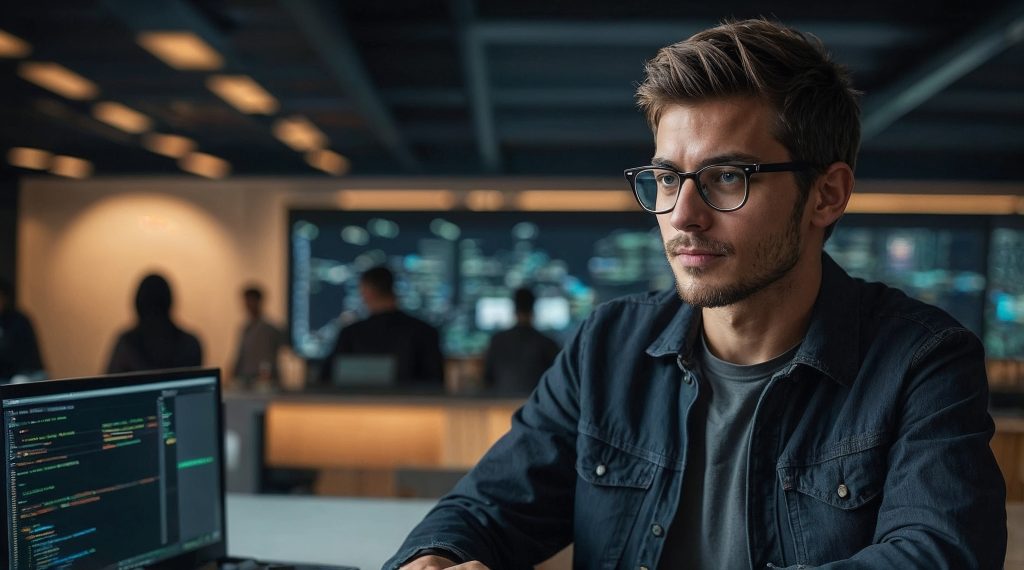
Exploring the World of Java Microservices
Have you ever wondered how tech giants like Netflix, Amazon, and Google manage to handle millions of users simultaneously without breaking a sweat? The secret sauce behind their success lies in a revolutionary architectural approach called microservices. In this blog post, we’re going to embark on an exciting journey through the world of Java microservices, uncovering the what, why, and how of this game-changing technology.
Microservices architecture has taken the software development world by storm, and for good reason. It’s not just a buzzword; it’s a paradigm shift that’s reshaping how we build and scale applications. But what exactly are microservices, and why should you care? Well, buckle up, because we’re about to dive deep into this fascinating topic, exploring everything from the basic concepts to advanced implementation strategies – all with a focus on Java, one of the most popular programming languages for building microservices.
What Are Microservices?
Let’s start with the basics. Microservices are a software architectural style that structures an application as a collection of loosely coupled, independently deployable services. Each service is responsible for a specific business capability and communicates with other services through well-defined APIs. Think of it as breaking down a monolithic application into smaller, more manageable pieces – like Lego blocks that can be assembled and reassembled in various ways to create a robust, scalable system.
But why the buzz around microservices? Well, imagine you’re building a house. In the traditional monolithic approach, you’d construct the entire house as one big, interconnected structure. If you wanted to change the kitchen layout, you might need to modify the living room and bedroom too. Microservices, on the other hand, are like building each room as a separate, self-contained unit. Want to upgrade the kitchen? No problem – just swap it out without touching the rest of the house. This modularity is what makes microservices so powerful and flexible.
The Benefits of Microservices Architecture
Now that we’ve got a handle on what microservices are, let’s explore why they’ve become the darling of modern software architecture. The benefits are numerous and compelling:
Scalability: Each microservice can be scaled independently based on its specific needs. This granular scalability allows for efficient resource utilization and better performance under varying loads.
Flexibility: Microservices enable teams to use different technologies and programming languages for different services, choosing the best tool for each job.
Resilience: If one service fails, it doesn’t bring down the entire application. This isolation improves the overall system’s fault tolerance.
Easier Maintenance: Smaller codebases are easier to understand, modify, and maintain. This leads to faster development cycles and reduced time-to-market for new features.
Improved Team Productivity: Different teams can work on different services simultaneously, leading to faster development and deployment cycles.
These benefits make microservices an attractive option for businesses looking to build agile, scalable, and resilient applications. But as with any architectural choice, it’s important to weigh the pros against the potential challenges, which we’ll discuss later in this post.
Java and Microservices: A Match Made in Heaven
Now, you might be wondering, “Why Java for microservices?” Well, Java has been a stalwart in enterprise software development for decades, and it’s perfectly positioned to capitalize on the microservices revolution. Here’s why Java and microservices are a match made in heaven:
Mature Ecosystem: Java boasts a rich ecosystem of libraries, frameworks, and tools that make building microservices a breeze. From Spring Boot to Quarkus, Java developers have a wealth of options at their fingertips.
Performance: Modern Java is fast. With improvements in JVM technology and the introduction of features like ahead-of-time compilation, Java microservices can deliver outstanding performance.
Scalability: Java’s ability to handle concurrent processing makes it ideal for building scalable microservices that can handle high loads.
Enterprise Readiness: Java’s long history in enterprise environments means it comes with battle-tested solutions for security, monitoring, and management – all crucial aspects of microservices architecture.
With these advantages, it’s no wonder that Java has become a go-to language for building microservices. Let’s dive deeper into how we can leverage Java to create robust, scalable microservices.
Building Your First Java Microservice
Enough theory – let’s get our hands dirty and build a simple Java microservice! We’ll use Spring Boot, one of the most popular frameworks for building microservices in Java. Our microservice will be a simple “Hello, World!” application that responds to HTTP requests.
First, let’s set up our project. You can use Spring Initializer (https://start.spring.io/) to create a new Spring Boot project with the web dependency. Once you have your project set up, create a new Java class called HelloWorldController
:
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RestController;
@RestController
public class HelloWorldController {
@GetMapping("/hello")
public String hello() {
return "Hello, Microservices World!";
}
}
This simple controller defines a single endpoint that responds to GET requests at the /hello
path. Now, let’s create our main application class:
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
@SpringBootApplication
public class HelloWorldApplication {
public static void main(String[] args) {
SpringApplication.run(HelloWorldApplication.class, args);
}
}
That’s it! You’ve just created your first Java microservice. When you run this application, it will start an embedded web server (usually Tomcat) and expose the /hello
endpoint. You can test it by navigating to http://localhost:8080/hello
in your web browser.
This example might seem simple, but it demonstrates the core concept of a microservice: a small, focused piece of functionality exposed via an API. In a real-world scenario, you’d have multiple such services, each responsible for a specific business capability.
Microservices Communication: RESTful APIs and Beyond
One of the key aspects of microservices architecture is how services communicate with each other. In our previous example, we created a RESTful API endpoint, which is one of the most common ways for microservices to interact. RESTful APIs are simple, stateless, and easy to understand, making them an excellent choice for microservices communication.
Let’s expand our example to include a second microservice that calls our Hello World service:
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RestController;
import org.springframework.web.client.RestTemplate;
@RestController
public class GreetingController {
private final RestTemplate restTemplate;
public GreetingController(RestTemplate restTemplate) {
this.restTemplate = restTemplate;
}
@GetMapping("/greeting")
public String greeting() {
String helloResponse = restTemplate.getForObject("http://localhost:8080/hello", String.class);
return "Greeting: " + helloResponse;
}
}
In this example, we’re using Spring’s RestTemplate
to make an HTTP call to our Hello World service. This demonstrates how one microservice can consume the API of another.
While REST is popular, it’s not the only communication pattern in the microservices world. Other common approaches include:
Message Queues: For asynchronous communication, message queues like RabbitMQ or Apache Kafka can be used to decouple services and handle high volumes of messages.
gRPC: Google’s high-performance RPC framework is gaining popularity for its efficiency and strong typing.
GraphQL: This query language for APIs provides a more flexible and efficient alternative to REST, allowing clients to request exactly the data they need.
The choice of communication pattern depends on your specific use case, performance requirements, and team expertise. It’s common to see a mix of these patterns in a single microservices ecosystem.
Containerization and Orchestration: Docker and Kubernetes
No discussion of microservices would be complete without mentioning containerization and orchestration. These technologies have revolutionized how we deploy and manage microservices at scale.
Docker allows you to package your microservice and all its dependencies into a standardized unit called a container. This ensures consistency across different environments and makes deployment a breeze. Here’s a simple Dockerfile for our Hello World microservice:
FROM openjdk:11-jre-slim
COPY target/hello-world-0.0.1-SNAPSHOT.jar app.jar
ENTRYPOINT ["java","-jar","/app.jar"]
This Dockerfile creates a lightweight container with Java 11 and our application JAR file. You can build and run this container with a few simple commands:
docker build -t hello-world-microservice .
docker run -p 8080:8080 hello-world-microservice
While Docker solves the problem of packaging and running individual microservices, managing a large number of containers across multiple hosts is challenging. This is where Kubernetes comes in. Kubernetes is an open-source container orchestration platform that automates the deployment, scaling, and management of containerized applications.
With Kubernetes, you can define how your microservices should be deployed and scaled using declarative YAML files. Here’s a simple example of a Kubernetes deployment for our Hello World service:
apiVersion: apps/v1
kind: Deployment
metadata:
name: hello-world
spec:
replicas: 3
selector:
matchLabels:
app: hello-world
template:
metadata:
labels:
app: hello-world
spec:
containers:
- name: hello-world
image: hello-world-microservice:latest
ports:
- containerPort: 8080
This YAML file tells Kubernetes to create three replicas of our Hello World service, ensuring high availability and load balancing. Kubernetes takes care of distributing these replicas across the available nodes in the cluster and managing their lifecycle.
Challenges and Best Practices in Microservices Architecture
While microservices offer numerous benefits, they also come with their own set of challenges. Let’s explore some of these challenges and the best practices to address them:
Distributed System Complexity: Microservices introduce the complexities of distributed systems. Network latency, partial failures, and data consistency become significant concerns. To address these, consider implementing patterns like Circuit Breaker (using libraries like Hystrix) and adopting eventual consistency where appropriate.
Service Discovery and Load Balancing: With multiple instances of services running, discovering and routing requests to the right instance becomes crucial. Tools like Netflix Eureka or Consul can help with service discovery, while Spring Cloud provides abstractions for these patterns.
Data Management: Each microservice typically has its own database, which can lead to data duplication and consistency issues. Consider using the Saga pattern for managing distributed transactions and event sourcing for maintaining data consistency across services.
Monitoring and Logging: With numerous services running independently, centralized logging and monitoring become essential. Tools like ELK stack (Elasticsearch, Logstash, Kibana) for logging and Prometheus with Grafana for monitoring can provide visibility into your microservices ecosystem.
Security: Securing inter-service communication and managing authentication/authorization across services is challenging. Implement OAuth 2.0 and JWT for authentication, and consider using an API Gateway for centralized security management.
Testing: Testing microservices requires a different approach compared to monolithic applications. Implement a combination of unit tests, integration tests, and contract tests. Tools like Spring Cloud Contract can help with consumer-driven contract testing.
By being aware of these challenges and implementing appropriate solutions, you can build robust, scalable, and maintainable microservices architectures.
Advanced Topics in Java Microservices
As you delve deeper into the world of Java microservices, you’ll encounter several advanced topics that can take your microservices architecture to the next level. Let’s explore a few of these:
Event-Driven Architecture: Event-driven microservices use events to communicate state changes between services. This approach can lead to more loosely coupled and scalable systems. Spring Cloud Stream provides abstractions for building event-driven microservices with various message brokers.
Here’s a simple example of a service that publishes events using Spring Cloud Stream:
import org.springframework.cloud.stream.annotation.EnableBinding;
import org.springframework.cloud.stream.messaging.Source;
import org.springframework.messaging.support.MessageBuilder;
import org.springframework.stereotype.Service;
@Service
@EnableBinding(Source.class)
public class OrderService {
private final Source source;
public OrderService(Source source) {
this.source = source;
}
public void createOrder(Order order) {
// Process order...
source.output().send(MessageBuilder.withPayload(order).build());
}
}
Service Mesh: As your microservices ecosystem grows, managing service-to-service communication becomes increasingly complex. A service mesh like Istio can help by providing features like traffic management, security, and observability at the network level.
Reactive Programming: Reactive programming can help build more responsive and resilient microservices. Spring WebFlux, part of the Spring 5 framework, allows you to build reactive microservices:
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RestController;
import reactor.core.publisher.Mono;
@RestController
public class ReactiveHelloWorldController {
@GetMapping("/reactive-hello")
public Mono<String> hello() {
return Mono.just("Hello, Reactive Microservices World!");
}
}
Domain-Driven Design (DDD): DDD principles can help you design microservices that align closely with business domains. This leads to more maintainable and scalable architectures. Consider using concepts like Bounded Contexts and Aggregates when designing your microservices.
Serverless Microservices: Serverless computing takes the idea of microservices even further by allowing you to deploy individual functions rather than entire services. Frameworks like Spring Cloud Function allow you to write cloud-agnostic serverless functions:
import java.util.function.Function;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.springframework.context.annotation.Bean;
@SpringBootApplication
public class ServerlessFunctionApplication {
public static void main(String[] args) {
SpringApplication.run(ServerlessFunctionApplication.class, args);
}
@Bean
public Function<String, String> uppercase() {
return String::toUpperCase;
}
}
These advanced topics represent the cutting edge of microservices development. As you explore them, you’ll find new ways to make your microservices more efficient, scalable, and maintainable.
Conclusion
As we wrap up our journey through the world of Java microservices, it’s clear that this architectural style represents a significant shift in how we build and deploy software. From improved scalability and flexibility to enhanced team productivity and faster time-to-market, microservices offer compelling benefits for modern software development.
We’ve covered a lot of ground in this post, from the basic concepts of microservices to advanced topics like event-driven architecture and serverless computing. We’ve seen how Java, with its rich ecosystem and enterprise-ready features, is perfectly positioned to take advantage of the microservices revolution.
But remember, microservices are not a silver bullet. They come with their own set of challenges and complexities. The key to success lies in understanding these challenges and implementing best practices to address them. Start small, focus on clear service boundaries, invest in automation, and continuously monitor and optimize your microservices ecosystem.
As you embark on your own microservices journey, keep learning and experimenting. The world of microservices is constantly evolving, with new tools, frameworks, and best practices emerging all the time. Stay curious, stay engaged, and most importantly, enjoy the process of building scalable, resilient, and flexible applications with Java microservices.
The future of software architecture is here, and it’s micro. Are you ready to embrace it?
Disclaimer: This blog post is intended for informational purposes only. While we strive for accuracy, technology and best practices in the field of microservices are rapidly evolving. Always refer to official documentation and consult with experienced professionals when implementing microservices architectures in production environments. If you notice any inaccuracies in this post, please report them so we can correct them promptly.