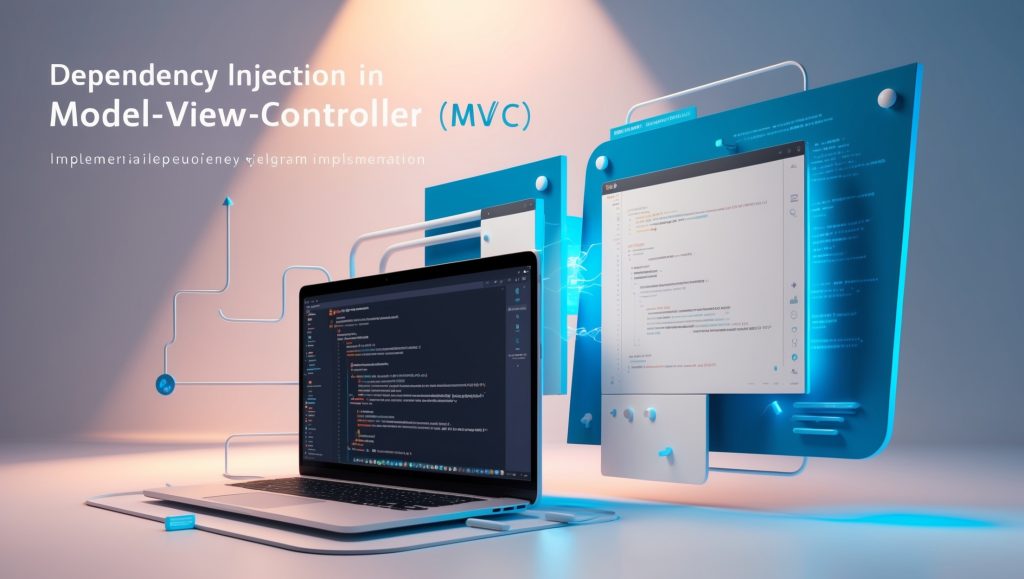
Implementing Dependency Injection in MVC
Dependency Injection (DI) stands as one of the most powerful design patterns in modern software development, particularly within the Model-View-Controller (MVC) architectural pattern. This fundamental technique helps developers create more maintainable, flexible, and testable applications by reducing tight coupling between components. As applications grow in complexity, managing dependencies becomes increasingly challenging, making DI an essential tool in a developer’s arsenal. When implemented correctly, DI can significantly improve code quality by promoting loose coupling, enabling better unit testing, and facilitating easier maintenance. This comprehensive guide will explore the implementation of DI in MVC applications, demonstrating practical examples in both Python and Java while highlighting best practices and common pitfalls to avoid.
Understanding Dependency Injection Fundamentals
Dependency Injection represents a design pattern where objects receive their dependencies from external sources rather than creating them internally. This approach aligns perfectly with the SOLID principles, particularly the Dependency Inversion Principle, which suggests that high-level modules should not depend on low-level modules but rather on abstractions. DI manifests in three primary forms: constructor injection, setter injection, and interface injection. Each method has its unique advantages and use cases within an MVC architecture. Constructor injection proves most popular due to its ability to ensure that all required dependencies are available at object creation time, making it impossible to create an object in an invalid state.
Key Benefits of Dependency Injection:
- Improved testability through easy mocking of dependencies
- Enhanced code modularity and reusability
- Reduced coupling between components
- Simplified maintenance and refactoring
- Better separation of concerns
- Increased code flexibility and scalability
Implementing DI in Python MVC Applications
Python’s dynamic nature and extensive ecosystem provide multiple approaches to implementing DI in MVC applications. Let’s explore a practical implementation using a simple example of a user management system.
from abc import ABC, abstractmethod
from typing import List
# Interfaces
class IUserRepository(ABC):
@abstractmethod
def get_all_users(self) -> List[dict]:
pass
@abstractmethod
def get_user_by_id(self, user_id: int) -> dict:
pass
# Implementation
class UserRepository(IUserRepository):
def __init__(self, database_connection):
self.db = database_connection
def get_all_users(self) -> List[dict]:
return self.db.query("SELECT * FROM users")
def get_user_by_id(self, user_id: int) -> dict:
return self.db.query("SELECT * FROM users WHERE id = ?", [user_id])
# Service Layer
class UserService:
def __init__(self, user_repository: IUserRepository):
self.user_repository = user_repository
def get_active_users(self) -> List[dict]:
users = self.user_repository.get_all_users()
return [user for user in users if user['status'] == 'active']
# Controller
class UserController:
def __init__(self, user_service: UserService):
self.user_service = user_service
def get_active_users(self):
try:
users = self.user_service.get_active_users()
return {"status": "success", "data": users}
except Exception as e:
return {"status": "error", "message": str(e)}
Setting Up DI in Java MVC Applications
Java’s robust ecosystem offers several DI frameworks, with Spring being the most popular. Here’s an example implementing DI in a Spring MVC application:
// Interface
public interface UserRepository {
List<User> getAllUsers();
User getUserById(Long id);
}
// Implementation
@Repository
public class UserRepositoryImpl implements UserRepository {
private final JdbcTemplate jdbcTemplate;
@Autowired
public UserRepositoryImpl(JdbcTemplate jdbcTemplate) {
this.jdbcTemplate = jdbcTemplate;
}
@Override
public List<User> getAllUsers() {
return jdbcTemplate.query(
"SELECT * FROM users",
(rs, rowNum) ->
new User(
rs.getLong("id"),
rs.getString("name"),
rs.getString("email")
)
);
}
@Override
public User getUserById(Long id) {
return jdbcTemplate.queryForObject(
"SELECT * FROM users WHERE id = ?",
new Object[]{id},
(rs, rowNum) ->
new User(
rs.getLong("id"),
rs.getString("name"),
rs.getString("email")
)
);
}
}
// Service Layer
@Service
public class UserService {
private final UserRepository userRepository;
@Autowired
public UserService(UserRepository userRepository) {
this.userRepository = userRepository;
}
public List<User> getActiveUsers() {
return userRepository.getAllUsers()
.stream()
.filter(user -> user.getStatus().equals("active"))
.collect(Collectors.toList());
}
}
// Controller
@RestController
@RequestMapping("/api/users")
public class UserController {
private final UserService userService;
@Autowired
public UserController(UserService userService) {
this.userService = userService;
}
@GetMapping("/active")
public ResponseEntity<List<User>> getActiveUsers() {
try {
List<User> users = userService.getActiveUsers();
return ResponseEntity.ok(users);
} catch (Exception e) {
return ResponseEntity.status(HttpStatus.INTERNAL_SERVER_ERROR).build();
}
}
}
Best Practices for DI Implementation
When implementing DI in MVC applications, following established best practices ensures maintainable and efficient code:
Constructor Injection vs. Setter Injection:
Injection Type | Advantages | Disadvantages |
---|---|---|
Constructor | – Ensures required dependencies | – Constructor can become complex |
– Promotes immutability | – All dependencies needed at creation | |
– Clear dependency declaration | ||
Setter | – Flexible dependency changes | – Dependencies can be null |
– Optional dependencies | – Object state can be invalid | |
– Easier circular dependency resolution | – Less clear dependency requirements |
Additional Best Practices:
- Always program to interfaces rather than concrete implementations
- Keep injection constructors simple and focused
- Use appropriate scope for injected dependencies
- Implement proper error handling and dependency validation
- Maintain clear documentation of dependencies
Testing with Dependency Injection
One of the primary benefits of DI is improved testability. Here’s an example of unit testing with DI in Python:
import unittest
from unittest.mock import Mock
class TestUserService(unittest.TestCase):
def setUp(self):
self.mock_repository = Mock(spec=IUserRepository)
self.user_service = UserService(self.mock_repository)
def test_get_active_users(self):
# Arrange
mock_users = [
{"id": 1, "name": "John", "status": "active"},
{"id": 2, "name": "Jane", "status": "inactive"},
{"id": 3, "name": "Bob", "status": "active"}
]
self.mock_repository.get_all_users.return_value = mock_users
# Act
active_users = self.user_service.get_active_users()
# Assert
self.assertEqual(len(active_users), 2)
self.assertTrue(all(user["status"] == "active" for user in active_users))
self.mock_repository.get_all_users.assert_called_once()
if __name__ == '__main__':
unittest.main()
And here’s an equivalent example in Java using JUnit and Mockito:
@SpringBootTest
public class UserServiceTest {
@Mock
private UserRepository userRepository;
@InjectMocks
private UserService userService;
@BeforeEach
void setUp() {
MockitoAnnotations.openMocks(this);
}
@Test
void testGetActiveUsers() {
// Arrange
List<User> mockUsers = Arrays.asList(
new User(1L, "John", "active"),
new User(2L, "Jane", "inactive"),
new User(3L, "Bob", "active")
);
when(userRepository.getAllUsers()).thenReturn(mockUsers);
// Act
List<User> activeUsers = userService.getActiveUsers();
// Assert
assertEquals(2, activeUsers.size());
assertTrue(activeUsers.stream()
.allMatch(user -> user.getStatus().equals("active")));
verify(userRepository, times(1)).getAllUsers();
}
}
Common Pitfalls and Solutions
When implementing DI in MVC applications, developers often encounter several challenges:
Constructor Over-injection:
// Bad Practice
public class UserController {
private final UserService userService;
private final LoggingService loggingService;
private final MetricsService metricsService;
private final CacheService cacheService;
private final ValidationService validationService;
// More dependencies...
@Autowired
public UserController(UserService userService, LoggingService loggingService,
MetricsService metricsService, CacheService cacheService,
ValidationService validationService) {
// Constructor with too many parameters
}
}
// Good Practice
public class UserController {
private final UserFacade userFacade;
@Autowired
public UserController(UserFacade userFacade) {
this.userFacade = userFacade;
}
}
@Service
public class UserFacade {
// Facade pattern to manage multiple dependencies
}
Advanced DI Patterns and Techniques
Modern applications often require sophisticated DI patterns to handle complex scenarios:
Factory Pattern with DI:
from typing import Dict, Type
class UserServiceFactory:
def __init__(self):
self._services: Dict[str, Type[UserService]] = {}
def register(self, name: str, service_class: Type[UserService]):
self._services[name] = service_class
def create(self, name: str, repository: IUserRepository) -> UserService:
service_class = self._services.get(name)
if not service_class:
raise ValueError(f"Unknown service: {name}")
return service_class(repository)
Performance Considerations
Implementing DI can impact application performance if not done correctly. Here are key considerations:
Scope Management:
Scope | Use Case | Performance Impact |
---|---|---|
Singleton | Shared state, heavy resources | Minimal – Single instance |
Prototype | Unique state per instance | Medium – New instance per request |
Request | Web request scope | High – New instance per HTTP request |
Session | User session scope | High – Instance per user session |
Conclusion
Implementing Dependency Injection in MVC applications represents a crucial step toward building maintainable, testable, and flexible software systems. Through proper implementation of DI principles and patterns, developers can create applications that are easier to test, maintain, and scale. The examples provided in both Python and Java demonstrate practical approaches to implementing DI across different technology stacks, while the best practices and pitfalls discussed help avoid common implementation mistakes. As applications continue to grow in complexity, mastering DI becomes increasingly important for modern software development.
Disclaimer: This article provides general guidance on implementing Dependency Injection in MVC applications. While every effort has been made to ensure accuracy, specific implementations may vary based on framework versions and project requirements. Code examples are simplified for demonstration purposes and may need additional error handling and security considerations for production use. Please report any inaccuracies or suggestions for improvement to our editorial team.