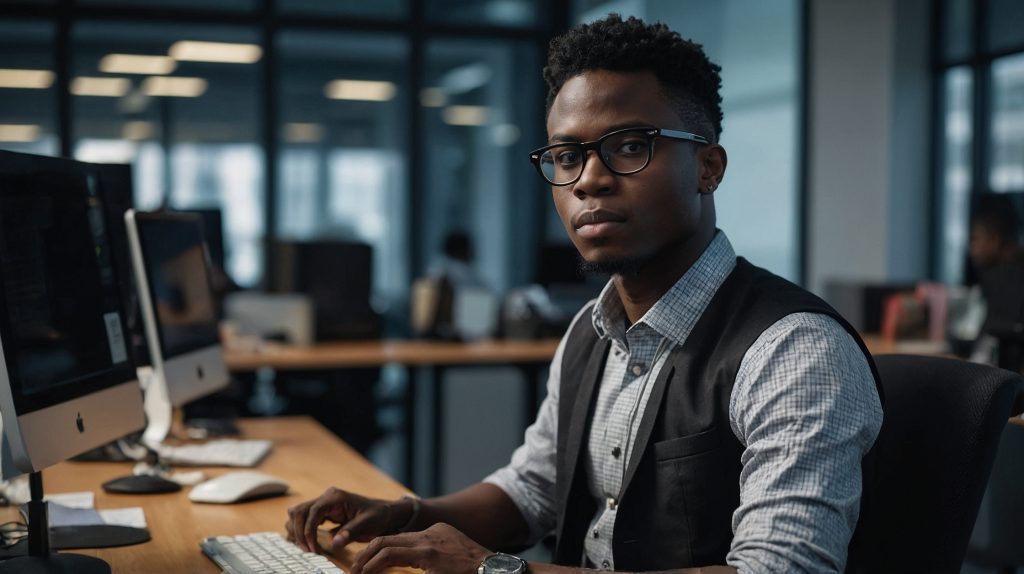
Git Branching Strategies for Effective CI/CD Pipelines
Hey there, fellow code wranglers! Ready to dive into the wild world of Git branching strategies? Buckle up, because we’re about to embark on an epic journey that’ll transform your CI/CD pipeline from a rickety wagon trail into a high-speed hyperloop. Whether you’re a seasoned developer or just dipping your toes into the version control waters, this guide will help you navigate the twists and turns of Git branching like a pro. So, grab your favorite caffeinated beverage, and let’s get branching!
The Branching Basics: Why All the Fuss?
Before we dive into the nitty-gritty of branching strategies, let’s take a step back and ask ourselves: why do we even need branches? Well, imagine you’re working on a massive project with a team of developers. Without branches, you’d all be stumbling over each other, trying to push changes to the same codebase. It’d be like trying to paint a masterpiece with ten people holding the same brush – chaotic, messy, and probably ending in tears (or at least some very frustrated developers).
Branching gives us the power to work on different features, bug fixes, and experiments simultaneously without stepping on each other’s toes. It’s like giving each developer their own sandbox to play in, where they can build castles (or write code) to their heart’s content without worrying about knocking over someone else’s creation. But with great power comes great responsibility, and that’s where branching strategies come into play.
A solid branching strategy is the secret sauce that turns your development process from a chaotic free-for-all into a well-oiled machine. It provides a structure for managing code changes, streamlines your workflow, and makes integrating new features smoother than a freshly waxed snowboard. When combined with continuous integration and continuous deployment (CI/CD), the right branching strategy can supercharge your development process, leading to faster releases, fewer conflicts, and happier developers (and managers!).
The Git Flow: The Granddaddy of Branching Strategies
Let’s kick things off with Git Flow, the branching strategy that started it all. Developed by Vincent Driessen back in 2010, Git Flow has been the go-to strategy for many development teams for years. It’s like the Swiss Army knife of branching strategies – robust, versatile, and with a tool for every situation.
The Main Branches
Git Flow revolves around two main branches:
master
(ormain
): This is where your production-ready code lives. It’s the branch that gets deployed to your live environment and should always be stable.develop
: This is the integration branch where features are combined and tested before making their way tomaster
.
Feature Branches
When you’re working on a new feature, you create a feature
branch off of develop
. These branches are where the magic happens – where new functionality is built and tested in isolation.
git checkout develop
git checkout -b feature/awesome-new-thing
Once your feature is complete and tested, it gets merged back into develop
:
git checkout develop
git merge --no-ff feature/awesome-new-thing
git push origin develop
Release Branches
When develop
has enough features for a release, you create a release
branch. This is where you do final tweaks, version bumps, and last-minute bug fixes.
git checkout develop
git checkout -b release/1.0.0
Once the release is ready, it gets merged into both master
and develop
:
git checkout master
git merge --no-ff release/1.0.0
git tag -a 1.0.0
git push origin master --tags
git checkout develop
git merge --no-ff release/1.0.0
git push origin develop
Hotfix Branches
For those critical bugs that need immediate attention in production, Git Flow uses hotfix
branches. These are created from master
, fixed, and then merged back into both master
and develop
.
git checkout master
git checkout -b hotfix/critical-bug-fix
# After fixing the bug
git checkout master
git merge --no-ff hotfix/critical-bug-fix
git tag -a 1.0.1
git push origin master --tags
git checkout develop
git merge --no-ff hotfix/critical-bug-fix
git push origin develop
Git Flow is comprehensive and provides a clear structure for managing releases. However, it can be a bit heavy for projects with frequent deployments or smaller teams. It’s like driving a tank to the grocery store – it’ll get you there, but it might be overkill for your needs.
GitHub Flow: Simplicity is the Ultimate Sophistication
If Git Flow feels like too much overhead for your project, GitHub Flow might be just what the doctor ordered. Developed by the GitHub team, this strategy is all about keeping things simple and streamlined.
The Main Branch
In GitHub Flow, there’s only one long-running branch: main
(or master
). This branch should always be deployable, containing only production-ready code.
Feature Branches
When you start work on a new feature or bug fix, you create a descriptive branch off of main
:
git checkout main
git checkout -b descriptive-branch-name
Work on your changes, commit often, and push your branch to the remote repository:
git push -u origin descriptive-branch-name
Pull Requests
Once your feature is complete, you open a pull request. This kick-starts the code review process, allowing team members to discuss the changes, suggest improvements, and catch potential issues before they make it to production.
Deployment
After the pull request is approved and all checks pass, the branch is merged into main
and deployed immediately. This keeps the deployment process simple and ensures that main
always reflects what’s in production.
git checkout main
git merge --no-ff descriptive-branch-name
git push origin main
GitHub Flow is perfect for teams practicing continuous delivery or those with simpler deployment needs. It’s like riding a bicycle instead of driving a car – less complex, more agile, and often gets you where you need to go just as fast (if not faster).
Trunk-Based Development: The Speed Demon’s Choice
For teams that want to move at breakneck speed, trunk-based development might be the way to go. This strategy takes the “keep it simple” mantra of GitHub Flow and cranks it up to eleven.
The Trunk
In trunk-based development, all work happens on a single branch, often called trunk
or main
. Developers commit their changes directly to this branch multiple times a day.
Short-Lived Feature Branches
If a feature is too big to complete in a day or two, developers create short-lived feature branches. These branches are merged back into the trunk as quickly as possible, usually within a day or two.
git checkout main
git checkout -b quick-feature
# Work on the feature
git checkout main
git merge --no-ff quick-feature
git push origin main
Feature Flags
To manage longer-running features without long-lived branches, trunk-based development often relies heavily on feature flags. These allow incomplete or experimental features to be merged into the trunk but kept hidden from users until they’re ready.
if feature_flags.is_enabled('new_awesome_feature'):
# New feature code
else:
# Old feature code
Continuous Integration
Trunk-based development relies heavily on robust CI practices. Every commit to the trunk triggers a build and a suite of automated tests to catch issues early.
# Example GitHub Actions workflow
name: CI
on:
push:
branches: [ main ]
pull_request:
branches: [ main ]
jobs:
build:
runs-on: ubuntu-latest
steps:
- uses: actions/checkout@v2
- name: Set up Python
uses: actions/setup-python@v2
with:
python-version: 3.8
- name: Install dependencies
run: |
python -m pip install --upgrade pip
pip install -r requirements.txt
- name: Run tests
run: |
python -m unittest discover tests
Trunk-based development is like drag racing – it’s all about speed and quick iterations. It works well for teams with strong testing practices and the ability to quickly fix issues that make it into the trunk.
GitLab Flow: The Best of Both Worlds
GitLab Flow takes inspiration from both Git Flow and GitHub Flow, aiming to strike a balance between simplicity and structure. It’s like the Goldilocks of branching strategies – not too complex, not too simple, but just right for many teams.
The Main Branch
Like GitHub Flow, GitLab Flow has a main
branch that contains production-ready code.
Feature Branches
Developers create feature branches off of main
for new work:
git checkout main
git checkout -b feature/new-awesome-thing
Merge Requests
Similar to GitHub’s pull requests, GitLab uses merge requests for code review and discussion before merging features into main
.
Environment Branches
Where GitLab Flow differs is in its use of environment branches. These branches represent different stages in your deployment pipeline, such as staging
and production
.
git checkout main
git checkout -b staging
git checkout -b production
Changes flow from main
to staging
to production
, allowing for additional testing and verification at each stage:
# After merging a feature into main
git checkout staging
git merge --no-ff main
git push origin staging
# After testing in staging
git checkout production
git merge --no-ff staging
git push origin production
Release Branches
For projects that need to maintain multiple versions, GitLab Flow introduces release branches:
git checkout main
git checkout -b release-1-0
Hotfixes can be applied to these release branches and then cherry-picked back to main
:
git checkout release-1-0
git checkout -b hotfix-critical-bug
# After fixing the bug
git checkout release-1-0
git merge --no-ff hotfix-critical-bug
git push origin release-1-0
git checkout main
git cherry-pick <commit-sha>
git push origin main
GitLab Flow provides a nice middle ground for teams that want more structure than GitHub Flow but less complexity than Git Flow. It’s particularly well-suited for teams practicing continuous delivery with multiple environments.
Choosing the Right Strategy: One Size Doesn’t Fit All
Now that we’ve explored some popular branching strategies, you might be wondering which one is right for your team. The truth is, there’s no one-size-fits-all solution. Choosing the right branching strategy is like picking out a pair of shoes – what works for one person (or team) might not work for another.
Consider Your Release Cycle
If you’re releasing frequently (daily or weekly), a simpler strategy like GitHub Flow or trunk-based development might be your best bet. These approaches minimize overhead and allow for rapid iterations.
On the other hand, if you have longer release cycles or need to maintain multiple versions of your software, a more structured approach like Git Flow or GitLab Flow could be more appropriate.
Team Size and Structure
Smaller teams or those with a high level of communication might thrive with a simpler strategy. Larger teams or those spread across different time zones might benefit from the additional structure provided by Git Flow or GitLab Flow.
Project Complexity
Simple projects with a single production environment might do well with GitHub Flow. More complex projects with multiple environments or those requiring extensive testing before release might need the additional stages provided by GitLab Flow or Git Flow.
CI/CD Maturity
Your branching strategy should complement your CI/CD practices. If you have a robust CI/CD pipeline with extensive automated testing, you might be able to adopt a more streamlined approach like trunk-based development. If you’re still building out your automation, a strategy with more manual gates (like Git Flow) might be safer.
Implementing Your Chosen Strategy
Once you’ve chosen a branching strategy, implementing it effectively is key to reaping its benefits. Here are some tips to help you make the most of your chosen approach:
Document and Communicate
Whatever strategy you choose, make sure it’s well-documented and communicated to your entire team. Create a clear guide that outlines:
- The overall structure of your branches
- Naming conventions for branches
- Procedures for creating, merging, and deleting branches
- How to handle conflicts and emergencies
Consider creating a visual diagram of your branching strategy to make it easier for team members to understand and remember.
Automate Where Possible
Use tools and scripts to automate as much of your branching process as possible. This could include:
- Creating branches with standardized names
- Running tests and linters before allowing merges
- Automatically deploying to different environments based on branch names
Here’s an example of a Git hook that enforces branch naming conventions:
#!/bin/bash
branch_name=$(git rev-parse --abbrev-ref HEAD)
valid_branch_regex="^(feature|bugfix|improvement|library|prerelease|release|hotfix)\/[a-z0-9._-]+$"
if [[ ! $branch_name =~ $valid_branch_regex ]]
then
echo "There is something wrong with your branch name. Branch names in this project must adhere to this contract: $valid_branch_regex. Your commit will be rejected. You should rename your branch to a valid name and try again."
exit 1
fi
exit 0
Enforce Code Review
Regardless of your chosen strategy, code review is crucial for maintaining code quality and sharing knowledge across the team. Set up your repository to require pull/merge requests and approvals before merging into key branches.
Monitor and Adjust
Keep an eye on how your chosen strategy is working in practice. Are there bottlenecks in your process? Are team members struggling with certain aspects? Be prepared to make adjustments as needed.
Invest in Training
Make sure your team is comfortable with Git and your chosen branching strategy. Consider holding training sessions or pairing less experienced team members with Git experts.
Branching Out
As you become more comfortable with your chosen branching strategy, you might want to explore some advanced techniques and tools to further optimize your workflow:
Git Aliases
Create Git aliases to streamline common branching operations. For example:
git config --global alias.new-feature '!git checkout -b feature/$1 develop'
Now you can create a new feature branch with:
git new-feature awesome-new-thing
Git Worktrees
For complex projects, Git worktrees allow you to check out multiple branches simultaneously in separate directories. This can be incredibly useful for managing long-running feature branches or maintaining multiple versions of your software.
git worktree add ../project-feature feature/big-new-thing
Branch Protection Rules
Use your Git hosting platform’s branch protection rules to enforce your branching strategy. For example, on GitHub:
- Go to your repository settings
- Click on “Branches”
- Add a branch protection rule for your main branch
- Configure options like requiring pull request reviews, status checks, and signed commits
Semantic Versioning
Implement semantic versioning to make your release process more predictable and manageable. This pairs particularly well with Git Flow’s release branches.
# Creating a release branch
git checkout develop
git checkout -b release/1.2.0
# Bumping version and tagging
npm version minor
git push origin release/1.2.0 --tags
Automated Release Notes
Use tools like conventional-changelog
to automatically generate release notes based on your commit messages. This encourages good commit practices and makes it easier to track what’s changing between versions.
npm install -g conventional-changelog-cli
conventional-changelog -p angular -i CHANGELOG.md -s
Final Thoughts
Whew! We’ve covered a lot of ground, from the basics of branching to advanced techniques for supercharging your Git workflow. Remember, the perfect branching strategy is the one that works best for your team and project. Don’t be afraid to experiment, adapt, and even mix elements from different strategies to create a custom approach that fits your needs like a glove.
As you implement your chosen strategy, keep in mind that the goal is to make your development process smoother, not to create unnecessary bureaucracy. If you find yourself spending more time managing branches than writing code, it might be time to reassess and simplify.
With the right branching strategy in place, your CI/CD pipeline will be humming along like a well-oiled machine, delivering high-quality code to production faster than ever before. You’ll be shipping features left and right, squashing bugs with ease, and making your project stakeholders wonder if you’ve somehow tapped into some sort of coding superpowers.
But remember, even the best branching strategy is only as good as the team implementing it. Foster a culture of collaboration, continuous learning, and relentless improvement. Encourage your team to share their Git tricks and tips, celebrate successful merges (merge party, anyone?), and don’t be afraid to have a post-mortem when things go awry. After all, in the world of software development, every merge conflict is just another opportunity to learn and grow.
So go forth, brave code wranglers, and may your branches be ever green, your merges conflict-free, and your deployments smooth as silk. Happy branching!
Bonus Tip: Git Hooks for the Win
Before we wrap up, let’s talk about one more powerful tool in your Git arsenal: hooks. Git hooks are scripts that run automatically every time a particular event occurs in a Git repository. They’re like little coding ninjas, silently enforcing your team’s best practices and catching potential issues before they snowball into major problems.
Here’s a quick example of a pre-commit hook that runs your tests before allowing a commit:
#!/bin/sh
# Run tests
npm test
# $? stores exit value of the last command
if [ $? -ne 0 ]; then
echo "Tests must pass before commit!"
exit 1
fi
Save this as .git/hooks/pre-commit
(don’t forget to make it executable with chmod +x
), and voilà! Now your tests will run automatically every time you try to make a commit. If the tests fail, the commit is aborted, saving you from the embarrassment of pushing broken code.
You can set up hooks for all sorts of things:
- Checking code style
- Updating version numbers
- Generating documentation
- Sending notifications
The possibilities are endless! Just remember, with great power comes great responsibility. Use hooks wisely to enhance your workflow, not to create unnecessary bottlenecks.
And there you have it, folks – a comprehensive guide to Git branching strategies for supercharged CI/CD pipelines. May your repositories be forever organized, your merges always smooth, and your deployments lightning-fast. Now go forth and branch with confidence!
Disclaimer: This blog post is intended for educational purposes only. While we strive for accuracy, Git and CI/CD best practices may evolve over time. Always refer to the official documentation and consult with your team before implementing new strategies. If you notice any inaccuracies in this post, please report them so we can correct them promptly. Happy coding!