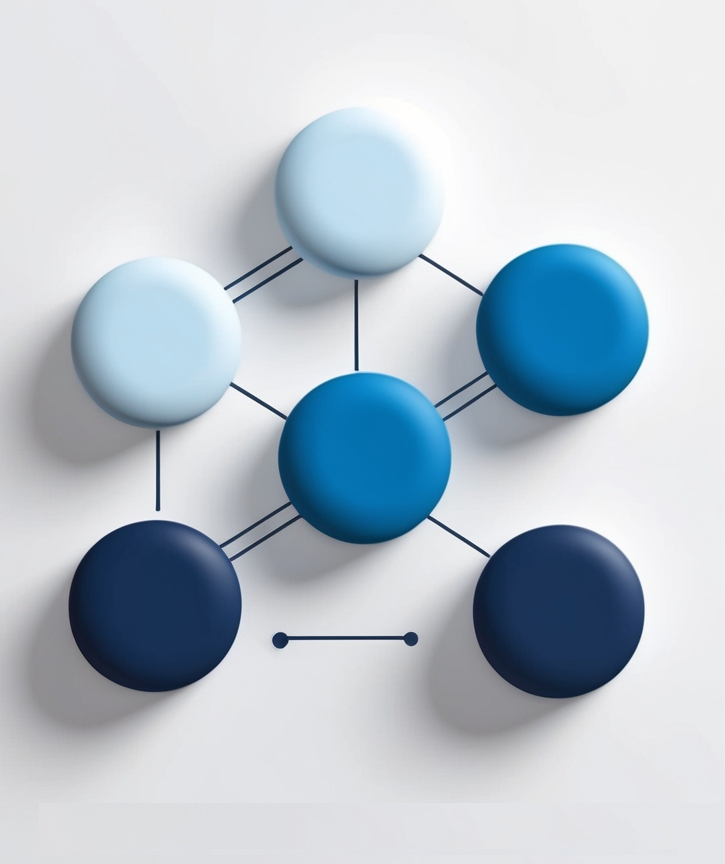
Grouping Data with GROUP BY in SQL
In the realm of data analysis and database management, the ability to efficiently organize and summarize information is paramount. SQL (Structured Query Language) provides a powerful tool for this purpose: the GROUP BY clause. This feature allows analysts and database administrators to group rows that have the same values in specified columns, enabling the performance of aggregate functions on each group rather than on the entire dataset. This blog post will delve into the intricacies of the GROUP BY clause, exploring its syntax, use cases, and advanced applications in SQL.
Understanding the Basics of GROUP BY
The GROUP BY clause is a fundamental component of SQL that works in conjunction with SELECT statements to arrange identical data into groups. It is particularly useful when you need to perform calculations or apply aggregate functions to subsets of your data based on one or more columns.
Syntax of GROUP BY
The basic syntax of a GROUP BY clause is as follows:
SELECT column1, column2, ..., aggregate_function(column)
FROM table_name
GROUP BY column1, column2, ...;
In this structure, the GROUP BY clause comes after the FROM clause and any WHERE conditions, but before ORDER BY. The columns listed in the GROUP BY clause determine how the rows will be grouped.
Purpose and Functionality
The primary purpose of GROUP BY is to collapse multiple rows into a single row for each unique combination of the grouped columns. This grouping allows for the application of aggregate functions like COUNT(), SUM(), AVG(), MAX(), and MIN() to each group independently, rather than to the entire result set.
For example, if you have a sales database and want to know the total sales for each product category, you would use GROUP BY to group the sales data by category and then apply the SUM() function to the sales amount for each group.
When to Use GROUP BY
The GROUP BY clause is particularly useful in scenarios where you need to analyze data in groups or categories. Here are some common use cases:
- Summarizing Data: When you need to calculate totals, averages, or counts for different categories or groups within your data.
- Identifying Patterns: GROUP BY can help in identifying trends or patterns within subsets of your data.
- Reporting: It’s often used in generating reports that require data to be presented in a summarized format by certain criteria.
- Data Analysis: When performing data analysis tasks that involve comparing metrics across different groups or categories.
- Performance Optimization: In some cases, grouping data can lead to more efficient queries, especially when dealing with large datasets.
Basic Examples of GROUP BY in Action
Let’s explore some basic examples to illustrate the power and versatility of the GROUP BY clause. For these examples, we’ll use a hypothetical database of an online bookstore.
Example 1: Counting Books by Genre
Suppose we want to know how many books we have in each genre. Here’s how we could use GROUP BY to achieve this:
SELECT genre, COUNT(*) as book_count
FROM books
GROUP BY genre;
This query will return a result set with two columns: ‘genre’ and ‘book_count’, where each row represents a unique genre and the count of books in that genre.
Example 2: Total Sales by Author
If we want to calculate the total sales for each author, we could use the following query:
SELECT author, SUM(sales_amount) as total_sales
FROM book_sales
GROUP BY author;
This query groups the sales data by author and calculates the sum of sales_amount for each author.
Example 3: Average Rating by Publisher
To find the average rating of books for each publisher:
SELECT publisher, AVG(rating) as avg_rating
FROM books
GROUP BY publisher;
This query groups the books by publisher and calculates the average rating for each publisher’s books.
Advanced GROUP BY Techniques
While the basic usage of GROUP BY is straightforward, there are several advanced techniques that can enhance its power and flexibility.
Using Multiple Columns in GROUP BY
You can group data based on multiple columns to create more specific groupings. For example, if you want to analyze book sales by both genre and year:
SELECT genre, publication_year, SUM(sales_amount) as total_sales
FROM books
JOIN book_sales ON books.book_id = book_sales.book_id
GROUP BY genre, publication_year;
This query will group the sales data first by genre, and then by publication year within each genre.
GROUP BY with HAVING Clause
The HAVING clause is used with GROUP BY to filter groups based on a specified condition. It’s similar to the WHERE clause, but it operates on groups rather than individual rows. For instance, if we want to find genres with more than 100 books:
SELECT genre, COUNT(*) as book_count
FROM books
GROUP BY genre
HAVING COUNT(*) > 100;
This query will only return genres that have more than 100 books.
Using GROUP BY with Subqueries
Subqueries can be powerful when used in conjunction with GROUP BY. For example, to find authors whose average book rating is higher than the overall average:
SELECT author, AVG(rating) as avg_rating
FROM books
GROUP BY author
HAVING AVG(rating) > (SELECT AVG(rating) FROM books);
This query calculates the average rating for each author and compares it to the overall average rating across all books.
Common Pitfalls and Best Practices
While GROUP BY is a powerful tool, there are some common pitfalls to avoid and best practices to follow:
Pitfall 1: Selecting Non-Aggregated Columns
One common mistake is selecting columns in the SELECT clause that are not included in the GROUP BY clause and are not part of an aggregate function. This can lead to unexpected results or errors in some database systems.
Incorrect:
SELECT author, title, AVG(rating)
FROM books
GROUP BY author;
Correct:
SELECT author, AVG(rating)
FROM books
GROUP BY author;
Best Practice: Always include all non-aggregated columns in the GROUP BY clause.
Pitfall 2: Misusing WHERE and HAVING
Remember that WHERE filters rows before grouping, while HAVING filters after grouping. Using them incorrectly can lead to unexpected results.
Incorrect (if you want to filter groups):
SELECT genre, AVG(rating)
FROM books
WHERE AVG(rating) > 4
GROUP BY genre;
Correct:
SELECT genre, AVG(rating)
FROM books
GROUP BY genre
HAVING AVG(rating) > 4;
Best Practice: Use WHERE for row-level filtering and HAVING for group-level filtering.
Pitfall 3: Ignoring NULL Values
GROUP BY treats NULL as a group, which can sometimes lead to unexpected results. Be aware of how your database system handles NULLs in GROUP BY operations.
Best Practice: Consider using COALESCE() or IFNULL() to handle NULL values if necessary.
Performance Considerations
When working with large datasets, the performance of GROUP BY operations can become a concern. Here are some tips to optimize GROUP BY queries:
- Indexing: Ensure that columns used in GROUP BY clauses are properly indexed. This can significantly speed up grouping operations.
- Limit the Number of Groups: If possible, try to limit the number of groups created. Too many groups can lead to performance issues.
- Pre-aggregation: For very large datasets, consider pre-aggregating data in separate tables or materialized views.
- Use EXPLAIN: Utilize the EXPLAIN command to analyze your query execution plan and identify potential bottlenecks.
- Consider Alternative Approaches: In some cases, window functions or subqueries might be more efficient than GROUP BY for certain types of analysis.
Real-World Applications of GROUP BY
The GROUP BY clause finds applications across various industries and use cases. Let’s explore some real-world scenarios where GROUP BY proves invaluable:
Sales Analysis in Retail
In the retail sector, GROUP BY is extensively used for sales analysis. For instance, a query to analyze sales performance by product category and region might look like this:
SELECT product_category, region, SUM(sales_amount) as total_sales,
AVG(sales_amount) as avg_sale, COUNT(*) as transaction_count
FROM sales_transactions
JOIN products ON sales_transactions.product_id = products.product_id
JOIN stores ON sales_transactions.store_id = stores.store_id
GROUP BY product_category, region;
This query provides a comprehensive view of sales performance, allowing retailers to identify top-performing categories in different regions.
Financial Reporting
In the finance industry, GROUP BY is crucial for generating various reports. For example, to analyze expenses by department and month:
SELECT department, EXTRACT(MONTH FROM transaction_date) as month,
SUM(amount) as total_expense
FROM expenses
GROUP BY department, EXTRACT(MONTH FROM transaction_date)
ORDER BY department, month;
This query helps in budgeting and identifying departments with unusually high expenses in specific months.
Web Analytics
For websites and applications, GROUP BY is used to analyze user behavior. To find the most active hours on a website:
SELECT EXTRACT(HOUR FROM access_time) as hour,
COUNT(*) as visit_count
FROM web_logs
GROUP BY EXTRACT(HOUR FROM access_time)
ORDER BY visit_count DESC;
This information can be used to optimize server resources or schedule content updates.
Healthcare Data Analysis
In healthcare, GROUP BY can be used to analyze patient data. For instance, to study the prevalence of different diagnoses by age group:
SELECT age_group, diagnosis, COUNT(*) as case_count
FROM patient_records
GROUP BY age_group, diagnosis
ORDER BY age_group, case_count DESC;
This type of analysis can help in resource allocation and targeted healthcare interventions.
Advanced GROUP BY Techniques with Window Functions
Window functions provide a powerful way to perform calculations across a set of rows that are related to the current row. When combined with GROUP BY, they offer even more analytical capabilities.
Running Totals
To calculate running totals within groups:
SELECT
category,
product,
sales_amount,
SUM(sales_amount) OVER (PARTITION BY category ORDER BY sales_amount) as running_total
FROM sales
GROUP BY category, product, sales_amount;
This query calculates a running total of sales within each category.
Ranking within Groups
To rank products within their categories based on sales:
SELECT
category,
product,
sales_amount,
RANK() OVER (PARTITION BY category ORDER BY sales_amount DESC) as rank_in_category
FROM sales
GROUP BY category, product, sales_amount;
This query assigns a rank to each product within its category based on sales amount.
GROUP BY in Different SQL Dialects
While the core functionality of GROUP BY is consistent across different SQL dialects, there are some variations and extensions worth noting:
MySQL
MySQL allows the use of aliases in the GROUP BY clause:
SELECT YEAR(order_date) as order_year, SUM(total_amount) as yearly_total
FROM orders
GROUP BY order_year;
PostgreSQL
PostgreSQL supports GROUPING SETS, which allow you to specify multiple grouping sets in a single query:
SELECT category, product, SUM(sales_amount)
FROM sales
GROUP BY GROUPING SETS ((category, product), (category), ());
This query generates results for different levels of grouping in a single output.
SQL Server
SQL Server introduces the OVER clause, which can be used with GROUP BY for more complex aggregations:
SELECT
category,
product,
sales_amount,
SUM(sales_amount) OVER (PARTITION BY category) as category_total
FROM sales
GROUP BY category, product, sales_amount;
This query calculates both individual product sales and total sales for each category.
Challenges and Limitations of GROUP BY
While GROUP BY is a powerful tool, it’s important to be aware of its limitations and challenges:
- Memory Usage: GROUP BY operations can be memory-intensive, especially with large datasets or many groups.
- Performance with Large Datasets: As the size of the dataset grows, GROUP BY operations can become slower.
- Complexity with Multiple Groupings: Queries with multiple levels of grouping can become complex and difficult to maintain.
- Null Handling: The treatment of NULL values in GROUP BY operations can sometimes lead to unexpected results.
- Limitations in Analytical Capabilities: While powerful, GROUP BY may not be sufficient for very complex analytical tasks, where more advanced techniques like OLAP cubes might be necessary.
Future Trends in Data Grouping and Analysis
As data analysis continues to evolve, we can expect to see advancements in how databases handle grouping and aggregation:
- In-Memory Processing: More databases are leveraging in-memory processing to speed up GROUP BY operations on large datasets.
- Machine Learning Integration: We may see tighter integration between SQL grouping operations and machine learning algorithms for more advanced analytics.
- Real-Time Grouping: As real-time analytics become more important, databases may offer new ways to perform grouping operations on streaming data.
- Enhanced Visualization: Improved integration between SQL engines and visualization tools may make it easier to explore grouped data visually.
Conclusion
The GROUP BY clause in SQL is a fundamental tool for data analysis and reporting. Its ability to aggregate data based on specified criteria makes it invaluable in various fields, from business intelligence to scientific research. By mastering GROUP BY along with its associated concepts like HAVING clauses and window functions, data professionals can unlock powerful insights from their datasets.
As we’ve explored in this blog post, GROUP BY offers a wide range of capabilities, from basic aggregations to complex analytical queries. However, it’s crucial to understand its proper usage, potential pitfalls, and performance implications to use it effectively.
Whether you’re a database administrator, data analyst, or software developer, a deep understanding of GROUP BY will enhance your ability to extract meaningful information from data. As data continues to grow in volume and importance, the skills to efficiently group, aggregate, and analyze this data will remain crucial in the world of data management and analysis.
Disclaimer: While every effort has been made to ensure the accuracy and reliability of the information presented in this blog post, it should be used for educational purposes only. Actual implementation may vary depending on the specific database system and version being used. Always refer to your database system’s official documentation for the most up-to-date and accurate information. If you notice any inaccuracies in this post, please report them so we can correct them promptly.