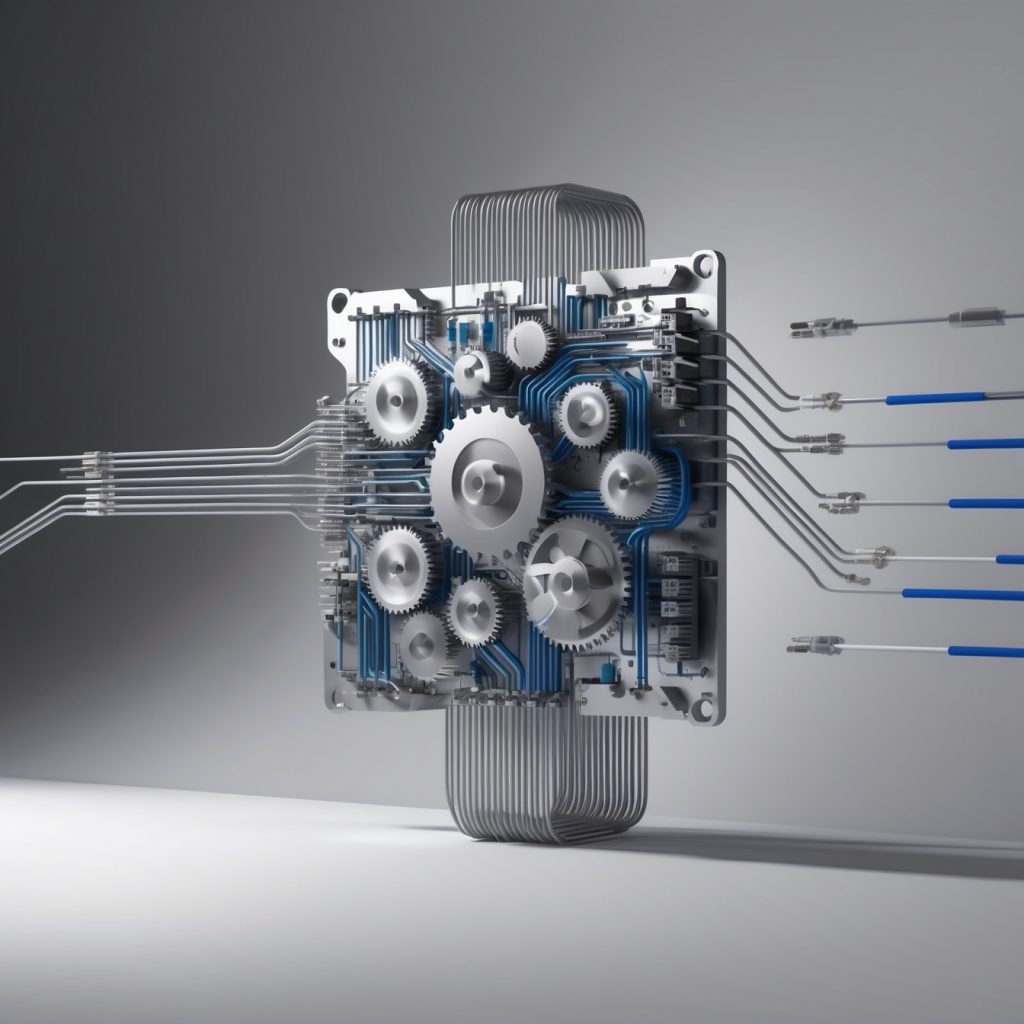
Exploring Java’s Functional Interfaces – Lambda Expressions and Method References
Java 8 introduced a revolutionary concept that transformed the way developers write code: functional interfaces, lambda expressions, and method references. These features brought functional programming paradigms to Java, enabling more concise, readable, and maintainable code. The integration of these concepts has not only modernized Java’s approach to handling functions as first-class citizens but also significantly improved developer productivity. In this comprehensive guide, we’ll dive deep into functional interfaces, understand how lambda expressions work, and explore the elegant syntax of method references. By mastering these concepts, you’ll be equipped to write more efficient and expressive Java code that aligns with modern programming practices.
Understanding Functional Interfaces
What is a Functional Interface?
A functional interface is a special type of interface in Java that contains exactly one abstract method. These interfaces serve as the foundation for lambda expressions and method references in Java. The @FunctionalInterface annotation, while optional, is commonly used to indicate that an interface is intended to be functional. This annotation helps catch potential errors during compilation if someone accidentally adds another abstract method to the interface. Some well-known functional interfaces in Java include Runnable, Callable, and Comparator.
Common Built-in Functional Interfaces
Java provides several built-in functional interfaces in the java.util.function package. Here are some of the most commonly used ones:
// Function<T,R> - Takes one argument and produces a result
Function<String, Integer> strLength = str -> str.length();
// Predicate<T> - Takes one argument and returns a boolean
Predicate<Integer> isEven = num -> num % 2 == 0;
// Consumer<T> - Takes one argument and returns no result
Consumer<String> printer = str -> System.out.println(str);
// Supplier<T> - Takes no argument and returns a result
Supplier<Double> randomNumber = () -> Math.random();
// BiFunction<T,U,R> - Takes two arguments and produces a result
BiFunction<Integer, Integer, Integer> add = (a, b) -> a + b;
Lambda Expressions Deep Dive
Syntax and Structure
Lambda expressions provide a clear and concise way to represent functional interfaces using an arrow-based notation. The basic syntax consists of parameters, the arrow token (->), and a body. The parameters can be explicitly typed or inferred by the compiler, and the body can be a single expression or a block of statements.
// Different forms of lambda expressions
(String s) -> s.length() // Explicit parameter type
s -> s.length() // Inferred parameter type
(x, y) -> x + y // Multiple parameters
() -> 42 // No parameters
(String s) -> { // Block with multiple statements
System.out.println(s);
return s.length();
}
Type Inference and Context
Java’s type inference system is particularly powerful when working with lambda expressions. The compiler can often determine the types of lambda parameters based on the context in which the lambda is used. This feature, known as target typing, makes code more concise while maintaining type safety.
public class TypeInferenceExample {
public static void main(String[] args) {
// Type inference in different contexts
List<String> names = Arrays.asList("Alice", "Bob", "Charlie");
// Compiler infers String parameter
names.forEach(name -> System.out.println(name));
// Compiler infers String parameter and boolean return type
Predicate<String> startsWithA = name -> name.startsWith("A");
// Complex type inference with generics
Map<String, Integer> nameLength = names.stream()
.collect(Collectors.toMap(
name -> name, // Key function
name -> name.length() // Value function
));
}
}
Method References
Understanding Method References
Method references provide an even more concise way to write lambda expressions that simply call an existing method. They use the double colon (::) operator and can reference static methods, instance methods, or constructors.
public class MethodReferenceExample {
public static void main(String[] args) {
List<String> names = Arrays.asList("Alice", "Bob", "Charlie");
// Static method reference
names.forEach(System.out::println);
// Instance method reference of a particular object
String prefix = "User: ";
names.forEach(prefix::concat);
// Instance method reference of an arbitrary object
List<String> sortedNames = names.stream()
.sorted(String::compareToIgnoreCase)
.collect(Collectors.toList());
// Constructor reference
List<StringBuilder> builders = names.stream()
.map(StringBuilder::new)
.collect(Collectors.toList());
}
}
Types of Method References
There are four types of method references in Java:
- Static method reference:
ClassName::staticMethod
- Instance method reference of a particular object:
object::instanceMethod
- Instance method reference of an arbitrary object of a particular type:
ClassName::instanceMethod
- Constructor reference:
ClassName::new
Practical Applications
Stream Operations
One of the most common use cases for functional interfaces and lambda expressions is working with Java streams. Streams provide a powerful way to process collections of data in a functional style.
public class StreamExample {
public static void main(String[] args) {
List<Integer> numbers = Arrays.asList(1, 2, 3, 4, 5, 6, 7, 8, 9, 10);
// Using multiple functional operations
List<Integer> processedNumbers = numbers.stream()
.filter(n -> n % 2 == 0) // Predicate
.map(n -> n * n) // Function
.sorted((a, b) -> b - a) // Comparator
.collect(Collectors.toList());
// Using method references
numbers.stream()
.filter(StreamExample::isEven)
.map(Math::sqrt)
.forEach(System.out::println);
}
private static boolean isEven(int n) {
return n % 2 == 0;
}
}
Custom Functional Interfaces
Creating custom functional interfaces allows you to define specific behavior for your application’s needs.
@FunctionalInterface
interface DataTransformer<T, R> {
R transform(T data);
// Default methods are allowed in functional interfaces
default R transformOrDefault(T data, R defaultValue) {
try {
return transform(data);
} catch (Exception e) {
return defaultValue;
}
}
}
public class CustomFunctionalInterfaceExample {
public static void main(String[] args) {
// Using custom functional interface
DataTransformer<String, Integer> wordCounter =
str -> str.split("\\s+").length;
String text = "Hello functional programming world";
int wordCount = wordCounter.transform(text);
// Using default method
String invalidText = null;
int defaultCount = wordCounter.transformOrDefault(invalidText, 0);
}
}
Best Practices and Guidelines
Clean Code Principles
When working with functional interfaces and lambda expressions, following these best practices ensures maintainable and readable code:
- Keep lambda expressions short and focused
- Extract complex lambda expressions into named methods
- Use method references when possible
- Choose appropriate functional interfaces based on your needs
- Consider composition for complex operations
public class BestPracticesExample {
public static void main(String[] args) {
List<String> names = Arrays.asList("Alice", "Bob", "Charlie");
// Bad: Complex lambda
names.stream()
.filter(name -> name.length() > 3 && Character.isUpperCase(name.charAt(0)))
.forEach(name -> System.out.println("Name: " + name));
// Good: Extracted method and method reference
names.stream()
.filter(BestPracticesExample::isValidName)
.forEach(BestPracticesExample::printName);
}
private static boolean isValidName(String name) {
return name.length() > 3 && Character.isUpperCase(name.charAt(0));
}
private static void printName(String name) {
System.out.println("Name: " + name);
}
}
Error Handling
Proper error handling in functional programming requires careful consideration. Here are some approaches:
public class ErrorHandlingExample {
public static void main(String[] args) {
List<String> inputs = Arrays.asList("123", "456", "abc", "789");
// Using Optional for safe operations
inputs.stream()
.map(ErrorHandlingExample::parseIntSafely)
.filter(Optional::isPresent)
.map(Optional::get)
.forEach(System.out::println);
// Using custom wrapper for error handling
inputs.stream()
.map(input -> {
try {
return Integer.parseInt(input);
} catch (NumberFormatException e) {
System.err.println("Failed to parse: " + input);
return null;
}
})
.filter(Objects::nonNull)
.forEach(System.out::println);
}
private static Optional<Integer> parseIntSafely(String input) {
try {
return Optional.of(Integer.parseInt(input));
} catch (NumberFormatException e) {
return Optional.empty();
}
}
}
Performance Considerations
Memory and CPU Usage
Understanding the performance implications of functional programming constructs is crucial for writing efficient code:
- Lambda expressions have minimal overhead compared to anonymous classes
- Method references can be more efficient than lambda expressions
- Parallel streams should be used judiciously based on workload and data size
- Consider using primitive specializations for better performance
public class PerformanceExample {
public static void main(String[] args) {
// Using primitive specializations for better performance
IntStream.range(0, 1000000)
.parallel()
.filter(n -> n % 2 == 0)
.mapToDouble(Math::sqrt)
.average()
.ifPresent(System.out::println);
// Using specialized functional interfaces
IntPredicate evenNumbers = n -> n % 2 == 0;
IntFunction<Double> squareRoot = n -> Math.sqrt(n);
}
}
Conclusion
Functional interfaces, lambda expressions, and method references have revolutionized Java programming by introducing functional programming concepts to the language. These features enable developers to write more concise, readable, and maintainable code while maintaining type safety and performance. By understanding and properly implementing these concepts, you can create more elegant solutions to complex programming challenges and take full advantage of Java’s modern features.
Disclaimer: This blog post is intended for educational purposes and reflects the current state of Java programming practices as of the writing date. While we strive for accuracy, Java features and best practices may evolve. Please consult the official Java documentation for the most up-to-date information. If you notice any inaccuracies in this post, please report them to our editorial team for prompt correction.