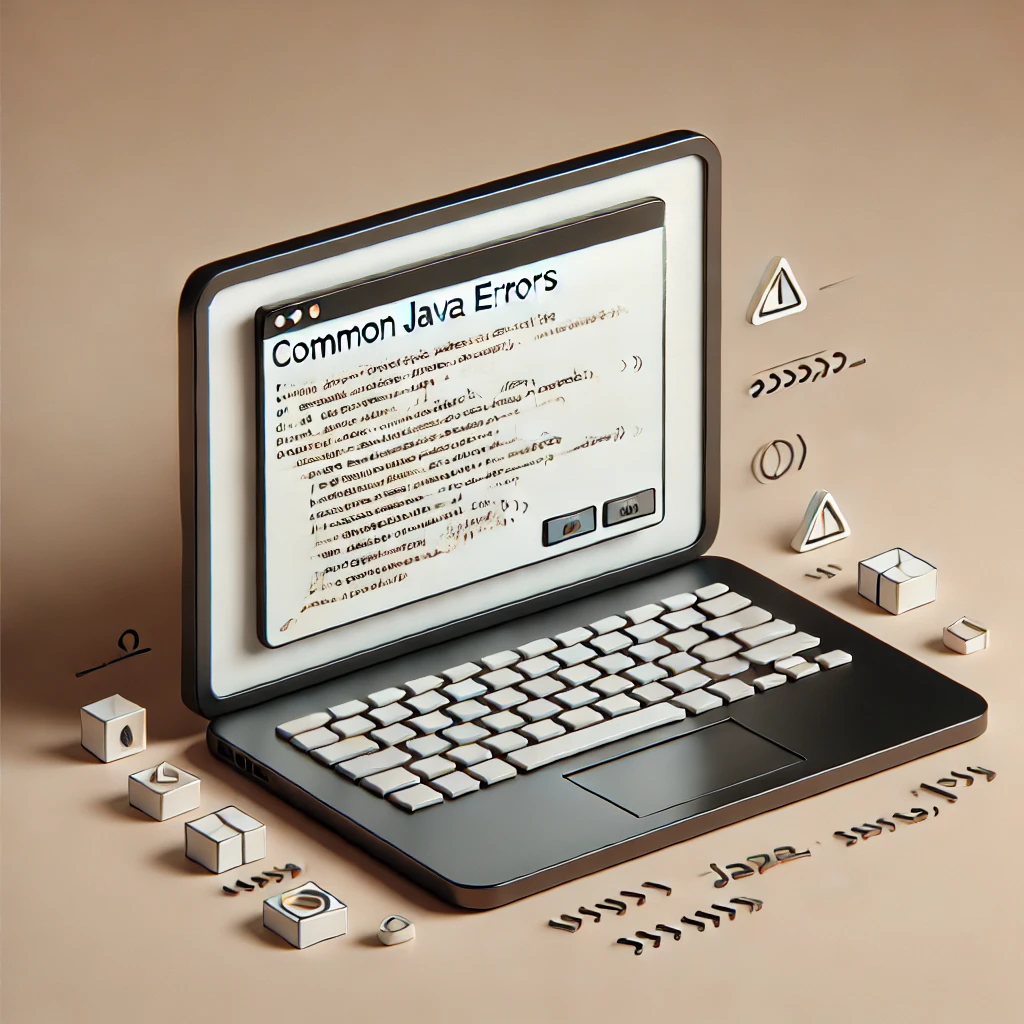
Common Java Errors and How to Fix Them: A Developer’s Guide to Smoother Coding
Java is a powerful and versatile programming language, but like any complex tool, it comes with its fair share of challenges. As developers, we’ve all been there – staring at an error message, scratching our heads, and wondering where we went wrong. But fear not! In this comprehensive guide, we’ll dive into some of the most common Java errors you’re likely to encounter and, more importantly, how to fix them. Whether you’re a seasoned pro or just starting your Java journey, this blog post will help you navigate the treacherous waters of Java programming with confidence. So, grab your favorite caffeinated beverage, fire up your IDE, and let’s embark on this error-busting adventure together!
The Notorious NullPointerException: Taming the Null Beast
Let’s kick things off with the granddaddy of all Java errors: the dreaded NullPointerException. This sneaky little devil has been the bane of Java developers since time immemorial. But what exactly is it, and why does it keep popping up like an uninvited guest at a party?
A NullPointerException occurs when you try to use a reference variable that points to a null object. In simpler terms, you’re trying to do something with an object that doesn’t actually exist. It’s like trying to drive a car that’s not there – you can’t exactly put the pedal to the metal if there’s no pedal to begin with!
Here’s a classic example of code that’s just begging for a NullPointerException:
String str = null;
int length = str.length(); // Boom! NullPointerException
In this case, we’re trying to call the length()
method on a String object that’s null. Java doesn’t take kindly to this sort of behavior and responds with a NullPointerException faster than you can say “Oh no, not again!”
So, how do we fix this? The key is to always check for null before using an object. Here’s a simple way to avoid the NullPointerException in our previous example:
String str = null;
if (str != null) {
int length = str.length();
System.out.println("String length: " + length);
} else {
System.out.println("The string is null");
}
By adding a null check, we’ve effectively tamed the null beast. But wait, there’s more! Java 8 introduced the Optional class, which provides a more elegant way to handle potentially null values. Let’s take a look at how we can use Optional to make our code even more robust:
import java.util.Optional;
String str = null;
Optional<String> optionalStr = Optional.ofNullable(str);
optionalStr.ifPresentOrElse(
s -> System.out.println("String length: " + s.length()),
() -> System.out.println("The string is null")
);
With Optional, we’re explicitly acknowledging that our string might be null, and we’re handling both cases (null and non-null) in a clean, functional style. It’s like giving your code a suit and tie – suddenly, it’s all grown up and ready to handle the null-infested world with grace and poise.
ArrayIndexOutOfBoundsException: Staying Within the Lines
Picture this: you’re coloring a beautiful picture, but you accidentally color outside the lines. That’s essentially what happens when you encounter an ArrayIndexOutOfBoundsException in Java. You’re trying to access an array element that doesn’t exist, and Java is not amused.
This exception occurs when you attempt to access an array index that’s either negative or greater than or equal to the array’s length. Let’s look at a simple example:
int[] numbers = {1, 2, 3, 4, 5};
System.out.println(numbers[5]); // Oops! ArrayIndexOutOfBoundsException
In this case, we’re trying to access the sixth element of an array that only has five elements. Remember, array indices in Java start at 0, so the valid indices for this array are 0 through 4. Trying to access index 5 is like trying to find the sixth toe on a normal human foot – it’s just not there!
So, how do we avoid coloring outside the lines? The key is to always check the array’s length before accessing its elements. Here’s a safer way to work with arrays:
int[] numbers = {1, 2, 3, 4, 5};
int index = 5;
if (index >= 0 && index < numbers.length) {
System.out.println(numbers[index]);
} else {
System.out.println("Index out of bounds!");
}
By adding a simple check, we’ve turned our code into a responsible adult who always looks both ways before crossing the street. But wait, there’s an even more elegant solution! Enter the foreach loop, Java’s way of saying “Hey, let’s make array iteration foolproof”:
int[] numbers = {1, 2, 3, 4, 5};
for (int number : numbers) {
System.out.println(number);
}
With the foreach loop, we’re guaranteed to stay within the bounds of the array. It’s like having a personal tour guide who makes sure you don’t wander off the designated path. No more ArrayIndexOutOfBoundsExceptions, just smooth sailing through your array elements.
ClassNotFoundException: The Case of the Missing Class
Imagine you’re at a party, and you’re looking for your friend Bob. You search high and low, but Bob is nowhere to be found. That’s essentially what happens when Java throws a ClassNotFoundException – it’s looking for a class that simply isn’t there.
This exception typically occurs when you’re trying to load a class using Class.forName() or when the Java ClassLoader is unable to find a class during runtime. Here’s an example of code that might throw a ClassNotFoundException:
try {
Class.forName("com.example.NonExistentClass");
} catch (ClassNotFoundException e) {
System.out.println("Oops! Class not found: " + e.getMessage());
}
In this case, we’re trying to load a class that doesn’t exist (unless you happen to have a class named NonExistentClass in the com.example package, in which case, kudos for your prescient naming skills!).
So, how do we fix this? The first step is to make sure that the class you’re trying to load actually exists and is in the correct package. Double-check your class name and package structure. If you’re using external libraries, ensure that the necessary JAR files are in your classpath.
Here’s an example of how to properly load a class and handle potential exceptions:
try {
Class<?> myClass = Class.forName("java.util.ArrayList");
System.out.println("Class loaded successfully: " + myClass.getName());
} catch (ClassNotFoundException e) {
System.out.println("Class not found: " + e.getMessage());
} catch (Exception e) {
System.out.println("An unexpected error occurred: " + e.getMessage());
}
In this example, we’re loading the ArrayList class, which we know exists in the java.util package. We’re also catching any unexpected exceptions, because in the world of programming, it’s always good to expect the unexpected!
Remember, ClassNotFoundException is a checked exception, which means Java forces you to handle it explicitly. It’s like a strict teacher making sure you’ve done your homework – annoying at times, but ultimately for your own good.
IOException: When Input/Output Goes Awry
In the digital world, input and output operations are like breathing – essential for life but occasionally prone to hiccups. That’s where IOException comes in. This exception is thrown when an input/output operation fails or is interrupted. It’s like trying to read a book in the dark or write with a pen that’s run out of ink – sometimes, things just don’t go as planned.
IOExceptions can occur in various scenarios, such as reading from or writing to files, network operations, or any other I/O-related tasks. Here’s a simple example that might throw an IOException:
import java.io.*;
try {
FileReader file = new FileReader("nonexistent.txt");
BufferedReader reader = new BufferedReader(file);
String line = reader.readLine();
System.out.println(line);
reader.close();
} catch (IOException e) {
System.out.println("An I/O error occurred: " + e.getMessage());
}
In this case, we’re trying to read from a file that doesn’t exist. Unless you have psychic powers and created this file just before running the code, you’re going to encounter an IOException.
So, how do we handle these I/O hiccups? The key is to use proper exception handling and to close your resources properly. Here’s an improved version of our code using try-with-resources, a feature introduced in Java 7 that automatically closes resources for us:
import java.io.*;
import java.nio.file.*;
try (BufferedReader reader = Files.newBufferedReader(Paths.get("example.txt"))) {
String line;
while ((line = reader.readLine()) != null) {
System.out.println(line);
}
} catch (IOException e) {
System.out.println("An I/O error occurred: " + e.getMessage());
}
In this version, we’re using the newer Files and Paths classes from the java.nio package, which provide more powerful and flexible I/O operations. The try-with-resources statement ensures that our BufferedReader is closed automatically, even if an exception occurs. It’s like having a responsible friend who always remembers to turn off the lights and lock the door when leaving – no need to worry about cleaning up!
ConcurrentModificationException: The Perils of Multitasking
In the world of Java collections, ConcurrentModificationException is like that annoying person who keeps moving your stuff while you’re trying to organize it. This exception occurs when you’re iterating over a collection and the collection is modified concurrently. It’s Java’s way of saying, “Hey! I can’t keep track of things if you keep changing them behind my back!”
Here’s a classic example of code that’s likely to throw a ConcurrentModificationException:
import java.util.*;
List<String> fruits = new ArrayList<>(Arrays.asList("Apple", "Banana", "Cherry"));
for (String fruit : fruits) {
if (fruit.equals("Banana")) {
fruits.remove(fruit); // Uh-oh, modifying while iterating!
}
}
In this case, we’re trying to remove an element from the list while iterating over it. This is a big no-no in the Java world, akin to trying to remove a card from a house of cards while it’s still standing – things are bound to collapse!
So, how do we avoid this house of cards situation? One approach is to use an Iterator and its remove() method:
import java.util.*;
List<String> fruits = new ArrayList<>(Arrays.asList("Apple", "Banana", "Cherry"));
Iterator<String> iterator = fruits.iterator();
while (iterator.hasNext()) {
String fruit = iterator.next();
if (fruit.equals("Banana")) {
iterator.remove(); // Safely remove the element
}
}
System.out.println(fruits); // Output: [Apple, Cherry]
By using an Iterator, we’re playing by Java’s rules. It’s like having a responsible adult supervising our collection modifications, ensuring everything stays nice and orderly.
But wait, there’s more! If you’re dealing with multi-threaded scenarios, you might want to consider using thread-safe collections from the java.util.concurrent package. For example:
import java.util.concurrent.*;
List<String> fruits = new CopyOnWriteArrayList<>(Arrays.asList("Apple", "Banana", "Cherry"));
for (String fruit : fruits) {
if (fruit.equals("Banana")) {
fruits.remove(fruit); // No ConcurrentModificationException here!
}
}
System.out.println(fruits); // Output: [Apple, Cherry]
CopyOnWriteArrayList creates a new copy of the underlying array whenever the list is modified. It’s like having a magical notepad that creates a new page every time you want to make a change – a bit wasteful, perhaps, but very safe and thread-friendly!
OutOfMemoryError: When Java Runs Out of Room
Imagine you’re trying to fit an elephant into a Mini Cooper. That’s essentially what happens when Java throws an OutOfMemoryError – you’re asking it to store more data than it has room for. This error occurs when the Java Virtual Machine (JVM) can’t allocate an object because it’s out of memory, and garbage collection can’t free up enough space.
Here’s a simple (albeit contrived) example that might trigger an OutOfMemoryError:
import java.util.*;
List<byte[]> memoryHog = new ArrayList<>();
while (true) {
memoryHog.add(new byte[1024 * 1024]); // Allocate 1MB each iteration
}
This code keeps allocating memory without ever releasing it, like a digital hoarder who never throws anything away. Eventually, Java will run out of space and throw an OutOfMemoryError.
So, how do we avoid turning our Java programs into digital hoarders? Here are a few strategies:
- Increase heap size: If you’re dealing with large datasets, you might need to give Java more memory to work with. You can do this by adjusting the JVM arguments:
java -Xmx2g MyProgram
This sets the maximum heap size to 2 gigabytes. It’s like giving your elephant a bigger car – sometimes, you just need more space!
- Use memory-efficient data structures: Sometimes, the way you store your data can make a big difference. For example, using a BitSet instead of a boolean[] can significantly reduce memory usage for large arrays of boolean values:
import java.util.BitSet;
// Instead of:
// boolean[] flags = new boolean[1000000];
BitSet flags = new BitSet(1000000);
flags.set(42); // Set bit 42 to true
System.out.println(flags.get(42)); // true
- Profile your application: Use tools like VisualVM or Eclipse Memory Analyzer to identify memory leaks and optimize your code. It’s like having a personal trainer for your Java program, helping it stay lean and efficient!
Remember, OutOfMemoryError is not a regular exception – it’s an Error, which means it’s usually catastrophic and not something you should try to catch and handle. Instead, focus on preventing it by writing memory-efficient code and properly sizing your JVM.
StackOverflowError: The Infinite Loop of Doom
If OutOfMemoryError is like trying to fit an elephant into a Mini Cooper, StackOverflowError is like a never-ending game of “She loves me, she loves me not” with a flower that has infinite petals. This error occurs when the call stack in Java grows too large, typically due to excessive recursion.
Here’s a classic example of code that’s begging for a StackOverflowError:
public class InfiniteRecursion {
public static void recursiveMethod() {
recursiveMethod(); // Call itself without any base case
}
public static void main(String[] args) {
recursiveMethod();
}
}
This code is like a dog chasing its own tail – it goes on forever, or at least until Java runs out of stack space and throws a StackOverflowError.
So, how do we avoid this infinite loop of doom? The key is to always have a base case in your recursive methods. Here’s a corrected version of our previous example, calculating factorial:
public class SafeRecursion {
public static int factorial(int n) {
if (n == 0 || n == 1) {
return 1; // Base case
}
return n * factorial(n - 1); // Recursive case
}
public static void main(String[] args) {
System.out.println(factorial(5)); // Output: 120
}
}
In this version, we have a clear base case (when n is 0 or 1) that stops the recursion. It’s like having a responsible adult supervising our game of “She loves me, she loves me not,” making sure we stop when we run out of petals.
But what if you need to perform a computation that’s too deep for recursion? In such cases, you might want to consider using iteration instead. Here’s an iterative version of our factorial function:
public static int factorialIterative(int n) {
int result = 1;
for (int i = 2; i <= n; i++) {
result *= i;
}
return result;
}
This iterative version accomplishes the same task without the risk of a StackOverflow Error. It’s like trading in our recursive dog-chasing-tail scenario for a well-behaved dog that walks in circles a specific number of times – much more predictable and less likely to cause problems!
NumberFormatException: When Strings and Numbers Don’t Mix
We’ve all been there – trying to convert a String to a number, only to find out that the String isn’t quite as numeric as we thought. That’s when Java throws a NumberFormatException, its way of saying, “Hey, that’s not a number!” This exception occurs when you attempt to convert a String to a numeric type (like int, double, or float), but the String doesn’t represent a valid number.
Here’s a classic example of code that’s likely to throw a NumberFormatException:
String notANumber = "Hello, World!";
int number = Integer.parseInt(notANumber); // NumberFormatException!
In this case, we’re trying to parse the string “Hello, World!” as an integer. Unless you’re working in a very strange number system, this isn’t going to end well.
So, how do we handle these pesky non-numeric strings? The key is to validate your input before trying to parse it. Here’s a more robust way to convert strings to numbers:
public static int safeParseInt(String str) {
try {
return Integer.parseInt(str);
} catch (NumberFormatException e) {
System.out.println("Invalid number format: " + str);
return 0; // or any default value, or you could re-throw the exception
}
}
public static void main(String[] args) {
System.out.println(safeParseInt("42")); // Output: 42
System.out.println(safeParseInt("Hello")); // Output: Invalid number format: Hello \n 0
}
In this version, we’re catching the NumberFormatException and handling it gracefully. It’s like having a bouncer at a numbers-only club – if a string tries to get in that’s not a number, we politely turn it away instead of causing a scene.
But what if you need to determine if a String is a valid number without actually parsing it? Regular expressions to the rescue!
public static boolean isNumeric(String str) {
return str.matches("-?\\d+(\\.\\d+)?");
}
public static void main(String[] args) {
System.out.println(isNumeric("42")); // true
System.out.println(isNumeric("3.14")); // true
System.out.println(isNumeric("-1.5")); // true
System.out.println(isNumeric("Hello")); // false
}
This regex pattern checks for an optional minus sign, followed by one or more digits, optionally followed by a decimal point and more digits. It’s like having a super-smart bouncer who can spot a fake ID (or in this case, a non-numeric string) from a mile away!
ClassCastException: When Objects Play Dress-Up
In the world of Java, objects sometimes try to masquerade as other types of objects. Most of the time, Java’s type system keeps everyone honest. But occasionally, an object tries to pull a fast one, and that’s when ClassCastException crashes the party. This exception occurs when you try to cast an object to a class of which it is not an instance.
Here’s a simple example that’s guaranteed to throw a ClassCastException:
Object obj = "I'm a String";
Integer number = (Integer) obj; // ClassCastException!
In this case, we’re trying to convince Java that a String object is actually an Integer. It’s like trying to convince your cat that it’s a dog – it’s not going to work, and someone (in this case, Java) is going to call you out on it.
So, how do we avoid these embarrassing cases of mistaken identity? The instanceof operator is your friend here:
Object obj = "I'm a String";
if (obj instanceof Integer) {
Integer number = (Integer) obj;
System.out.println("Number: " + number);
} else {
System.out.println("Not an Integer: " + obj);
}
By using instanceof, we’re checking the object’s true identity before trying to cast it. It’s like asking for ID before letting someone into the “Integer-only” club.
But wait, there’s more! Java 14 introduced pattern matching for instanceof, which makes this process even smoother:
Object obj = "I'm a String";
if (obj instanceof Integer number) {
System.out.println("Number: " + number);
} else {
System.out.println("Not an Integer: " + obj);
}
With pattern matching, we’re combining the type check and the cast into a single, elegant operation. It’s like having a bouncer who not only checks IDs but also hands out name tags – efficient and stylish!
Debugging with Confidence
We’ve journeyed through some of the most common Java errors, from the notorious NullPointerException to the sneaky ClassCastException. Armed with this knowledge, you’re now better equipped to face these challenges head-on. Remember, errors are not your enemies – they’re opportunities to learn and improve your code.
As you continue your Java adventure, keep these tips in mind:
- Read the error message carefully: Java often tells you exactly what went wrong and where.
- Use a debugger: Step through your code line by line to see where things go awry.
- Write unit tests: Catch errors before they make it to production.
- Keep learning: The Java ecosystem is vast and ever-evolving. Stay curious!
Programming is a journey of continuous learning and improvement. Embrace the errors, learn from them, and watch your code grow stronger and more resilient. Happy coding, and may your exceptions be few and your builds always successful!
Disclaimer: While we strive for accuracy in all our content, the world of programming is vast and ever-changing. If you spot any inaccuracies in this blog post, please let us know so we can correct them promptly. Remember, the best way to truly understand these concepts is through hands-on practice and experimentation. Happy coding!