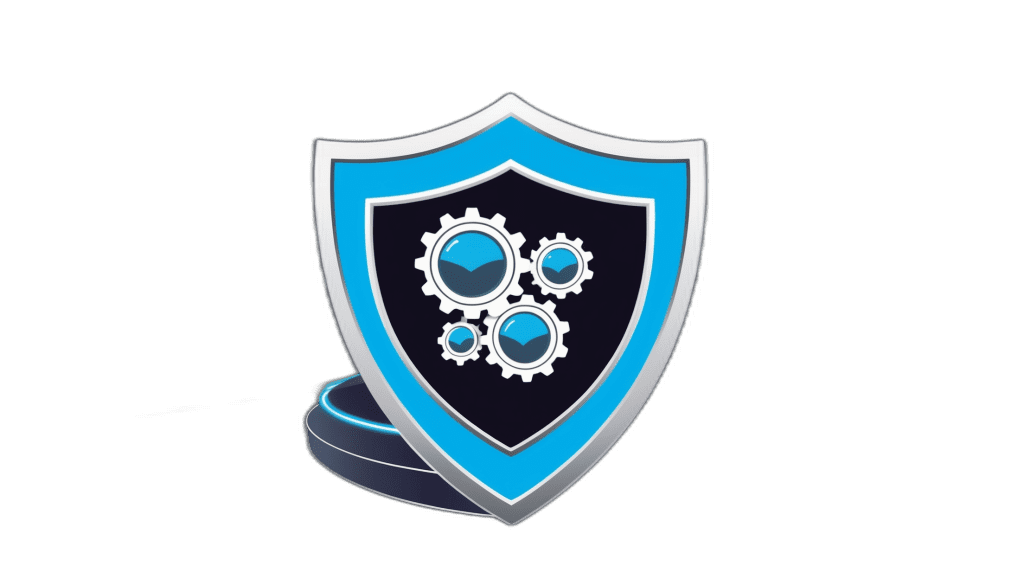
DevOps Superpowers: Unleashing the Power of Continuous Integration and Continuous Deployment
In the fast-paced world of software development, staying ahead of the curve is crucial. Enter DevOps, the game-changing methodology that’s revolutionizing how we build, test, and deploy software. But what if I told you that within DevOps lies a set of superpowers that can transform your development process? Today, we’re diving deep into two of these superpowers: Continuous Integration (CI) and Continuous Deployment (CD). Buckle up, because we’re about to embark on a journey that will change the way you think about software development forever.
The DevOps Revolution: A Brief Overview
Before we dive into the nitty-gritty of CI/CD, let’s take a moment to appreciate the bigger picture. DevOps isn’t just another buzzword; it’s a cultural shift that’s bridging the gap between development and operations teams. By fostering collaboration, automation, and a shared responsibility for the entire software lifecycle, DevOps is helping organizations deliver better software faster than ever before. It’s like giving your entire development process a turbo boost, and CI/CD are the nitrous oxide in this high-octane engine.
Continuous Integration: The First Superpower
What is Continuous Integration?
Imagine a world where code conflicts are a thing of the past, where bugs are caught before they can wreak havoc, and where your team moves in perfect harmony. That’s the world of Continuous Integration. At its core, CI is the practice of regularly merging code changes into a central repository, followed by automated builds and tests. It’s like having a vigilant guardian watching over your codebase, ensuring that every change plays nicely with the rest of your project.
The Benefits of Continuous Integration
CI isn’t just about making developers’ lives easier (although it certainly does that). It’s about improving the overall quality of your software and accelerating your development process. By integrating code changes frequently, you’re able to detect errors quickly, reduce integration problems, and improve software quality. It’s like having a crystal ball that shows you potential issues before they become major headaches.
Implementing Continuous Integration
Now, you might be thinking, “This sounds great, but how do I actually implement CI?” Fear not! Setting up a CI pipeline is easier than you might think. Here’s a basic example using Jenkins, one of the most popular CI tools:
pipeline {
agent any
stages {
stage('Build') {
steps {
sh 'mvn clean package'
}
}
stage('Test') {
steps {
sh 'mvn test'
}
}
stage('Deploy') {
steps {
sh 'mvn deploy'
}
}
}
post {
always {
junit '**/target/surefire-reports/TEST-*.xml'
}
}
}
This Jenkinsfile defines a simple CI pipeline that builds your project, runs tests, and deploys the application. It also includes a post-build action to publish test results. Of course, this is just scratching the surface. As you become more comfortable with CI, you can add more sophisticated steps, like code quality checks, security scans, and performance tests.
Continuous Deployment: The Second Superpower
What is Continuous Deployment?
If Continuous Integration is about merging and testing code changes, Continuous Deployment is about taking those changes and automatically pushing them into production. It’s the natural evolution of CI, taking automation to the next level. With CD, every code change that passes your automated tests is automatically deployed to production. It’s like having a teleportation device for your code, instantly beaming it from your development environment to your users’ hands.
The Benefits of Continuous Deployment
CD isn’t just about speed (although that’s a major benefit). It’s about reducing the risk associated with releases, improving feedback loops, and enabling rapid iteration. By deploying small changes frequently, you’re able to catch and fix issues quickly, gather user feedback faster, and continuously improve your product. It’s like giving your entire development process a nitro boost, allowing you to zoom past the competition.
Implementing Continuous Deployment
Implementing CD builds on your CI pipeline, adding the crucial step of automatic deployment. Here’s an example of how you might extend our previous Jenkins pipeline to include CD:
pipeline {
agent any
stages {
stage('Build') {
steps {
sh 'mvn clean package'
}
}
stage('Test') {
steps {
sh 'mvn test'
}
}
stage('Deploy to Staging') {
steps {
sh 'ansible-playbook -i inventory/staging deploy.yml'
}
}
stage('Run Integration Tests') {
steps {
sh 'mvn integration-test'
}
}
stage('Deploy to Production') {
when {
expression { currentBuild.resultIsBetterOrEqualTo('SUCCESS') }
}
steps {
sh 'ansible-playbook -i inventory/production deploy.yml'
}
}
}
post {
always {
junit '**/target/surefire-reports/TEST-*.xml'
}
}
}
This extended pipeline includes stages for deploying to a staging environment, running integration tests, and then deploying to production if all tests pass. It’s like having a well-oiled machine that takes your code from commit to production with minimal human intervention.
The CI/CD Dream Team: Better Together
While CI and CD are powerful on their own, they truly shine when used together. The combination of CI/CD creates a seamless pipeline that takes your code from commit to production, ensuring quality and speed at every step. It’s like having a superhero duo, each with their own unique powers, working together to save the day (or in this case, your development process).
Faster Time to Market
One of the most significant benefits of CI/CD is the dramatic reduction in time to market. By automating the build, test, and deployment processes, you can push out new features and bug fixes faster than ever before. It’s like strapping a rocket to your development cycle, propelling your product forward at lightning speed.
Improved Code Quality
With CI/CD, every code change goes through a gauntlet of automated tests before it reaches production. This means bugs are caught earlier, when they’re easier and cheaper to fix. It’s like having a team of tireless QA engineers working around the clock to ensure your code is always in top shape.
Reduced Risk
By deploying smaller changes more frequently, you reduce the risk associated with each individual deployment. If something does go wrong, it’s easier to identify and roll back the problematic change. It’s like having a safety net under your development process, giving you the confidence to move fast without breaking things.
Overcoming CI/CD Challenges
Of course, implementing CI/CD isn’t without its challenges. Let’s address some common hurdles and how to overcome them:
Cultural Resistance
One of the biggest challenges in adopting CI/CD is often cultural resistance. Some team members might be hesitant to change established processes or worry about job security. The key to overcoming this is education and communication. Help your team understand the benefits of CI/CD, involve them in the implementation process, and celebrate the wins together. It’s like turning your development team into a group of CI/CD evangelists, spreading the good word throughout your organization.
Technical Debt
If your codebase is riddled with technical debt, implementing CI/CD can be challenging. You might need to invest time in refactoring and improving your test coverage before you can fully benefit from automation. Think of it as spring cleaning for your codebase – it might be a bit painful at first, but the end result is a cleaner, more manageable system.
Tool Selection
With so many CI/CD tools available, choosing the right ones for your needs can be overwhelming. Take the time to evaluate different options based on your specific requirements, team skills, and budget. Remember, the best tool is the one that fits your needs and that your team will actually use. It’s like finding the perfect pair of shoes – they need to fit well and be comfortable for the long haul.
Best Practices for CI/CD Success
To truly harness the superpowers of CI/CD, consider these best practices:
Automate Everything
The more you can automate, the more you’ll benefit from CI/CD. This includes not just your builds and tests, but also your infrastructure provisioning, configuration management, and monitoring. Tools like Terraform, Ansible, and Prometheus can help you achieve this level of automation. It’s like building a self-driving car for your development process – once it’s set up, it can take you where you need to go with minimal intervention.
Embrace Infrastructure as Code
Treat your infrastructure with the same care and version control as your application code. This makes it easier to replicate environments, track changes, and roll back if necessary. Here’s a simple example using Terraform to provision an AWS EC2 instance:
provider "aws" {
region = "us-west-2"
}
resource "aws_instance" "example" {
ami = "ami-0c55b159cbfafe1f0"
instance_type = "t2.micro"
tags = {
Name = "example-instance"
}
}
This code defines an EC2 instance in a declarative way, allowing you to version control your infrastructure alongside your application code. It’s like having a blueprint for your entire system, making it easy to replicate and modify as needed.
Implement Feature Flags
Feature flags allow you to toggle features on and off without deploying new code. This can be incredibly useful for testing new features with a subset of users or quickly disabling problematic features. Here’s a simple example of how you might implement feature flags in Python:
import os
def is_feature_enabled(feature_name):
return os.environ.get(f"FEATURE_{feature_name.upper()}", "false").lower() == "true"
def new_feature():
if is_feature_enabled("new_feature"):
print("New feature is enabled!")
else:
print("New feature is disabled.")
new_feature()
By using environment variables to control feature flags, you can easily toggle features on and off without changing code. It’s like having a control panel for your application, allowing you to fine-tune the user experience in real-time.
Monitor Everything
With CI/CD, changes are happening faster than ever. This makes robust monitoring crucial. Implement comprehensive logging and monitoring to catch issues quickly and gather data for continuous improvement. Tools like ELK stack (Elasticsearch, Logstash, and Kibana) or the Prometheus/Grafana combo can help you achieve this. It’s like having a mission control center for your application, giving you real-time insights into its health and performance.
The Future of CI/CD
As we look to the future, CI/CD continues to evolve. Here are some trends to watch:
AI and Machine Learning in CI/CD
Artificial Intelligence and Machine Learning are starting to make their way into CI/CD pipelines. From predicting build failures to automatically optimizing test suites, AI has the potential to make our CI/CD processes even smarter and more efficient. It’s like giving your CI/CD pipeline a brain of its own, capable of learning and improving over time.
Serverless CI/CD
Serverless architectures are changing the way we think about infrastructure, and CI/CD is no exception. Serverless CI/CD pipelines, which only consume resources when they’re actually running, can lead to significant cost savings and improved scalability. It’s like having a CI/CD pipeline that expands and contracts based on your exact needs at any given moment.
GitOps
GitOps is an emerging practice that uses Git as the single source of truth for declarative infrastructure and applications. By using Git pull requests to manage infrastructure changes, teams can improve collaboration, versioning, and auditability. Here’s a simple example of how you might use GitOps with Kubernetes:
apiVersion: apps/v1
kind: Deployment
metadata:
name: my-app
spec:
replicas: 3
selector:
matchLabels:
app: my-app
template:
metadata:
labels:
app: my-app
spec:
containers:
- name: my-app
image: my-app:latest
ports:
- containerPort: 8080
By storing this Kubernetes deployment configuration in Git, you can use tools like Flux or ArgoCD to automatically sync your cluster state with your Git repository. It’s like having a magical connection between your code repository and your infrastructure, ensuring they’re always in sync.
Conclusion
Continuous Integration and Continuous Deployment are more than just buzzwords – they’re superpowers that can transform your development process. By embracing CI/CD, you’re not just improving your software delivery; you’re giving your entire organization the ability to move faster, adapt quicker, and deliver better products to your users.
Remember, adopting CI/CD is a journey, not a destination. Start small, celebrate your wins, learn from your setbacks, and continuously improve your processes. Before you know it, you’ll be wielding these DevOps superpowers like a pro, delivering amazing software at a pace you never thought possible.
So, are you ready to unleash your DevOps superpowers? The future of software development is here, and it’s continuous. Embrace it, and watch your team soar to new heights of productivity and innovation. The only question left is: what amazing things will you build with your newfound superpowers?
Disclaimer: This blog post is intended for informational purposes only. While we strive for accuracy, technologies and best practices in the field of DevOps and CI/CD are constantly evolving. Always refer to official documentation and consult with experts when implementing CI/CD in your organization. If you notice any inaccuracies in this post, please report them so we can correct them promptly.