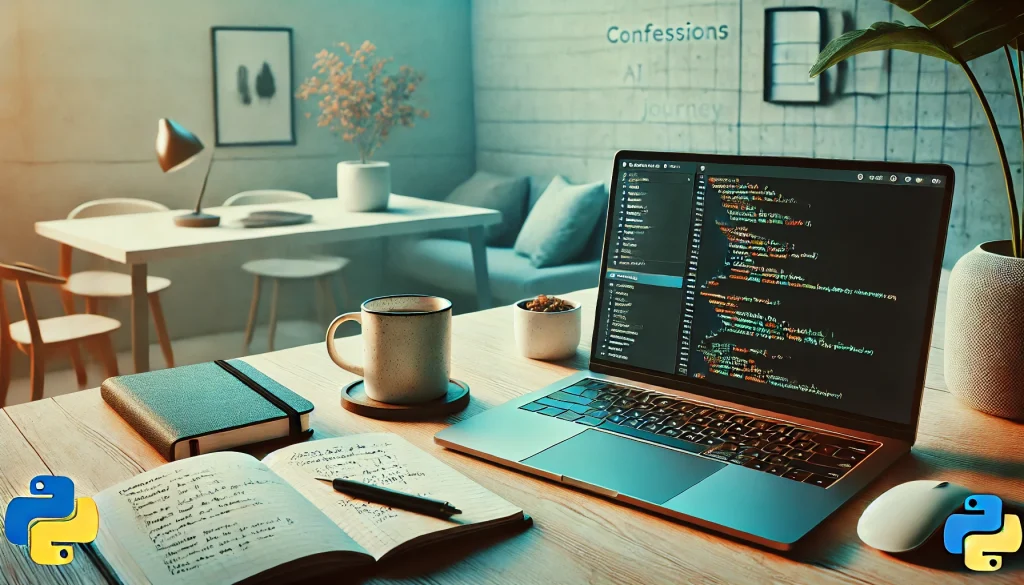
Confessions of a Python Newbie: My AI Learning Journey
Hello, world! I’m excited to share my journey of diving into the fascinating world of Artificial Intelligence (AI) using Python. It’s been a whirlwind of emotions, from the initial trepidation to the exhilaration of seeing my first model in action. This blog aims to recount my experiences, the challenges I faced, and the lessons learned along the way. So, buckle up and join me as I navigate the labyrinth of AI with Python.
The Spark: Discovering AI and Python
Finding My Motivation
Like many tech enthusiasts, my interest in AI was piqued by the groundbreaking advancements and applications making headlines. From self-driving cars to intelligent personal assistants, AI seemed like a realm of endless possibilities. I was particularly fascinated by how AI could solve complex problems, automate tasks, and even create art. But where to start? Python, with its simple syntax and powerful libraries, was the obvious choice.
Getting Started with Python
My first encounter with Python was when I was doing my MSc in Advanced Software Engineering, that was a few years back, it was both thrilling and intimidating. I had some experience with other programming languages, but Python’s readability and extensive libraries were entirely new to me. I started with the basics: understanding variables, loops, and functions. Here’s a snippet of my very first Python script:
# My First Python Script
print("Hello, world!")
Seeing “Hello, world!” printed on my screen was a small yet significant victory. It marked the beginning of my journey into the depths of AI.
Diving Deeper: Understanding the Basics
Mastering Python Fundamentals
To build a solid foundation, I immersed myself in Python’s fundamentals. I learned about data types, control structures, and error handling. I found online courses, tutorials, and books incredibly helpful. Here’s an example of a basic function I wrote to calculate the factorial of a number:
def factorial(n):
if n == 0:
return 1
else:
return n * factorial(n-1)
# Test the function
print(factorial(5)) # Output: 120
Understanding recursion was a bit challenging, but practicing these concepts made them clear over time.
Libraries Galore
Python’s strength lies in its extensive libraries. I started exploring popular libraries like NumPy and pandas for data manipulation and analysis. Here’s a simple example of how I used pandas to analyze a dataset:
import pandas as pd
# Load dataset
data = pd.read_csv('data.csv')
# Display the first 5 rows
print(data.head())
These libraries are the building blocks of AI and machine learning in Python, making complex tasks more manageable.
First Steps into AI: Machine Learning Basics
Understanding Machine Learning
Machine learning (ML) is a subset of AI that enables computers to learn from data. I started with supervised learning, where the model is trained on labeled data. My first project was a simple linear regression model to predict housing prices. Here’s a snippet of my code:
import numpy as np
import pandas as pd
from sklearn.model_selection import train_test_split
from sklearn.linear_model import LinearRegression
# Load dataset
data = pd.read_csv('housing.csv')
# Prepare data
X = data[['Area', 'Bedrooms', 'Age']]
y = data['Price']
# Split data
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=0)
# Train model
model = LinearRegression()
model.fit(X_train, y_train)
# Make predictions
predictions = model.predict(X_test)
# Evaluate model
print(model.score(X_test, y_test))
Seeing the model make predictions was incredibly rewarding. It was my first taste of how powerful machine learning could be.
Challenges Along the Way
The journey wasn’t without hurdles. Understanding the intricacies of ML algorithms, tuning hyperparameters, and handling overfitting were all challenging. But each obstacle was a learning opportunity, and the satisfaction of overcoming them kept me motivated.
Exploring Advanced Topics: Deep Learning and Neural Networks
Introduction to Deep Learning
As I grew more comfortable with machine learning, I ventured into deep learning, a more complex subset of AI. Deep learning involves neural networks with multiple layers, mimicking the human brain’s structure. I started with the basics of neural networks and worked my way up to more complex architectures.
Building My First Neural Network
Using TensorFlow and Keras, I built my first neural network to classify handwritten digits from the MNIST dataset. Here’s a simplified version of my code:
import tensorflow as tf
from tensorflow.keras import layers, models
# Load dataset
mnist = tf.keras.datasets.mnist
(x_train, y_train), (x_test, y_test) = mnist.load_data()
# Normalize data
x_train, x_test = x_train / 255.0, x_test / 255.0
# Build model
model = models.Sequential([
layers.Flatten(input_shape=(28, 28)),
layers.Dense(128, activation='relu'),
layers.Dropout(0.2),
layers.Dense(10, activation='softmax')
])
# Compile model
model.compile(optimizer='adam',
loss='sparse_categorical_crossentropy',
metrics=['accuracy'])
# Train model
model.fit(x_train, y_train, epochs=5)
# Evaluate model
model.evaluate(x_test, y_test)
Training the model and seeing it achieve high accuracy was exhilarating. It was a testament to the potential of deep learning.
Overcoming Deep Learning Challenges
Deep learning presented new challenges, such as understanding the architecture of neural networks, dealing with large datasets, and requiring significant computational power. However, the online community and abundant resources were invaluable in overcoming these obstacles.
Real-World Applications: Implementing AI Projects
Project 1: Sentiment Analysis
One of my favorite projects was a sentiment analysis tool. I used natural language processing (NLP) techniques to analyze the sentiment of text data. Here’s a snippet of the code I used:
import pandas as pd
from sklearn.model_selection import train_test_split
from sklearn.feature_extraction.text import CountVectorizer
from sklearn.naive_bayes import MultinomialNB
# Load dataset
data = pd.read_csv('reviews.csv')
# Prepare data
X = data['review']
y = data['sentiment']
# Vectorize text data
vectorizer = CountVectorizer()
X = vectorizer.fit_transform(X)
# Split data
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=0)
# Train model
model = MultinomialNB()
model.fit(X_train, y_train)
# Make predictions
predictions = model.predict(X_test)
# Evaluate model
print(model.score(X_test, y_test))
The ability to classify text sentiment accurately opened up numerous possibilities, from customer feedback analysis to social media monitoring.
Project 2: Image Classification
Another exciting project was building an image classification model. Using convolutional neural networks (CNNs), I created a model to classify images from the CIFAR-10 dataset. Here’s a simplified version of my code:
import tensorflow as tf
from tensorflow.keras import layers, models
# Load dataset
cifar10 = tf.keras.datasets.cifar10
(x_train, y_train), (x_test, y_test) = cifar10.load_data()
# Normalize data
x_train, x_test = x_train / 255.0, x_test / 255.0
# Build model
model = models.Sequential([
layers.Conv2D(32, (3, 3), activation='relu', input_shape=(32, 32, 3)),
layers.MaxPooling2D((2, 2)),
layers.Conv2D(64, (3, 3), activation='relu'),
layers.MaxPooling2D((2, 2)),
layers.Conv2D(64, (3, 3), activation='relu'),
layers.Flatten(),
layers.Dense(64, activation='relu'),
layers.Dense(10, activation='softmax')
])
# Compile model
model.compile(optimizer='adam',
loss='sparse_categorical_crossentropy',
metrics=['accuracy'])
# Train model
model.fit(x_train, y_train, epochs=10, validation_data=(x_test, y_test))
This project not only enhanced my understanding of CNNs but also demonstrated the practical applications of image classification in various fields, from healthcare to autonomous driving.
Continuous Learning: Staying Updated with AI Trends
Keeping Up with the AI Community
AI is a rapidly evolving field, and staying updated is crucial. I joined online forums, attended webinars, and participated in hackathons to keep up with the latest trends and advancements. Engaging with the AI community provided invaluable insights and fostered a sense of camaraderie.
Exploring New Horizons
With a solid foundation in AI and Python, I started exploring more advanced topics like reinforcement learning, generative adversarial networks (GANs), and AI ethics. Each new topic expanded my understanding and opened up new avenues for exploration and application.
Reflection: The Journey So Far and Future Aspirations
Looking Back
Reflecting on my journey, I’m amazed at how far I’ve come. From struggling with basic syntax to building complex models, the progress has been substantial. Each project, each line of code, and each error message has contributed to my growth as an AI enthusiast.
Future Goals
Looking ahead, I aim to delve deeper into AI research, contribute to open-source projects, and perhaps even develop AI applications that can make a positive impact on society. The possibilities are endless, and I’m excited to see where this journey will take me.
Encouragement for Fellow Newbies
To my fellow newbies embarking on this journey, remember that persistence is key. The learning curve might be steep, and the challenges might seem daunting, but the rewards are worth it. Surround yourself with supportive communities, leverage the plethora of resources available, and never hesitate to experiment. Each mistake is a step closer to mastery.
Conclusion
The path to mastering AI with Python is an ongoing adventure filled with highs and lows. Embrace each moment, from the small victories of running your first script to the larger triumphs of deploying a successful model. This journey is not just about acquiring technical skills but also about fostering a mindset of curiosity and resilience.
If you’re passionate about AI, there’s no better time to start than now. Whether you’re a student, a professional looking to pivot careers, or simply a curious mind, dive into the world of Python and AI. Start small, stay consistent, and soon enough, you’ll find yourself making significant strides in this exciting field.
Disclaimer
This blog is based on my personal experiences and learning journey in AI and Python. While I strive to provide accurate and helpful information, some details may vary depending on individual experiences and the evolving nature of the field. If you notice any inaccuracies or have suggestions for improvement, please feel free to report them so I can make necessary corrections promptly.