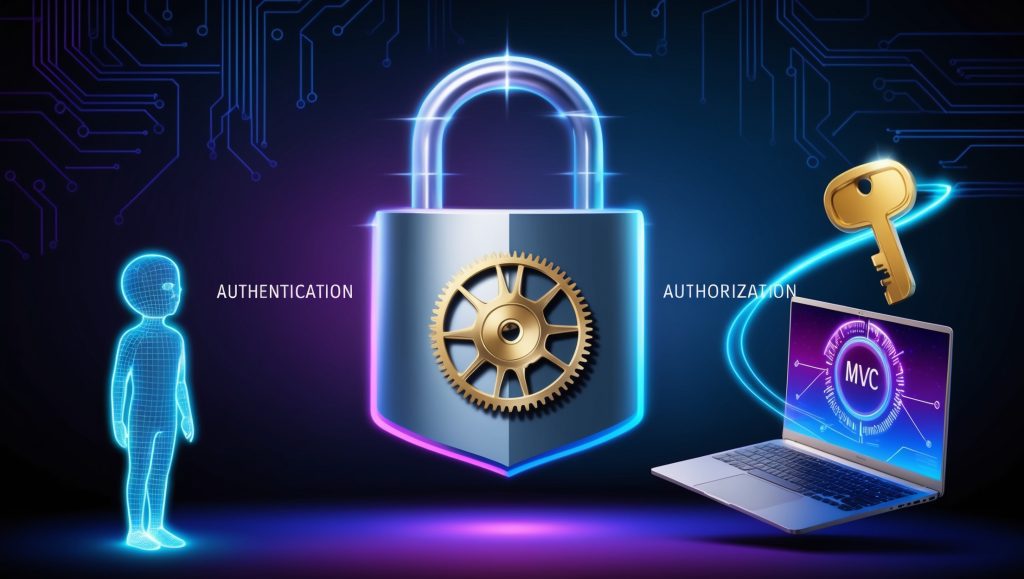
Implementing Authentication and Authorization in MVC
Before diving into implementation details, it’s essential to understand the key concepts of authentication and authorization. Authentication verifies the identity of users attempting to access your application, while authorization determines what actions authenticated users can perform. These two security layers work together to create a comprehensive security framework for your MVC application.
Key Security Concepts
- Authentication: The process of verifying user identity through credentials like username/password, tokens, or biometrics
- Authorization: The process of determining user permissions and access rights
- Session Management: Maintaining user state across multiple requests
- Password Security: Implementing secure password storage and validation
- Access Control: Restricting access to specific resources based on user roles
Setting Up the Authentication System
Let’s start by implementing a basic authentication system in both Python (using Flask) and Java (using Spring MVC). We’ll create a secure login system with proper password hashing and session management.
Python Implementation with Flask
from flask import Flask, request, session
from werkzeug.security import generate_password_hash, check_password_hash
from functools import wraps
import sqlite3
app = Flask(__name__)
app.secret_key = 'your-secret-key-here' # Change this to a secure secret key
# Database setup
def init_db():
conn = sqlite3.connect('users.db')
c = conn.cursor()
c.execute('''
CREATE TABLE IF NOT EXISTS users
(id INTEGER PRIMARY KEY AUTOINCREMENT,
username TEXT UNIQUE NOT NULL,
password TEXT NOT NULL,
role TEXT NOT NULL)
''')
conn.commit()
conn.close()
# User registration
@app.route('/register', methods=['POST'])
def register():
data = request.get_json()
username = data.get('username')
password = data.get('password')
role = data.get('role', 'user') # Default role is 'user'
if not username or not password:
return {'error': 'Missing credentials'}, 400
hashed_password = generate_password_hash(password)
try:
conn = sqlite3.connect('users.db')
c = conn.cursor()
c.execute('INSERT INTO users (username, password, role) VALUES (?, ?, ?)',
(username, hashed_password, role))
conn.commit()
conn.close()
return {'message': 'User registered successfully'}, 201
except sqlite3.IntegrityError:
return {'error': 'Username already exists'}, 409
# User login
@app.route('/login', methods=['POST'])
def login():
data = request.get_json()
username = data.get('username')
password = data.get('password')
if not username or not password:
return {'error': 'Missing credentials'}, 400
conn = sqlite3.connect('users.db')
c = conn.cursor()
c.execute('SELECT * FROM users WHERE username = ?', (username,))
user = c.fetchone()
conn.close()
if user and check_password_hash(user[2], password):
session['user_id'] = user[0]
session['role'] = user[3]
return {'message': 'Login successful'}, 200
return {'error': 'Invalid credentials'}, 401
Java Implementation with Spring MVC
@Configuration
@EnableWebSecurity
public class SecurityConfig extends WebSecurityConfigurerAdapter {
@Autowired
private UserDetailsService userDetailsService;
@Bean
public PasswordEncoder passwordEncoder() {
return new BCryptPasswordEncoder();
}
@Override
protected void configure(HttpSecurity http) throws Exception {
http
.authorizeRequests()
.antMatchers("/public/**").permitAll()
.antMatchers("/api/**").authenticated()
.antMatchers("/admin/**").hasRole("ADMIN")
.anyRequest().authenticated()
.and()
.formLogin()
.loginPage("/login")
.permitAll()
.and()
.logout()
.permitAll();
}
@Override
public void configure(AuthenticationManagerBuilder auth) throws Exception {
auth.userDetailsService(userDetailsService)
.passwordEncoder(passwordEncoder());
}
}
@Entity
@Table(name = "users")
public class User implements UserDetails {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
@Column(unique = true, nullable = false)
private String username;
@Column(nullable = false)
private String password;
@ElementCollection(fetch = FetchType.EAGER)
private Set<String> roles = new HashSet<>();
// Implement UserDetails methods
@Override
public Collection<? extends GrantedAuthority> getAuthorities() {
return roles.stream()
.map(role -> new SimpleGrantedAuthority("ROLE_" + role))
.collect(Collectors.toList());
}
// Other UserDetails implementations...
}
Implementing Authorization
Once authentication is in place, we need to implement authorization to control what resources users can access. We’ll implement role-based access control (RBAC) and method-level security.
Python Decorator for Role-Based Authorization
def require_role(role):
def decorator(f):
@wraps(f)
def wrapped(*args, **kwargs):
if 'role' not in session:
return {'error': 'Unauthorized'}, 401
if session['role'] != role:
return {'error': 'Forbidden'}, 403
return f(*args, **kwargs)
return wrapped
return decorator
# Example protected route
@app.route('/admin/dashboard')
@require_role('admin')
def admin_dashboard():
return {'message': 'Welcome to admin dashboard'}
# Example user route
@app.route('/user/profile')
@require_role('user')
def user_profile():
return {'message': 'Welcome to user profile'}
Java Method-Level Security
@Service
public class UserService {
@PreAuthorize("hasRole('ADMIN')")
public List<User> getAllUsers() {
// Implementation
}
@PreAuthorize("hasRole('USER') and #username == authentication.principal.username")
public UserProfile getUserProfile(String username) {
// Implementation
}
}
@RestController
@RequestMapping("/api")
public class UserController {
@Autowired
private UserService userService;
@GetMapping("/users")
@PreAuthorize("hasRole('ADMIN')")
public ResponseEntity<List<User>> getAllUsers() {
return ResponseEntity.ok(userService.getAllUsers());
}
@GetMapping("/profile/{username}")
public ResponseEntity<UserProfile> getUserProfile(@PathVariable String username) {
return ResponseEntity.ok(userService.getUserProfile(username));
}
}
Session Management and Security Best Practices
Proper session management is crucial for maintaining security. Here’s a table of important security considerations and their implementations:
Security Measure | Purpose | Implementation |
---|---|---|
Session Timeout | Prevent unauthorized access to abandoned sessions | Set appropriate session timeout values |
CSRF Protection | Prevent cross-site request forgery attacks | Implement CSRF tokens |
Secure Cookies | Protect session cookies | Set secure and HttpOnly flags |
Rate Limiting | Prevent brute force attacks | Implement request rate limiting |
Python Session Security Implementation
from flask_limiter import Limiter
from flask_limiter.util import get_remote_address
# Configure session security
app.config.update(
SESSION_COOKIE_SECURE=True,
SESSION_COOKIE_HTTPONLY=True,
SESSION_COOKIE_SAMESITE='Lax',
PERMANENT_SESSION_LIFETIME=timedelta(minutes=30)
)
# Setup rate limiting
limiter = Limiter(
app,
key_func=get_remote_address,
default_limits=["200 per day", "50 per hour"]
)
# Apply rate limiting to login route
@app.route('/login', methods=['POST'])
@limiter.limit("5 per minute")
def login():
# Login implementation
pass
Java Session Security Configuration
@Configuration
public class SessionConfig extends WebSecurityConfigurerAdapter {
@Override
protected void configure(HttpSecurity http) throws Exception {
http
.sessionManagement()
.sessionCreationPolicy(SessionCreationPolicy.IF_REQUIRED)
.invalidSessionUrl("/login")
.maximumSessions(1)
.maxSessionsPreventsLogin(true)
.and()
.csrf()
.csrfTokenRepository(CookieCsrfTokenRepository.withHttpOnlyFalse())
.and()
.headers()
.frameOptions().deny()
.xssProtection()
.and()
.contentSecurityPolicy("default-src 'self'");
}
}
Testing Security Implementation
Security testing is essential to ensure your authentication and authorization mechanisms work as intended. Here’s how to implement security tests:
Python Security Tests
import unittest
from app import app
class SecurityTests(unittest.TestCase):
def setUp(self):
self.app = app.test_client()
self.app.testing = True
def test_unauthorized_access(self):
response = self.app.get('/admin/dashboard')
self.assertEqual(response.status_code, 401)
def test_successful_login(self):
credentials = {
'username': 'test_user',
'password': 'test_password'
}
response = self.app.post('/login', json=credentials)
self.assertEqual(response.status_code, 200)
def test_invalid_credentials(self):
credentials = {
'username': 'test_user',
'password': 'wrong_password'
}
response = self.app.post('/login', json=credentials)
self.assertEqual(response.status_code, 401)
Java Security Tests
@SpringBootTest
@AutoConfigureMockMvc
public class SecurityTests {
@Autowired
private MockMvc mockMvc;
@Test
public void whenAccessingProtectedEndpoint_thenUnauthorized() throws Exception {
mockMvc.perform(get("/api/admin/users"))
.andExpect(status().isUnauthorized());
}
@Test
@WithMockUser(roles = "USER")
public void whenUserAccessesAdminEndpoint_thenForbidden() throws Exception {
mockMvc.perform(get("/api/admin/users"))
.andExpect(status().isForbidden());
}
@Test
@WithMockUser(roles = "ADMIN")
public void whenAdminAccessesAdminEndpoint_thenSuccess() throws Exception {
mockMvc.perform(get("/api/admin/users"))
.andExpect(status().isOk());
}
}
Monitoring and Logging Security Events
Implementing comprehensive logging and monitoring is crucial for maintaining security and detecting potential threats:
Python Security Logging
import logging
from datetime import datetime
logging.basicConfig(
filename='security.log',
level=logging.INFO,
format='%(asctime)s - %(levelname)s - %(message)s'
)
def log_security_event(event_type, user, details):
logging.info({
'timestamp': datetime.utcnow().isoformat(),
'event_type': event_type,
'user': user,
'details': details,
'ip_address': request.remote_addr
})
@app.route('/login', methods=['POST'])
def login():
username = request.json.get('username')
try:
# Login logic here
log_security_event('login_success', username, 'Successful login')
return {'message': 'Login successful'}
except Exception as e:
log_security_event('login_failure', username, str(e))
return {'error': 'Login failed'}, 401
Java Security Logging
@Aspect
@Component
public class SecurityAuditAspect {
private static final Logger logger = LoggerFactory.getLogger(SecurityAuditAspect.class);
@Pointcut("@annotation(org.springframework.security.access.prepost.PreAuthorize)")
public void securedMethods() {}
@Around("securedMethods()")
public Object auditSecurityEvent(ProceedingJoinPoint joinPoint) throws Throwable {
Authentication authentication = SecurityContextHolder.getContext().getAuthentication();
String username = authentication != null ? authentication.getName() : "anonymous";
try {
Object result = joinPoint.proceed();
logSecurityEvent("ACCESS_GRANTED", username, joinPoint.getSignature().getName());
return result;
} catch (AccessDeniedException e) {
logSecurityEvent("ACCESS_DENIED", username, joinPoint.getSignature().getName());
throw e;
}
}
private void logSecurityEvent(String eventType, String username, String method) {
SecurityEvent event = SecurityEvent.builder()
.timestamp(LocalDateTime.now())
.eventType(eventType)
.username(username)
.method(method)
.build();
logger.info("Security Event: {}", event);
}
}
Conclusion
Implementing authentication and authorization in MVC applications requires careful consideration of multiple security aspects. By following the practices and implementations outlined in this guide, you can create a robust security framework that protects your application and its users. Remember to regularly update security measures, conduct security audits, and stay informed about new security threats and best practices.
Disclaimer: The code examples and security implementations provided in this blog post are for educational purposes only. While we strive to provide accurate and up-to-date information, security requirements may vary based on your specific use case. Always review and test security implementations thoroughly before deploying to production environments. Please report any inaccuracies to our technical team for prompt correction.