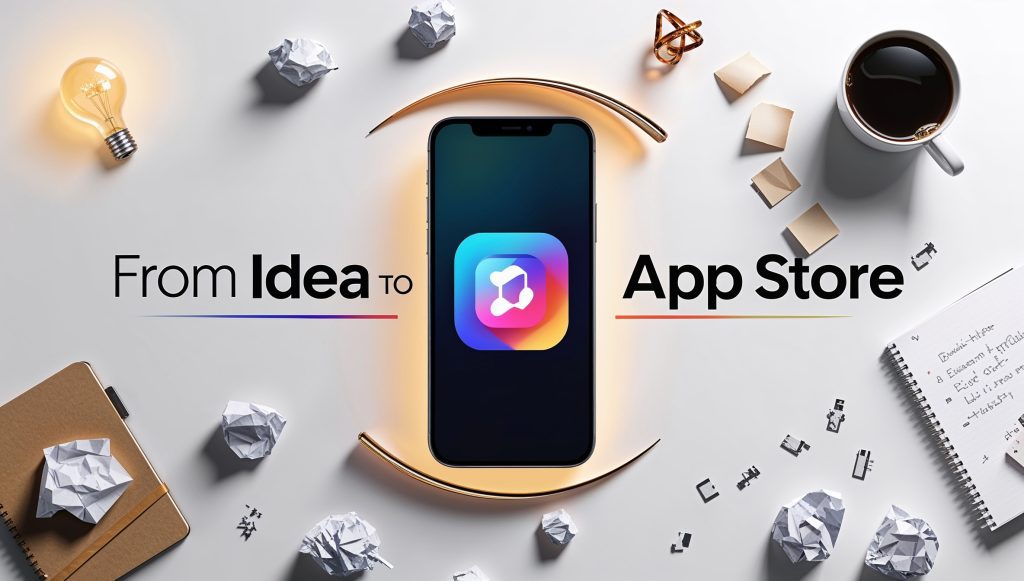
From Idea to App Store
Ever had a lightbulb moment where you thought, “Hey, this would make a great app!”? You’re not alone. In today’s digital age, mobile apps have become an integral part of our lives, solving problems and providing entertainment at our fingertips. But how do you transform that brilliant idea into a fully-fledged app that people can download from the App Store? Enter the world of DevOps – a set of practices that combines software development (Dev) and IT operations (Ops) to streamline the app creation process. In this blog, we’ll take you on a journey from conceptualization to launch, exploring the DevOps methodologies that can turn your app dreams into reality. So, buckle up and get ready for an exciting ride through the app development landscape!
Understanding the DevOps Approach
What is DevOps, and why does it matter?
Before we dive into the nitty-gritty of app development, let’s take a moment to understand what DevOps is all about. DevOps is more than just a buzzword; it’s a culture, a philosophy, and a set of practices that aim to improve collaboration between development and operations teams. By breaking down silos and fostering communication, DevOps enables faster, more reliable software delivery. This approach is particularly crucial in the fast-paced world of mobile app development, where user expectations are high, and competition is fierce. With DevOps, you can iterate quickly, respond to user feedback swiftly, and maintain a high-quality app that stands out in the crowded App Store. So, whether you’re a solo developer or part of a larger team, embracing DevOps principles can be the key to your app’s success.
Planning Your App: The Blueprint for Success
Defining your app’s purpose and features
Every great app starts with a solid plan. This is where you’ll define your app’s purpose, target audience, and key features. Ask yourself: What problem does your app solve? Who will use it? What makes it unique? These questions will help you create a clear vision for your app and guide your development process. Remember, simplicity is often the key to success. Start with a core set of features that address your users’ primary needs, and plan for future iterations to add more functionality. This approach, known as the Minimum Viable Product (MVP) strategy, allows you to get your app to market faster and gather real user feedback to inform future development.
Creating user stories and defining acceptance criteria
Once you have a high-level plan, it’s time to break it down into manageable chunks. User stories are a great way to do this. They describe features from the user’s perspective, helping you focus on delivering value. For example:
As a fitness enthusiast,
I want to track my daily steps,
So that I can monitor my activity levels and meet my fitness goals.
Accompany each user story with acceptance criteria – specific conditions that must be met for the feature to be considered complete. This helps ensure that everyone on the team has a clear understanding of what needs to be built. For instance:
Acceptance Criteria for Step Tracking:
1. The app accurately counts steps using the device's built-in sensors.
2. Users can set daily step goals.
3. The app displays current step count and progress towards the daily goal.
4. Users receive notifications when they reach their daily goal.
By creating detailed user stories and acceptance criteria, you’re laying the groundwork for a focused and efficient development process.
Setting Up Your Development Environment
Choosing the right tools for the job
With your plan in place, it’s time to set up your development environment. The tools you choose can significantly impact your productivity and the quality of your app. For iOS development, you’ll need Xcode, Apple’s integrated development environment (IDE). For Android, Android Studio is the go-to choice. But DevOps is about more than just coding tools. You’ll also need version control systems like Git, continuous integration/continuous deployment (CI/CD) tools like Jenkins or GitLab CI, and project management tools like Jira or Trello. These tools will help you manage your code, automate your build and deployment processes, and keep track of your project’s progress.
Configuring your version control system
Version control is a crucial aspect of DevOps, allowing you to track changes, collaborate with team members, and maintain different versions of your app. Git is the most popular version control system, and platforms like GitHub or GitLab provide additional collaboration features. Here’s a quick example of how to initialize a Git repository and make your first commit:
# Initialize a new Git repository
git init
# Add all files to the staging area
git add .
# Commit the changes with a descriptive message
git commit -m "Initial commit: Basic app structure"
# Create a new branch for feature development
git branch feature/user-authentication
# Switch to the new branch
git checkout feature/user-authentication
By using version control from the start, you’re setting yourself up for a smooth development process and making it easier to collaborate with others as your project grows.
Writing Clean, Maintainable Code
Adopting coding best practices
As you start coding your app, it’s essential to establish and follow coding best practices. This includes using meaningful variable and function names, writing comments to explain complex logic, and adhering to the DRY (Don’t Repeat Yourself) principle. Consistent code formatting also plays a crucial role in maintaining readability. Many IDEs offer auto-formatting features, or you can use tools like SwiftLint for iOS or ktlint for Android to enforce style guidelines automatically. Here’s an example of clean, well-commented Swift code:
/// Represents a user in the fitness tracking app
struct User {
let id: UUID
var name: String
var dailyStepGoal: Int
/// Initializes a new User instance
/// - Parameters:
/// - name: The user's name
/// - dailyStepGoal: The user's daily step goal (default: 10000)
init(name: String, dailyStepGoal: Int = 10000) {
self.id = UUID()
self.name = name
self.dailyStepGoal = dailyStepGoal
}
/// Calculates the percentage of the daily step goal achieved
/// - Parameter currentSteps: The number of steps taken today
/// - Returns: The percentage of the goal achieved (0-100)
func calculateGoalProgress(currentSteps: Int) -> Double {
let progress = Double(currentSteps) / Double(dailyStepGoal) * 100
return min(progress, 100) // Ensure the progress doesn't exceed 100%
}
}
By writing clean, well-documented code from the start, you’re making life easier for yourself and any future developers who might work on your project.
Implementing Continuous Integration
Automating your build and test processes
Continuous Integration (CI) is a core principle of DevOps that involves automatically building and testing your code every time you make changes. This helps catch bugs early and ensures that your app remains in a releasable state. There are many CI tools available, but let’s look at an example using GitLab CI. First, you’ll need to create a .gitlab-ci.yml
file in your project root:
stages:
- build
- test
variables:
LC_ALL: "en_US.UTF-8"
LANG: "en_US.UTF-8"
before_script:
- pod install
build_project:
stage: build
script:
- xcodebuild clean -workspace YourApp.xcworkspace -scheme "YourApp" | xcpretty
- xcodebuild test -workspace YourApp.xcworkspace -scheme "YourApp" -destination 'platform=iOS Simulator,name=iPhone 12,OS=latest' | xcpretty -s
run_tests:
stage: test
script:
- xcodebuild test -workspace YourApp.xcworkspace -scheme "YourApp" -destination 'platform=iOS Simulator,name=iPhone 12,OS=latest' | xcpretty -s
This configuration sets up a pipeline that builds your project and runs tests on every push to the repository. By implementing CI, you’re ensuring that your app remains stable as you add new features and make changes.
Embracing Test-Driven Development
Writing tests before code
Test-Driven Development (TDD) is a software development approach where you write tests before implementing the actual code. This might seem counterintuitive at first, but it helps ensure that your code meets the specified requirements and remains maintainable as your app grows. Let’s look at an example of TDD in action, using Swift and XCTest:
import XCTest
@testable import YourApp
class StepCounterTests: XCTestCase {
func testStepCountIncrement() {
// Arrange
let stepCounter = StepCounter()
let initialCount = stepCounter.stepCount
// Act
stepCounter.incrementSteps(by: 100)
// Assert
XCTAssertEqual(stepCounter.stepCount, initialCount + 100, "Step count should increase by 100")
}
func testDailyGoalAchievement() {
// Arrange
let stepCounter = StepCounter(dailyGoal: 10000)
// Act
stepCounter.incrementSteps(by: 10000)
// Assert
XCTAssertTrue(stepCounter.isDailyGoalAchieved, "Daily goal should be achieved")
}
}
After writing these tests, you would then implement the StepCounter
class to make the tests pass. This approach helps you focus on the desired behavior of your app and catch edge cases early in the development process.
Implementing Continuous Deployment
Automating your release process
Continuous Deployment (CD) takes CI a step further by automatically deploying your app to testing or production environments when certain conditions are met. For mobile apps, this often means automatically submitting your app to TestFlight or the Google Play Console for beta testing. Here’s an example of how you might set up CD for an iOS app using Fastlane and GitLab CI:
First, set up your Fastfile:
default_platform(:ios)
platform :ios do
desc "Push a new beta build to TestFlight"
lane :beta do
build_app(workspace: "YourApp.xcworkspace", scheme: "YourApp")
upload_to_testflight
end
end
Then, update your .gitlab-ci.yml
file to include a deployment stage:
stages:
- build
- test
- deploy
# ... (previous build and test stages)
deploy_to_testflight:
stage: deploy
script:
- fastlane beta
only:
- main # Only deploy when changes are pushed to the main branch
With this setup, every time you push changes to your main branch, your app will be automatically built, tested, and deployed to TestFlight for beta testing. This rapid feedback loop is a key advantage of the DevOps approach, allowing you to iterate quickly and respond to user feedback.
Monitoring and Analytics: Understanding Your Users
Implementing app analytics
Once your app is in the hands of users, it’s crucial to understand how they’re using it. This is where app analytics come in. Tools like Firebase Analytics, Mixpanel, or Apple’s App Store Connect can provide valuable insights into user behavior, app performance, and crash reports. Here’s a quick example of how to set up Firebase Analytics in an iOS app:
First, add the Firebase SDK to your project using CocoaPods. Add this to your Podfile:
pod 'Firebase/Analytics'
Then, in your AppDelegate, set up Firebase:
import UIKit
import Firebase
@UIApplicationMain
class AppDelegate: UIResponder, UIApplicationDelegate {
func application(_ application: UIApplication, didFinishLaunchingWithOptions launchOptions: [UIApplication.LaunchOptionsKey: Any]?) -> Bool {
FirebaseApp.configure()
return true
}
}
Now you can track specific events in your app:
import Firebase
// Track when a user achieves their daily step goal
Analytics.logEvent("daily_goal_achieved", parameters: [
"steps": 10000,
"goal": 10000
])
By implementing analytics, you gain valuable insights into how users interact with your app, helping you make data-driven decisions for future updates and features.
Iterating and Improving: The Feedback Loop
Responding to user feedback
The journey doesn’t end when your app hits the App Store. In fact, that’s when the real work begins. DevOps is all about continuous improvement, and that means listening to your users and iterating on your app based on their feedback. Regularly check your app’s reviews and ratings, and use the insights from your analytics to identify areas for improvement. Maybe users are struggling with a particular feature, or perhaps there’s a common request for new functionality. Use this information to prioritize your backlog and plan your next sprint.
Planning for scalability
As your user base grows, you’ll need to ensure your app can handle the increased load. This might involve optimizing your code, refactoring your architecture, or scaling your backend infrastructure. DevOps practices like infrastructure as code (IaC) can help you manage this growth efficiently. For example, you might use a tool like Terraform to manage your cloud resources:
provider "aws" {
region = "us-west-2"
}
resource "aws_instance" "app_server" {
ami = "ami-0c55b159cbfafe1f0"
instance_type = "t2.micro"
tags = {
Name = "AppServerInstance"
}
}
resource "aws_db_instance" "default" {
allocated_storage = 10
engine = "mysql"
engine_version = "5.7"
instance_class = "db.t3.micro"
name = "mydb"
username = "foo"
password = "foobarbaz"
parameter_group_name = "default.mysql5.7"
skip_final_snapshot = true
}
This Terraform configuration sets up an EC2 instance for your app server and an RDS instance for your database. By managing your infrastructure as code, you can easily version control and replicate your setup, making it easier to scale as your app grows.
Security and Compliance: Protecting Your Users
Implementing security best practices
In today’s digital landscape, security is paramount. As a DevOps practitioner, it’s your responsibility to ensure that your app protects user data and complies with relevant regulations. This involves implementing secure coding practices, using encryption for sensitive data, and regularly updating your dependencies to patch known vulnerabilities. For example, in iOS development, you might use the Keychain to securely store sensitive user data:
import Security
class KeychainManager {
static func save(key: String, data: Data) -> OSStatus {
let query = [
kSecClass as String : kSecClassGenericPassword as String,
kSecAttrAccount as String : key,
kSecValueData as String : data
] as [String : Any]
SecItemDelete(query as CFDictionary)
return SecItemAdd(query as CFDictionary, nil)
}
static func load(key: String) -> Data? {
let query = [
kSecClass as String : kSecClassGenericPassword,
kSecAttrAccount as String : key,
kSecReturnData as String : kCFBooleanTrue!,
kSecMatchLimit as String : kSecMatchLimitOne
] as [String : Any]
var dataTypeRef: AnyObject?
let status: OSStatus = SecItemCopyMatching(query as CFDictionary, &dataTypeRef)
if status == noErr {
return dataTypeRef as! Data?
} else {
return nil
}
}
}
This KeychainManager
class provides a simple interface for securely storing and retrieving data using the iOS Keychain. By implementing such security measures, you’re not only protecting your users but also building trust in your app.
Celebrating Success and Learning from Failures
The importance of retrospectives
As you navigate the DevOps journey, it’s crucial to take time to reflect on your successes and learn from your failures. Regular retrospectives with your team (or self-reflection if you’re a solo developer) can help you identify what’s working well and what needs improvement. Maybe your CI/CD pipeline is running smoothly, but your test coverage could use some work. Or perhaps your app is performing well, but user engagement isn’t as high as you’d like. These retrospectives are an opportunity to celebrate your wins and strategize on how to overcome challenges. Remember, DevOps is about continuous improvement, so treat every setback as a learning opportunity.
Measuring success beyond downloads
While app store rankings and download numbers are important metrics, they don’t tell the whole story of your app’s success. Consider tracking metrics like user retention, daily active users (DAU), and customer lifetime value (CLV). These can give you a more nuanced understanding of how well your app is meeting user needs. For example, you might use Firebase Analytics to track user retention:
import Firebase
// In your app delegate or wherever you initialize Firebase
Analytics.setUserProperty("premium", forName: "user_type")
// Later, when tracking an important event
Analytics.logEvent("level_completed", parameters: [
"level_name": "Beginner",
"time_spent": 300
])
By tracking these deeper metrics, you can gain insights into user behavior and make data-driven decisions to improve your app.
The Never-Ending Journey of DevOps
Embracing lifelong learning
The world of app development and DevOps is constantly evolving. New technologies, frameworks, and best practices emerge regularly, and it’s crucial to stay updated. Make continuous learning a part of your DevOps journey. This might involve attending conferences, participating in online courses, or contributing to open-source projects. For example, you might explore emerging technologies like SwiftUI for iOS development:
import SwiftUI
struct ContentView: View {
@State private var stepCount = 0
var body: some View {
VStack {
Text("Steps Today: \(stepCount)")
.font(.title)
Button("Add Steps") {
stepCount += 100
}
.padding()
.background(Color.blue)
.foregroundColor(.white)
.cornerRadius(10)
}
}
}
By staying curious and open to new technologies, you ensure that your skills remain sharp and your apps stay cutting-edge.
Summing It Up
As we wrap up this journey from idea to App Store, it’s clear that DevOps is more than just a set of practices – it’s a mindset. It’s about breaking down barriers, fostering collaboration, and continuously improving both your app and your development process. Whether you’re a solo developer with a bright idea or part of a larger team working on the next big thing, embracing DevOps principles can help you build better apps, faster.
Remember, the path from concept to successful app is rarely a straight line. There will be challenges, setbacks, and moments of doubt. But with the DevOps approach, you have the tools and mindset to overcome these obstacles. You’ll iterate quickly, learn from failures, and celebrate successes along the way.
So, as you embark on your own DevOps journey, keep an open mind, stay curious, and never stop learning. The App Store awaits your creation, and with DevOps as your guide, you’re well-equipped to turn your app dreams into reality. Who knows? Your app might just be the next big thing that changes how we live, work, or play. The adventure is yours for the taking – so what are you waiting for? Start coding, start learning, and start your DevOps journey today!
Disclaimer: This blog post is intended for informational purposes only. While we strive for accuracy, technologies and best practices in app development and DevOps are constantly evolving. Always refer to the most up-to-date documentation and guidelines when implementing any techniques or tools mentioned in this post. If you notice any inaccuracies, please report them so we can correct them promptly.