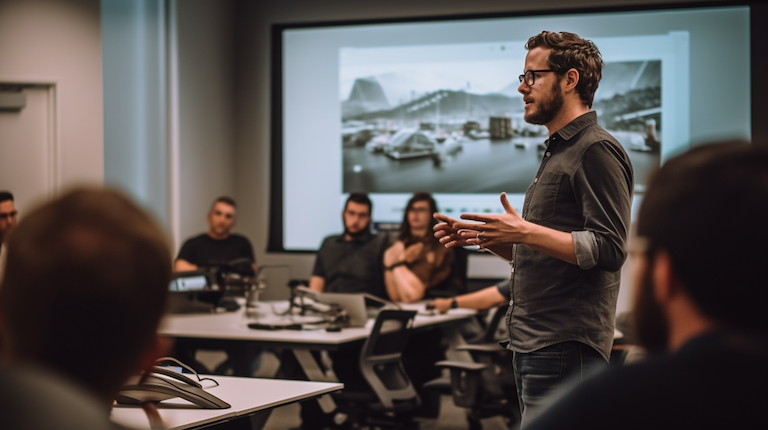
Cohesion and Coupling in Java
Cohesion and coupling are two critical software design principles that help developers create more maintainable, robust, and efficient software solutions. By understanding these concepts and applying them to Java development, you can achieve the right balance between modularity and interdependence, leading to better code quality and long-term maintainability. In this blog post, we’ll explore the concepts of cohesion and coupling, discuss their importance, and provide practical tips and examples to help you apply these design principles to your Java projects.
Understanding Cohesion and Coupling
Cohesion refers to the degree to which the elements within a module, such as a class or a method, are related to each other. High cohesion means that the elements within a module are closely related and focused on a single responsibility, making the module more maintainable, understandable, and robust.
Coupling, on the other hand, refers to the degree to which one module depends on other modules. Low coupling means that the dependencies between modules are minimal, making the overall system more modular, maintainable, and easier to refactor.
In Java development, the goal is to achieve high cohesion and low coupling to create software solutions that are easier to understand, modify, and extend.
Benefits of High Cohesion and Low Coupling
Focusing on high cohesion and low coupling in Java development offers several benefits:
- Maintainability: High cohesion and low coupling make the code more maintainable, as changes to one module are less likely to impact other modules.
- Reusability: Modules with high cohesion and low coupling can be easily reused in other parts of the application or even in other projects.
- Testability: Modules with high cohesion and low coupling are easier to test, as their functionality is focused, and they have fewer dependencies on other modules.
- Robustness: High cohesion and low coupling help create more robust software solutions, as bugs and errors in one module are less likely to propagate through the entire system.
Best Practices for Achieving High Cohesion and Low Coupling in Java Development
To effectively achieve high cohesion and low coupling in your Java projects, consider the following best practices:
- Single Responsibility Principle (SRP): Ensure that each class or method is focused on a single responsibility, promoting high cohesion.
- Encapsulation: Hide the internal details of a class and expose only the necessary interface, reducing coupling between classes.
- Use interfaces and abstract classes: Promote low coupling by using interfaces and abstract classes to define contracts between classes, rather than relying on concrete implementations.
- Modularize your code: Break your code into smaller, modular components that can be easily combined, reused, and extended.
- Dependency Injection (DI): Use dependency injection to manage dependencies between modules, reducing coupling and enhancing maintainability.
Practical Examples of High Cohesion and Low Coupling in Java
To illustrate the concepts of high cohesion and low coupling in Java development, let’s examine a practical example. Consider the following code snippet, which violates the principles of high cohesion and low coupling:
public class BankingService {
private List<Account> accounts;
private List<String> notifications;
public BankingService() {
this.accounts = new ArrayList<>();
this.notifications = new ArrayList<>();
}
public void addAccount(Account account) {
accounts.add(account);
// Logging
System.out.println("Account added: " + account.getId());
}
public void removeAccount(Account account) {
accounts.remove(account);
// Logging
System.out.println("Account removed: " + account.getId());
}
public void sendNotification(String message) {
notifications.add(message);
// Logging
System.out.println("Notification sent: " + message);
}
public void deposit(String accountId, double amount) {
// Account operation
// ...
// Notification
sendNotification("Deposit made to account: " + accountId);
// Logging
System.out.println("Deposit: " + amount + " to " + accountId);
}
public void withdraw(String accountId, double amount) {
// Account operation
// ...
// Notification
sendNotification("Withdrawal made from account: " + accountId);
// Logging
System.out.println("Withdrawal: " + amount + " from " + accountId);
}
// Other unrelated methods
}
In this example,
The BankingService
class is an example of low cohesion because it mixes account management, sending notifications, and logging activities all in one place. This approach makes the class harder to understand, maintain, and extend since it’s dealing with multiple unrelated responsibilities.
In a well-designed system, these responsibilities would be separated into different classes, each focusing on a single responsibility, thereby increasing cohesion. For example, account management would be handled by an AccountManager
, notifications by a NotificationService
, and logging by a Logger
class.
public class AccountManager {
private List<Account> accounts;
public AccountManager() {
this.accounts = new ArrayList<>();
}
public void addAccount(Account account) {
accounts.add(account);
}
public void removeAccount(Account account) {
accounts.remove(account);
}
public Account findAccountById(String accountId) {
for (Account account : accounts) {
if (account.getId().equals(accountId)) {
return account;
}
}
return null; // or throw an exception
}
public void deposit(String accountId, double amount) {
Account account = findAccountById(accountId);
if (account != null) {
account.deposit(amount);
}
}
public void withdraw(String accountId, double amount) {
Account account = findAccountById(accountId);
if (account != null) {
account.withdraw(amount);
}
}
// Other account management operations
}
This AccountManager
class is highly cohesive because it focuses solely on account management tasks such as adding, removing, finding, depositing to, and withdrawing from accounts.
Balancing Cohesion and Coupling with Other Design Concerns
While achieving high cohesion and low coupling is essential in software design, it’s important to balance these principles with other design concerns, such as performance, readability, and simplicity. In some cases, strictly adhering to high cohesion and low coupling may not be the most appropriate solution for a particular problem. By considering the specific needs and requirements of your project, you can make informed decisions about when to prioritize cohesion and coupling and when to focus on other design aspects.
Cohesion and coupling are fundamental software design principles that can help Java developers create more maintainable, robust, and efficient software solutions. By understanding these concepts and applying them to your Java projects, you can achieve the right balance between modularity and interdependence, leading to better code quality and long-term maintainability.
To effectively achieve high cohesion and low coupling in your Java projects, remember to:
- Follow the Single Responsibility Principle (SRP) to promote high cohesion.
- Encapsulate internal details and expose only the necessary interface.
- Use interfaces and abstract classes to define contracts between classes.
- Modularize your code into smaller, reusable components.
- Utilize dependency injection to manage dependencies between modules.
By mastering cohesion and coupling and incorporating these design principles into your Java development practices, you can build high-quality, well-structured, and maintainable software solutions that stand the test of time.