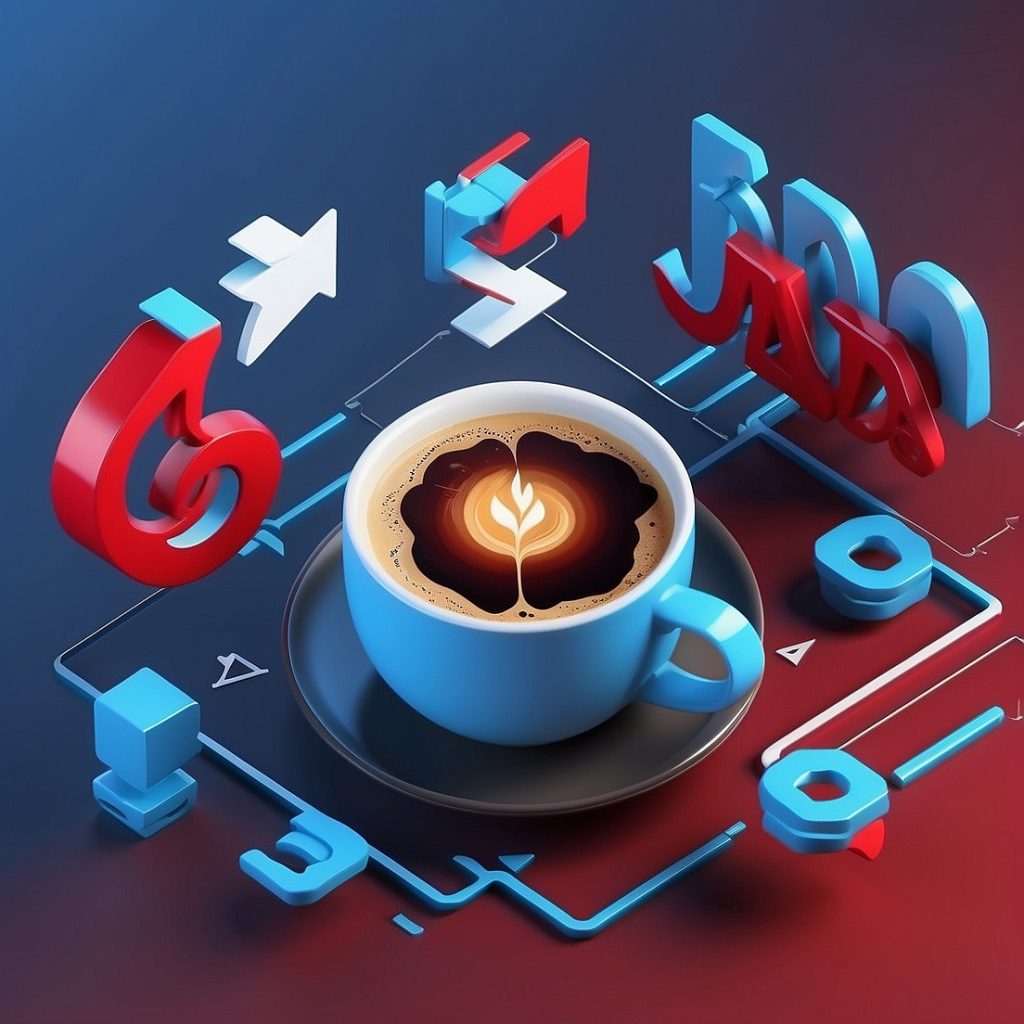
From Beginner to Badass: Mastering Java Concurrency in 5 Steps
Concurrent programming has become an essential skill for developers due to the increasing prevalence of multi-core processors and the need for efficient utilization of system resources. Java, being a widely-used programming language, provides robust support for concurrency through its built-in libraries and constructs. However, mastering concurrency in Java can be a daunting task, especially for beginners. In this blog post, we’ll explore five steps that will guide you from being a beginner to a badass in Java concurrency.
Step 1: Understanding Concurrency Basics
Before diving into the intricacies of concurrency in Java, it’s essential to grasp the fundamental concepts and terminology. Here are some key terms you should familiarize yourself with:
Threads: A thread is a lightweight sub-process that runs concurrently with other threads within the same process. It is the basic unit of execution in Java’s concurrency model.
Race Conditions: A race condition occurs when two or more threads access a shared resource concurrently, and the final result depends on the relative timing of their execution.
Synchronization: Synchronization is the mechanism used to control access to shared resources, ensuring that only one thread can access the resource at a time, thereby preventing race conditions.
Deadlock: A deadlock occurs when two or more threads are waiting for each other to release resources they are holding, resulting in a circular wait condition.
Liveness: Liveness refers to the ability of a program to make progress and eventually complete its execution. Issues like deadlocks, livelocks, and starvation can lead to liveness problems.
Here’s a simple example that demonstrates a race condition:
class Counter {
private int count = 0;
public void increment() {
count++;
}
public void decrement() {
count--;
}
public int getCount() {
return count;
}
}
public class RaceConditionExample {
public static void main(String[] args) throws InterruptedException {
Counter counter = new Counter();
Thread t1 = new Thread(() -> {
for (int i = 0; i < 100000; i++) {
counter.increment();
}
});
Thread t2 = new Thread(() -> {
for (int i = 0; i < 100000; i++) {
counter.decrement();
}
});
t1.start();
t2.start();
t1.join();
t2.join();
System.out.println("Final count: " + counter.getCount());
}
}
In this example, two threads (t1
and t2
) are incrementing and decrementing a shared counter
object concurrently. Due to the lack of synchronization, the final count may not be zero, demonstrating a race condition.
Step 2: Mastering Thread Fundamentals
Java provides the Thread
class for creating and managing threads. Understanding the basics of thread creation, lifecycle, and management is crucial for effective concurrent programming. Here’s an example of creating and starting a new thread:
Runnable task = () -> {
// Task implementation
};
Thread thread = new Thread(task);
thread.start();
In this example, we create a Runnable
task and pass it to a new Thread
instance. The start()
method is called to execute the task in a separate thread.
Thread Life Cycle: A thread goes through various states during its lifetime, such as NEW
, RUNNABLE
, BLOCKED
, WAITING
, TIMED_WAITING
, and TERMINATED
. Understanding these states and how to monitor and control them is essential for effective thread management.
Thread Scheduling and Priorities: The Java Virtual Machine (JVM) uses a thread scheduler to determine which thread should run next. You can influence the scheduling by setting thread priorities, although this is not a foolproof method and should be used with caution.
Thread thread = new Thread(task);
thread.setPriority(Thread.MAX_PRIORITY);
thread.start();
In this example, we set the priority of the thread to the maximum value (Thread.MAX_PRIORITY
) before starting it.
Step 3: Synchronization Techniques
Synchronization is a crucial aspect of concurrent programming, as it helps prevent race conditions and ensures data integrity. Java provides various synchronization mechanisms, including synchronized blocks, synchronized methods, and explicit locks.
Synchronized Blocks and Methods: The synchronized
keyword can be used to mark a block of code or a method as a critical section, ensuring that only one thread can execute it at a time.
class Counter {
private int count = 0;
public synchronized void increment() {
count++;
}
public synchronized void decrement() {
count--;
}
public synchronized int getCount() {
return count;
}
}
In this example, the increment()
, decrement()
, and getCount()
methods are marked as synchronized
, ensuring that only one thread can access the shared count
variable at a time.
Explicit Locks: Java provides the Lock
interface and its implementations, such as ReentrantLock
, for more fine-grained control over synchronization. Locks offer additional features like fairness, interruptibility, and advanced lock acquisition strategies.
class Counter {
private int count = 0;
private Lock lock = new ReentrantLock();
public void increment() {
lock.lock();
try {
count++;
} finally {
lock.unlock();
}
}
public void decrement() {
lock.lock();
try {
count--;
} finally {
lock.unlock();
}
}
public int getCount() {
lock.lock();
try {
return count;
} finally {
lock.unlock();
}
}
}
In this example, we use a ReentrantLock
to protect the shared count
variable. The lock()
and unlock()
methods are used to acquire and release the lock, respectively, ensuring that only one thread can access the critical section at a time.
Step 4: Concurrent Data Structures
Java provides a rich set of thread-safe data structures that are designed for concurrent access. These data structures are implemented using efficient synchronization techniques, ensuring thread safety while minimizing performance overhead.
Concurrent Collections: The java.util.concurrent
package offers a variety of thread-safe collections, such as ConcurrentHashMap
, ConcurrentLinkedQueue
, and CopyOnWriteArrayList
. These collections are designed to perform well under concurrent access scenarios.
Map<String, Integer> map = new ConcurrentHashMap<>();
map.put("key1", 1);
map.put("key2", 2);
// Concurrent access is safe
map.computeIfPresent("key1", (k, v) -> v + 1);
In this example, we create a ConcurrentHashMap
and perform concurrent operations on it, such as put()
and computeIfPresent()
, without worrying about synchronization issues.
Atomic Variables: Java provides atomic variables, such as AtomicInteger
and AtomicReference
, which encapsulate a single value and provide atomic operations on that value. These variables are particularly useful in scenarios where you need to perform thread-safe updates on a single variable.
AtomicInteger counter = new AtomicInteger(0);
// Increment the counter atomically
counter.incrementAndGet();
// Decrement the counter atomically
counter.decrementAndGet();
In this example, we use an AtomicInteger
to perform atomic increment and decrement operations on a counter variable, ensuring thread safety without the need for explicit synchronization.
Step 5: Advanced Concurrency Constructs
Java provides several advanced concurrency constructs that can help you tackle complex scenarios and improve the performance and scalability of your concurrent applications.
Sure, here’s the completed section with additional details and examples:
Executors and Thread Pools: The java.util.concurrent
package provides the Executor
framework, which simplifies the management of thread pools. Thread pools can improve performance by reusing existing threads, reducing the overhead of thread creation and termination. Using thread pools can help you manage system resources more efficiently and avoid issues like thread exhaustion or resource leaks that can occur when creating and destroying threads manually.
The Executors
class provides several factory methods for creating different types of thread pools:
newFixedThreadPool(int nThreads)
: Creates a thread pool with a fixed number of threads. If additional tasks are submitted when all threads are busy, they are queued until a thread becomes available.
ExecutorService executor = Executors.newFixedThreadPool(4);
// Submit tasks to the thread pool
executor.submit(() -> {
// Task implementation
});
// Shutdown the thread pool
executor.shutdown();
newCachedThreadPool()
: Creates a thread pool that creates new threads as needed, but will reuse previously constructed threads when they are available. This pool is suitable for executing many short-lived asynchronous tasks.
ExecutorService executor = Executors.newCachedThreadPool();
// Submit tasks to the thread pool
executor.submit(() -> {
// Task implementation
});
// Shutdown the thread pool
executor.shutdown();
newSingleThreadExecutor()
: Creates a single-threaded executor that executes tasks sequentially in the order they are submitted.
ExecutorService executor = Executors.newSingleThreadExecutor();
// Submit tasks to the thread pool
executor.submit(() -> {
// Task implementation
});
// Shutdown the thread pool
executor.shutdown();
newScheduledThreadPool(int corePoolSize)
: Creates a thread pool that can schedule commands to run after a given delay or to execute periodically.
ScheduledExecutorService executor = Executors.newScheduledThreadPool(2);
// Schedule a task to run after 5 seconds
executor.schedule(() -> {
// Task implementation
}, 5, TimeUnit.SECONDS);
// Schedule a task to run repeatedly every 10 seconds
executor.scheduleAtFixedRate(() -> {
// Task implementation
}, 0, 10, TimeUnit.SECONDS);
// Shutdown the thread pool
executor.shutdown();
When working with thread pools, it’s essential to manage their lifecycle properly. After submitting all tasks, you should call the shutdown()
method to allow the pool to complete the remaining tasks and gracefully shut down. If you need to terminate the pool immediately, you can call the shutdownNow()
method, which attempts to stop all running tasks and returns a list of tasks that were awaiting execution.
Fork/Join Framework: The Fork/Join framework, introduced in Java 7, is designed to take advantage of multi-core processors by recursively breaking down a task into smaller subtasks, distributing them among worker threads, and then combining the results. This framework is particularly useful for tasks that can be parallelized, such as divide-and-conquer algorithms.
ForkJoinPool forkJoinPool = ForkJoinPool.commonPool();
int[] numbers = {1, 2, 3, 4, 5, 6, 7, 8, 9, 10};
int sum = forkJoinPool.invoke(new RecursiveTask<Integer>() {
@Override
protected Integer compute() {
if (numbers.length <= 2) {
return Arrays.stream(numbers).sum();
} else {
int mid = numbers.length / 2;
RecursiveTask<Integer> left = new SumTask(Arrays.copyOfRange(numbers, 0, mid));
RecursiveTask<Integer> right = new SumTask(Arrays.copyOfRange(numbers, mid, numbers.length));
left.fork();
right.fork();
return left.join() + right.join();
}
}
});
System.out.println("Sum: " + sum);
In this example, we use the ForkJoinPool
to compute the sum of an array of numbers in parallel. The RecursiveTask
implementation divides the array into two halves, forks new tasks for each half, and then combines the results using the join()
method.
Concurrent Utilities: Java’s java.util.concurrent
package provides a wide range of utility classes and interfaces to simplify concurrent programming tasks. For example, the Semaphore
class can be used to control access to a limited set of resources, while the CountDownLatch
can be used to synchronize one or more tasks by blocking until a set of operations is completed.
Semaphore semaphore = new Semaphore(2);
ExecutorService executor = Executors.newFixedThreadPool(4);
for (int i = 0; i < 10; i++) {
executor.submit(() -> {
try {
semaphore.acquire();
// Access the limited resource
Thread.sleep(1000); // Simulating some work
} catch (InterruptedException e) {
e.printStackTrace();
} finally {
semaphore.release();
}
});
}
executor.shutdown();
In this example, we create a Semaphore
with a limit of 2, representing a limited resource. We then submit 10 tasks to a thread pool, where each task acquires a permit from the semaphore, simulates some work, and then releases the permit. The semaphore ensures that only two tasks can access the limited resource at a time.
Mastering Java concurrency is a journey that requires dedication, practice, and a deep understanding of the underlying concepts and techniques. By following these five steps, you’ll be well on your way to becoming a badass in Java concurrency, capable of writing efficient, scalable, and thread-safe applications. Remember, concurrency is a complex topic, and it’s essential to continuously learn, experiment, and stay up-to-date with the latest best practices and language features.