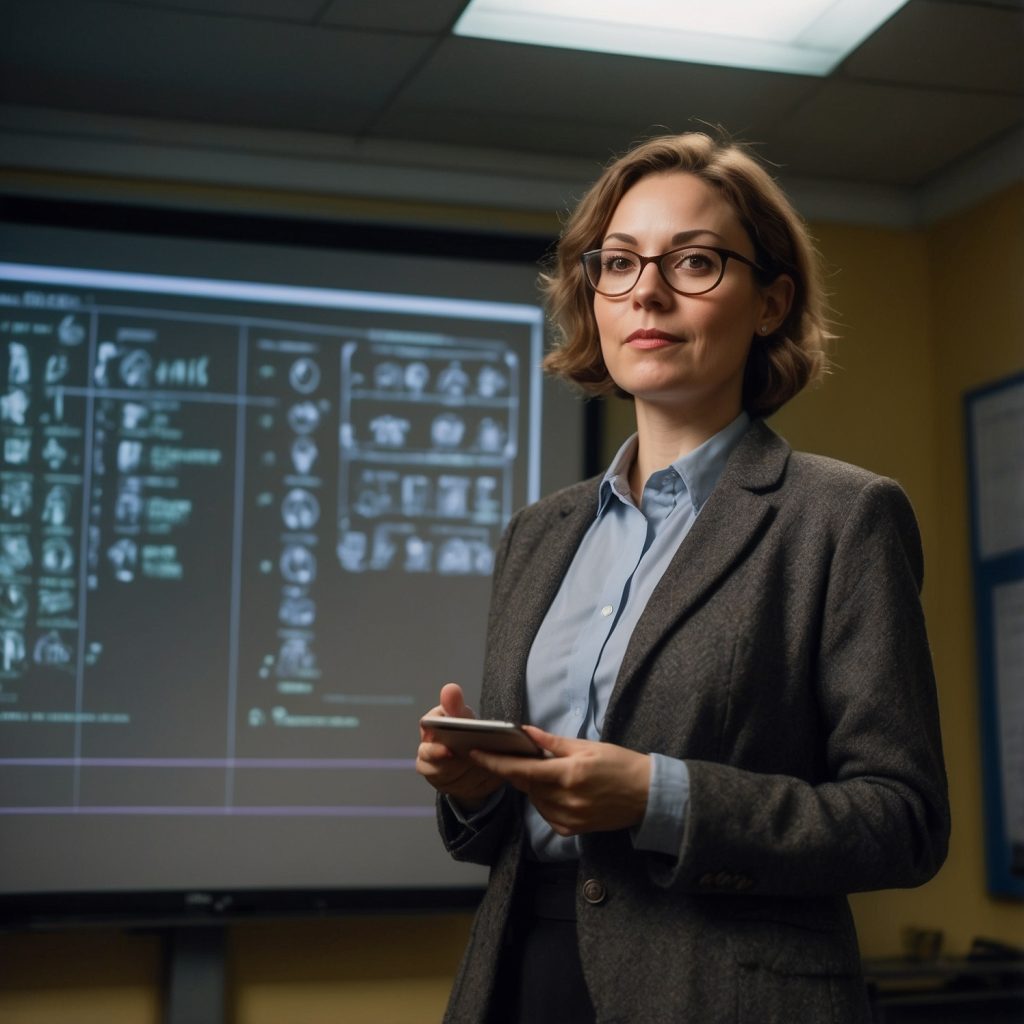
Building Your First Mobile App: A Step-by-Step Guide
Building a mobile app can seem like a daunting task, especially for beginners. However, with the right guidance and tools, you can create a functional and visually appealing app. This guide will walk you through the entire process, from idea conception to deployment on app stores. We’ll cover essential topics, including setting up your development environment, designing your app, writing the code, and testing your application.
Getting Started: Setting Up Your Development Environment
Before you start building your app, you need to set up your development environment. This involves choosing the right tools and platforms that suit your needs.
Choosing Your Platform and Tools
First, decide whether you want to develop for iOS, Android, or both. For iOS development, you’ll need a Mac computer with Xcode installed. For Android development, you can use a Windows, Mac, or Linux machine with Android Studio installed. If you want to develop for both platforms, consider using a cross-platform development tool like Flutter or React Native.
Installing Xcode for iOS Development
Xcode is the official IDE for iOS development. You can download it from the Mac App Store. Once installed, Xcode provides all the tools you need to build, test, and debug your app.
// Install Xcode via the Mac App Store
$ open -a "App Store" https://apps.apple.com/us/app/xcode/id497799835?mt=12
Installing Android Studio for Android Development
Android Studio is the official IDE for Android development. Download it from the official website and follow the installation instructions.
// Download and install Android Studio
$ https://developer.android.com/studio
Setting Up Flutter
Flutter is a popular framework for building cross-platform apps. To install Flutter, download it from the Flutter website and add it to your system path.
// Clone the Flutter repository from GitHub
$ git clone https://github.com/flutter/flutter.git -b stable
// Add Flutter to your path
$ export PATH="$PATH:`pwd`/flutter/bin"
Setting Up React Native
React Native allows you to build mobile apps using JavaScript and React. To install React Native, you’ll need Node.js, npm, and the React Native CLI.
// Install Node.js and npm
$ brew install node
// Install the React Native CLI
$ npm install -g react-native-cli
Planning Your App: Ideation and Design
Before you start coding, it’s essential to plan your app thoroughly. This includes defining its purpose, features, and user interface design.
Defining the Purpose and Features
Begin by identifying the problem your app will solve or the need it will fulfill. Define the core features that will make your app useful and appealing to users. Create a list of these features and prioritize them based on their importance and complexity.
Creating Wireframes and Mockups
Wireframes are simple sketches of your app’s screens and their layout. They help you visualize the app’s structure and flow. Tools like Sketch, Figma, and Adobe XD are great for creating wireframes and high-fidelity mockups.
Example Wireframe for a Simple To-Do App
- Home Screen: Displays a list of tasks.
- Add Task Screen: Allows users to add a new task.
- Task Detail Screen: Displays details of a selected task.
Building the User Interface
With your wireframes and mockups ready, it’s time to start building your app’s user interface (UI).
Building the UI with Flutter
Flutter uses a widget-based approach to build the UI. Here’s an example of a simple “Hello World” app in Flutter:
import 'package:flutter/material.dart';
void main() => runApp(MyApp());
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
appBar: AppBar(
title: Text('Hello World App'),
),
body: Center(
child: Text('Hello, World!'),
),
),
);
}
}
Building the UI with React Native
React Native uses a combination of JavaScript and JSX to build the UI. Here’s an example of a simple “Hello World” app in React Native:
import React from 'react';
import { Text, View } from 'react-native';
const App = () => {
return (
<View style={{ flex: 1, justifyContent: 'center', alignItems: 'center' }}>
<Text>Hello, World!</Text>
</View>
);
};
export default App;
Adding Functionality to Your App
Now that your UI is in place, it’s time to add functionality to your app. This involves writing the logic that makes your app work.
Handling User Input in Flutter
To handle user input in Flutter, you can use TextField widgets and manage their state with TextEditingController.
import 'package:flutter/material.dart';
void main() => runApp(MyApp());
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
home: HomeScreen(),
);
}
}
class HomeScreen extends StatefulWidget {
@override
_HomeScreenState createState() => _HomeScreenState();
}
class _HomeScreenState extends State<HomeScreen> {
final TextEditingController _controller = TextEditingController();
void _showInput() {
showDialog(
context: context,
builder: (context) {
return AlertDialog(
content: Text('You typed: ${_controller.text}'),
);
},
);
}
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text('Input Handling Example'),
),
body: Padding(
padding: const EdgeInsets.all(16.0),
child: Column(
children: [
TextField(
controller: _controller,
decoration: InputDecoration(labelText: 'Type something'),
),
SizedBox(height: 20),
ElevatedButton(
onPressed: _showInput,
child: Text('Show Input'),
),
],
),
),
);
}
}
Handling User Input in React Native
In React Native, you can use TextInput components and manage their state with React hooks.
import React, { useState } from 'react';
import { Text, TextInput, View, Button, Alert } from 'react-native';
const App = () => {
const [text, setText] = useState('');
const showAlert = () => {
Alert.alert('You typed: ' + text);
};
return (
<View style={{ padding: 16 }}>
<TextInput
style={{ height: 40, borderColor: 'gray', borderWidth: 1, marginBottom: 20 }}
placeholder="Type something"
onChangeText={text => setText(text)}
value={text}
/>
<Button title="Show Input" onPress={showAlert} />
</View>
);
};
export default App;
Connecting to a Backend
Most mobile apps need to communicate with a backend server to fetch and store data. This can be done using APIs.
Setting Up a Simple Backend with Node.js
We’ll create a simple REST API using Node.js and Express.
const express = require('express');
const app = express();
const port = 3000;
app.use(express.json());
let tasks = [
{ id: 1, title: 'First Task' },
{ id: 2, title: 'Second Task' },
];
app.get('/tasks', (req, res) => {
res.json(tasks);
});
app.post('/tasks', (req, res) => {
const newTask = { id: tasks.length + 1, title: req.body.title };
tasks.push(newTask);
res.status(201).json(newTask);
});
app.listen(port, () => {
console.log(`Server running at http://localhost:${port}`);
});
Connecting Your Flutter App to the Backend
Use the http package to make API requests in Flutter.
import 'package:flutter/material.dart';
import 'package:http/http.dart' as http;
import 'dart:convert';
void main() => runApp(MyApp());
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
home: TaskScreen(),
);
}
}
class TaskScreen extends StatefulWidget {
@override
_TaskScreenState createState() => _TaskScreenState();
}
class _TaskScreenState extends State<TaskScreen> {
List tasks = [];
Future<void> fetchTasks() async {
final response = await http.get('http://localhost:3000/tasks');
if (response.statusCode == 200) {
setState(() {
tasks = json.decode(response.body);
});
} else {
throw Exception('Failed to load tasks');
}
}
@override
void initState() {
super.initState();
fetchTasks();
}
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text('Tasks'),
),
body: ListView.builder(
itemCount: tasks.length,
itemBuilder: (context, index) {
return ListTile(
title: Text(tasks[index]['title']),
);
},
),
);
}
}
Connecting Your React Native App to the Backend
Use the fetch API to make API requests in React Native.
import React, { useEffect, useState } from 'react';
import { Text, View, FlatList, StyleSheet } from 'react-native';
const App = () => {
const [tasks, setTasks] = useState([]);
const fetchTasks = async () => {
try {
const response = await fetch('http://localhost:3000/tasks');
const data = await response.json();
setTasks(data);
} catch (error) {
console.error('Error fetching tasks:', error);
}
};
useEffect(() => {
fetchTasks();
}, []);
return (
Tasks item.id.toString()} renderItem={({ item }) => {item.title}} />
);
};
const styles = StyleSheet.create({
container: {
flex: 1,
justifyContent: 'center',
padding: 16,
},
header: {
fontSize: 24,
marginBottom: 20,
},
item: {
padding: 10,
fontSize: 18,
height: 44,
},
});
export default App;
### Testing Your App
Testing is a crucial part of the development process. It ensures that your app is working as expected and is free of bugs.
**Testing in Flutter**
Flutter provides a robust testing framework that includes unit tests, widget tests, and integration tests.
**Unit Testing in Flutter**
Here's an example of a simple unit test in Flutter:
Testing in React Native
React Native uses Jest for testing JavaScript code and React components.
Unit Testing in React Native
Here’s an example of a simple unit test in React Native using Jest:
const capitalize = (input) => {
if (!input) return input;
return input[0].toUpperCase() + input.slice(1);
};
test('String should be capitalized', () => {
expect(capitalize('hello')).toBe('Hello');
expect(capitalize('world')).toBe('World');
});
Deploying Your App
After testing and ensuring that everything works correctly, it’s time to deploy your app to the app stores.
Deploying to the Apple App Store
To deploy your iOS app, you need an Apple Developer account. Follow these steps to submit your app:
1. Create an App Store Connect account: Log in to App Store Connect with your Apple ID and create an account.
2. Archive your app: In Xcode, select your project and choose “Product” > “Archive”.
3. Upload to App Store Connect: Once archived, click “Distribute App” and follow the prompts to upload your app.
4. Submit for review: In App Store Connect, provide the necessary information about your app and submit it for review.
Deploying to the Google Play Store
To deploy your Android app, you need a Google Developer account. Follow these steps to submit your app:
1. Create a Google Developer account: Sign in to the Google Play Console with your Google account and create a developer account.
2. Generate a signed APK: In Android Studio, select “Build” > “Generate Signed APK”.
3. Upload to Google Play Console: Follow the instructions in the Google Play Console to upload your APK.
4. Submit for review: Provide the necessary information about your app and submit it for review.
Maintaining Your App
Once your app is live, your work isn’t done. Regular maintenance is necessary to keep your app running smoothly and to meet user expectations.
Collecting User Feedback
Gather feedback from users to identify bugs and areas for improvement. This can be done through in-app feedback forms, app store reviews, and social media.
Releasing Updates
Regularly release updates to fix bugs, add new features, and improve performance. Ensure that you thoroughly test each update before release to avoid introducing new issues.
Monitoring App Performance
Use analytics tools like Google Analytics, Firebase, or Flurry to monitor your app’s performance. Track key metrics such as user engagement, crash reports, and retention rates to understand how users interact with your app and identify areas for improvement.
Example Code for Integrating Firebase Analytics in Flutter
import 'package:firebase_core/firebase_core.dart';
import 'package:firebase_analytics/firebase_analytics.dart';
void main() async {
WidgetsFlutterBinding.ensureInitialized();
await Firebase.initializeApp();
runApp(MyApp());
}
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
home: HomeScreen(),
);
}
}
class HomeScreen extends StatelessWidget {
final FirebaseAnalytics analytics = FirebaseAnalytics();
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text('Firebase Analytics Example'),
),
body: Center(
child: ElevatedButton(
onPressed: () {
analytics.logEvent(name: 'button_pressed', parameters: null);
},
child: Text('Press me'),
),
),
);
}
}
Example Code for Integrating Firebase Analytics in React Native
import analytics from '@react-native-firebase/analytics';
import React from 'react';
import { Button, View } from 'react-native';
const App = () => {
const logEvent = async () => {
await analytics().logEvent('button_press', {
id: 1,
item: 'button',
description: 'A button press',
});
};
return (
<View style={{ flex: 1, justifyContent: 'center', alignItems: 'center' }}>
<Button title="Press me" onPress={logEvent} />
</View>
);
};
export default App;
Building your first mobile app is a rewarding experience that combines creativity, logic, and problem-solving. By following this step-by-step guide, you can go from an idea to a fully functional app available on the app stores. Remember to start with a clear plan, choose the right tools, and follow best practices in design, development, testing, and deployment. With persistence and continuous learning, you’ll be able to build more complex and sophisticated apps in the future. Happy coding!