Are you preparing for your first Java developer interview? Mastering these fundamental Java interview questions will help you build confidence and demonstrate your programming knowledge effectively. This comprehensive guide covers the most common Java interview questions for beginners, along with detailed explanations and practical examples to help you ace your next interview. 1. What are the main features of Java, […]
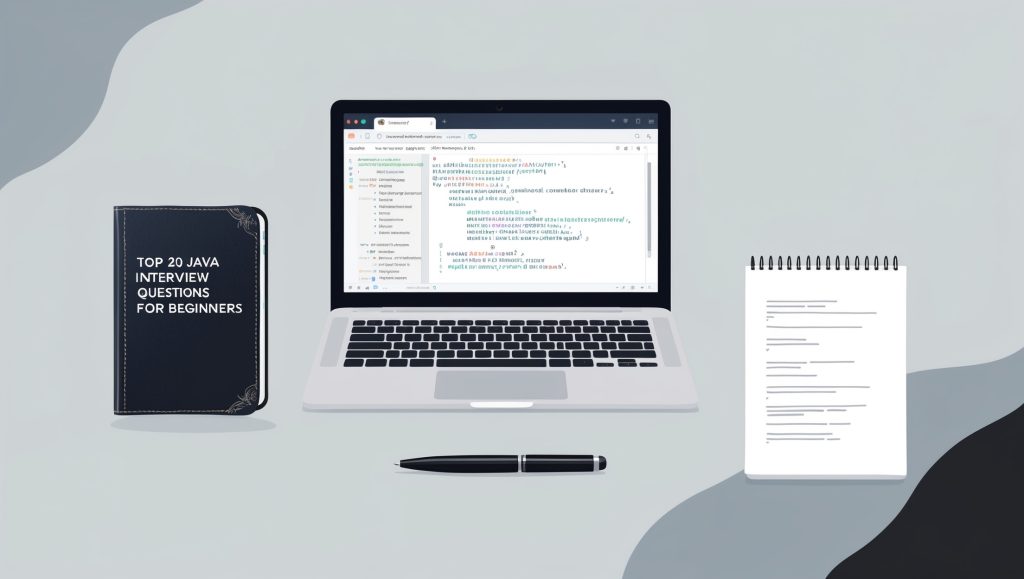