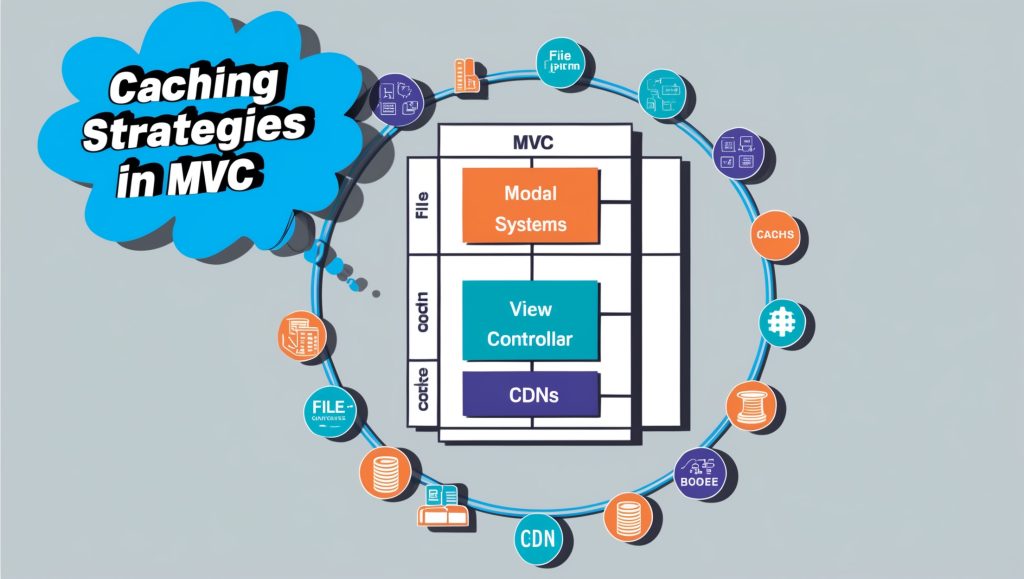
Caching Strategies in MVC
In modern web development, performance optimization stands as a crucial factor in delivering exceptional user experiences. As applications grow in complexity and user base, the need for efficient data retrieval and processing becomes paramount. Model-View-Controller (MVC) architecture, while providing excellent separation of concerns and maintainability, can benefit significantly from strategic caching implementations. This comprehensive guide explores various caching strategies within the MVC paradigm, demonstrating how properly implemented caching mechanisms can dramatically improve application performance, reduce server load, and enhance user satisfaction. We’ll delve into different caching levels, from in-memory to distributed caching, and provide practical implementations in both Python and Java frameworks.
Understanding Caching in MVC Architecture
The MVC pattern separates an application into three distinct components: Model (data and business logic), View (presentation layer), and Controller (request handling and coordination). Each of these components presents unique opportunities for caching optimization. The primary goal of caching in MVC is to store frequently accessed data in a faster access medium, reducing the need for expensive database queries, complex calculations, or resource-intensive operations. When implemented correctly, caching can significantly reduce response times and server load while improving scalability.
Types of Caching in MVC Applications
In-Memory Caching
In-memory caching stores data directly in the application’s memory space, providing the fastest possible access times. This approach is particularly effective for small to medium-sized datasets that are frequently accessed. Here’s an implementation example in Python using Django’s caching framework:
from django.core.cache import cache
from django.views import View
from django.http import JsonResponse
class ProductListView(View):
def get(self, request):
# Try to get data from cache
product_list = cache.get('all_products')
if product_list is None:
# Cache miss - fetch from database
product_list = Product.objects.all().values()
# Store in cache for 30 minutes
cache.set('all_products', product_list, timeout=1800)
return JsonResponse({'products': product_list})
And here’s an equivalent implementation in Java using Spring’s caching abstraction:
@Service
public class ProductService {
@Autowired
private ProductRepository productRepository;
@Cacheable(value = "products", key = "'all'")
public List<Product> getAllProducts() {
// This method will be cached automatically
return productRepository.findAll();
}
@CacheEvict(value = "products", key = "'all'")
public void addProduct(Product product) {
productRepository.save(product);
}
}
Distributed Caching
Distributed caching extends beyond a single server’s memory, providing a shared cache across multiple application instances. This approach is crucial for scalable applications running on multiple servers. Here’s an example using Redis with Python:
import redis
from django.conf import settings
from django.core.cache import cache
class DistributedCacheManager:
def __init__(self):
self.redis_client = redis.Redis(
host=settings.REDIS_HOST,
port=settings.REDIS_PORT,
db=0
)
def get_or_set_data(self, key, callback, timeout=3600):
data = self.redis_client.get(key)
if data is None:
data = callback()
self.redis_client.setex(key, timeout, str(data))
return data
Usage in a view
class UserProfileView(View):
def get(self, request, user_id):
cache_manager = DistributedCacheManager()
user_data = cache_manager.get_or_set_data(
f'user_profile_{user_id}',
lambda: User.objects.get(id=user_id).to_dict()
)
return JsonResponse(user_data)
Java implementation using Redis with Spring:
@Configuration
@EnableCaching
public class RedisCacheConfig {
@Bean
public RedisCacheManager cacheManager(RedisConnectionFactory connectionFactory) {
RedisCacheConfiguration config = RedisCacheConfiguration.defaultCacheConfig()
.entryTtl(Duration.ofHours(1))
.serializeKeysWith(RedisSerializationContext.SerializationPair
.fromSerializer(new StringRedisSerializer()))
.serializeValuesWith(RedisSerializationContext.SerializationPair
.fromSerializer(new GenericJackson2JsonRedisSerializer()));
return RedisCacheManager.builder(connectionFactory)
.cacheDefaults(config)
.build();
}
}
Caching Strategies and Patterns
Cache-Aside Pattern
The Cache-Aside pattern, also known as Lazy Loading, involves checking the cache first and, upon a cache miss, loading data from the source and updating the cache. This pattern is particularly effective for read-heavy applications.
class CacheAsidePattern:
def __init__(self, cache_client, data_source):
self.cache = cache_client
self.data_source = data_source
def get_data(self, key):
# Try cache first
data = self.cache.get(key)
if data is None:
# Cache miss - read from source
data = self.data_source.get_data(key)
# Update cache
self.cache.set(key, data, timeout=3600)
return data
Write-Through Pattern
The Write-Through pattern updates both the cache and the underlying data store as part of the same transaction. This ensures consistency but may introduce additional latency during writes.
@Service
public class WriteThoughCacheService {
@Autowired
private CacheManager cacheManager;
@Autowired
private DataRepository repository;
@Transactional
public void saveData(String key, Object data) {
// Save to database
repository.save(data);
// Update cache
Cache cache = cacheManager.getCache("myCache");
cache.put(key, data);
}
}
Cache Invalidation Strategies
Time-Based Invalidation
from datetime import timedelta
from django.core.cache import cache
class TimeBasedCache:
@staticmethod
def cache_with_timeout(key, value, hours=1):
timeout = timedelta(hours=hours).total_seconds()
cache.set(key, value, timeout=timeout)
Event-Based Invalidation
@Service
public class EventBasedCacheService {
@Autowired
private CacheManager cacheManager;
@CacheEvict(value = "dataCache", allEntries = true)
public void invalidateCache() {
// Cache will be cleared when this method is called
logger.info("Cache invalidated due to event");
}
@CacheEvict(value = "dataCache", key = "#id")
public void invalidateSpecificEntry(String id) {
// Only specified entry will be removed
logger.info("Cache entry {} invalidated", id);
}
}
Performance Monitoring and Optimization
Cache Hit Ratio Monitoring
class CacheMonitor:
def __init__(self):
self.hits = 0
self.misses = 0
def record_hit(self):
self.hits += 1
def record_miss(self):
self.misses += 1
def get_hit_ratio(self):
total = self.hits + self.misses
return self.hits / total if total > 0 else 0
def get_stats(self):
return {
'hits': self.hits,
'misses': self.misses,
'hit_ratio': self.get_hit_ratio()
}
Cache Configuration Best Practices
Parameter | Recommended Setting | Description |
---|---|---|
Cache Size | 20% of available RAM | Balance between performance and resource usage |
TTL | 1-24 hours | Depends on data volatility |
Eviction Policy | LRU (Least Recently Used) | Most efficient for most use cases |
Compression | Enable for large objects | Reduces memory usage |
Implementation Considerations and Challenges
Handling Race Conditions
import threading
from django.core.cache import cache
class ThreadSafeCaching:
_lock = threading.Lock()
@classmethod
def get_or_compute(cls, key, compute_func):
value = cache.get(key)
if value is None:
with cls._lock:
# Double-check pattern
value = cache.get(key)
if value is None:
value = compute_func()
cache.set(key, value)
return value
Cache Consistency
@Service
public class ConsistentCacheService {
@Autowired
private CacheManager cacheManager;
@Transactional
@CachePut(value = "dataCache", key = "#result.id")
public Data updateData(Data data) {
// Update database
Data updated = repository.save(data);
// Notify other services about the update
eventPublisher.publishUpdate(updated);
return updated;
}
}
Advanced Caching Techniques
Hierarchical Caching
class HierarchicalCache:
def __init__(self, l1_cache, l2_cache, l3_cache):
self.l1 = l1_cache # Memory cache
self.l2 = l2_cache # Redis cache
self.l3 = l3_cache # Database
def get(self, key):
# Try L1 cache
value = self.l1.get(key)
if value:
return value
# Try L2 cache
value = self.l2.get(key)
if value:
self.l1.set(key, value) # Populate L1
return value
# Get from L3
value = self.l3.get(key)
if value:
self.l2.set(key, value) # Populate L2
self.l1.set(key, value) # Populate L1
return value
Conclusion
Implementing effective caching strategies in MVC applications requires careful consideration of various factors, including data access patterns, consistency requirements, and scalability needs. By leveraging appropriate caching mechanisms and following best practices, developers can significantly improve application performance while maintaining code maintainability and reliability. Regular monitoring and optimization of caching strategies ensure that the implementation continues to meet performance requirements as the application evolves.
Disclaimer: The code examples and recommendations provided in this blog post are based on general best practices and may need to be adapted to specific use cases. While we strive for accuracy, technology evolves rapidly, and some information may become outdated. Please report any inaccuracies or outdated information to our editorial team, and we will promptly update the content. The performance improvements mentioned may vary depending on your specific implementation and infrastructure.