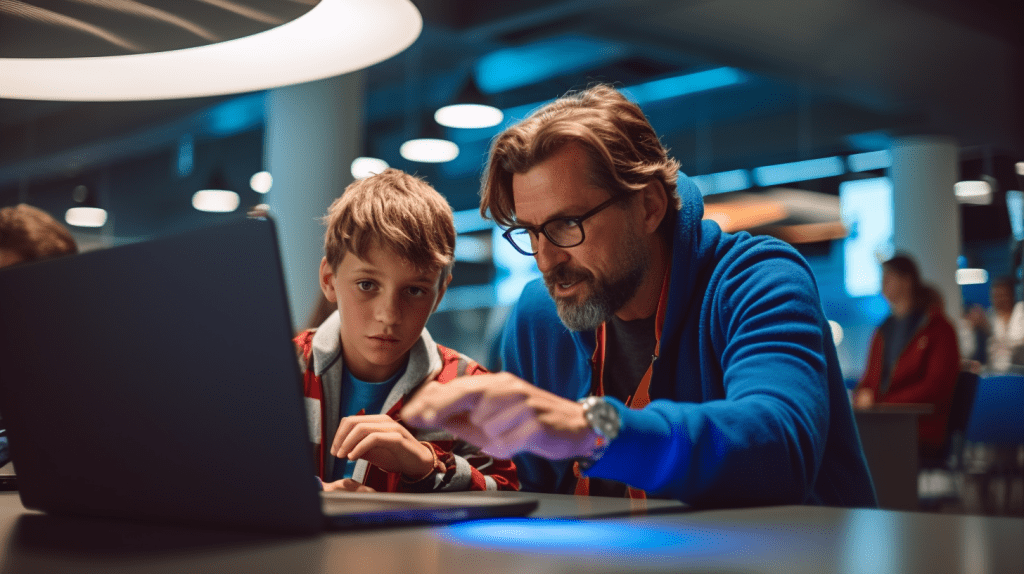
Encapsulation in Java: Enhancing Modularity and Robustness with Best Practices
Encapsulation is a fundamental principle of object-oriented programming that promotes the bundling of data and methods operating on that data within a single unit, or class. By implementing encapsulation in Java development, developers can create more maintainable, modular, and robust software solutions that are less prone to errors and easier to modify. In this blog post, we’ll explore the concept of encapsulation, discuss its benefits, and provide practical tips and examples to help you apply this powerful design principle to your Java projects.
Understanding Encapsulation
Encapsulation refers to the practice of restricting access to certain components of an object and exposing only what is necessary, thereby hiding the internal details of the object. This approach leads to better organization and separation of concerns, making the code more maintainable and less prone to bugs. In Java, encapsulation is achieved by using access modifiers, such as private, protected, and public, and by providing public methods (getters and setters) to access and modify the private data members of a class.
Benefits of Encapsulation
Implementing encapsulation in Java development offers several benefits:
- Modularity: Encapsulation promotes the creation of modular, self-contained components that can be easily combined, reused, and extended.
- Maintainability: By hiding the internal details of a class and exposing only a well-defined interface, encapsulation makes the code more maintainable, as changes to the internal implementation do not affect the external users of the class.
- Robustness: Encapsulation helps reduce the risk of errors and unintended side effects by preventing direct access to the internal state of an object, ensuring that the object’s state can only be changed through its public methods.
- Ease of use: Encapsulating the complexity of a class behind a simple, well-defined interface makes it easier for other developers to use and understand the class without needing to know its internal details.
Best Practices for Implementing Encapsulation in Java Development
To effectively apply encapsulation in your Java projects, consider the following best practices:
- Use access modifiers: Leverage access modifiers, such as private, protected, and public, to control the visibility and accessibility of the class members.
- Provide getters and setters: Offer public getter and setter methods to access and modify the private data members of a class while maintaining encapsulation.
- Minimize the use of public fields: Avoid using public fields, as they can expose the internal state of an object and compromise encapsulation.
- Favor immutability: Whenever possible, create immutable classes, which have state that cannot be changed after instantiation. Immutable classes are inherently more robust and less prone to errors.
Practical Examples of Encapsulation in Java
To illustrate the concept of encapsulation in Java development, let’s examine a practical example:
public class BankAccount {
private double balance;
public BankAccount(double balance) {
this.balance = balance;
}
public double getBalance() {
return balance;
}
public void deposit(double amount) {
if (amount > 0) {
balance += amount;
}
}
public void withdraw(double amount) {
if (amount > 0 && balance >= amount) {
balance -= amount;
}
}
}
In this example, the BankAccount
class encapsulates the balance
field by making it private and providing public getter
and setter methods to access and modify the balance. The deposit
and withdraw
methods ensure that only valid operations are performed on the balance, maintaining the object’s internal state’s integrity.
Balancing Encapsulation with Other Design Concerns
While encapsulation is a vital design principle, it’s essential to balance it with other software design considerations, such as performance, readability, and usability. In some cases, strict encapsulation may not be the most appropriate solution for a particular problem. By considering the specific needs and requirements of your project, you can make informed decisions about when to prioritize encapsulation and when to focus on other design aspects.
Encapsulation is a foundational design principle that can help Java developers create more maintainable, modular, and robust software solutions by promoting the organization and separation of concerns. By embracing encapsulation and applying best practices, you can build software that is less prone to errors, easier to modify, and more user-friendly.
To effectively implement encapsulation in your Java projects, remember to:
- Use access modifiers to control the visibility and accessibility of class members.
- Provide getters and setters to access and modify private data members.
- Minimize the use of public fields to maintain encapsulation.
- Favor immutability for increased robustness and reduced risk of errors.
By mastering encapsulation and incorporating it into your Java development practices, you can build high-quality, well-structured, and maintainable software solutions that stand the test of time.