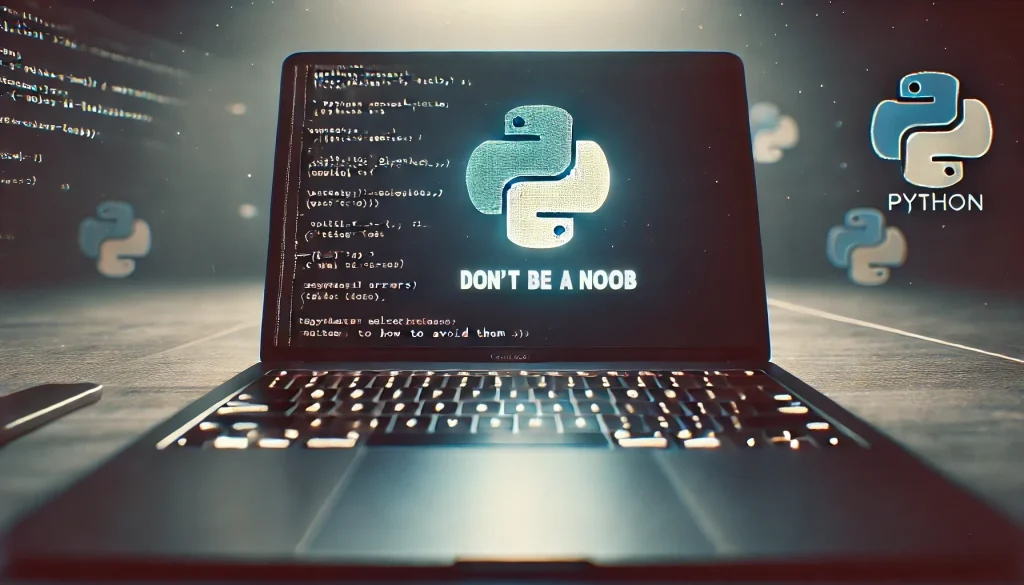
Don’t Be a Noob: Python Mistakes AI Beginners Make (And How to Avoid Them)
Welcome to the exciting world of Python programming and artificial intelligence (AI)! If you’re here, you’re likely just starting your journey, and it’s crucial to get off on the right foot. Python is the go-to language for AI, thanks to its simplicity and powerful libraries. However, even seasoned coders make mistakes, and as a beginner, you might find yourself tripping over some common pitfalls. Don’t worry! This blog will guide you through these common mistakes and teach you how to avoid them.
Why Python for AI?
Before diving into the mistakes, let’s quickly discuss why Python is the preferred language for AI.
Python offers an extensive array of libraries and frameworks such as TensorFlow, Keras, and PyTorch, making it a powerhouse for machine learning and AI development. Its readability and simplicity allow you to focus on solving problems rather than struggling with complex syntax. Plus, the vibrant community and wealth of resources mean you’ll never be short of help when you need it.
Mistake #1: Not Understanding Python Basics
Skipping Fundamental Concepts
Diving straight into AI without a solid understanding of Python basics is a recipe for disaster. Understanding fundamental concepts like data types, loops, conditionals, and functions is essential.
Example:
# Incorrect
number = "5"
result = number + 10
print(result) # This will raise a TypeError
# Correct
number = 5
result = number + 10
print(result) # Outputs: 15
Tip: Spend time learning Python basics. Websites like Codecademy or Python’s official documentation are great places to start.
Misusing Indentation
Python relies on indentation to define the scope of loops, functions, and classes. Misusing indentation can lead to errors that are hard to debug.
Example:
# Incorrect
for i in range(5):
print(i) # This will raise an IndentationError
# Correct
for i in range(5):
print(i) # Outputs: 0 1 2 3 4
Tip: Always use four spaces for indentation. Avoid mixing tabs and spaces in your code.
Mistake #2: Ignoring Data Types and Structures
Using the Wrong Data Types
Choosing the wrong data types can lead to inefficient code and bugs. For instance, using lists when you need a set can lead to performance issues.
Example:
# Incorrect
my_list = [1, 2, 3, 4, 5]
if 3 in my_list:
print("Found") # Outputs: Found
# Correct
my_set = {1, 2, 3, 4, 5}
if 3 in my_set:
print("Found") # Outputs: Found
Tip: Learn about Python’s different data types and their use cases. This will help you write more efficient and readable code.
Neglecting Data Structures
Understanding data structures like lists, dictionaries, sets, and tuples is crucial for effective programming. Misusing these can lead to convoluted and error-prone code.
Example:
# Incorrect
my_list = [(1, "apple"), (2, "banana"), (3, "cherry")]
for item in my_list:
if item[0] == 2:
print(item[1]) # Outputs: banana
# Correct
my_dict = {1: "apple", 2: "banana", 3: "cherry"}
print(my_dict[2]) # Outputs: banana
Tip: Familiarize yourself with Python’s built-in data structures and choose the right one for the task.
Mistake #3: Poor Error Handling
Ignoring Exceptions
Ignoring exceptions can cause your program to crash unexpectedly. Proper error handling ensures your code can gracefully handle unforeseen situations.
Example:
# Incorrect
def divide(a, b):
return a / b
print(divide(10, 0)) # This will raise a ZeroDivisionError
# Correct
def divide(a, b):
try:
return a / b
except ZeroDivisionError:
return "Division by zero is not allowed"
print(divide(10, 0)) # Outputs: Division by zero is not allowed
Tip: Always anticipate potential errors and handle them appropriately using try-except blocks.
Overusing Exceptions
While handling exceptions is crucial, overusing them can make your code hard to read and maintain. Use exceptions for exceptional situations, not for regular control flow.
Example:
# Incorrect
try:
result = int("not a number")
except ValueError:
result = 0
# Correct
if "not a number".isdigit():
result = int("not a number")
else:
result = 0
Tip: Validate inputs and use exceptions sparingly to keep your code clean and efficient.
Mistake #4: Inefficient Use of Libraries
Rewriting Existing Functions
Python has a rich ecosystem of libraries. Rewriting existing functions instead of leveraging these libraries can waste time and lead to less reliable code.
Example:
# Incorrect
def calculate_mean(numbers):
total = sum(numbers)
count = len(numbers)
return total / count
# Correct
import statistics
numbers = [1, 2, 3, 4, 5]
mean = statistics.mean(numbers)
print(mean) # Outputs: 3
Tip: Familiarize yourself with Python’s standard library and popular third-party libraries to avoid reinventing the wheel.
Not Using List Comprehensions
List comprehensions are a powerful feature in Python that can make your code more concise and readable. Not using them can lead to unnecessarily verbose code.
Example:
# Incorrect
squares = []
for x in range(10):
squares.append(x**2)
# Correct
squares = [x**2 for x in range(10)]
print(squares) # Outputs: [0, 1, 4, 9, 16, 25, 36, 49, 64, 81]
Tip: Practice using list comprehensions to write more Pythonic code.
Mistake #5: Ineffective Code Organization
Writing Monolithic Code
Writing all your code in a single script can make it hard to manage and debug. Organize your code into functions and modules to improve readability and maintainability.
Example:
# Incorrect
def main():
data = [1, 2, 3, 4, 5]
mean = sum(data) / len(data)
print(f"The mean is {mean}")
main()
# Correct
def calculate_mean(data):
return sum(data) / len(data)
def main():
data = [1, 2, 3, 4, 5]
mean = calculate_mean(data)
print(f"The mean is {mean}")
main()
Tip: Break your code into smaller, reusable functions and organize related functions into modules.
Ignoring Code Comments and Documentation
Skipping comments and documentation can make your code hard to understand for others and for yourself in the future. Good comments and documentation help in maintaining and scaling your projects.
Example:
# Incorrect
def calc(data):
return sum(data) / len(data)
# Correct
def calculate_mean(data):
"""
Calculate the mean of a list of numbers.
Parameters:
data (list): A list of numerical values.
Returns:
float: The mean of the numbers in the list.
"""
return sum(data) / len(data)
Tip: Write docstrings for your functions and use comments to explain complex logic.
Mistake #6: Not Testing Your Code
Skipping Unit Tests
Unit tests are crucial for verifying that your code works as expected. Skipping tests can lead to bugs and regressions in your codebase.
Example:
# Correct
import unittest
def add(a, b):
return a + b
class TestMathFunctions(unittest.TestCase):
def test_add(self):
self.assertEqual(add(2, 3), 5)
self.assertEqual(add(-1, 1), 0)
if __name__ == "__main__":
unittest.main()
Tip: Use testing frameworks like unittest
or pytest
to write and run tests for your code.
Not Using Test-Driven Development (TDD)
Test-Driven Development (TDD) involves writing tests before you write the actual code. This approach helps in designing better functions and catching bugs early.
Example:
# Step 1: Write a test
import unittest
class TestMathFunctions(unittest.TestCase):
def test_add(self):
self.assertEqual(add(2, 3), 5)
# Step 2: Write the function to pass the test
def add(a, b):
return a + b
if __name__ == "__main__":
unittest.main()
Tip: Practice TDD to improve your coding discipline and produce more reliable code.
Mistake #7: Neglecting Version Control
Not Using Git
Version control systems like Git are essential for tracking changes in your code and collaborating with others. Not using Git can lead to lost code and difficult collaborations.
Example:
# Initialize a new Git repository
git init
# Add files to the repository
git add .
# Commit the changes
git commit -m "Initial commit"
Tip: Learn the basics of Git and use it to manage
your code from the start. Platforms like GitHub and GitLab are also great for hosting and collaborating on projects.
Making Infrequent Commits
Making large, infrequent commits makes it hard to track changes and identify where things went wrong. Small, frequent commits with meaningful messages are much better.
Example:
# Incorrect
git commit -m "Did a bunch of stuff"
# Correct
git commit -m "Added function to calculate mean"
git commit -m "Fixed bug in divide function"
Tip: Commit often and write descriptive commit messages to make your commit history informative and useful.
Mistake #8: Overlooking Optimization
Ignoring Code Performance
Writing correct code is essential, but writing efficient code is equally important. Ignoring performance can lead to slow and unresponsive programs.
Example:
# Incorrect
def find_duplicates(numbers):
duplicates = []
for i in range(len(numbers)):
for j in range(i + 1, len(numbers)):
if numbers[i] == numbers[j]:
duplicates.append(numbers[i])
return duplicates
# Correct
def find_duplicates(numbers):
seen = set()
duplicates = set()
for number in numbers:
if number in seen:
duplicates.add(number)
else:
seen.add(number)
return list(duplicates)
Tip: Use appropriate data structures and algorithms to optimize your code’s performance.
Neglecting Memory Usage
Efficient memory usage is crucial, especially when working with large datasets in AI. Neglecting memory can cause your programs to crash or run slowly.
Example:
# Incorrect
data = [i for i in range(10000000)]
# Correct
import numpy as np
data = np.arange(10000000)
Tip: Use libraries like NumPy for handling large datasets efficiently.
Mistake #9: Failing to Keep Up with Updates
Using Outdated Libraries
AI and Python libraries are constantly evolving. Using outdated libraries can lead to compatibility issues and missing out on performance improvements and new features.
Example:
# Incorrect
pip install tensorflow==1.5.0
# Correct
pip install tensorflow==latest
Tip: Regularly update your libraries and read the release notes to stay informed about new features and changes.
Ignoring Python’s New Features
Python frequently releases new versions with improvements and new features. Ignoring these updates means missing out on potential enhancements.
Example:
# Incorrect (Python 2)
print "Hello, World!"
# Correct (Python 3)
print("Hello, World!")
Tip: Keep your Python version up to date and explore the new features it offers.
Mistake #10: Not Engaging with the Community
Avoiding Community Interaction
The Python and AI communities are rich resources for learning and growth. Not engaging with these communities means missing out on valuable knowledge and support.
Example:
# Join forums
# Participate in coding challenges
# Attend meetups and conferences
Tip: Engage with the community through forums like Stack Overflow, GitHub, Reddit, and local meetups.
Not Contributing to Open Source
Contributing to open-source projects is a great way to learn and give back to the community. It helps you improve your coding skills and gain real-world experience.
Example:
# Find a project on GitHub
# Fork the repository
# Make your changes
# Submit a pull request
Tip: Start by contributing to documentation or fixing small bugs in open-source projects to get the hang of it.
Conclusion
Embarking on your Python and AI journey can be daunting, but by being aware of these common mistakes and how to avoid them, you’ll be well on your way to becoming a proficient coder. Remember, practice makes perfect, and don’t be afraid to make mistakes—they’re a crucial part of the learning process. Keep coding, stay curious, and always strive to improve.
Disclaimer: The information provided in this blog is for educational purposes only. The code examples are simplified to illustrate common mistakes and how to avoid them. Please report any inaccuracies so we can correct them promptly.
Thank you for reading! If you found this blog helpful, share it with your fellow aspiring AI developers and leave a comment below with your thoughts or questions. Happy coding!