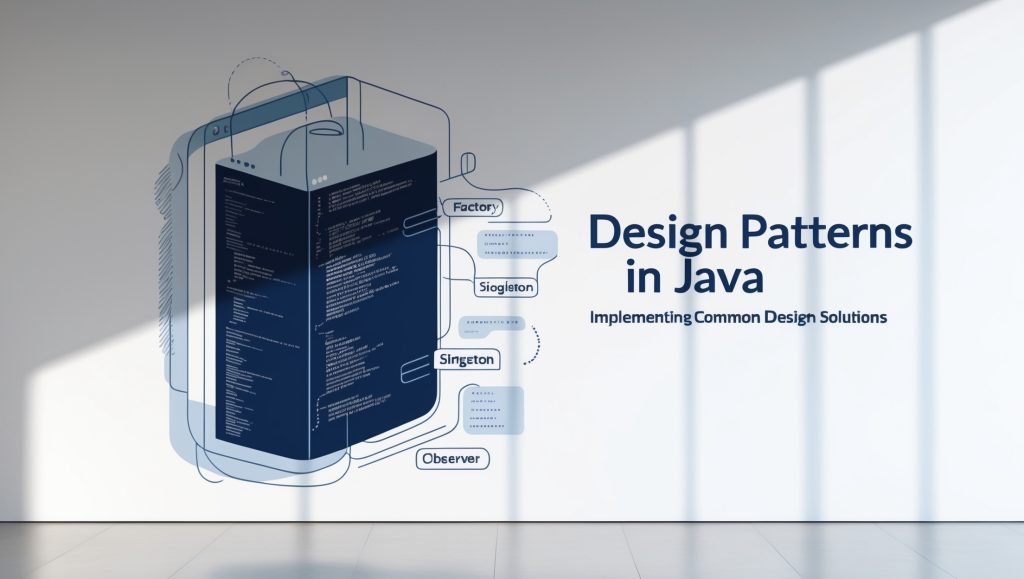
Design Patterns in Java – Implementing common design solutions
Design patterns are essential tools in a developer’s arsenal, representing time-tested solutions to common software design problems. In this comprehensive guide, we’ll explore various design patterns in Java, their practical implementations, and real-world use cases. Whether you’re a seasoned developer or just starting your journey, understanding these patterns will help you write more maintainable, flexible, and robust code.
Understanding Design Patterns
Design patterns are reusable solutions to commonly occurring problems in software design. They provide a template for solving issues that can be used in many different situations. In Java programming, design patterns are particularly important because they help create more maintainable and scalable applications while promoting code reuse and loose coupling.
Types of Design Patterns
Design patterns are typically categorized into three main types:
- Creational Patterns: Deal with object creation mechanisms
- Structural Patterns: Focus on how classes and objects are composed
- Behavioral Patterns: Concentrate on communication between objects
Creational Design Patterns
Creational patterns provide various object creation mechanisms that increase flexibility and reuse of existing code. Let’s explore some of the most commonly used creational patterns in Java.
Singleton Pattern
The Singleton pattern ensures that a class has only one instance and provides a global point of access to that instance. This pattern is particularly useful when exactly one object is needed to coordinate actions across the system.
public class Singleton {
private static Singleton instance;
private static final Object lock = new Object();
private Singleton() {}
public static Singleton getInstance() {
if (instance == null) {
synchronized (lock) {
if (instance == null) {
instance = new Singleton();
}
}
}
return instance;
}
public void showMessage() {
System.out.println("Hello from Singleton!");
}
}
Factory Method Pattern
The Factory Method pattern provides an interface for creating objects but allows subclasses to decide which class to instantiate. It promotes loose coupling by eliminating the need to bind application-specific classes into the code.
// Product interface
interface Animal {
void makeSound();
}
// Concrete products
class Dog implements Animal {
@Override
public void makeSound() {
System.out.println("Woof!");
}
}
class Cat implements Animal {
@Override
public void makeSound() {
System.out.println("Meow!");
}
}
// Factory class
class AnimalFactory {
public Animal createAnimal(String type) {
if (type.equalsIgnoreCase("dog")) {
return new Dog();
} else if (type.equalsIgnoreCase("cat")) {
return new Cat();
}
throw new IllegalArgumentException("Unknown animal type");
}
}
Builder Pattern
The Builder pattern is used to construct complex objects step by step. It’s particularly useful when an object needs to be created with numerous possible configurations.
public class Computer {
private String CPU;
private String RAM;
private String storage;
private String GPU;
private Computer(ComputerBuilder builder) {
this.CPU = builder.CPU;
this.RAM = builder.RAM;
this.storage = builder.storage;
this.GPU = builder.GPU;
}
public static class ComputerBuilder {
private String CPU;
private String RAM;
private String storage;
private String GPU;
public ComputerBuilder(String CPU, String RAM) {
this.CPU = CPU;
this.RAM = RAM;
}
public ComputerBuilder setStorage(String storage) {
this.storage = storage;
return this;
}
public ComputerBuilder setGPU(String GPU) {
this.GPU = GPU;
return this;
}
public Computer build() {
return new Computer(this);
}
}
}
// Usage example
Computer computer = new Computer.ComputerBuilder("Intel i7", "16GB")
.setStorage("1TB SSD")
.setGPU("RTX 3080")
.build();
Structural Design Patterns
Structural patterns deal with object composition and typically identify simple ways to realize relationships between different objects. These patterns help ensure that when one part of a system changes, the entire structure doesn’t need to change.
Adapter Pattern
The Adapter pattern allows incompatible interfaces to work together by wrapping an object in an adapter to make it compatible with another class. This pattern is particularly useful when integrating new features with existing code.
// Target interface
interface MediaPlayer {
void play(String audioType, String fileName);
}
// Adaptee interface
interface AdvancedMediaPlayer {
void playVlc(String fileName);
void playMp4(String fileName);
}
// Concrete Adaptee
class VlcPlayer implements AdvancedMediaPlayer {
@Override
public void playVlc(String fileName) {
System.out.println("Playing vlc file: " + fileName);
}
@Override
public void playMp4(String fileName) {
// Do nothing
}
}
// Adapter
class MediaAdapter implements MediaPlayer {
AdvancedMediaPlayer advancedMusicPlayer;
public MediaAdapter(String audioType) {
if (audioType.equalsIgnoreCase("vlc")) {
advancedMusicPlayer = new VlcPlayer();
}
}
@Override
public void play(String audioType, String fileName) {
if (audioType.equalsIgnoreCase("vlc")) {
advancedMusicPlayer.playVlc(fileName);
}
}
}
Decorator Pattern
The Decorator pattern allows behavior to be added to individual objects dynamically without affecting other objects of the same class. This pattern is often used to comply with the Single Responsibility Principle.
// Component interface
interface Coffee {
double getCost();
String getDescription();
}
// Concrete component
class SimpleCoffee implements Coffee {
@Override
public double getCost() {
return 1.0;
}
@Override
public String getDescription() {
return "Simple coffee";
}
}
// Decorator
abstract class CoffeeDecorator implements Coffee {
protected Coffee decoratedCoffee;
public CoffeeDecorator(Coffee coffee) {
this.decoratedCoffee = coffee;
}
public double getCost() {
return decoratedCoffee.getCost();
}
public String getDescription() {
return decoratedCoffee.getDescription();
}
}
// Concrete decorators
class Milk extends CoffeeDecorator {
public Milk(Coffee coffee) {
super(coffee);
}
public double getCost() {
return super.getCost() + 0.5;
}
public String getDescription() {
return super.getDescription() + ", milk";
}
}
Behavioral Design Patterns
Behavioral patterns are concerned with communication between objects, how objects interact, and distribute responsibility. These patterns help make the communication between objects more flexible and manageable.
Observer Pattern
The Observer pattern defines a one-to-many dependency between objects so that when one object changes state, all its dependents are notified and updated automatically. This pattern is particularly useful in event-driven programming.
import java.util.*;
// Subject interface
interface Subject {
void registerObserver(Observer observer);
void removeObserver(Observer observer);
void notifyObservers();
}
// Observer interface
interface Observer {
void update(String message);
}
// Concrete subject
class NewsAgency implements Subject {
private List<Observer> observers = new ArrayList<>();
private String news;
@Override
public void registerObserver(Observer observer) {
observers.add(observer);
}
@Override
public void removeObserver(Observer observer) {
observers.remove(observer);
}
@Override
public void notifyObservers() {
for (Observer observer : observers) {
observer.update(news);
}
}
public void setNews(String news) {
this.news = news;
notifyObservers();
}
}
// Concrete observer
class NewsChannel implements Observer {
private String name;
public NewsChannel(String name) {
this.name = name;
}
@Override
public void update(String news) {
System.out.println(name + " received news: " + news);
}
}
Strategy Pattern
The Strategy pattern defines a family of algorithms, encapsulates each one, and makes them interchangeable. It lets the algorithm vary independently from clients that use it.
// Strategy interface
interface PaymentStrategy {
void pay(int amount);
}
// Concrete strategies
class CreditCardPayment implements PaymentStrategy {
private String cardNumber;
public CreditCardPayment(String cardNumber) {
this.cardNumber = cardNumber;
}
@Override
public void pay(int amount) {
System.out.println(amount + " paid with credit card " + cardNumber);
}
}
class PayPalPayment implements PaymentStrategy {
private String email;
public PayPalPayment(String email) {
this.email = email;
}
@Override
public void pay(int amount) {
System.out.println(amount + " paid using PayPal account " + email);
}
}
// Context
class ShoppingCart {
private PaymentStrategy paymentStrategy;
public void setPaymentStrategy(PaymentStrategy strategy) {
this.paymentStrategy = strategy;
}
public void checkout(int amount) {
paymentStrategy.pay(amount);
}
}
Best Practices for Implementing Design Patterns
When implementing design patterns in Java, consider the following best practices:
- **Choose Patterns Wisely**: Not every problem requires a design pattern. Use patterns only when they provide clear benefits and solve specific problems.
- **Keep It Simple**: Don’t force patterns where they don’t fit naturally. Sometimes a simple solution is better than a pattern-based one.
- **Consider Maintenance**: Think about how the pattern will affect code maintenance and future modifications.
- **Document Your Patterns**: Clearly document why and how you’re using specific patterns in your code.
- **Understand Pattern Relationships**: Many patterns can work together to solve complex problems. Understanding these relationships is crucial.
Common Anti-Patterns to Avoid
While implementing design patterns, be aware of these common anti-patterns:
Over-Engineering
- Don’t use patterns just because you can
- Avoid implementing patterns prematurely
-
Don’t combine too many patterns unnecessarily
Pattern Misuse
- Using Singleton where global variables would suffice
- Implementing Factory pattern for simple object creation
- Over-complicating simple solutions with unnecessary patterns
Conclusion
Design patterns are powerful tools that can significantly improve code quality and maintainability when used appropriately. They provide tested solutions to common problems and promote code reuse. However, it’s essential to remember that patterns are guidelines, not rules. Always evaluate whether a pattern truly solves your specific problem before implementing it.
Understanding and correctly implementing design patterns in Java requires practice and experience. Start with simple patterns and gradually work your way up to more complex ones. Remember to focus on writing clean, maintainable code that solves real problems rather than implementing patterns for their own sake.
Disclaimer: While every effort has been made to ensure the accuracy of the information and code examples provided in this blog post, software development practices and patterns continue to evolve. The implementations shown are examples and may need to be adapted for specific use cases. If you notice any inaccuracies or have suggestions for improvements, please report them to our editorial team for prompt correction.