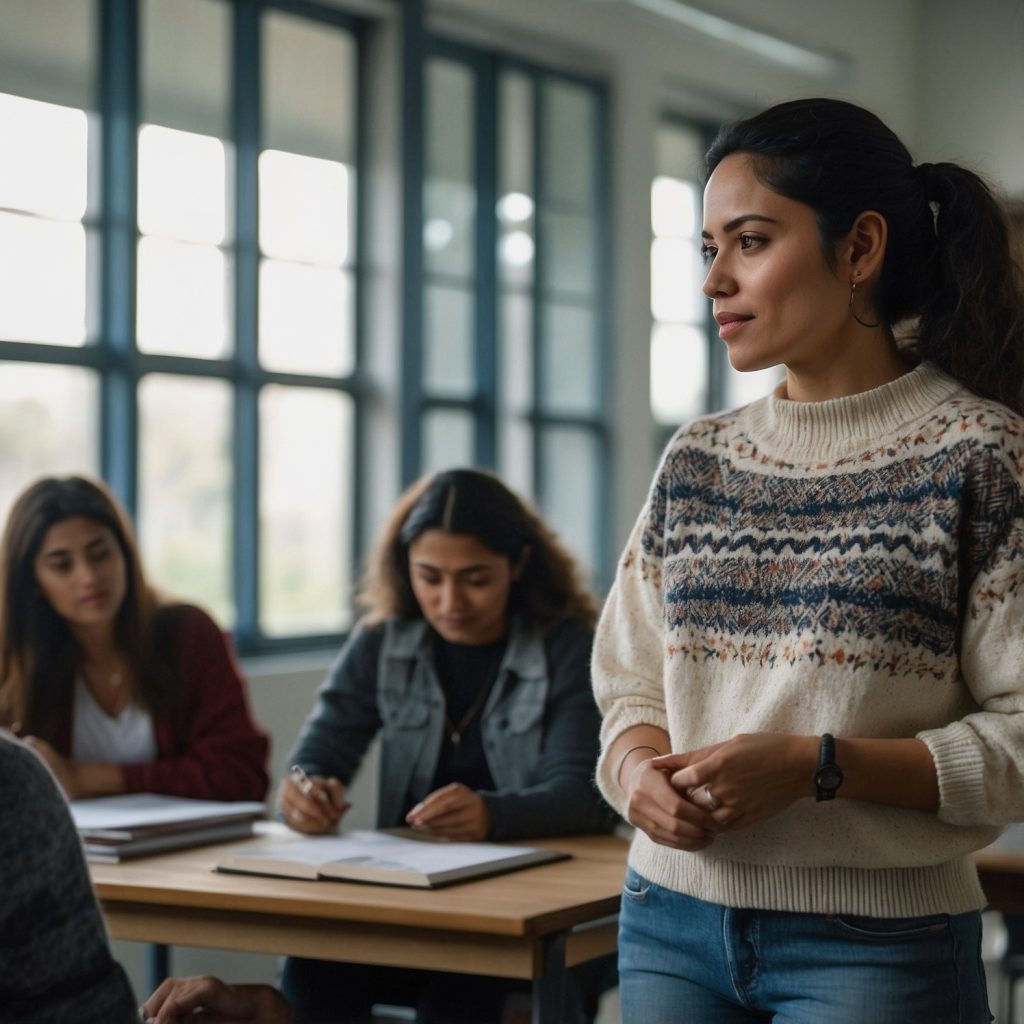
Exploring Different Programming Paradigms
Programming paradigms are essential frameworks that guide how code is written and structured. Each paradigm offers unique ways to think about and solve problems, which can significantly affect the design and functionality of software. In this blog, we’ll dive into the world of programming paradigms, exploring their concepts, benefits, and use cases. We’ll cover four major paradigms: procedural, object-oriented, functional, and declarative programming. By the end, you’ll have a better understanding of how these paradigms shape the programming landscape.
Procedural Programming
What is Procedural Programming?
Procedural programming is a paradigm derived from structured programming, based on the concept of procedure calls. Procedures, also known as routines, subroutines, or functions, are fundamental building blocks in this approach.
Procedural programming organizes code into procedures or functions that operate on data. It follows a linear top-down approach, making it easy to follow and understand. This paradigm is widely used due to its simplicity and ease of implementation.
Advantages:
- Simplicity: Easy to learn and use, especially for beginners.
- Reusability: Functions can be reused across the program, reducing redundancy.
- Modularity: Code can be divided into smaller, manageable functions.
Example Code:
Here’s a simple example of procedural programming in C:
#include <stdio.h>
void greetUser() {
printf("Hello, User!\n");
}
int addNumbers(int a, int b) {
return a + b;
}
int main() {
greetUser();
int sum = addNumbers(5, 10);
printf("Sum: %d\n", sum);
return 0;
}
In this example, the program consists of functions that perform specific tasks, such as greeting the user and adding numbers.
Object-Oriented Programming
What is Object-Oriented Programming (OOP)?
Object-oriented programming is a paradigm that uses “objects” to model real-world entities. Objects encapsulate data and behaviors, promoting code reusability and modularity. OOP is centered around four main concepts: encapsulation, abstraction, inheritance, and polymorphism.
Advantages:
- Encapsulation: Protects data by restricting direct access and only exposing necessary functionalities.
- Abstraction: Simplifies complex systems by modeling them as high-level objects.
- Inheritance: Allows new classes to inherit properties and methods from existing classes, promoting code reuse.
- Polymorphism: Enables objects to be treated as instances of their parent class, enhancing flexibility and integration.
Example Code:
Here’s an example of object-oriented programming in Python:
class Animal:
def __init__(self, name):
self.name = name
def speak(self):
raise NotImplementedError("Subclass must implement abstract method")
class Dog(Animal):
def speak(self):
return f"{self.name} says Woof!"
class Cat(Animal):
def speak(self):
return f"{self.name} says Meow!"
dog = Dog("Buddy")
cat = Cat("Whiskers")
print(dog.speak())
print(cat.speak())
In this example, Dog
and Cat
classes inherit from the Animal
class and implement the speak
method differently.
Functional Programming
What is Functional Programming?
Functional programming is a paradigm that treats computation as the evaluation of mathematical functions. It avoids changing-state and mutable data, emphasizing the use of pure functions and higher-order functions.
Advantages:
- Immutability: Promotes the use of immutable data structures, leading to more predictable and bug-free code.
- First-Class Functions: Treats functions as first-class citizens, enabling the creation of higher-order functions.
- Concurrency: Naturally fits concurrent execution due to the absence of side effects.
Example Code:
Here’s an example of functional programming in JavaScript:
const numbers = [1, 2, 3, 4, 5];
// Pure function to double a number
const double = (n) => n * 2;
// Higher-order function to map over an array
const map = (fn, arr) => arr.map(fn);
const doubledNumbers = map(double, numbers);
console.log(doubledNumbers); // [2, 4, 6, 8, 10]
In this example, double
is a pure function that doesn’t alter any state, and map
is a higher-order function that applies double
to each element in the array.
Declarative Programming
What is Declarative Programming?
Declarative programming is a paradigm that expresses the logic of computation without describing its control flow. It focuses on what the program should accomplish rather than how it should be accomplished. Common declarative programming languages include SQL and HTML.
Advantages:
- Readability: High-level code that’s easy to read and understand.
- Maintainability: Simplifies complex logic, making the code easier to maintain.
- Less Error-Prone: Reduces bugs by abstracting the control flow.
Example Code:
Here’s an example of declarative programming using SQL:
-- Select all employees from the employees table where the department is 'Sales'
SELECT * FROM employees
WHERE department = 'Sales';
In this example, the SQL query specifies what data to retrieve without describing how to fetch it.
Comparing Programming Paradigms
Understanding the differences between these paradigms can help you choose the right one for your project:
Procedural vs. Object-Oriented:
- Procedural programming is straightforward and suitable for simple tasks.
- Object-oriented programming is more powerful and suitable for complex, large-scale applications.
Functional vs. Declarative:
- Functional programming focuses on pure functions and immutability, making it ideal for tasks requiring high reliability and concurrency.
- Declarative programming is best for tasks where defining the desired outcome is more straightforward than describing the process, such as database queries and user interface design.
Practical Use Cases
Procedural Programming:
- Simple scripts and automation tasks
- Legacy systems
- Applications where performance is a critical factor
Object-Oriented Programming:
- Enterprise-level applications
- Software with complex data models
- Systems requiring frequent updates and maintenance
Functional Programming:
- Data processing tasks
- Applications requiring concurrent operations
- Systems where robustness and reliability are crucial
Declarative Programming:
- Database queries
- Web development (HTML, CSS)
- Configuration management
Conclusion
Exploring different programming paradigms allows you to broaden your problem-solving toolkit and choose the best approach for your projects. Whether you prefer the straightforwardness of procedural programming, the modularity of object-oriented programming, the purity of functional programming, or the simplicity of declarative programming, each paradigm offers unique strengths that can enhance your software development process.
By understanding and applying these paradigms, you can write more efficient, maintainable, and robust code. Happy coding!