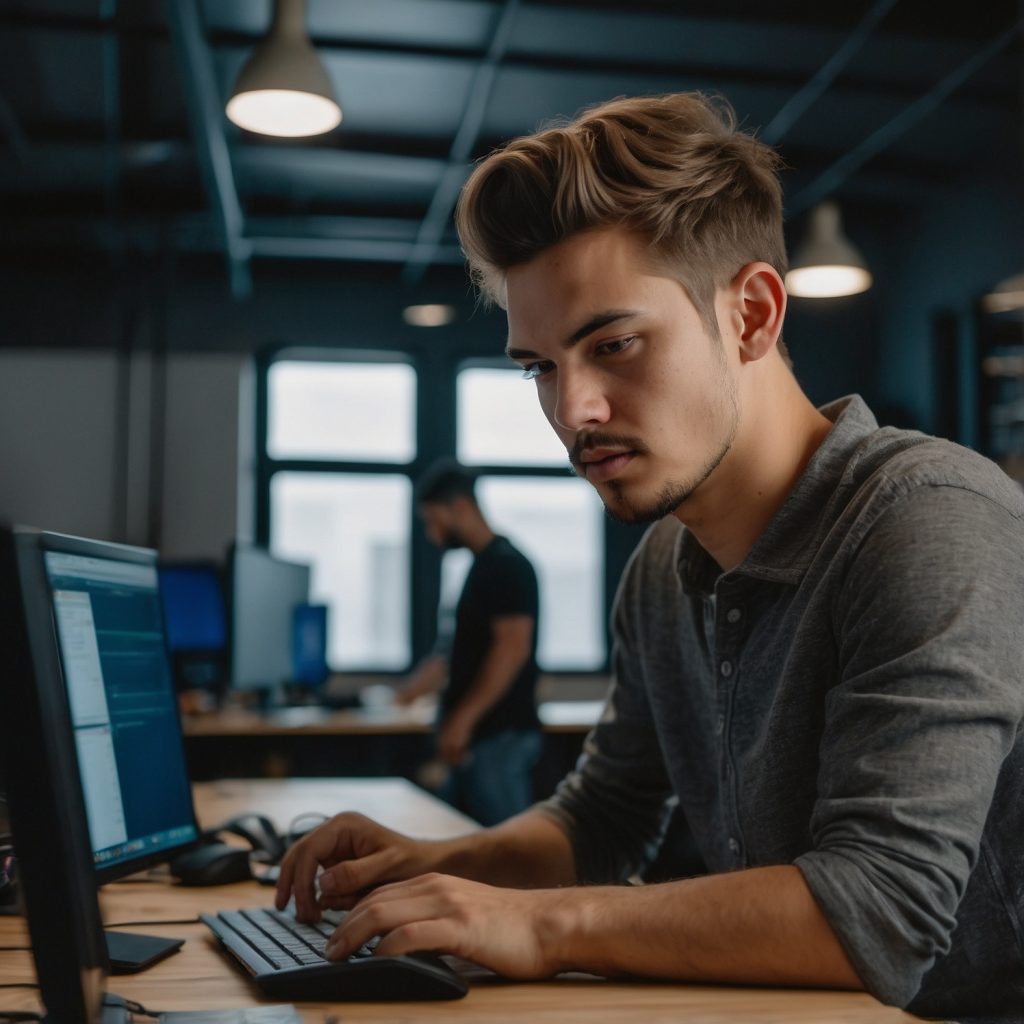
Getting Started with Python
Python has rapidly become one of the most popular programming languages due to its simplicity and versatility. Whether you’re a complete beginner or an experienced developer looking to add Python to your skill set, this guide will help you get started. We’ll cover the basics of Python, provide sample codes, and ensure you have a solid foundation to build upon.
Why Learn Python?
Ease of Learning: Python’s syntax is straightforward and easy to read, making it an excellent choice for beginners. Unlike other programming languages that require complex syntax, Python is more like writing in plain English.
Versatility: Python can be used for a wide range of applications, including web development, data analysis, artificial intelligence, scientific computing, and automation. This versatility makes it a valuable skill in various industries.
Community Support: Python has a large and active community. This means you can find a wealth of tutorials, forums, and documentation to help you overcome any challenges you might face.
Career Opportunities: Python developers are in high demand. Learning Python can open up numerous job opportunities and can significantly boost your career prospects.
Setting Up Python
Download and Install Python: The first step to getting started with Python is to download and install it on your computer. You can download the latest version of Python from the official website. Follow the installation instructions specific to your operating system.
Verify Installation: After installing Python, open your command prompt (Windows) or terminal (Mac/Linux) and type python --version
. You should see the version number of Python that you installed, indicating that Python is correctly installed.
Integrated Development Environment (IDE): An IDE can make coding in Python more efficient. Some popular IDEs for Python include PyCharm, VS Code, and Jupyter Notebook. Download and install an IDE of your choice.
Your First Python Program
Let’s start with a simple Python program. Open your IDE and create a new Python file (e.g., hello.py
). In this file, type the following code:
print("Hello, World!")
Save the file and run it. You should see the output:
Hello, World!
Congratulations! You’ve just written and executed your first Python program.
Python Basics
Variables and Data Types: In Python, you don’t need to declare the type of a variable. You can simply assign a value to a variable, and Python will handle the rest.
# Integer
age = 25
# Float
height = 5.9
# String
name = "Alice"
# Boolean
is_student = True
print(age, height, name, is_student)
Basic Operators: Python supports various operators for arithmetic, comparison, and logical operations.
# Arithmetic Operators
x = 10
y = 5
print(x + y) # Addition
print(x - y) # Subtraction
print(x * y) # Multiplication
print(x / y) # Division
# Comparison Operators
print(x == y) # Equal to
print(x != y) # Not equal to
print(x > y) # Greater than
print(x < y) # Less than
# Logical Operators
a = True
b = False
print(a and b) # Logical AND
print(a or b) # Logical OR
print(not a) # Logical NOT
Control Structures
Conditional Statements: Python uses if
, elif
, and else
statements to make decisions.
age = 18
if age < 18:
print("You are a minor.")
elif age == 18:
print("You are exactly 18 years old.")
else:
print("You are an adult.")
Loops: Python supports for
and while
loops to iterate over sequences.
# For Loop
for i in range(5):
print(i)
# While Loop
count = 0
while count < 5:
print(count)
count += 1
Functions
Functions allow you to organize your code into reusable blocks. You can define a function using the def
keyword.
def greet(name):
print(f"Hello, {name}!")
greet("Alice")
greet("Bob")
You can also define functions with default parameters and return values.
def add(a, b=10):
return a + b
result = add(5)
print(result) # Outputs 15
result = add(5, 15)
print(result) # Outputs 20
Working with Lists
Lists are one of the most commonly used data structures in Python. They are mutable, meaning you can change their content without changing their identity.
# Creating a List
fruits = ["apple", "banana", "cherry"]
# Accessing List Items
print(fruits[0]) # Outputs "apple"
# Changing List Items
fruits[1] = "blueberry"
print(fruits) # Outputs ["apple", "blueberry", "cherry"]
# Looping Through a List
for fruit in fruits:
print(fruit)
# List Methods
fruits.append("orange")
print(fruits) # Outputs ["apple", "blueberry", "cherry", "orange"]
fruits.remove("blueberry")
print(fruits) # Outputs ["apple", "cherry", "orange"]
Dictionaries
Dictionaries store data in key-value pairs. They are mutable and unordered, meaning the items have no defined order.
# Creating a Dictionary
person = {
"name": "Alice",
"age": 25,
"city": "New York"
}
# Accessing Dictionary Items
print(person["name"]) # Outputs "Alice"
# Changing Dictionary Items
person["age"] = 26
print(person) # Outputs {"name": "Alice", "age": 26, "city": "New York"}
# Looping Through a Dictionary
for key, value in person.items():
print(key, value)
# Dictionary Methods
person["email"] = "alice@example.com"
print(person) # Outputs {"name": "Alice", "age": 26, "city": "New York", "email": "alice@example.com"}
del person["city"]
print(person) # Outputs {"name": "Alice", "age": 26, "email": "alice@example.com"}
File Handling
Python makes it easy to work with files. You can open, read, write, and close files using built-in functions.
# Writing to a File
with open("example.txt", "w") as file:
file.write("Hello, World!")
# Reading from a File
with open("example.txt", "r") as file:
content = file.read()
print(content)
Error Handling
Handling errors gracefully is essential in programming. Python uses try
, except
, and finally
blocks to handle exceptions.
try:
x = 10 / 0
except ZeroDivisionError:
print("Cannot divide by zero!")
finally:
print("This will always execute.")
Modules and Packages
Modules and packages allow you to organize your code into manageable sections. A module is a Python file that can contain functions, classes, and variables. A package is a collection of modules.
Creating a Module:
Create a file named mymodule.py
and add the following code:
def greet(name):
return f"Hello, {name}!"
You can then import and use this module in another Python file:
import mymodule
print(mymodule.greet("Alice"))
Installing Packages:
You can install additional packages using pip
, Python’s package installer. For example, to install the requests
package, open your terminal and type:
pip install requests
Then you can use the package in your code:
import requests
response = requests.get("https://api.github.com")
print(response.json())
Conclusion
Getting started with Python is an exciting journey filled with opportunities to learn and grow. By mastering the basics outlined in this guide, you will be well on your way to becoming proficient in Python. Remember to practice regularly, explore different libraries, and take on small projects to apply what you’ve learned.
Python’s simplicity and power make it a great choice for both beginners and experienced developers. Whether you’re interested in web development, data science, artificial intelligence, or automation, Python has the tools and libraries to support your goals. Happy coding!