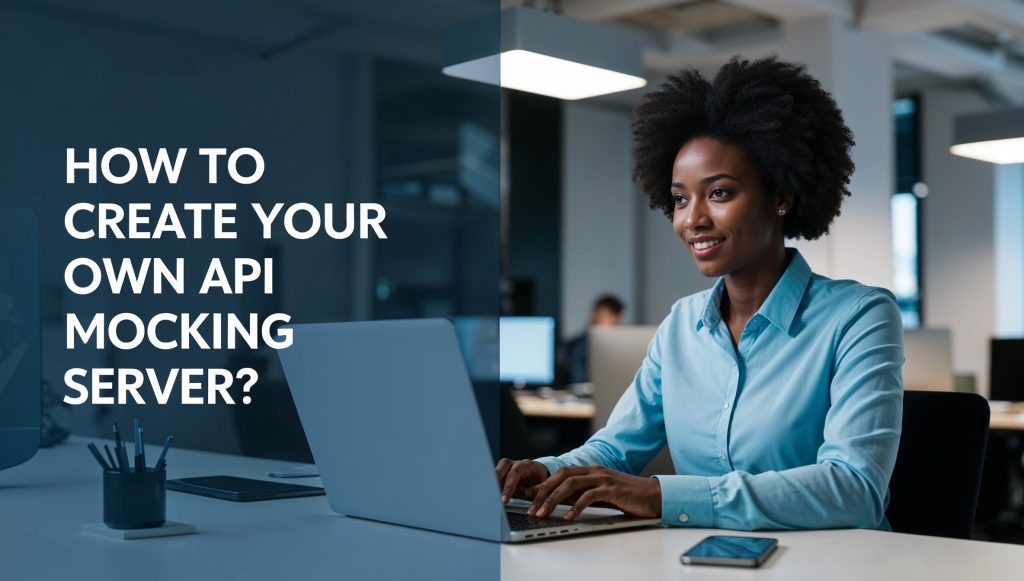
How To Creat Your Own API Mocking Server using WireMock
Are you tired of your frontend development being held hostage by backend delays? Do you find yourself constantly battling with unreliable or rate-limited APIs during testing? Well, my friend, it’s time to break free from these shackles and take control of your development environment with WireMock! In this comprehensive guide, we’ll walk you through everything you need to know about setting up and using WireMock as a standalone application for HTTP mocking. So, grab your favorite code editor, pour yourself a fresh cup of coffee, and let’s dive into the liberating world of mock HTTP services!
What is WireMock and Why Go Standalone?
Before we roll up our sleeves and get our hands dirty with code, let’s take a moment to understand what WireMock is and why running it as a standalone application is such a game-changer. WireMock is a flexible library for stubbing and mocking web services. It creates an HTTP server that we can connect to as we would to an actual web service. When running as a standalone app, it becomes a mock API server that can be shared by multiple services or developers. It’s like having a Swiss Army knife for API mocking – versatile, reliable, and always ready when you need it!
But why should you care about running WireMock as a standalone application? Well, imagine you’re working on a complex frontend application that interacts with multiple backend services. These services might be under development, unreliable, or have usage limits. With standalone WireMock, you can create a mock server that simulates all these backend services. This means you can develop and test your frontend independently of the backend status. It’s like having a stunt double for your backend – always available, never tired, and willing to perform any scenario you throw at it!
Downloading and Setting Up WireMock Standalone
Now that we’ve piqued your interest, let’s roll up our sleeves and get WireMock up and running on your machine. Don’t worry; it’s easier than assembling flat-pack furniture, and we promise you won’t have any leftover pieces at the end!
Step 1: Downloading WireMock
First things first, we need to get our hands on the WireMock standalone JAR file. Follow these steps:
- Navigate to the WireMock releases page on GitHub: https://github.com/wiremock/wiremock/releases
- Look for the latest release (as of this writing, it’s 3.0.0-beta-9)
- Download the file named
wiremock-standalone-3.0.0-beta-9.jar
(or whatever the latest version is)
Congratulations! You’ve just downloaded WireMock. It’s like you’ve just acquired a magical genie in a JAR, ready to grant your API mocking wishes!
Step 2: Setting Up WireMock
Now that we have our WireMock JAR, let’s set it up. The beauty of WireMock standalone is its simplicity. Here’s how to get it running:
- Open your terminal or command prompt
- Navigate to the directory where you downloaded the WireMock JAR
- Run the following command:
java -jar wiremock-standalone-3.0.0-beta-9.jar
That’s it! You should see output similar to this:
/$$ /$$ /$$ /$$ /$$ /$$
| $$ /$ | $$|__/ | $$$ /$$$ | $$
| $$ /$$$| $$ /$$ /$$$$$$ /$$$$$$ | $$$$ /$$$$ /$$$$$$ /$$$$$$$| $$ /$$
| $$/$$ $$ $$| $$ /$$__ $$ /$$__ $$| $$ $$/$$ $$ /$$__ $$ /$$_____/| $$ /$$/
| $$$$_ $$$$| $$| $$ \__/| $$$$$$$$| $$ $$$| $$| $$ \ $$| $$ | $$$$$$/
| $$$/ \ $$$| $$| $$ | $$_____/| $$\ $ | $$| $$ | $$| $$ | $$_ $$
| $$/ \ $$| $$| $$ | $$$$$$$| $$ \/ | $$| $$$$$$/| $$$$$$$| $$ \ $$
|__/ \__/|__/|__/ \_______/|__/ |__/ \______/ \_______/|__/ \__/
port: 8080
enable-browser-proxying: false
disable-banner: false
no-request-journal: false
verbose: false
WireMock is now running on http://localhost:8080
. It’s like you’ve just opened a portal to a parallel universe where all APIs behave exactly as you want them to!
Setting Up Mock Services
Now that our WireMock server is up and running, it’s time to populate it with some mock services. Think of this as stocking your API supermarket with all the responses your frontend might need. Let’s start with some basic examples and then move on to more advanced scenarios.
Creating Your First Mock Service
Let’s create a simple mock service that returns a greeting when we hit a specific endpoint. We’ll use WireMock’s JSON API to set this up. Here’s how:
- Open a new terminal window (keep WireMock running in the first one)
- Use curl to send a POST request to WireMock’s
__admin/mappings
endpoint:
curl -X POST http://localhost:8080/__admin/mappings \
--data '{
"request": {
"method": "GET",
"url": "/hello"
},
"response": {
"status": 200,
"body": "Hello, WireMock!",
"headers": {
"Content-Type": "text/plain"
}
}
}'
Congratulations! You’ve just created your first mock service. It’s like you’re a wizard, conjuring API responses out of thin air!
Creating a JSON Response
Most modern APIs deal with JSON, so let’s create a mock service that returns a JSON response:
curl -X POST http://localhost:8080/__admin/mappings \
--data '{
"request": {
"method": "GET",
"url": "/api/users/1"
},
"response": {
"status": 200,
"jsonBody": {
"id": 1,
"name": "John Doe",
"email": "john@example.com"
},
"headers": {
"Content-Type": "application/json"
}
}
}'
Now we have a mock user API. It’s like having a virtual population ready to respond to your frontend’s every whim!
Simulating Different HTTP Methods
RESTful APIs often use different HTTP methods for different operations. Let’s create a mock service that simulates creating a new user with a POST request:
curl -X POST http://localhost:8080/__admin/mappings \
--data '{
"request": {
"method": "POST",
"url": "/api/users",
"bodyPatterns" : [ {
"equalToJson" : { "name": "Jane Doe", "email": "jane@example.com" }
} ]
},
"response": {
"status": 201,
"jsonBody": {
"id": 2,
"name": "Jane Doe",
"email": "jane@example.com"
},
"headers": {
"Content-Type": "application/json",
"Location": "/api/users/2"
}
}
}'
This mock service will return a 201 Created status and the newly created user data when it receives a POST request with the specified JSON body. It’s like giving your API the power to create virtual users on demand!
Adding Delay to Responses
Sometimes, we want to simulate slow responses to test how our frontend handles delays. WireMock makes this easy:
curl -X POST http://localhost:8080/__admin/mappings \
--data '{
"request": {
"method": "GET",
"url": "/api/slow-response"
},
"response": {
"status": 200,
"body": "Sorry for the delay!",
"fixedDelayMilliseconds": 2000
}
}'
This mock service will wait 2 seconds before responding. It’s like teaching your API the art of dramatic pause!
Invoking Mocked Services from a Frontend Application
Now that we have our mock services set up, let’s see how we can use them in a frontend application. We’ll use JavaScript and the Fetch API for our examples, but the principles apply to any frontend technology.
Basic GET Request
Let’s start with a simple GET request to our hello endpoint:
fetch('http://localhost:8080/hello')
.then(response => response.text())
.then(data => console.log(data))
.catch(error => console.error('Error:', error));
When you run this code, you should see “Hello, WireMock!” logged to the console. It’s like your frontend and WireMock are now best friends, chatting away!
Fetching JSON Data
Now let’s fetch our mock user data:
fetch('http://localhost:8080/api/users/1')
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error('Error:', error));
This will log the user object to the console. It’s like your frontend has a direct line to a virtual user database!
Posting Data
Let’s try creating a new user using our POST endpoint:
fetch('http://localhost:8080/api/users', {
method: 'POST',
headers: {
'Content-Type': 'application/json',
},
body: JSON.stringify({
name: 'Jane Doe',
email: 'jane@example.com'
}),
})
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error('Error:', error));
This should log the newly created user object. It’s like your frontend has gained the power to create virtual users at will!
Handling Delays
Finally, let’s see how we can handle the delayed response:
console.log('Sending request...');
fetch('http://localhost:8080/api/slow-response')
.then(response => response.text())
.then(data => {
console.log('Response received:', data);
})
.catch(error => console.error('Error:', error));
You’ll see “Sending request…” logged immediately, but “Response received: Sorry for the delay!” will appear after about 2 seconds. This is great for testing loading states in your frontend!
Advanced WireMock Features
Now that we’ve covered the basics, let’s explore some of WireMock’s more advanced features. These are like the secret levels in a video game – not necessary to finish, but they make the experience so much more enjoyable!
Request Matching
WireMock allows for complex request matching based on headers, query parameters, and more. Here’s an example:
curl -X POST http://localhost:8080/__admin/mappings \
--data '{
"request": {
"method": "GET",
"url": "/api/items",
"queryParameters": {
"category": {
"equalTo": "books"
}
},
"headers": {
"Authorization": {
"matches": "Bearer [a-zA-Z0-9_-]+"
}
}
},
"response": {
"status": 200,
"jsonBody": [
{ "id": 1, "title": "To Kill a Mockingbird" },
{ "id": 2, "title": "1984" }
]
}
}'
This mock will only respond if the request has a query parameter category=books
and an Authorization header that looks like a Bearer token. It’s like giving your mock API a very picky bouncer!
Stateful Behavior
WireMock can maintain state between requests, allowing you to simulate more complex API behaviors:
curl -X POST http://localhost:8080/__admin/mappings \
--data '{
"scenarioName": "Login Flow",
"requiredScenarioState": "Started",
"newScenarioState": "Logged In",
"request": {
"method": "POST",
"url": "/api/login"
},
"response": {
"status": 200,
"body": "Login successful"
}
}'
curl -X POST http://localhost:8080/__admin/mappings \
--data '{
"scenarioName": "Login Flow",
"requiredScenarioState": "Logged In",
"request": {
"method": "GET",
"url": "/api/protected-resource"
},
"response": {
"status": 200,
"body": "This is a protected resource"
}
}'
curl -X POST http://localhost:8080/__admin/mappings \
--data '{
"scenarioName": "Login Flow",
"requiredScenarioState": "Started",
"request": {
"method": "GET",
"url": "/api/protected-resource"
},
"response": {
"status": 401,
"body": "Unauthorized"
}
}'
This set of mocks simulates a login flow. The /api/protected-resource
will return different responses based on whether a successful login has occurred. It’s like giving your mock API a memory!
Response Templating
WireMock supports response templating, allowing you to generate dynamic responses:
curl -X POST http://localhost:8080/__admin/mappings \
--data '{
"request": {
"method": "GET",
"url": "/api/template"
},
"response": {
"status": 200,
"bodyFileName": "template.txt",
"transformers": ["response-template"]
}
}'
echo 'Today is {{now format="yyyy-MM-dd"}}' > __files/template.txt
This will return the current date when you hit /api/template
. It’s like teaching your mock API to use a calendar!
Best Practices for Using WireMock Standalone
As you become more comfortable with WireMock, it’s important to develop good habits to keep your mocking setup manageable and effective. Here are some best practices to keep in mind:
Organize Your Mappings
As your API mocking needs grow, you might find yourself with dozens or even hundreds of stub mappings. Keeping these organized is crucial. Consider using WireMock’s file-based configuration:
- Create a directory structure for your mappings, e.g.:
wiremock/
mappings/
users/
get-user.json
create-user.json
products/
get-products.json
create-product.json
__files/
user-response.json
product-list.json
- Start WireMock with the
--root-dir
option:
java -jar wiremock-standalone-3.0.0-beta-9.jar --root-dir ./wiremock
This approach keeps your mock API organized and makes it easy to version control your stub mappings. It’s like giving your mock API a well-organized filing cabinet!
[…previous content remains the same…]
Use Naming Conventions
Adopt a consistent naming convention for your stub files. For example:
<http-method>-<resource>-<scenario>.json
This might result in files like get-user-success.json
or post-order-invalid-input.json
. A good naming convention makes it easy to find the stub you’re looking for at a glance. It’s like giving each of your mock responses a clear name tag!
Version Control Your Stubs
Treat your stub mappings like code. Store them in version control along with your application code. This allows you to:
- Track changes to your mock API over time
- Share consistent mocks across your team
- Maintain different sets of mocks for different environments or scenarios
It’s like giving your mock API its own historical record!
Use Priority for Overlapping Stubs
When you have multiple stubs that could match the same request, use the priority
field to determine which one should be used:
{
"priority": 1,
"request": {
"method": "GET",
"url": "/api/data"
},
"response": {
"status": 200,
"body": "Specific response"
}
}
Lower numbers indicate higher priority. This allows you to have specific stubs take precedence over more general ones. It’s like teaching your mock API to make decisions!
Leverage Proxy Mode for Development
WireMock can act as a proxy to a real API, allowing you to selectively override certain endpoints while passing through others. Start WireMock with:
java -jar wiremock-standalone-3.0.0-beta-9.jar --proxy-all="http://api.real-service.com"
This is incredibly useful during development when you want to mock out certain endpoints but use the real API for others. It’s like giving your mock API a direct line to the real world!
Use Record and Playback for Complex APIs
For complex APIs, use WireMock’s record and playback feature to capture real API responses:
- Start WireMock in recording mode:
java -jar wiremock-standalone-3.0.0-beta-9.jar --proxy-all="http://api.real-service.com" --record-mappings
- Make requests to
http://localhost:8080
- WireMock will forward these to the real API and save the responses as stub mappings
This allows you to quickly create a comprehensive mock API based on real responses. It’s like teaching your mock API by example!
Conclusion: Embracing the Power of Standalone WireMock
And there you have it, folks – a comprehensive guide to setting up and using WireMock as a standalone application for HTTP mocking. We’ve covered everything from basic setup to advanced features, best practices, and even some troubleshooting tips. By now, you should be well-equipped to harness the power of WireMock in your development workflow.
Remember, WireMock standalone is more than just a tool – it’s a game-changer in the world of frontend development and API testing. With WireMock at your side, you can:
- Develop your frontend independently of backend services
- Test your application against a wide range of scenarios, including error conditions
- Improve the speed and reliability of your automated tests
- Simulate complex API behaviors without needing to set up a full backend environment
As you continue your journey with WireMock, don’t be afraid to experiment and push the boundaries of what’s possible. Try combining different features, like using response templating with stateful behavior to create complex, dynamic mocks. Or use the record and playback functionality to quickly create a mock version of a complex third-party API.
Remember, the goal isn’t to replace your entire backend with mocks, but to use mocking strategically to enhance your development and testing capabilities. Like a master chef choosing the right ingredients, you’ll learn when to use real services and when to reach for WireMock.
As you become more proficient with WireMock, you might even find yourself thinking differently about API design and testing. You might start considering how to make your APIs more mockable, or how to structure your applications to be more easily testable with tools like WireMock.
So go forth and mock with confidence! Your development process will be more efficient, your tests more reliable, and your applications more robust. And who knows? You might even start to enjoy the process of API integration. (Okay, let’s not get carried away – but you’ll definitely dread it less!)
Thank you for joining me on this deep dive into the world of standalone WireMock. Happy mocking, and may all your API calls be successful (even when they’re not supposed to be)!
Disclaimer: While every effort has been made to ensure the accuracy and reliability of this guide, software and its ecosystem are constantly evolving. The information provided here is based on the latest available knowledge at the time of writing. Please always refer to the official WireMock documentation for the most up-to-date information. If you notice any inaccuracies or have suggestions for improvements, please report them so we can correct them promptly. Remember, in the world of software development, learning is a continuous journey – stay curious, keep exploring, and always be ready to adapt to new challenges and opportunities!