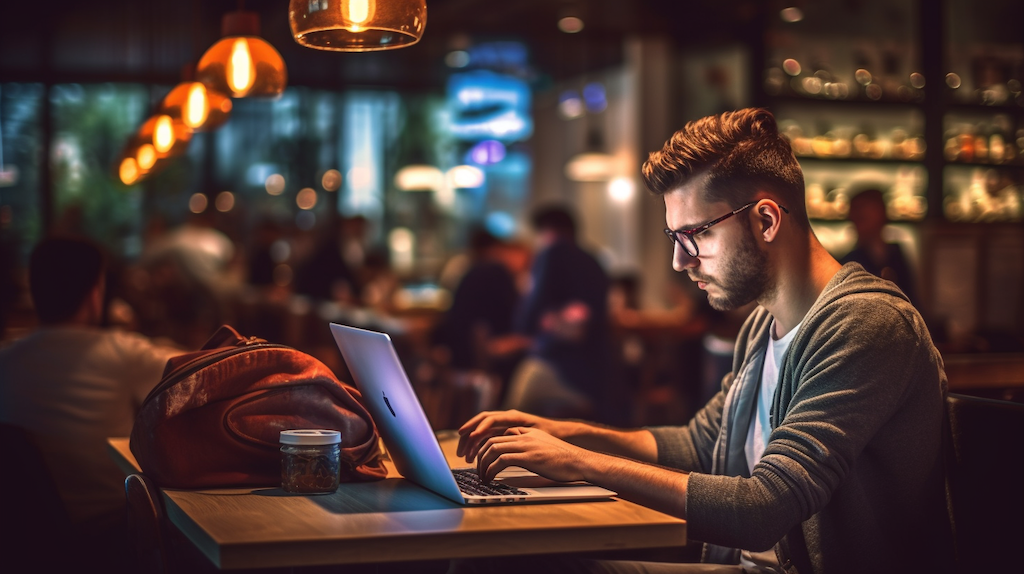
Coding for Beginners: How to Build Your First Website or App
Hey there! Have you ever thought about learning how to code and build your own website or app? Well you’ve come to the right place!
Learning to code can definitely seem daunting at first. I remember when I wrote my first line of code – I had no idea what I was doing! But with a little guidance and lots of practice, anyone can pick up coding fundamentals and start bringing their ideas to life.
So why learn to code in the first place? Well for starters, it allows you to create things and solve problems. Pretty cool right? Whether you want to build a website, app, or game, knowing how to code gives you the power to make it happen yourself. You don’t have to rely on anyone else. It also teaches you computational thinking and problem solving skills that are valuable even outside of coding.
Plus, coding is a lot of fun! It feels really rewarding to start with a blank text file and end up with a fully functioning website or app that you built yourself. It’s like magic. As you learn and improve, you can constantly challenge yourself with new projects and goals. The possibilities are endless.
Now that you’re pumped up to start coding, let’s look at some of the basics.
Choosing a Programming Language
First things first – you’ll need to choose a programming language to learn. Some popular options for beginners include:
- HTML & CSS – For building websites and web pages
- JavaScript – For adding dynamic functionality to websites
- Python – A versatile general-purpose language used in web dev and beyond
- Swift – For building iOS apps for Apple devices
- Java – Used for Android app development and back-end web systems
I’d recommend starting with HTML, CSS, and JavaScript, as those are the core languages used in web development. With just those, you can build fully functioning (though basic) websites. Python and Swift are also beginner-friendly choices if you’re interested in app development later on.
Once you pick a language, there are tons of free resources to help you learn the basics – from interactive code playgrounds and online courses to books and YouTube tutorials. More on that later!
Understanding Web Basics
Before you can build a website or web app, you need to understand how webpages and websites actually work under the hood.
At the most basic level, websites are made up of webpages. Each webpage is a document, usually written in HTML, that your browser loads and renders. HTML gives the webpage structure and semantics, while CSS controls the styling and layout. JavaScript can then be used to add interactive elements and dynamic effects.
Many websites have multiple linked webpages – like different pages for “About Us”, “Contact”, etc. This is what forms a multi-page website.
All the webpages are hosted together on a web server. This allows the website to be accessed online via a domain name (like google.com). When you type a URL into your browser, it makes a request to the remote web server to fetch the files for that webpage which are then rendered on your screen.
So in summary:
- Webpage – A document written in HTML, CSS, JavaScript
- Website – Collection of linked webpages on a domain
- Web server – Hosts and delivers the webpage files
- Domain name – The website URL
With this foundation, you’re ready to start coding your own basic webpages!
Building Your First Webpage
Let’s walk through how to create a simple “Hello World” webpage to see HTML in action. Don’t worry if the syntax seems strange at first – it will start to make sense with a little practice.
First, open up a plain text editor like Notepad or TextEdit. Then type out the following:
<!DOCTYPE html>
<html>
<head>
<title>Hello World</title>
</head>
<body>
<h1>Hello World!</h1>
<p>My first webpage!</p>
</body>
</html>
Let’s break down what each part does:
<!DOCTYPE html>
– Declares this is an HTML5 document<html>
– The root element that wraps the whole page<head>
– Contains info about the page like the title<title>
– The title displayed in the browser tab<body>
– The visible content shown to the user<h1>
– A top-level heading<p>
– A paragraph of text
Now save the file with a .html
extension, like hello.html
and open it in your web browser. Congrats, you just made your first web page!
With this basic structure, you can now add new elements like images, links, lists, etc. The possibilities are endless.
Spicing It Up with CSS
So we’ve got a webpage up and running. But it looks pretty plain so far. This is where CSS comes in – it allows you to style and layout your HTML content to make it look amazing.
CSS works by targeting HTML elements and applying styling rules like colors, fonts, spacing, backgrounds, effects, and more.
Let’s add some CSS to our “Hello World” page. In the head section, add:
<style>
body {
background-color: #FEF2F2;
font-family: Arial, Helvetica, sans-serif;
}
h1 {
color: #FF006E;
text-align: center;
}
</style>
This targets the <body>
and <h1>
tags and applies styling rules. Refresh the page, and you should see a pink background with centered, pink heading text. Much better!
With CSS combined with HTML, you can create beautiful, engaging webpages and websites. The visual possibilities are endless.
Advancing to JavaScript
So far we’ve used HTML for content and structure and CSS for presentation. Now let’s add some interactivity with JavaScript.
JavaScript is a programming language that lets you add dynamic functionality to websites. You can respond to user actions, dynamically update pages, validate forms, and much more.
Let’s add a simple script to our page that shows a pop-up alert. In the body, add:
<script>
alert('Welcome to my page!');
</script>
Reload the page, and ta-da! You should see the alert pop up. With a few lines of JavaScript, we took a static page and added some logic to it.
Here are just some examples of what you can do with JavaScript:
- Show popups, alerts, prompts
- Change HTML content and styles dynamically
- Respond to clicks, hovers, typing
- Create animations and effects
- Form validation and submission
- Interactive galleries, widgets, games
- Send and receive data from servers (AJAX, APIs)
The sky’s really the limit here. JavaScript is used everywhere on the modern web. So it’s a must-learn for aspiring coders!
Putting It All Together
Now that you have the basic building blocks – HTML, CSS, JavaScript – it’s time to put them together to make your first real webpage.
A simple starter idea is an “About Me” page. It can have:
- A headline with your name
- A profile photo
- Paragraphs with bios/facts about you
- An interests/hobbies list
- A “Contact Me” button that pops up your email
- Some CSS styling and colors to spice it up
Piece it together bit by bit, and don’t be afraid to refer back to tutorials or examples online. Part of coding is looking things up!
Some key tips:
- Use semantic HTML tags like
<header>, <section>, <article>
to structure content - Style elements by class or ID instead of tag name
- Keep your CSS code organized and reusable
- Add JavaScript event handlers for interactivity
With an About Me page under your belt, you can then expand and create more complex sites! Turn it into a full personal portfolio site, social network, or any kind of project you can dream up.
Learning to code takes persistence. Here are sample codes you can play around. You can download the code from our GitHub page, go to GitHub and look for search for the felixrante.com repo. Enjoy!
- Personal Portfolio – This webpage will showcase your personal information, skills, and projects. It will include sections for an introduction, about me, skills, projects, and contact information.
- Simple Calculator – This webpage will allow users to perform basic arithmetic operations like addition, subtraction, multiplication, and division.
- Todo List – This webpage will allow users to create, manage, and track their todo items.
- Image Gallery – This webpage will display a collection of images in a gallery format, allowing users to view them individually.