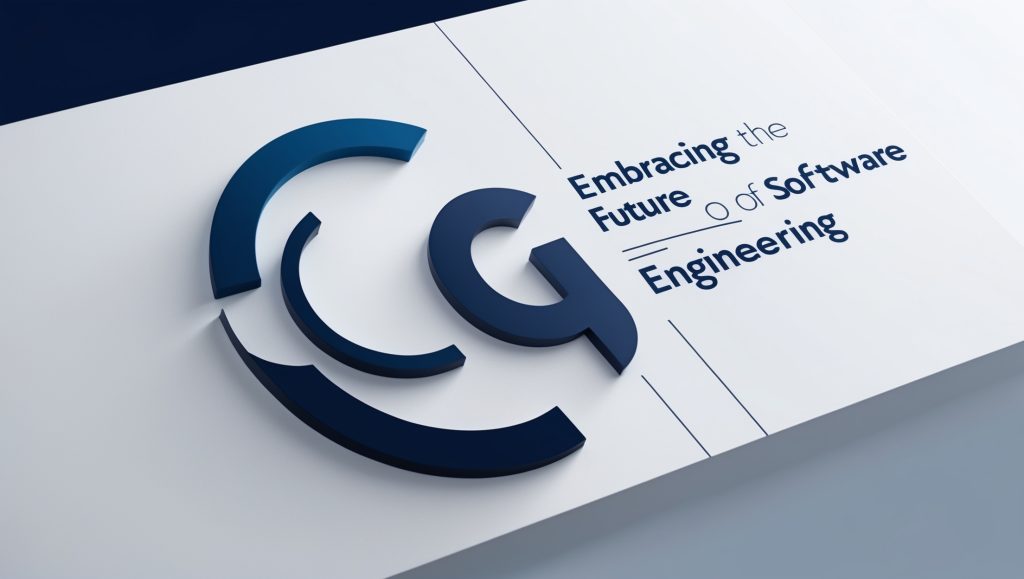
Cloud-Native Java Development: Embracing the Future of Software Engineering
Welcome, fellow Java enthusiasts and curious developers! Today, we’re diving deep into the exciting world of cloud-native Java development. If you’ve been feeling like your trusty old Java skills need a refresh for the modern age, you’re in the right place. The cloud has revolutionized how we build, deploy, and scale applications, and Java is right at the forefront of this transformation.
Remember the days when setting up a Java application meant wrestling with local servers, complex configurations, and the dreaded “works on my machine” syndrome? Well, those days are fading into the rearview mirror. Cloud-native development is here to stay, and it’s changing the game for Java developers worldwide. In this blog post, we’ll explore what cloud-native Java development means, why it’s crucial for modern software engineering, and how you can embrace this paradigm shift to supercharge your Java projects.
So, grab your favorite caffeinated beverage, fire up your IDE, and let’s embark on this cloud-native journey together. By the end of this post, you’ll have a solid understanding of cloud-native principles, practical Java examples, and the confidence to take your first steps into this brave new world of development. Ready? Let’s dive in!
What is Cloud-Native Development?
Before we get into the nitty-gritty of Java-specific cloud-native practices, let’s take a moment to understand what “cloud-native” really means. At its core, cloud-native development is an approach to building and running applications that fully embraces the advantages of the cloud computing model. It’s not just about hosting your app on a cloud platform; it’s a fundamental shift in how we design, implement, and operate software.
Key Principles of Cloud-Native Development:
- Microservices Architecture: Breaking down monolithic applications into smaller, independently deployable services.
- Containerization: Packaging applications and their dependencies into lightweight, portable containers.
- Dynamic Orchestration: Using tools like Kubernetes to manage and scale containerized applications automatically.
- Continuous Delivery: Implementing automated pipelines for building, testing, and deploying code changes.
- Scalability and Resilience: Designing systems that can handle variable loads and recover gracefully from failures.
Cloud-native development isn’t tied to any specific programming language or technology stack. However, Java, with its rich ecosystem and enterprise-grade capabilities, is particularly well-suited for this approach. The Java community has been quick to adapt, creating frameworks, tools, and best practices that align perfectly with cloud-native principles.
As we progress through this blog post, we’ll see how these cloud-native concepts translate into practical Java development techniques. You’ll discover how to leverage Java’s strengths in a cloud environment and overcome traditional limitations to build scalable, resilient, and efficient applications.
Why Java for Cloud-Native Development?
You might be wondering, “Why should I choose Java for cloud-native development when there are so many trendy languages out there?” It’s a fair question, and the answer lies in Java’s unique combination of maturity, performance, and adaptability.
Java’s Cloud-Native Advantages:
- Robust Ecosystem: Java boasts a vast collection of libraries, frameworks, and tools that have evolved to support cloud-native development.
- Performance Optimizations: Recent JVM improvements and features like ahead-of-time compilation make Java more efficient in cloud environments.
- Enterprise-Grade Security: Java’s strong security model and extensive security libraries are crucial for building secure cloud applications.
- Scalability: Java’s multithreading capabilities and efficient resource management make it ideal for handling high-concurrency workloads.
- Community Support: The Java community is actively developing cloud-native solutions, ensuring a wealth of resources and expertise.
Let’s look at a simple example of how Java’s features align with cloud-native principles. Consider this basic microservice using Spring Boot:
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RestController;
@SpringBootApplication
@RestController
public class CloudNativeServiceApplication {
public static void main(String[] args) {
SpringApplication.run(CloudNativeServiceApplication.class, args);
}
@GetMapping("/hello")
public String hello() {
return "Hello, Cloud-Native World!";
}
}
This small piece of code demonstrates several cloud-native concepts:
- It’s a self-contained microservice that can be easily containerized.
- Spring Boot’s auto-configuration simplifies deployment in various cloud environments.
- The RESTful endpoint can be part of a larger distributed system.
- It’s lightweight and starts up quickly, ideal for dynamic scaling.
As we delve deeper into cloud-native Java development, you’ll see how Java’s capabilities expand to meet even the most demanding cloud-native requirements.
Getting Started with Cloud-Native Java Development
Now that we’ve covered the why, let’s focus on the how. Getting started with cloud-native Java development might seem daunting at first, but with the right tools and mindset, you’ll be up and running in no time.
Essential Tools for Cloud-Native Java Development:
- Java Development Kit (JDK) 11 or later
- A cloud-native framework (e.g., Spring Boot, Quarkus, or Micronaut)
- Docker for containerization
- Kubernetes for orchestration (or a managed Kubernetes service like Amazon EKS or Google Kubernetes Engine)
- A CI/CD tool (e.g., Jenkins, GitLab CI, or GitHub Actions)
Let’s walk through setting up a basic cloud-native Java project using Spring Boot and Docker. We’ll create a simple RESTful service and package it as a Docker container.
First, use Spring Initializr (https://start.spring.io/) to generate a new Spring Boot project with the “Spring Web” dependency. Once you’ve downloaded and extracted the project, open it in your favorite IDE.
Now, let’s create a simple REST controller:
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RestController;
@RestController
public class GreetingController {
@GetMapping("/greeting")
public String greeting() {
return "Welcome to cloud-native Java development!";
}
}
Next, we’ll create a Dockerfile in the root of our project to containerize our application:
FROM openjdk:11-jre-slim
VOLUME /tmp
ARG JAR_FILE=target/*.jar
COPY ${JAR_FILE} app.jar
ENTRYPOINT ["java","-jar","/app.jar"]
This Dockerfile uses a slim JRE image, copies our compiled JAR file, and sets up the entry point to run our application.
To build and run the Docker container, use the following commands:
./mvnw package
docker build -t cloud-native-java-app .
docker run -p 8080:8080 cloud-native-java-app
Voila! You now have a cloud-native Java application running in a Docker container. This is just the beginning, but it demonstrates how quickly you can get started with cloud-native Java development.
As we progress, we’ll explore more advanced topics like scaling, service discovery, and resilience patterns, all within the context of Java and cloud-native principles.
Microservices Architecture with Java
One of the cornerstones of cloud-native development is the microservices architecture. This approach involves breaking down your application into smaller, loosely coupled services that can be developed, deployed, and scaled independently. Java, with its robust ecosystem, provides excellent tools for building microservices.
Benefits of Microservices in Java:
- Modularity: Easier to understand, develop, and maintain individual services.
- Scalability: Scale services independently based on demand.
- Technology Diversity: Use different Java frameworks or even languages for different services.
- Resilience: Failures in one service don’t necessarily affect others.
- Continuous Delivery: Smaller codebases enable faster development and deployment cycles.
Let’s look at how we can create a simple microservices architecture using Spring Boot and Spring Cloud.
First, we’ll create a service registry using Netflix Eureka:
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.springframework.cloud.netflix.eureka.server.EnableEurekaServer;
@SpringBootApplication
@EnableEurekaServer
public class ServiceRegistryApplication {
public static void main(String[] args) {
SpringApplication.run(ServiceRegistryApplication.class, args);
}
}
Next, let’s create two microservices: a product service and an order service.
Product Service:
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.springframework.cloud.client.discovery.EnableDiscoveryClient;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RestController;
@SpringBootApplication
@EnableDiscoveryClient
@RestController
public class ProductServiceApplication {
public static void main(String[] args) {
SpringApplication.run(ProductServiceApplication.class, args);
}
@GetMapping("/products")
public String getProducts() {
return "List of products";
}
}
Order Service:
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.springframework.cloud.client.discovery.EnableDiscoveryClient;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RestController;
@SpringBootApplication
@EnableDiscoveryClient
@RestController
public class OrderServiceApplication {
public static void main(String[] args) {
SpringApplication.run(OrderServiceApplication.class, args);
}
@GetMapping("/orders")
public String getOrders() {
return "List of orders";
}
}
These microservices register themselves with the Eureka server, enabling service discovery. You can now deploy these services independently and scale them as needed.
To make these microservices truly cloud-native, you’d want to add features like:
- Centralized configuration management
- Circuit breakers for resilience
- API gateways for routing and load balancing
- Distributed tracing for monitoring
Spring Cloud provides a comprehensive set of tools to implement these patterns, making it easier to build robust, cloud-native microservices architectures in Java.
Containerization and Orchestration with Java
Containerization has revolutionized how we package and deploy applications, and it’s a crucial component of cloud-native development. For Java applications, containerization offers consistency across different environments, efficient resource utilization, and rapid deployment capabilities.
Docker and Java: A Perfect Match
Docker has become the de facto standard for containerization, and it works beautifully with Java applications. Let’s expand on our earlier Dockerfile example to create a more production-ready container for a Spring Boot application:
# Use the official OpenJDK image as a parent image
FROM openjdk:11-jre-slim as runtime
# Set the working directory in the container
WORKDIR /app
# Copy the jar file into the container
COPY target/*.jar app.jar
# Run the jar file
ENTRYPOINT ["java", "-Djava.security.egd=file:/dev/./urandom", "-jar", "/app/app.jar"]
This Dockerfile includes some best practices:
- Using a slim JRE image to reduce container size
- Setting a working directory
- Using the
java.security.egd
system property to improve startup time
To build and run this container, you’d use:
docker build -t my-java-app .
docker run -p 8080:8080 my-java-app
Kubernetes: Orchestrating Java Containers
While Docker takes care of containerization, Kubernetes handles orchestration – the process of managing, scaling, and maintaining containerized applications. Here’s a basic Kubernetes deployment YAML for our Java application:
apiVersion: apps/v1
kind: Deployment
metadata:
name: my-java-app
spec:
replicas: 3
selector:
matchLabels:
app: my-java-app
template:
metadata:
labels:
app: my-java-app
spec:
containers:
- name: my-java-app
image: my-java-app:latest
ports:
- containerPort: 8080
This YAML file defines a Kubernetes Deployment that:
- Creates 3 replicas of our application
- Uses the
my-java-app:latest
Docker image - Exposes port 8080
To deploy this to a Kubernetes cluster, you’d use:
kubectl apply -f deployment.yaml
Kubernetes offers many advanced features that are particularly useful for Java applications:
- Horizontal Pod Autoscaling: Automatically scale your Java applications based on CPU usage or custom metrics.
- ConfigMaps and Secrets: Manage configuration and sensitive data separately from your Java code.
- Liveness and Readiness Probes: Ensure your Java services are healthy and ready to receive traffic.
- Rolling Updates: Deploy new versions of your Java applications with zero downtime.
Here’s an example of how you might define a Horizontal Pod Autoscaler for your Java application:
apiVersion: autoscaling/v2beta1
kind: HorizontalPodAutoscaler
metadata:
name: my-java-app-hpa
spec:
scaleTargetRef:
apiVersion: apps/v1
kind: Deployment
name: my-java-app
minReplicas: 2
maxReplicas: 10
metrics:
- type: Resource
resource:
name: cpu
targetAverageUtilization: 50
This HPA will automatically scale your Java application between 2 and 10 replicas based on CPU utilization, aiming to maintain an average CPU usage of 50%.
By leveraging containerization and orchestration, you can ensure your Java applications are truly cloud-native, benefiting from improved scalability, portability, and resilience.
Continuous Integration and Continuous Deployment (CI/CD) for Java
In the cloud-native world, the ability to rapidly and reliably deliver new features and fixes is crucial. This is where Continuous Integration and Continuous Deployment (CI/CD) comes into play. For Java applications, implementing a robust CI/CD pipeline can significantly streamline your development process and improve the quality of your software.
Setting Up a CI/CD Pipeline for Java
Let’s walk through setting up a basic CI/CD pipeline for a Java application using GitHub Actions. This pipeline will build our Java application, run tests, create a Docker image, and deploy it to a Kubernetes cluster.
First, create a .github/workflows/main.yml
file in your repository:
name: Java CI/CD
on:
push:
branches: [ main ]
pull_request:
branches: [ main ]
jobs:
build:
runs-on: ubuntu-latest
steps:
- uses: actions/checkout@v2
- name: Set up JDK 11
uses: actions/setup-java@v2
with:
java-version: '11'
distribution: 'adopt'
- name: Build with Maven
run: mvn clean package
- name: Run tests
run: mvn test
- name: Build Docker image
run: docker build -t my-java-app:${{ github.sha }} .
- name: Push to Docker Hub
env:
DOCKER_USERNAME: ${{ secrets.DOCKER_USERNAME }}
DOCKER_PASSWORD: ${{ secrets.DOCKER_PASSWORD }}
run: |
echo $DOCKER_PASSWORD | docker login -u $DOCKER_USERNAME --password-stdin
docker push my-java-app:${{ github.sha }}
- name: Deploy to Kubernetes
env:
KUBE_CONFIG: ${{ secrets.KUBE_CONFIG }}
run: |
echo "$KUBE_CONFIG" > kubeconfig
kubectl --kubeconfig=kubeconfig set image deployment/my-java-app my-java-app=my-java-app:${{ github.sha }}
This GitHub Actions workflow does the following:
- Triggers on pushes to the main branch or pull requests
- Sets up a Java 11 environment
- Builds the application using Maven
- Runs tests
- Builds a Docker image
- Pushes the image to Docker Hub
- Deploys the new image to a Kubernetes cluster
To make this work, you’ll need to set up secrets in your GitHub repository for your Docker Hub credentials and Kubernetes configuration