello, " + name + "! Welcome to your first Android app!";
textViewGreeting.setText(greeting);
} else {
textViewGreeting.setText("Please enter your name.");
}
}
}
Let’s break down this code to understand what’s happening:
- We declare private variables for our UI components (EditText, Button, and TextView).
- In the onCreate() method, we initialize these variables using findViewById() to connect them to the corresponding XML elements.
- We set a click listener on the “Greet” button that calls the greetUser() method when clicked.
- The greetUser() method retrieves the entered name, constructs a greeting message, and sets it as the text of our TextView.
Running and Testing Your App
Using the Android Emulator
Now that we have a functional app, it’s time to see it in action! Android Studio provides a built-in emulator that allows you to run and test your app on various virtual devices. Here’s how to use it:
- Click on the “Run” button (green play icon) in the toolbar.
- If you haven’t created an Android Virtual Device (AVD) yet, you’ll be prompted to do so. Follow the wizard to create a new AVD, selecting a device definition and system image that matches your target API level.
- Once the AVD is created (or if you already have one), select it from the list and click “OK” to launch the emulator.
- Wait for the emulator to boot up and your app to install and launch.
You should now see your app running in the emulator. Try entering your name and clicking the “Greet” button to see the greeting message appear.
Debugging and Troubleshooting
As you develop your app, you’ll inevitably encounter bugs or unexpected behavior. Android Studio offers powerful debugging tools to help you identify and fix issues:
- Set breakpoints in your code by clicking in the left margin of the editor next to the line numbers.
- Run your app in debug mode by clicking the “Debug” button (bug icon) instead of the “Run” button.
- When the app hits a breakpoint, you can step through the code, inspect variables, and analyze the call stack to understand what’s happening.
Don’t be discouraged if you encounter errors – debugging is an essential part of the development process and a valuable skill to develop.
Enhancing Your App
Adding More Features
Now that you have a basic working app, let’s add a few more features to make it more interesting and showcase some additional Android development concepts.
1. Saving User Preferences
Let’s add the ability to save the user’s name so they don’t have to enter it every time they open the app. We’ll use SharedPreferences, a simple key-value storage system provided by Android:
Add the following methods to your MainActivity class:
private static final String PREFS_NAME = "MyAppPrefs";
private static final String KEY_NAME = "user_name";
private void saveUserName(String name) {
SharedPreferences prefs = getSharedPreferences(PREFS_NAME, MODE_PRIVATE);
SharedPreferences.Editor editor = prefs.edit();
editor.putString(KEY_NAME, name);
editor.apply();
}
private String loadUserName() {
SharedPreferences prefs = getSharedPreferences(PREFS_NAME, MODE_PRIVATE);
return prefs.getString(KEY_NAME, "");
}
Now, modify your onCreate() method to load the saved name when the app starts:
@Override
protected void onCreate(Bundle savedInstanceState) {
// ... (previous code)
// Load saved name
String savedName = loadUserName();
if (!savedName.isEmpty()) {
editTextName.setText(savedName);
greetUser();
}
}
And update the greetUser() method to save the name when greeting:
private void greetUser() {
String name = editTextName.getText().toString().trim();
if (!name.isEmpty()) {
saveUserName(name);
String greeting = "Hello, " + name + "! Welcome to your first Android app!";
textViewGreeting.setText(greeting);
} else {
textViewGreeting.setText("Please enter your name.");
}
}
2. Adding a Menu
Let’s add a simple menu with an option to clear the saved name. First, create a new XML file called menu_main.xml in the res/menu directory:
<?xml version="1.0" encoding="utf-8"?>
<menu xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto">
<item
android:id="@+id/action_clear_name"
android:title="Clear Name"
app:showAsAction="never" />
</menu>
Now, add the following methods to your MainActivity class:
@Override
public boolean onCreateOptionsMenu(Menu menu) {
getMenuInflater().inflate(R.menu.menu_main, menu);
return true;
}
@Override
public boolean onOptionsItemSelected(MenuItem item) {
int id = item.getItemId();
if (id == R.id.action_clear_name) {
clearUserName();
return true;
}
return super.onOptionsItemSelected(item);
}
private void clearUserName() {
editTextName.setText("");
textViewGreeting.setText("");
saveUserName("");
}
These additions create a menu with a “Clear Name” option that resets the app state when selected.
Polishing Your App
Adding Styles and Themes
To give your app a more polished look, you can customize its appearance using styles and themes. Create a new file called styles.xml in the res/values directory:
<resources>
<style name="AppTheme" parent="Theme.AppCompat.Light.DarkActionBar">
<item name="colorPrimary">@color/colorPrimary</item>
<item name="colorPrimaryDark">@color/colorPrimaryDark</item>
<item name="colorAccent">@color/colorAccent</item>
</style>
<style name="ButtonStyle" parent="Widget.AppCompat.Button">
<item name="android:background">@color/colorAccent</item>
<item name="android:textColor">@android:color/white</item>
</style>
</resources>
And update your colors.xml file in the res/values directory:
<?xml version="1.0" encoding="utf-8"?>
<resources>
<color name="colorPrimary">#3F51B5</color>
<color name="colorPrimaryDark">#303F9F</color>
<color name="colorAccent">#FF4081</color>
</resources>
Now, apply the ButtonStyle to your “Greet” button in activity_main.xml:
<Button
android:id="@+id/buttonGreet"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginTop="16dp"
android:text="Greet"
style="@style/ButtonStyle" />
These changes will give your app a more cohesive and attractive appearance.
Publishing Your App
Preparing for Release
Before you can share your app with the world, you need to prepare it for release:
- Generate a signed APK:
- Go to Build > Generate Signed Bundle / APK.
- Follow the wizard to create a new keystore or use an existing one.
- Choose a build variant (usually “release”) and finish the process.
- Test your release build thoroughly on multiple devices or emulators to ensure everything works as expected.
Uploading to Google Play Store
To publish your app on the Google Play Store:
- Create a Google Play Developer account (there’s a one-time registration fee).
- Access the Google Play Console and click “Create Application.”
- Fill in your app’s details, including title, description, screenshots, and pricing.
- Upload your signed APK or Android App Bundle.
- Set up your app’s content rating and pricing.
- Submit your app for review.
Remember that the review process can take several days, and Google may request changes before approving your app.
Conclusion
Congratulations! You’ve just created your first Android app with Java and taken the first steps towards becoming an Android developer. This journey has introduced you to essential concepts like:
- Setting up your development environment with Android Studio
- Creating a user interface with XML layouts
- Implementing app logic with Java
- Running and debugging your app using the Android Emulator
- Enhancing your app with additional features like data persistence and menus
- Polishing your app’s appearance with styles and themes
- Preparing your app for release and publishing on the Google Play Store
Remember, this is just the beginning of your Android development journey. There’s so much more to explore, from advanced UI designs and animations to integrating APIs and working with databases. As you continue to learn and experiment, you’ll discover the incredible potential of Android app development and the endless possibilities it offers.
Don’t be afraid to dive deeper into topics that interest you, explore the official Android documentation, and participate in developer communities. The world of Android development is vast and ever-evolving, offering exciting opportunities for creativity and innovation.
Next Steps in Your Android Development Journey
As you build upon the foundation laid in this tutorial, consider exploring these areas to further enhance your skills:
1. Material Design
Dive into Google’s Material Design guidelines to create visually appealing and intuitive user interfaces. Implement material components and transitions to give your app a modern, professional look.
2. Data Storage and Databases
Learn how to work with SQLite databases using Room, Android’s recommended database solution. This will allow you to store and manage larger amounts of structured data in your apps.
3. Networking and APIs
Explore how to make network requests and integrate third-party APIs into your apps. Libraries like Retrofit and OkHttp can simplify this process and open up a world of possibilities for your apps.
4. Android Jetpack
Familiarize yourself with Android Jetpack, a set of libraries, tools, and architectural guidance to help you build robust, maintainable apps. Components like ViewModel, LiveData, and Navigation can significantly improve your app’s architecture and user experience.
5. Kotlin Programming Language
While we focused on Java in this tutorial, consider learning Kotlin, Google’s preferred language for Android development. Kotlin offers modern language features and can coexist with Java in your projects.
6. Testing and Performance Optimization
Develop skills in writing unit tests and UI tests for your apps. Learn how to profile your app’s performance and optimize it for better user experience and battery efficiency.
7. Publishing and Monetization Strategies
Explore different strategies for monetizing your apps, such as in-app purchases, subscriptions, or ad integration. Learn about app store optimization (ASO) to improve your app’s visibility on the Google Play Store.
Remember, the key to becoming a proficient Android developer is consistent practice and a willingness to learn. Don’t hesitate to take on challenging projects, contribute to open-source initiatives, or even start building that app idea you’ve been dreaming about. With each project, you’ll gain valuable experience and insights that will help you grow as a developer.
As you embark on this exciting journey, stay curious, be persistent, and most importantly, enjoy the process of bringing your ideas to life through Android app development. The skills you’ve learned today are just the beginning of what promises to be an rewarding and dynamic career in mobile app development.
Happy coding, and may your first Android app be the start of many more to come!
Disclaimer: This blog post is intended for educational purposes only. While we strive to provide accurate and up-to-date information, the field of Android development is rapidly evolving. Always refer to the official Android documentation for the most current best practices and guidelines. The code examples provided are simplified for illustrative purposes and may not include all necessary error handling or optimizations for production use. If you notice any inaccuracies or have suggestions for improvement, please report them so we can correct them promptly.