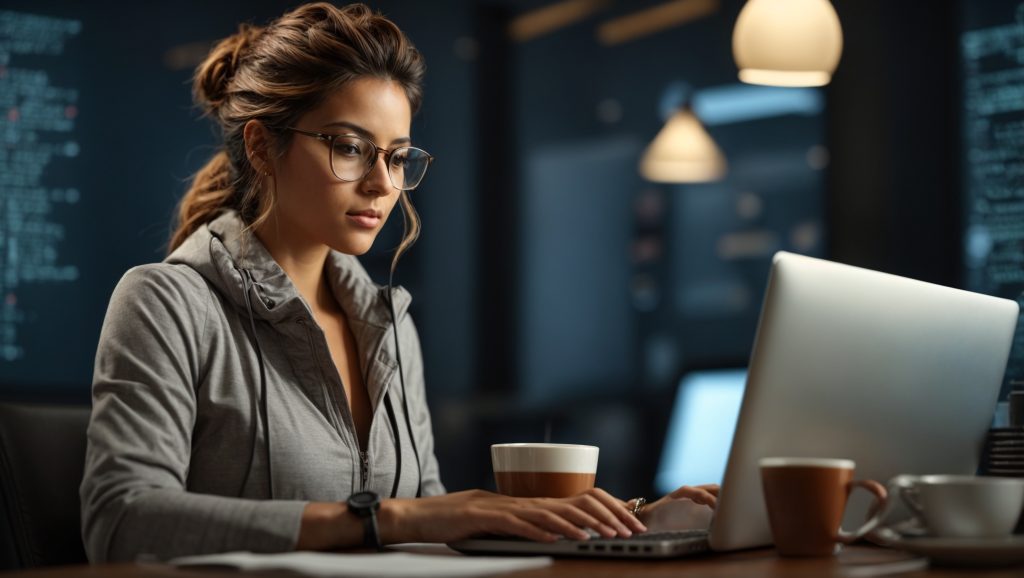
Code Reusability in Java: Write Once, Use Everywhere
Code reusability is a fundamental aspect of efficient and maintainable software development. By promoting reusability, Java developers can create modular, scalable, and efficient software solutions that save time and effort. In this blog post, we’ll delve into the concept of code reusability in Java development, discuss its benefits, and provide practical tips and examples to help you harness the power of reusable code in your projects.
Understanding Code Reusability
Code reusability refers to the practice of writing code that can be used in multiple parts of a software solution or even across different projects. By creating reusable components, Java developers can minimize code duplication, reduce development time, and improve maintainability. Reusable code is typically modular, encapsulated, and adheres to established design principles, such as SOLID and DRY (Don’t Repeat Yourself).
Benefits of Code Reusability
Embracing code reusability in Java development offers several benefits:
- Reduced development time: By reusing existing components, developers can save time and effort, allowing them to deliver software more quickly and efficiently.
- Improved maintainability: Reusable code is typically modular and well-encapsulated, making it easier to understand, modify, and maintain.
- Enhanced consistency: Reusing code components promotes consistency across different parts of a software solution or even across multiple projects, improving the overall quality and reliability of the software.
- Cost savings: By leveraging reusable components, developers can reduce development and maintenance costs, providing a more cost-effective solution for their clients or organizations.
Tips for Enhancing Code Reusability in Java Development
To effectively promote code reusability in your Java projects, consider the following tips:
- Encapsulate functionality: Encapsulation is a fundamental concept in object-oriented programming that involves hiding the internal details of a component and exposing a well-defined interface. By encapsulating functionality in your Java classes, you can create reusable components that are easy to understand, maintain, and reuse.
- Use inheritance and interfaces: Inheritance and interfaces are powerful Java features that enable code reuse through polymorphism. By designing your classes and interfaces with reusability in mind, you can create flexible and extensible code components that can be easily reused in different contexts.
- Apply design patterns: Design patterns are reusable solutions to common problems in software design. By incorporating design patterns into your Java code, you can enhance reusability and create more modular and maintainable software solutions.
- Leverage Java libraries and frameworks: Java offers a rich ecosystem of libraries and frameworks that can help you reuse existing components and functionality. By leveraging these resources, you can save time and effort while improving the overall quality and reusability of your code.
- Follow best practices: Adopting Java best practices, such as adhering to established naming conventions and code organization, can make your code more consistent, maintainable, and reusable.
Practical Examples of Reusability in Java
To illustrate code reusability in Java development, let’s examine a few practical examples:
Utility classes
Utility classes are a common way to encapsulate reusable functionality in Java. By creating utility classes with static methods, you can provide a central location for reusable code that can be easily accessed and reused throughout your project.
public final class BankingUtils {
private BankingUtils() {
// Private constructor to prevent instantiation
}
public static double calculateInterest(double amount, double rate, int years) {
return amount * rate * years / 100;
}
public static double calculateMonthlyRepayment(double loanAmount, double rate, int termInYears) {
double monthlyRate = rate / 12 / 100;
int termInMonths = termInYears * 12;
return (loanAmount * monthlyRate) / (1 - Math.pow(1 + monthlyRate, -termInMonths));
}
}
Inheritance
Inheritance allows you to create new classes that reuse, extend, or modify the behavior of existing classes. By leveraging inheritance, you can promote code reusability and create more maintainable and extensible software solutions.
public class Account {
protected double balance;
public Account(double balance) {
this.balance = balance;
}
public void deposit(double amount) {
balance += amount;
}
public void withdraw(double amount) {
balance -= amount;
}
// Other common account methods
}
public class SavingsAccount extends Account {
private double interestRate;
public SavingsAccount(double balance, double interestRate) {
super(balance);
this.interestRate = interestRate;
}
public void addInterest() {
double interest = balance * interestRate / 100;
deposit(interest);
}
// Specific methods for a Savings Account
}
public class CheckingAccount extends Account {
// Constructor and specific methods for a Checking Account
}
Interfaces
Interfaces in Java define a contract that implementing classes must adhere to, enabling polymorphism and code reusability. By designing your classes with interfaces, you can create flexible and reusable components that can be easily adapted to different contexts.
public interface Transactional {
void deposit(double amount);
void withdraw(double amount);
double getBalance();
}
public class PersonalAccount implements Transactional {
private double balance;
@Override
public void deposit(double amount) {
balance += amount;
}
@Override
public void withdraw(double amount) {
balance -= amount;
}
@Override
public double getBalance() {
return balance;
}
// Additional methods specific to personal account
}
public class CorporateAccount implements Transactional {
private double balance;
// Implementations for deposit, withdraw, and getBalance
// Additional methods specific to corporate account
}
Balancing Reusability with Other Design Concerns
While code reusability is an important goal in Java development, it’s essential to balance reusability with other software design concerns, such as readability, maintainability, and performance. Striving for reusability at all costs can lead to overly abstract or complex code, which may be difficult to understand, maintain, or optimize. By considering the specific needs and requirements of your project, you can make informed decisions about when and how to prioritize code reusability.
Code reusability is a crucial aspect of efficient, maintainable, and modular Java development. By embracing reusability techniques, design patterns, and best practices, you can create software solutions that save time, effort, and costs while improving overall quality and reliability.
To effectively promote code reusability in your Java projects, remember to:
- Encapsulate functionality to create reusable components.
- Use inheritance and interfaces to enable code reuse through polymorphism.
- Apply design patterns to enhance reusability and modularity.
- Leverage Java libraries and frameworks to reuse existing components and functionality.
- Follow best practices to ensure consistent, maintainable, and reusable code.
By mastering code reusability and incorporating it into your Java development practices, you can build robust, efficient, and adaptable software solutions that stand the test of time. Write once, use everywhere!