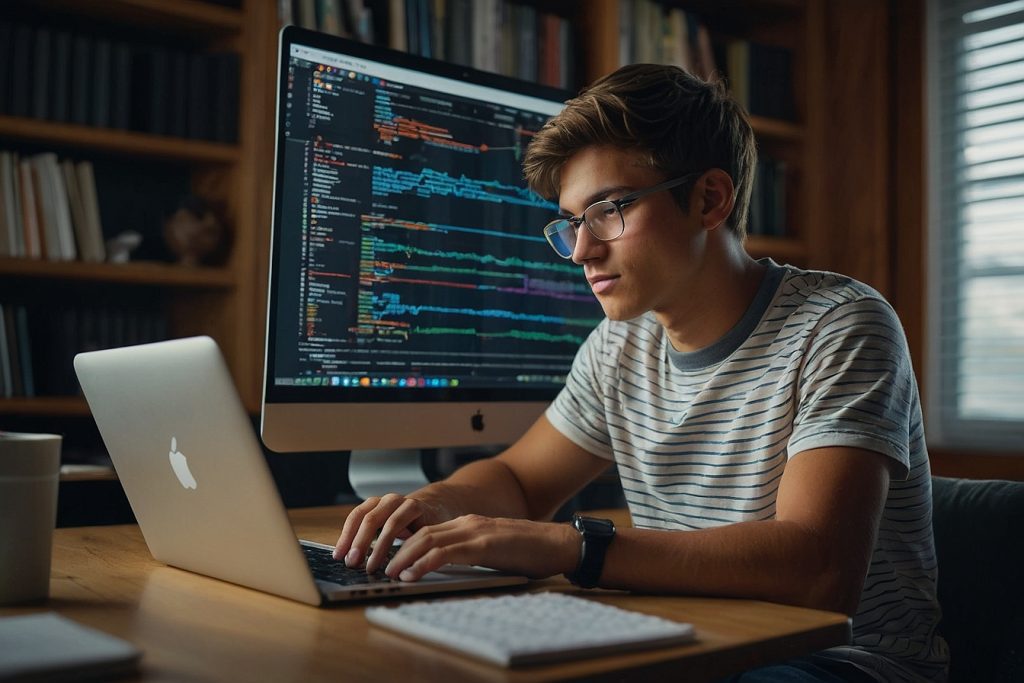
Composition Over Inheritance in Java
Composition Over Inheritance is a software design principle that encourages the use of composition, where one class is composed of other classes, rather than inheritance, where one class extends another, to achieve code reuse and flexibility. This approach can help developers create more maintainable, flexible, and extensible software solutions by promoting loose coupling, modularity, and reduced complexity. In this blog post, we’ll explore the concept of Composition Over Inheritance in Java, discuss its benefits, and provide practical tips and examples to help you apply this powerful design principle to your projects.
Understanding Composition Over Inheritance
Inheritance is a fundamental concept in object-oriented programming that enables one class to inherit properties and methods from another class. While inheritance can provide a simple way to reuse code and create a well-defined class hierarchy, it can also lead to inflexible and tightly-coupled code, especially when used excessively.
Composition Over Inheritance is a design principle that promotes the use of composition, where one class is made up of instances of other classes, instead of inheritance for achieving code reuse and flexibility. By favoring composition over inheritance, developers can create loosely-coupled, modular, and more maintainable software solutions.
Benefits of Composition Over Inheritance
Implementing Composition Over Inheritance in Java development offers several benefits:
- Increased flexibility: Composition allows for greater flexibility in your code, as you can easily change the behavior of a class by replacing or modifying its composed components without modifying the class itself.
- Enhanced modularity: Favoring composition over inheritance encourages the creation of modular and self-contained components that can be easily combined, reused, and extended.
- Reduced complexity: By using composition, you can avoid the complexity and tight coupling that can result from deep inheritance hierarchies, making your code easier to understand, maintain, and test.
- Improved code reuse: Composition enables better code reuse by promoting the creation of small, focused components that can be easily reused and combined in different contexts.
Tips for Implementing Composition Over Inheritance in Java Development
To effectively apply Composition Over Inheritance in your Java projects, consider the following tips:
- Use interfaces: Leverage interfaces to define the contract for your components, making them more modular and easier to replace or extend.
- Favor delegation: Use delegation, where a class delegates some of its responsibilities to another class, instead of inheritance to reuse code and create more flexible and maintainable software solutions.
- Apply design patterns: Design patterns, such as the Strategy, Decorator, and Composite patterns, can help you implement Composition Over Inheritance in your Java code and create well-structured, maintainable software solutions.
- Keep inheritance hierarchies shallow: While inheritance still has its place, try to keep inheritance hierarchies shallow and focused, using composition for greater flexibility and maintainability.
Practical Examples of Composition Over Inheritance in Java
To illustrate Composition Over Inheritance in Java development, let’s examine the following code implementation:
Strategy pattern: The Strategy pattern is a behavioral design pattern that enables selecting an algorithm at runtime. By using composition, you can create flexible and maintainable software solutions that can easily adapt to new requirements.
This example encapsulates the interest calculation logic for each account type into separate strategy implementations. The Account
class (context) uses these strategies to calculate interest, allowing for the interest calculation logic to be changed dynamically at runtime without modifying the account classes.
interface InterestCalculationStrategy {
double calculateInterest(double accountBalance);
}
class SavingsAccountInterestStrategy implements InterestCalculationStrategy {
@Override
public double calculateInterest(double accountBalance) {
// Simplified interest calculation for demonstration
return accountBalance * 0.03; // 3% interest rate
}
}
class CheckingAccountInterestStrategy implements InterestCalculationStrategy {
@Override
public double calculateInterest(double accountBalance) {
return accountBalance * 0.01; // 1% interest rate
}
}
class FixedDepositAccountInterestStrategy implements InterestCalculationStrategy {
@Override
public double calculateInterest(double accountBalance) {
return accountBalance * 0.05; // 5% interest rate
}
}
class BankAccount {
private double balance;
private InterestCalculationStrategy interestCalculationStrategy;
public BankAccount(double balance, InterestCalculationStrategy strategy) {
this.balance = balance;
this.interestCalculationStrategy = strategy;
}
public void setInterestCalculationStrategy(InterestCalculationStrategy strategy) {
this.interestCalculationStrategy = strategy;
}
public void addInterest() {
double interest = interestCalculationStrategy.calculateInterest(balance);
System.out.printf("Adding interest: $%.2f\n", interest);
balance += interest;
}
public double getBalance() {
return balance;
}
}
public class RetailBankingSystemDemo {
public static void main(String[] args) {
BankAccount savings = new BankAccount(1000, new SavingsAccountInterestStrategy());
BankAccount checking = new BankAccount(2000, new CheckingAccountInterestStrategy());
BankAccount fixedDeposit = new BankAccount(5000, new FixedDepositAccountInterestStrategy());
savings.addInterest();
checking.addInterest();
fixedDeposit.addInterest();
System.out.printf("Savings Account Balance: $%.2f\n", savings.getBalance());
System.out.printf("Checking Account Balance: $%.2f\n", checking.getBalance());
System.out.printf("Fixed Deposit Account Balance: $%.2f\n", fixedDeposit.getBalance());
}
}
Summary:
- The
InterestCalculationStrategy
interface defines the contract for interest calculation strategies with a single methodcalculateInterest
. - Concrete strategy classes (
SavingsAccountInterestStrategy
,CheckingAccountInterestStrategy
,FixedDepositAccountInterestStrategy
) implement theInterestCalculationStrategy
interface, each providing a different way to calculate interest for an account. - The
BankAccount
class acts as the context, holding a reference to anInterestCalculationStrategy
. It delegates the interest calculation to the strategy object, allowing for the use of different interest calculation methods. - In the
RetailBankingSystemDemo
main method, we create instances ofBankAccount
with different strategies to demonstrate how interest is calculated differently based on the account type.
This approach allows for easy extension and modification of interest calculation logic without changing the BankAccount
class, adhering to the open/closed principle and demonstrating the use of composition over inheritance for flexible system behavior.
Decorator pattern: The Decorator pattern is a structural design pattern that enables adding new functionality to an object without altering its structure. By using composition, you can create extensible and maintainable software solutions that can easily incorporate new features.
Here’s an example that demonstrates using the Decorator Pattern to add features to bank accounts. We can use the Decorator Pattern to add features to bank accounts dynamically. For instance, we might want to add various services like overdraft protection, international transfer capabilities, or premium customer services to only specific accounts without changing the account classes themselves.
// Component Interface
interface BankAccount {
void deposit(double amount);
void withdraw(double amount);
double getBalance();
}
// Concrete Component
class BasicAccount implements BankAccount {
private double balance;
@Override
public void deposit(double amount) {
balance += amount;
}
@Override
public void withdraw(double amount) {
balance -= amount;
}
@Override
public double getBalance() {
return balance;
}
}
// Decorator Base Class
abstract class AccountDecorator implements BankAccount {
protected BankAccount decoratedAccount;
public AccountDecorator(BankAccount account) {
this.decoratedAccount = account;
}
public void deposit(double amount) {
decoratedAccount.deposit(amount);
}
public void withdraw(double amount) {
decoratedAccount.withdraw(amount);
}
public double getBalance() {
return decoratedAccount.getBalance();
}
}
// Concrete Decorator
class OverdraftProtection extends AccountDecorator {
private double overdraftLimit;
public OverdraftProtection(BankAccount account, double overdraftLimit) {
super(account);
this.overdraftLimit = overdraftLimit;
}
@Override
public void withdraw(double amount) {
if (amount > decoratedAccount.getBalance() + overdraftLimit) {
System.out.println("Withdrawal amount exceeds overdraft limit.");
} else {
decoratedAccount.withdraw(amount);
}
}
}
// Another Concrete Decorator
class InterestEarningFeature extends AccountDecorator {
private double interestRate;
public InterestEarningFeature(BankAccount account, double interestRate) {
super(account);
this.interestRate = interestRate;
}
public void addInterest() {
double interest = decoratedAccount.getBalance() * interestRate / 100;
System.out.printf("Adding interest: $%.2f\n", interest);
decoratedAccount.deposit(interest);
}
}
// Demonstration
public class RetailBankingSystem {
public static void main(String[] args) {
BankAccount basicAccount = new BasicAccount();
basicAccount.deposit(1000);
BankAccount overdraftProtectedAccount = new OverdraftProtection(basicAccount, 500);
overdraftProtectedAccount.withdraw(1400); // Withdrawal within overdraft limit
BankAccount interestEarningAccount = new InterestEarningFeature(basicAccount, 5);
if (interestEarningAccount instanceof InterestEarningFeature) {
((InterestEarningFeature) interestEarningAccount).addInterest();
}
System.out.println("Final Balance: " + basicAccount.getBalance());
}
}
Summary:
BankAccount
is the component interface with basic banking operations.BasicAccount
is a concrete component implementingBankAccount
, representing a simple bank account.AccountDecorator
is an abstract decorator implementing theBankAccount
interface and holding a reference to aBankAccount
object. It delegates all operations to the decorated account object.OverdraftProtection
andInterestEarningFeature
are concrete decorators extendingAccountDecorator
to add specific functionalities (overdraft protection and interest earning, respectively) to theBankAccount
object they decorate.
The Decorator Pattern allows us to dynamically add responsibilities to objects without modifying their existing classes, providing a flexible alternative to subclassing for extending functionality.
Balancing Composition Over Inheritance with Other Design Concerns
While Composition Over Inheritance is a powerful design principle, it’s essential to balance it with other software design considerations, such as performance, readability, and maintainability. In some cases, inheritance may be the most appropriate solution for a particular problem, while in other cases, composition might be more suitable. By considering the specific needs and requirements of your project, you can make informed decisions about when to use composition, inheritance, or a combination of both.
Takeaway:
Composition Over Inheritance is a valuable design principle that can help Java developers create more flexible, maintainable, and extensible software solutions by encouraging the use of composition instead of inheritance. By embracing this principle and applying best practices, you can build software that is easier to understand, modify, and extend, resulting in more robust and efficient solutions.
To effectively implement Composition Over Inheritance in your Java projects, remember to:
- Use interfaces to define contracts for your components.
- Favor delegation over inheritance to create flexible and maintainable software solutions.
- Apply design patterns that support Composition Over Inheritance, such as Strategy, Decorator, and Composite patterns.
- Keep inheritance hierarchies shallow and focused, using composition when greater flexibility is required.
By mastering Composition Over Inheritance and incorporating it into your Java development practices, you can build high-quality, modular, and maintainable software solutions that stand the test of time.