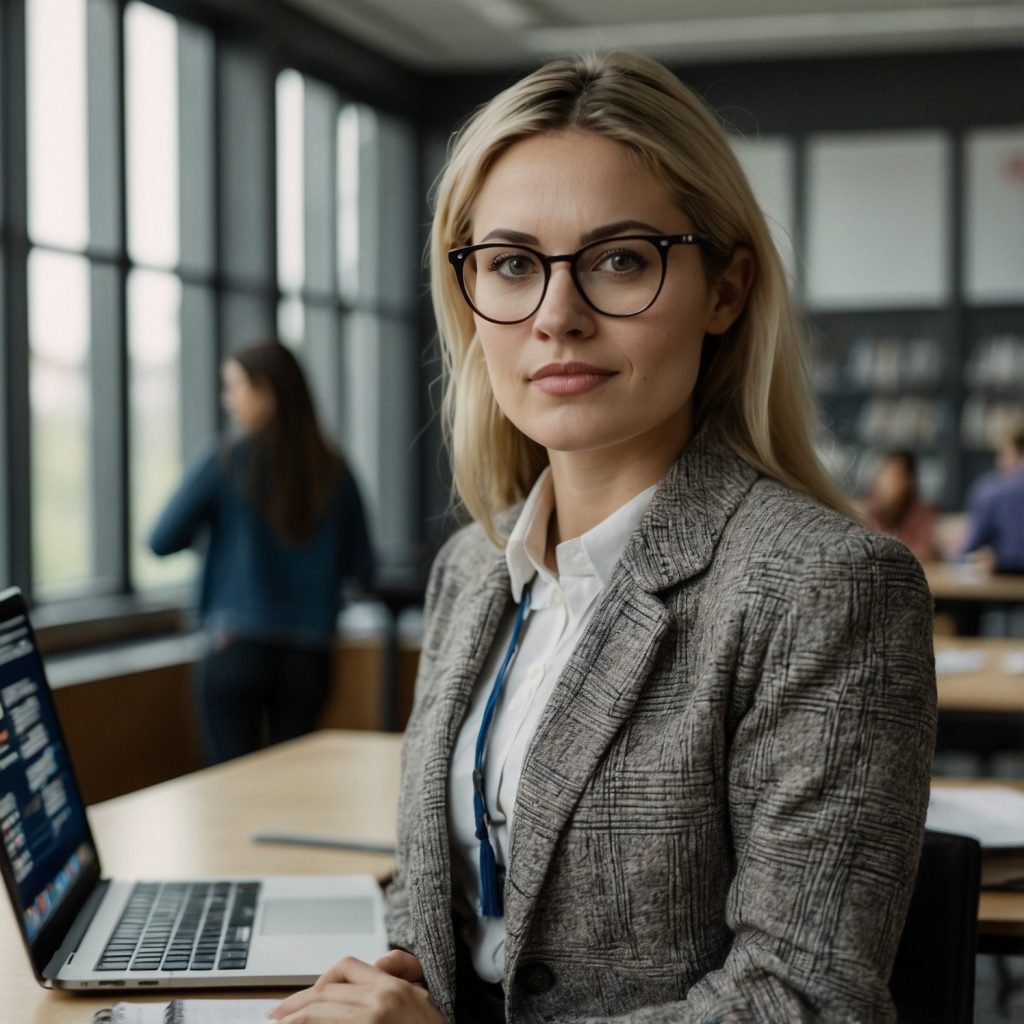
How to Build a RESTful API
Building a RESTful API is a crucial skill for modern web development, allowing different systems to communicate seamlessly over the internet. In this blog, we will explore the process of creating a RESTful API, breaking down each step to ensure clarity and comprehension. By the end, you’ll have a solid understanding of how to build your own RESTful API from scratch.
What is a RESTful API?
Definition and Principles
A RESTful API, or Representational State Transfer API, adheres to a set of architectural principles for designing networked applications. It relies on stateless communication, meaning each request from a client contains all the information needed to process it. RESTful APIs use standard HTTP methods, such as GET, POST, PUT, DELETE, and PATCH, which correspond to CRUD (Create, Read, Update, Delete) operations in database systems.
Advantages of RESTful APIs
One of the primary advantages of RESTful APIs is their simplicity and ease of use. They leverage the existing HTTP infrastructure, making them lightweight and fast. Additionally, RESTful APIs are scalable and can handle multiple types of calls, return different data formats, and allow for easy integration with various systems.
Setting Up Your Development Environment
Choosing the Right Tools
To build a RESTful API, you’ll need a set of development tools. For this example, we will use Node.js for our server-side environment, Express.js as our framework, and MongoDB as our database. These technologies are widely used and provide robust features for building scalable APIs.
Installing Node.js and Express.js
First, ensure you have Node.js installed on your system. You can download it from the official website. Once installed, you can set up your project directory and initialize a new Node.js project:
mkdir restful-api
cd restful-api
npm init -y
Next, install Express.js and other necessary dependencies:
npm install express mongoose body-parser
Designing the API
Defining Endpoints and Methods
The first step in designing your API is to define the endpoints and methods you will use. An endpoint is a specific URL where the API responds to requests. Each endpoint corresponds to a particular function or resource within your application. For example, if you are building a simple blog API, you might have endpoints for posts, comments, and users.
Example Endpoints for a Blog API
GET /posts
– Retrieve all postsGET /posts/:id
– Retrieve a specific post by IDPOST /posts
– Create a new postPUT /posts/:id
– Update an existing post by IDDELETE /posts/:id
– Delete a post by ID
Creating a Router in Express.js
To handle these endpoints, we will create a router in Express.js. Create a new file called routes.js
and define the routes as follows:
const express = require('express');
const router = express.Router();
// Mock data for demonstration
let posts = [
{ id: 1, title: 'First Post', content: 'This is the first post' },
{ id: 2, title: 'Second Post', content: 'This is the second post' },
];
// Get all posts
router.get('/posts', (req, res) => {
res.json(posts);
});
// Get a specific post by ID
router.get('/posts/:id', (req, res) => {
const post = posts.find(p => p.id == req.params.id);
if (post) {
res.json(post);
} else {
res.status(404).send('Post not found');
}
});
// Create a new post
router.post('/posts', (req, res) => {
const newPost = {
id: posts.length + 1,
title: req.body.title,
content: req.body.content,
};
posts.push(newPost);
res.status(201).json(newPost);
});
// Update an existing post by ID
router.put('/posts/:id', (req, res) => {
const post = posts.find(p => p.id == req.params.id);
if (post) {
post.title = req.body.title;
post.content = req.body.content;
res.json(post);
} else {
res.status(404).send('Post not found');
}
});
// Delete a post by ID
router.delete('/posts/:id', (req, res) => {
posts = posts.filter(p => p.id != req.params.id);
res.status(204).send();
});
module.exports = router;
Implementing the Server
Setting Up the Express.js Server
Now that we have defined our routes, let’s set up the Express.js server. Create a new file called server.js
and configure the server to use the routes we created:
const express = require('express');
const bodyParser = require('body-parser');
const routes = require('./routes');
const app = express();
const PORT = process.env.PORT || 3000;
app.use(bodyParser.json());
app.use('/api', routes);
app.listen(PORT, () => {
console.log(`Server is running on port ${PORT}`);
});
With this setup, your server will listen for incoming requests on port 3000 and use the routes defined in routes.js
. You can now start your server by running the following command:
node server.js
Connecting to a Database
Using MongoDB with Mongoose
To store and manage data, we will use MongoDB, a NoSQL database. Mongoose is an Object Data Modeling (ODM) library for MongoDB and Node.js that provides a straightforward, schema-based solution to model your application data.
Installing and Setting Up Mongoose
First, ensure MongoDB is installed on your system. Then, install Mongoose in your project:
npm install mongoose
Next, update server.js
to connect to MongoDB:
const mongoose = require('mongoose');
mongoose.connect('mongodb://localhost:27017/restful-api', {
useNewUrlParser: true,
useUnifiedTopology: true,
});
const db = mongoose.connection;
db.on('error', console.error.bind(console, 'connection error:'));
db.once('open', () => {
console.log('Connected to MongoDB');
});
Creating Models and Schemas
Defining Data Models
In a RESTful API, data models represent the structure of the data we store in the database. For our blog API, we will create a model for posts. Create a new file called models/Post.js
and define the schema:
const mongoose = require('mongoose');
const postSchema = new mongoose.Schema({
title: String,
content: String,
}, {
timestamps: true,
});
module.exports = mongoose.model('Post', postSchema);
Updating Routes to Use Mongoose
Update routes.js
to use the Post model for database operations:
const express = require('express');
const router = express.Router();
const Post = require('./models/Post');
// Get all posts
router.get('/posts', async (req, res) => {
try {
const posts = await Post.find();
res.json(posts);
} catch (error) {
res.status(500).send(error);
}
});
// Get a specific post by ID
router.get('/posts/:id', async (req, res) => {
try {
const post = await Post.findById(req.params.id);
if (post) {
res.json(post);
} else {
res.status(404).send('Post not found');
}
} catch (error) {
res.status(500).send(error);
}
});
// Create a new post
router.post('/posts', async (req, res) => {
const post = new Post({
title: req.body.title,
content: req.body.content,
});
try {
const savedPost = await post.save();
res.status(201).json(savedPost);
} catch (error) {
res.status(400).send(error);
}
});
// Update an existing post by ID
router.put('/posts/:id', async (req, res) => {
try {
const post = await Post.findById(req.params.id);
if (post) {
post.title = req.body.title;
post.content = req.body.content;
const updatedPost = await post.save();
res.json(updatedPost);
} else {
res.status(404).send('Post not found');
}
} catch (error) {
res.status(400).send(error);
}
});
// Delete a post by ID
router.delete('/posts/:id', async (req, res) => {
try {
await Post.findByIdAndDelete(req.params.id);
res.status(204).send();
} catch (error) {
res.status(500).send(error);
}
});
module.exports = router;
Testing Your API
Using Postman for API Testing
To test your API, you can use Postman, a popular tool for testing APIs. Download and install Postman from the official website. Once installed, you can create requests to your API endpoints and verify their responses.
Example Test Cases
- GET /posts – Verify that it returns a list of all posts.
- GET /posts/:id – Verify that it returns the correct post by ID.
- POST /posts – Verify that it creates a new post.
- PUT /posts/:id – Verify that it updates the post with the given ID.
- **DELETE /posts/:id
Securing Your RESTful API
Authentication and Authorization
Securing your API is crucial to protect it from unauthorized access and potential abuse. Authentication verifies the identity of a user, while authorization determines what resources a user can access. One common method for securing APIs is using JSON Web Tokens (JWT).
Installing JWT
First, install the necessary packages:
npm install jsonwebtoken bcryptjs
Implementing JWT Authentication
Update your server.js
file to include the JWT middleware:
const jwt = require('jsonwebtoken');
const bcrypt = require('bcryptjs');
const User = require('./models/User'); // Assume you have a User model
// Middleware to protect routes
function authenticateToken(req, res, next) {
const token = req.headers['authorization'];
if (!token) return res.status(401).send('Access Denied');
try {
const verified = jwt.verify(token, process.env.TOKEN_SECRET);
req.user = verified;
next();
} catch (error) {
res.status(400).send('Invalid Token');
}
}
// Route to register a new user
app.post('/register', async (req, res) => {
const salt = await bcrypt.genSalt(10);
const hashedPassword = await bcrypt.hash(req.body.password, salt);
const user = new User({
username: req.body.username,
password: hashedPassword,
});
try {
const savedUser = await user.save();
res.send({ user: user._id });
} catch (error) {
res.status(400).send(error);
}
});
// Route to login
app.post('/login', async (req, res) => {
const user = await User.findOne({ username: req.body.username });
if (!user) return res.status(400).send('Username or password is wrong');
const validPass = await bcrypt.compare(req.body.password, user.password);
if (!validPass) return res.status(400).send('Invalid password');
const token = jwt.sign({ _id: user._id }, process.env.TOKEN_SECRET);
res.header('auth-token', token).send(token);
});
Protecting Routes
To protect specific routes, you can use the authenticateToken
middleware:
router.post('/posts', authenticateToken, async (req, res) => {
const post = new Post({
title: req.body.title,
content: req.body.content,
});
try {
const savedPost = await post.save();
res.status(201).json(savedPost);
} catch (error) {
res.status(400).send(error);
}
});
Error Handling
Implementing Error Handling
Error handling is a crucial aspect of building robust APIs. It ensures that your API can gracefully handle unexpected situations and provide meaningful error messages to clients.
Adding a Global Error Handler
Update your server.js
to include a global error handler:
app.use((err, req, res, next) => {
console.error(err.stack);
res.status(500).send('Something broke!');
});
Handling Validation Errors
For validation errors, you can use a library like express-validator
to simplify the process. Install it using:
npm install express-validator
Then, update your routes to include validation checks:
const { body, validationResult } = require('express-validator');
router.post('/posts', [
body('title').isLength({ min: 5 }).withMessage('Title must be at least 5 characters long'),
body('content').notEmpty().withMessage('Content cannot be empty')
], async (req, res) => {
const errors = validationResult(req);
if (!errors.isEmpty()) {
return res.status(400).json({ errors: errors.array() });
}
const post = new Post({
title: req.body.title,
content: req.body.content,
});
try {
const savedPost = await post.save();
res.status(201).json(savedPost);
} catch (error) {
res.status(400).send(error);
}
});
Documentation and Testing
Creating API Documentation
Proper documentation is essential for helping developers understand how to use your API. Tools like Swagger can automate the creation of interactive API documentation.
Installing Swagger
First, install the necessary packages:
npm install swagger-ui-express swagger-jsdoc
Setting Up Swagger
Create a new file called swagger.js
:
const swaggerJsDoc = require('swagger-jsdoc');
const swaggerUi = require('swagger-ui-express');
const swaggerOptions = {
swaggerDefinition: {
openapi: '3.0.0',
info: {
title: 'Blog API',
version: '1.0.0',
description: 'API for a simple blog application',
},
},
apis: ['./routes.js'],
};
const swaggerDocs = swaggerJsDoc(swaggerOptions);
module.exports = (app) => {
app.use('/api-docs', swaggerUi.serve, swaggerUi.setup(swaggerDocs));
};
Update server.js
to include Swagger documentation:
const swagger = require('./swagger');
swagger(app);
Performance Optimization
Improving API Performance
Optimizing the performance of your API ensures that it can handle a high number of requests efficiently. Techniques include using caching, optimizing database queries, and implementing rate limiting.
Using Caching
Implement caching to reduce the load on your server and improve response times. Redis is a popular in-memory data structure store that can be used for caching.
Installing Redis
First, install the Redis package:
npm install redis
Implementing Caching with Redis
Update your server.js
to include Redis:
const redis = require('redis');
const client = redis.createClient();
client.on('error', (err) => {
console.log('Redis error: ', err);
});
// Middleware to check cache
function checkCache(req, res, next) {
const { id } = req.params;
client.get(id, (err, data) => {
if (err) throw err;
if (data !== null) {
res.send(JSON.parse(data));
} else {
next();
}
});
}
// Use caching for fetching a specific post
router.get('/posts/:id', checkCache, async (req, res) => {
try {
const post = await Post.findById(req.params.id);
if (post) {
client.setex(req.params.id, 600, JSON.stringify(post)); // Cache for 10 minutes
res.json(post);
} else {
res.status(404).send('Post not found');
}
} catch (error) {
res.status(500).send(error);
}
});
Building a RESTful API involves several steps, from setting up your development environment to designing endpoints, implementing authentication, handling errors, and optimizing performance. By following the principles and best practices outlined in this guide, you can create a robust and scalable API that meets the needs of your application.
In summary, we have covered:
- The basics of RESTful APIs and their advantages
- Setting up a development environment with Node.js, Express.js, and MongoDB
- Designing and implementing API endpoints
- Securing the API with JWT authentication
- Handling errors and validating data
- Documenting the API with Swagger
- Optimizing performance using caching
By mastering these concepts, you’ll be well-equipped to build high-quality RESTful APIs that can serve as the backbone of your web applications. Happy coding!