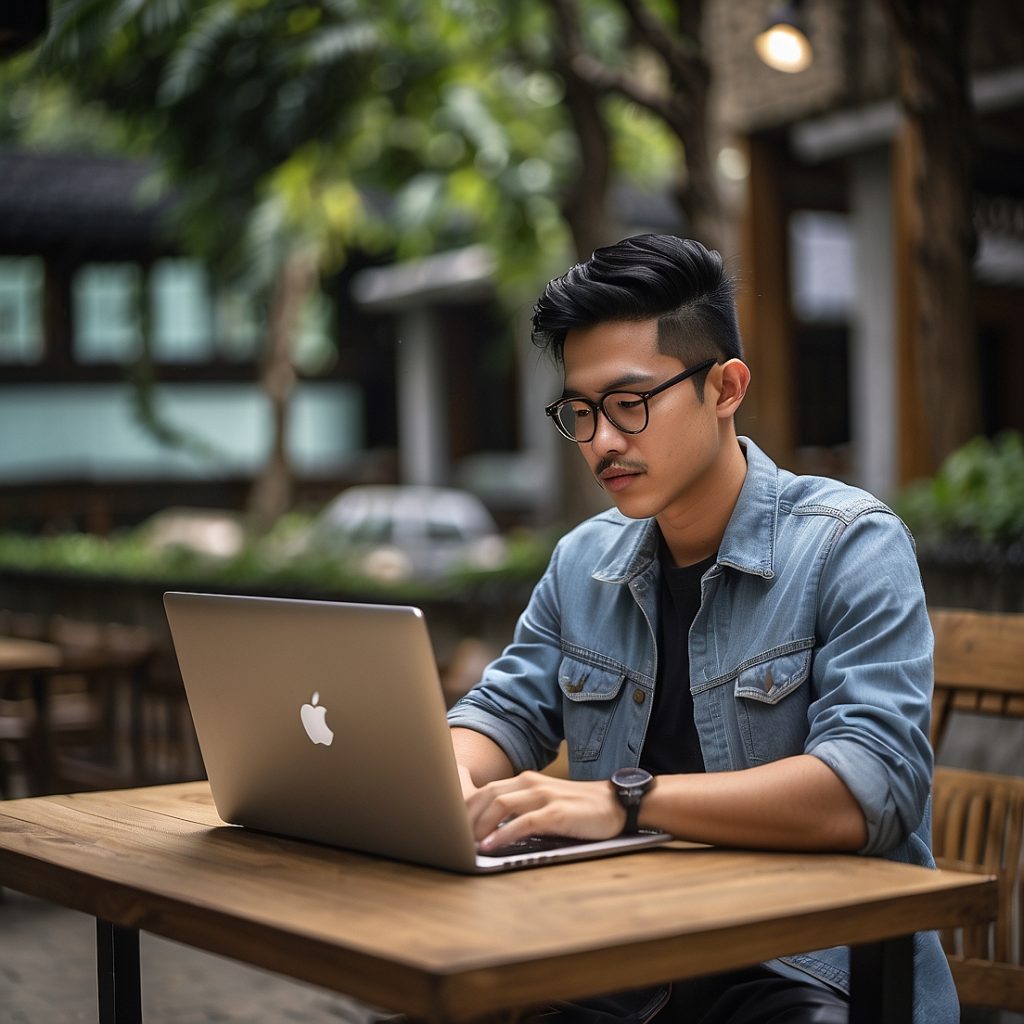
From Zero to Hero: Conquering Pythonic Problem Solving
If you’ve ever looked at a block of Python code and thought, “What kind of wizardry is this?”, then you’re in the right place. Welcome to your ultimate guide to mastering Pythonic problem solving. Whether you’re a complete newbie or a seasoned coder looking to level up, this blog will transform you from zero to hero with practical examples, clear explanations, and a sprinkle of humor to keep things interesting.
Getting Started with Python: The Basics
Before we dive into solving problems like a pro, let’s make sure we have a solid foundation. Python is known for its readability and simplicity, making it a great language for beginners. But don’t let that fool you; it’s powerful enough for seasoned developers as well.
Hello, World!
Let’s start with the classic “Hello, World!” program. This simple program is a rite of passage for every coder.
print("Hello, World!")
That’s it! One line of code and you’ve written your first Python program. Python’s simplicity is one of its greatest strengths.
Variables and Data Types
Understanding variables and data types is crucial. In Python, you don’t need to declare a variable’s type; you just assign a value to it.
# Integer
age = 25
# Float
height = 5.9
# String
name = "Alice"
# Boolean
is_student = True
Python supports several data types, and you can change the type of a variable at any time. This flexibility makes Python both powerful and easy to use.
Basic Operations
Python supports basic arithmetic operations, which are straightforward to use.
a = 10
b = 3
# Addition
print(a + b) # Output: 13
# Subtraction
print(a - b) # Output: 7
# Multiplication
print(a * b) # Output: 30
# Division
print(a / b) # Output: 3.3333333333333335
# Floor Division
print(a // b) # Output: 3
# Modulus
print(a % b) # Output: 1
# Exponentiation
print(a ** b) # Output: 1000
These operations form the backbone of many problem-solving techniques.
Control Flow: Making Decisions and Repeating Tasks
In programming, making decisions and repeating tasks are essential. Python offers elegant solutions with its control flow statements.
If Statements
The if
statement allows you to execute code based on a condition.
age = 18
if age >= 18:
print("You are an adult.")
else:
print("You are a minor.")
You can also use elif
for multiple conditions.
score = 85
if score >= 90:
print("Grade: A")
elif score >= 80:
print("Grade: B")
elif score >= 70:
print("Grade: C")
else:
print("Grade: F")
Loops
Loops let you repeat code multiple times. Python has for
and while
loops.
# For loop
for i in range(5):
print(i) # Output: 0 1 2 3 4
# While loop
count = 0
while count < 5:
print(count)
count += 1 # Output: 0 1 2 3 4
Loops are particularly useful for iterating over collections.
Break and Continue
Use break
to exit a loop early and continue
to skip to the next iteration.
# Break example
for i in range(10):
if i == 5:
break
print(i) # Output: 0 1 2 3 4
# Continue example
for i in range(10):
if i % 2 == 0:
continue
print(i) # Output: 1 3 5 7 9
Functions: Writing Reusable Code
Functions are a cornerstone of Pythonic problem solving. They help you write reusable and organized code.
Defining Functions
Here’s how you define and call a function in Python:
def greet(name):
return f"Hello, {name}!"
print(greet("Alice")) # Output: Hello, Alice!
Functions can take multiple arguments and return values.
def add(a, b):
return a + b
result = add(5, 3)
print(result) # Output: 8
Default Arguments and Keyword Arguments
Python allows you to set default values for arguments and use keyword arguments for clarity.
def greet(name, greeting="Hello"):
return f"{greeting}, {name}!"
print(greet("Bob")) # Output: Hello, Bob!
print(greet("Alice", "Hi")) # Output: Hi, Alice!
Lambda Functions
For short, throwaway functions, you can use lambda expressions.
add = lambda x, y: x + y
print(add(3, 5)) # Output: 8
Lambda functions are useful in functional programming paradigms and when passing functions as arguments.
Data Structures: Organizing Data Efficiently
Python provides powerful data structures to store and manipulate data efficiently.
Lists
Lists are ordered, mutable collections.
fruits = ["apple", "banana", "cherry"]
# Accessing elements
print(fruits[0]) # Output: apple
# Modifying elements
fruits[1] = "blueberry"
print(fruits) # Output: ['apple', 'blueberry', 'cherry']
# Adding elements
fruits.append("date")
print(fruits) # Output: ['apple', 'blueberry', 'cherry', 'date']
# Removing elements
fruits.remove("apple")
print(fruits) # Output: ['blueberry', 'cherry', 'date']
Tuples
Tuples are ordered, immutable collections.
coordinates = (10, 20)
# Accessing elements
print(coordinates[0]) # Output: 10
# Tuples are immutable, so you can't modify them
# coordinates[0] = 15 # This will raise an error
Dictionaries
Dictionaries store key-value pairs, offering fast lookups.
student = {
"name": "Alice",
"age": 20,
"grades": [85, 90, 92]
}
# Accessing values
print(student["name"]) # Output: Alice
# Modifying values
student["age"] = 21
print(student) # Output: {'name': 'Alice', 'age': 21, 'grades': [85, 90, 92]}
# Adding key-value pairs
student["major"] = "Computer Science"
print(student) # Output: {'name': 'Alice', 'age': 21, 'grades': [85, 90, 92], 'major': 'Computer Science'}
Sets
Sets store unique elements and offer efficient operations for set theory.
colors = {"red", "green", "blue"}
# Adding elements
colors.add("yellow")
print(colors) # Output: {'yellow', 'red', 'green', 'blue'}
# Removing elements
colors.remove("green")
print(colors) # Output: {'yellow', 'red', 'blue'}
List Comprehensions and Generator Expressions
Python offers concise ways to create lists and generators, making your code more Pythonic.
List Comprehensions
List comprehensions provide a concise way to create lists.
squares = [x**2 for x in range(10)]
print(squares) # Output: [0, 1, 4, 9, 16, 25, 36, 49, 64, 81]
You can include conditions in list comprehensions.
even_squares = [x**2 for x in range(10) if x % 2 == 0]
print(even_squares) # Output: [0, 4, 16, 36, 64]
Generator Expressions
Generator expressions create iterators that yield items one at a time.
squares_gen = (x**2 for x in range(10))
for square in squares_gen:
print(square)
Generators are memory efficient because they generate items on the fly.
Handling Errors: Exceptions and Assertions
Handling errors gracefully is a key part of Pythonic problem solving.
Try-Except Blocks
Use try-except
blocks to handle exceptions.
try:
result = 10 / 0
except ZeroDivisionError:
print("Cannot divide by zero!")
You can handle multiple exceptions and add an else
block for code that runs if no exceptions occur.
try:
value = int("42")
except ValueError:
print("Invalid number!")
else:
print("Conversion successful!")
Finally Block
Use finally
to run code regardless of whether an exception occurred.
try:
file = open("example.txt", "r")
content = file.read()
except FileNotFoundError:
print("File not found!")
finally:
file.close()
Assertions
Assertions help you catch bugs by verifying conditions.
def divide(a, b):
assert b != 0, "Division by zero!"
return a /
b
print(divide(10, 2)) # Output: 5.0
# print(divide(10, 0)) # This will raise an AssertionError
Object-Oriented Programming: Building Reusable Components
Object-oriented programming (OOP) allows you to create reusable and organized code.
Classes and Objects
Define a class using the class
keyword and create objects from it.
class Dog:
def __init__(self, name):
self.name = name
def bark(self):
return f"{self.name} says woof!"
my_dog = Dog("Buddy")
print(my_dog.bark()) # Output: Buddy says woof!
Inheritance
Inheritance allows you to create a new class based on an existing class.
class Animal:
def __init__(self, name):
self.name = name
def speak(self):
raise NotImplementedError("Subclasses must implement this method")
class Cat(Animal):
def speak(self):
return f"{self.name} says meow!"
my_cat = Cat("Whiskers")
print(my_cat.speak()) # Output: Whiskers says meow!
Encapsulation
Encapsulation restricts access to certain components and prevents accidental modifications.
class Account:
def __init__(self, owner, balance=0):
self.owner = owner
self.__balance = balance
def deposit(self, amount):
self.__balance += amount
def withdraw(self, amount):
if amount <= self.__balance:
self.__balance -= amount
else:
print("Insufficient funds!")
def get_balance(self):
return self.__balance
my_account = Account("Alice", 100)
my_account.deposit(50)
print(my_account.get_balance()) # Output: 150
my_account.withdraw(75)
print(my_account.get_balance()) # Output: 75
Polymorphism
Polymorphism allows you to use a unified interface for different data types.
class Bird:
def speak(self):
return "Tweet!"
class Fish:
def speak(self):
return "Blub!"
animals = [Bird(), Fish()]
for animal in animals:
print(animal.speak())
Advanced Pythonic Techniques: Decorators and Context Managers
For the advanced Pythonista, decorators and context managers provide powerful tools for clean, readable code.
Decorators
Decorators modify the behavior of functions or classes.
def my_decorator(func):
def wrapper():
print("Something is happening before the function is called.")
func()
print("Something is happening after the function is called.")
return wrapper
@my_decorator
def say_hello():
print("Hello!")
say_hello()
Context Managers
Context managers ensure resources are properly managed.
with open("example.txt", "w") as file:
file.write("Hello, World!")
You can create your own context managers using the contextlib
module.
from contextlib import contextmanager
@contextmanager
def my_context():
print("Entering the context")
yield
print("Exiting the context")
with my_context():
print("Inside the context")
Congratulations! You’ve taken a whirlwind tour through Pythonic problem solving, covering everything from basic syntax to advanced techniques. Remember, the key to becoming a Python hero is practice and curiosity. Keep experimenting, keep learning, and most importantly, keep coding. Python is a language that grows with you, and its community is one of the most welcoming you’ll find. So go forth and conquer those coding challenges, one Pythonic solution at a time. Happy coding!