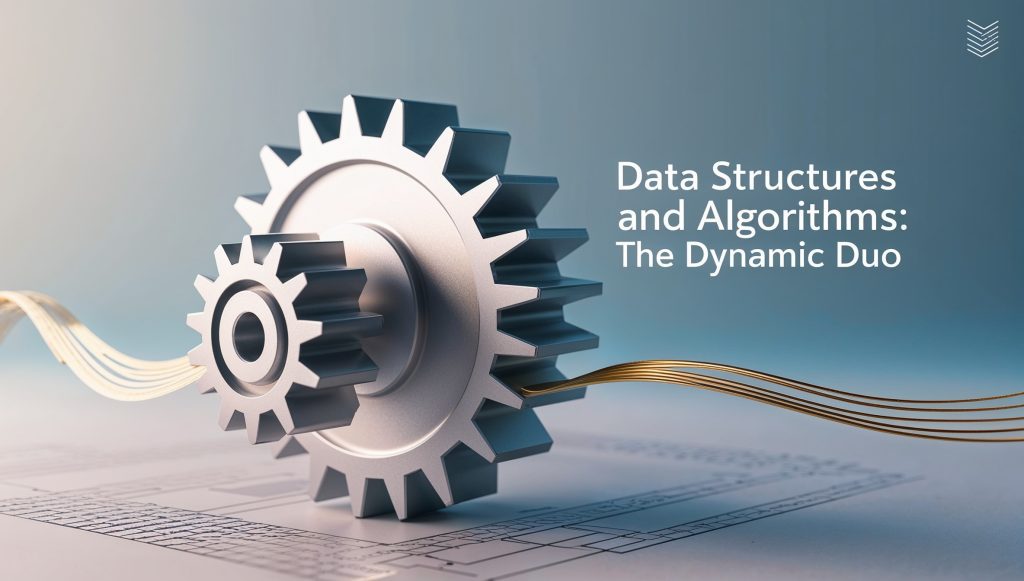
Data Structures and Algorithms: The Dynamic Duo of Efficient Programming
Picture yourself as a master chef in the kitchen of programming. In one hand, you hold a diverse array of ingredients – these are your data structures. In the other, you clutch a book of time-tested recipes – these are your algorithms. Just as the perfect dish comes from combining the right ingredients with the ideal cooking method, elegant and efficient code emerges when you pair the appropriate data structure with the most suitable algorithm.
Welcome to the flavorful world of Data Structures and Algorithms – the essential ingredients and recipes for cooking up efficient, optimized code. In this post, we’ll explore how these two fundamental concepts in computer science work in tandem to solve complex problems and power the software that runs our digital lives.
What Are Data Structures?
Imagine walking into a well-organized pantry. You see shelves neatly stocked with canned goods, a refrigerator for perishables, and maybe even a spice rack for quick access to seasonings. Each of these storage solutions is designed to hold specific types of ingredients efficiently. In the world of programming, data structures serve a similar purpose.
Definition: Data structures are specialized formats for organizing, storing, and managing data in a computer’s memory. They provide a way to collect and arrange data so that it can be used efficiently.
Just as you wouldn’t store ice cream in a cupboard or dry pasta in the fridge, choosing the right data structure for your specific needs is crucial for writing efficient code. Let’s take a quick tour through some common data structures:
Arrays: The Swiss Army Knife of Data Structures
- Picture an array as a line of boxes, each holding a piece of data.
- Great for storing and accessing elements in a specific order.
- Ideal when you know the size of your data in advance.
Linked Lists: The Flexible Chain
- Imagine a chain where each link holds data and points to the next link.
- Excellent for frequent insertions and deletions.
- Dynamic size, but slower for random access.
Stacks: The Pile of Pancakes
- Think of a stack of pancakes – you can only add or remove from the top.
- Perfect for tracking function calls or implementing undo functionality.
- Follows the Last-In-First-Out (LIFO) principle.
Queues: The Checkout Line
- Picture a line at a grocery store – first in, first out.
- Ideal for managing tasks in order, like print jobs or data buffers.
- Follows the First-In-First-Out (FIFO) principle.
Trees: The Family Tree of Data
- Visualize an upside-down tree with a root node and branches.
- Excellent for representing hierarchical relationships.
- Variants like binary search trees are great for efficient searching and sorting.
Graphs: The Social Network of Data Structures
- Think of a map where cities (nodes) are connected by roads (edges).
- Perfect for representing complex relationships and networks.
- Used in social media connections, GPS navigation, and more.
Each of these data structures has its own strengths and weaknesses, much like how different ingredients shine in different recipes. But to truly bring out their potential, we need to pair them with the right algorithms.
What Are Algorithms?
If data structures are the ingredients, then algorithms are the cooking techniques that transform those ingredients into a delectable dish. They’re the step-by-step procedures that tell us how to solve problems or perform tasks.
Definition: An algorithm is a finite sequence of well-defined instructions, typically used to solve a specific problem or perform a computation.
Algorithms are the secret recipes of programming. They define how we should manipulate our data structures to achieve desired outcomes efficiently. Let’s look at a few classic algorithms:
Searching Algorithms: The Hide and Seek Champions
- Linear Search: Like looking through a unsorted drawer, checking each item one by one.
- Binary Search: Imagine searching a dictionary – you start in the middle and eliminate half the remaining options with each step.
Sorting Algorithms: The Great Organizers
- Bubble Sort: Picture bubbles rising in a champagne glass, swapping elements as they go.
- Quick Sort: Think of it as the efficient librarian, quickly categorizing books into rough sections before fine-tuning their order.
Graph Algorithms: The Pathfinders
- Dijkstra’s Algorithm: Like a GPS finding the shortest route between two points.
- Breadth-First Search: Imagine exploring a maze by checking all nearby paths before venturing deeper.
These algorithms, and many others, form the cookbook of computer science. But their true power is unleashed when we pair them with the right data structures.
The Dynamic Duo in Action
Now that we’ve met our star players, let’s see how they work together to create programming magic. Here are a few examples of data structures and algorithms joining forces:
Binary Search on a Sorted Array
When you have a large, sorted array of data, finding a specific element can be like looking for a needle in a haystack. But with the binary search algorithm, we can turn this daunting task into a breeze.
Here’s a Python implementation to illustrate:
def binary_search(arr, target):
left, right = 0, len(arr) - 1
while left <= right:
mid = (left + right) // 2
if arr[mid] == target:
return mid # Found the target
elif arr[mid] < target:
left = mid + 1 # Target is in the right half
else:
right = mid - 1 # Target is in the left half
return -1 # Target not found
# Example usage
sorted_array = [1, 3, 5, 7, 9, 11, 13, 15, 17, 19]
target = 13
result = binary_search(sorted_array, target)
print(f"Element {target} found at index: {result}")
This combination of a sorted array (data structure) and binary search (algorithm) allows us to find elements quickly, even in very large datasets. It’s like having a well-organized bookshelf and knowing exactly how to find any book in seconds!
Dijkstra’s Algorithm on a Graph
Imagine you’re building a GPS navigation system. You need to find the shortest path between two points in a city. This is where graphs (to represent the city’s road network) and Dijkstra’s algorithm (to find the shortest path) come together beautifully.
Here’s a simplified implementation:
import heapq
def dijkstra(graph, start, end):
distances = {node: float('infinity') for node in graph}
distances[start] = 0
pq = [(0, start)]
previous = {node: None for node in graph}
while pq:
current_distance, current_node = heapq.heappop(pq)
if current_node == end:
path = []
while current_node:
path.append(current_node)
current_node = previous[current_node]
return path[::-1], current_distance
if current_distance > distances[current_node]:
continue
for neighbor, weight in graph[current_node].items():
distance = current_distance + weight
if distance < distances[neighbor]:
distances[neighbor] = distance
previous[neighbor] = current_node
heapq.heappush(pq, (distance, neighbor))
return None, float('infinity')
# Example usage
graph = {
'A': {'B': 4, 'C': 2},
'B': {'A': 4, 'C': 1, 'D': 5},
'C': {'A': 2, 'B': 1, 'D': 8, 'E': 10},
'D': {'B': 5, 'C': 8, 'E': 2, 'F': 6},
'E': {'C': 10, 'D': 2, 'F': 3},
'F': {'D': 6, 'E': 3}
}
start, end = 'A', 'F'
path, distance = dijkstra(graph, start, end)
print(f"Shortest path from {start} to {end}: {' -> '.join(path)}")
print(f"Total distance: {distance}")
This pairing of a graph data structure with Dijkstra’s algorithm allows us to solve complex pathfinding problems efficiently. It’s the secret sauce behind many modern navigation systems!
Choosing the Right Pair
Selecting the perfect data structure and algorithm combination is an art form in itself. It’s like being a master chef who knows exactly which ingredients to use and how to prepare them for the best result. Here are some factors to consider:
- Nature of the Data: Is your data inherently hierarchical (use a tree), sequential (maybe an array or linked list), or interconnected (consider a graph)?
- Required Operations: Do you need fast insertions and deletions (linked list might be good) or quick random access (array could be better)?
- Size of the Dataset: For small datasets, simpler structures and algorithms often suffice. Large datasets might require more sophisticated approaches.
- Time Complexity: How fast does your algorithm need to run? Some algorithms are quicker but more complex to implement.
- Space Complexity: How much memory can you afford to use? Some data structures trade space for speed.
- Frequency of Operations: Which operations will be performed most often? Optimize for these.
Remember, the choice of data structure can dramatically impact the efficiency of your algorithm. For instance, searching an unsorted array takes O(n) time, but searching a binary search tree takes only O(log n) on average. It’s all about finding the right tool for the job!
Real-World Applications
The synergy between data structures and algorithms isn’t just theoretical – it powers many of the applications we use daily. Here are a few examples:
- Social Media Feeds
- Data Structure: Graphs (to represent user connections)
- Algorithm: Various recommendation algorithms Your social media feed isn’t random. It’s carefully curated using graph algorithms that analyze your connections, interactions, and preferences to show you content you’re likely to engage with.
- E-commerce Product Search
- Data Structure: Inverted Index (for fast keyword searching)
- Algorithm: TF-IDF (Term Frequency-Inverse Document Frequency) for relevance ranking When you search for a product on an e-commerce site, these structures and algorithms work together to quickly find and rank relevant items from millions of possibilities.
- GPS Navigation
- Data Structure: Weighted Graph (representing road networks)
- Algorithm: A* Search or Dijkstra’s Algorithm (for finding optimal routes) Your GPS doesn’t just find any path from A to B – it finds the optimal path considering distance, traffic, and other factors, all in real-time!
- Video Streaming Services
- Data Structure: Priority Queue
- Algorithm: Adaptive Bitrate Streaming algorithms Streaming services use these to manage video buffering and adjust video quality based on your internet speed, ensuring a smooth viewing experience.
- File Compression
- Data Structure: Huffman Tree
- Algorithm: Huffman Coding When you create a zip file, these work together to efficiently compress your data, saving valuable storage space.
These examples just scratch the surface. From the autocomplete in your search bar to the fraud detection in your bank transactions, the clever pairing of data structures and algorithms is constantly at work, making our digital experiences faster, smarter, and more efficient.
The Recipe for Efficient Code
As we’ve explored, data structures and algorithms are truly the dynamic duo of efficient programming. Like a master chef combining the finest ingredients with time-tested techniques, a skilled programmer knows how to select the right data structure and pair it with the perfect algorithm to cook up efficient, optimized code.
Remember:
- Data structures are the ingredients – they organize and store your data.
- Algorithms are the recipes – they define how to manipulate and process that data.
- The magic happens when you combine them effectively to solve real-world problems.
As you continue your journey in programming, take the time to explore different data structures and algorithms. Experiment with them, implement them from scratch, and practice solving problems using various combinations. Like any skill, mastery comes with practice and experience.
The world of computer science is vast and ever-evolving, with new data structures and algorithms being developed to tackle emerging challenges. By building a strong foundation in these fundamental concepts, you’ll be well-equipped to understand, use, and even contribute to these advancements.
So, the next time you’re faced with a programming challenge, remember to ask yourself: “What’s the best data structure for this job, and which algorithm will help me make the most of it?” With this mindset, you’ll be well on your way to cooking up some truly impressive code!
Happy coding, and may your programs always run with the efficiency of a well-oiled machine and the elegance of a gourmet meal!
Disclaimer:
This blog post provides a foundational understanding of the relationship between data structures and algorithms. Advanced topics like amortized analysis, specialized data structures, and algorithm design paradigms are beyond the scope of this introductory guide. Readers are encouraged to explore further resources and delve deeper into these areas for a more comprehensive understanding.