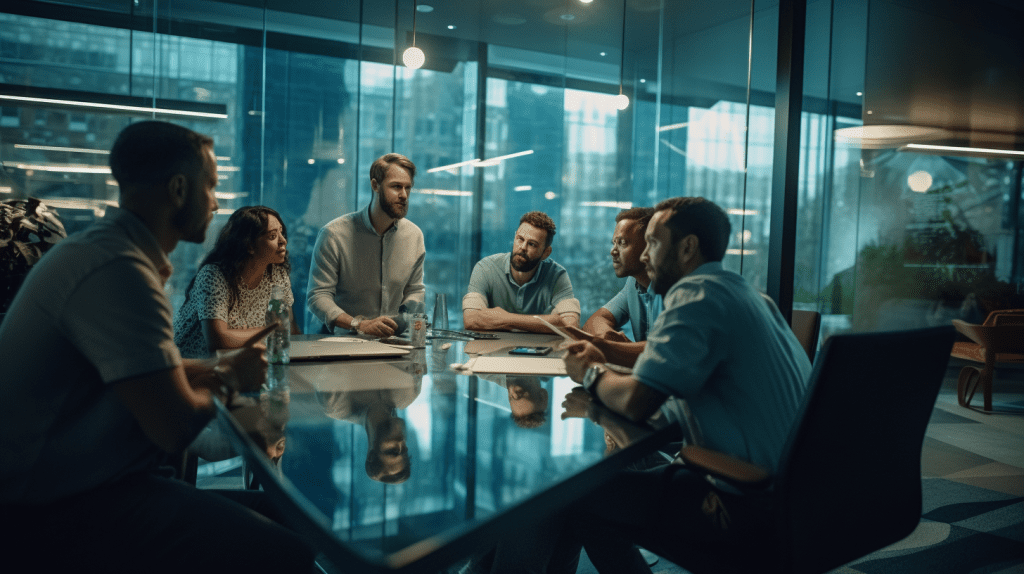
Essential Software Development Principles for Java Developers
As a Java developer, understanding and applying essential software development principles is crucial to creating high-quality software. These principles, including SOLID and DRY, provide guidelines for crafting efficient, maintainable, and scalable solutions, setting a strong foundation for successful software development projects. In this blog post, we’ll provide a high-level introduction to these principles and their importance in Java development.
SOLID Principles
SOLID principles, introduced by Robert C. Martin, are a set of guidelines for object-oriented programming (OOP) that promote code readability, modularity, and maintainability. SOLID consists of five principles:
- Single Responsibility Principle (SRP): A class should have only one reason to change, ensuring it is responsible for a single piece of functionality.
- Open-Closed Principle (OCP): Classes should be open for extension but closed for modification, allowing developers to extend existing functionality without altering the original class.
- Liskov Substitution Principle (LSP): Objects of a superclass should be replaceable by objects of a subclass without affecting the correctness of the program.
- Interface Segregation Principle (ISP): A class should not be forced to implement interfaces it does not use, emphasizing the importance of small, focused interfaces.
- Dependency Inversion Principle (DIP): High-level modules should depend on abstractions, not on concrete implementations, promoting a flexible and extensible codebase.
By adhering to SOLID principles, Java developers can create robust software designs that are easy to understand, refactor, and maintain.
DRY Principle
The DRY (Don’t Repeat Yourself) principle, introduced by Andy Hunt and Dave Thomas, asserts that every piece of code should have a single, unambiguous representation within a system. By eliminating code duplication, developers can create maintainable, efficient, and reusable code that is less prone to bugs and inconsistencies. In Java development, the DRY principle can be applied through techniques such as methods, inheritance, and design patterns.
KISS Principle
The KISS (Keep It Simple, Stupid) principle is a software design philosophy that encourages developers to create simple and straightforward solutions. By favoring simplicity over complexity, Java developers can write code that is easy to understand, maintain, and modify. This principle often goes hand-in-hand with the YAGNI principle, as both advocate for focusing on the most essential aspects of a software solution.
YAGNI Principle
YAGNI (You Aren’t Gonna Need It) is a software development principle that advises developers to avoid adding unnecessary features or complexity to their applications. By focusing on the current requirements and resisting the temptation to implement “future-proof” solutions, Java developers can create software that is easier to understand, maintain, and extend. This principle aligns with agile methodologies and emphasizes the importance of iterative development.
Code Reusability
Code reusability is a key software development principle that promotes the use of existing code components in new contexts. By creating modular and reusable code, Java developers can reduce development time, minimize duplication, and improve maintainability. In Java, code reusability can be achieved through methods, inheritance, polymorphism, and design patterns.
Separation of Concerns (SoC)
Separation of Concerns is a design principle that encourages developers to organize their code into distinct sections, each responsible for a specific aspect of the application. By dividing code into modular, focused components, Java developers can create maintainable, scalable, and testable software. SoC can be implemented in Java through various techniques, including package organization, encapsulation, and layered architecture.
Composition over Inheritance
Composition over Inheritance is a software design principle that promotes the use of object composition instead of class inheritance to achieve code reuse and flexibility. By favoring composition, Java developers can create more modular, flexible, and maintainable code that is less prone to the issues associated with deep inheritance hierarchies. This principle is particularly useful when working with complex systems or when inheritance is not a suitable option.
Encapsulation
Encapsulation is a fundamental OOP concept that involves bundling data and methods operating on that data within a single unit, such as a class. By using encapsulation, Java developers can hide the internal state of an object and expose only what is necessary through a well-defined interface. Encapsulation promotes code maintainability, modularity, and data integrity.
Law of Demeter (LoD)
The Law of Demeter, also known as the Principle of Least Knowledge, is a software design guideline that encourages objects to communicate with their immediate neighbors and avoid interacting with distant objects directly. By adhering to the LoD, Java developers can create loosely coupled, modular, and maintainable code. The LoD can be implemented in Java through techniques such as object composition, encapsulation, and dependency injection.
Cohesion and Coupling
Cohesion and coupling are essential software design principles that determine the quality of a software system. High cohesion means that a module or class is focused on a single responsibility, while low coupling refers to the minimal dependency between modules or classes. By striving for high cohesion and low coupling, Java developers can create modular, maintainable, and flexible software systems.
Understanding and applying essential software development principles, such as SOLID, DRY, KISS, and YAGNI, is vital for crafting high-quality software in Java. By adhering to these principles, Java developers can create efficient, maintainable, and scalable solutions that stand the test of time. As you continue your journey as a Java developer, make it a priority to master these principles and incorporate them into your software development practices, ensuring the success of your software projects.