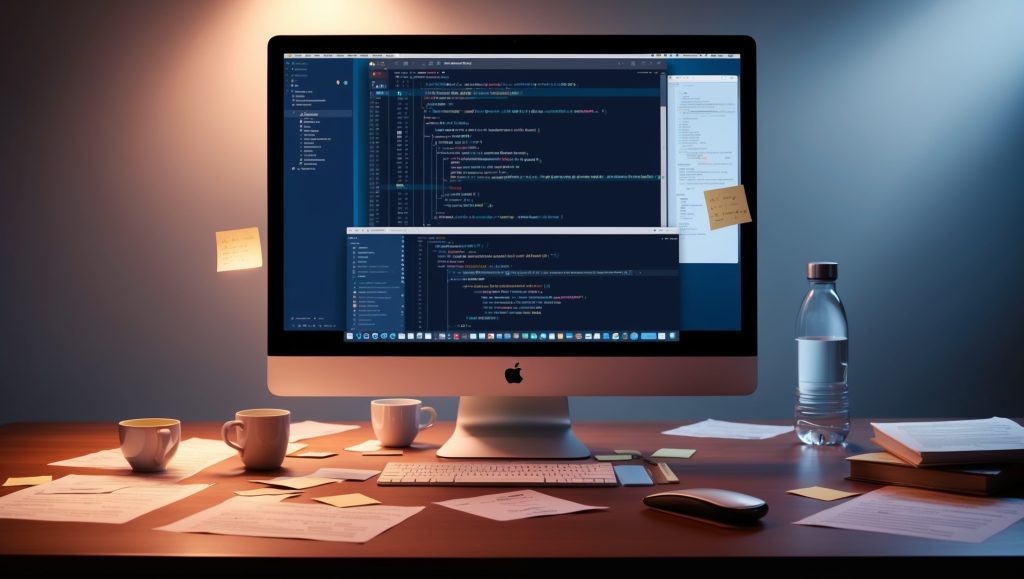
Implementing the Singleton Pattern in MVC
The Singleton pattern stands as one of the most fundamental and widely-used design patterns in software engineering, particularly within the Model-View-Controller (MVC) architectural pattern. This design pattern ensures that a class has only one instance throughout the application’s lifecycle while providing a global point of access to that instance. In modern web applications, where resource management and state control are crucial, implementing the Singleton pattern effectively can significantly improve performance and maintain data consistency. This comprehensive guide explores the implementation of the Singleton pattern within MVC architecture, focusing on practical applications, best practices, and real-world scenarios. We’ll examine implementations in both Python and Java, discussing the advantages, potential pitfalls, and optimal use cases for this powerful design pattern.
Understanding the Singleton Pattern Fundamentals
The Singleton pattern addresses a common challenge in software development: ensuring that certain classes have exactly one instance while providing global access to that instance. This pattern becomes particularly relevant in MVC applications where shared resources, such as database connections, configuration settings, or cache managers, need to be managed efficiently. The pattern’s primary characteristics include a private constructor to prevent direct instantiation, a private static instance of the class, and a public static method that returns the instance.
Key Characteristics of the Singleton Pattern:
- Single Instance Guarantee
- Global Access Point
- Lazy Initialization Support
- Thread Safety Considerations
- State Management Capabilities
Basic Implementation in Python
Let’s start with a basic implementation of the Singleton pattern in Python, demonstrating both thread-safe and non-thread-safe versions.
# Basic Singleton Implementation
class Singleton:
_instance = None
def __new__(cls):
if cls._instance is None:
cls._instance = super().__new__(cls)
return cls._instance
def __init__(self):
self.data = []
# Thread-Safe Implementation
from threading import Lock
class ThreadSafeSingleton:
_instance = None
_lock = Lock()
def __new__(cls):
with cls._lock:
if cls._instance is None:
cls._instance = super().__new__(cls)
return cls._instance
def __init__(self):
self.data = []
Java Implementation with Double-Checked Locking
The Java implementation requires additional consideration for thread safety, commonly implemented using the double-checked locking pattern.
public class Singleton {
private static volatile Singleton instance;
private List<String> data;
private Singleton() {
data = new ArrayList<>();
}
public static Singleton getInstance() {
if (instance == null) {
synchronized (Singleton.class) {
if (instance == null) {
instance = new Singleton();
}
}
}
return instance;
}
public void addData(String item) {
data.add(item);
}
public List<String> getData() {
return new ArrayList<>(data);
}
}
Implementing Singleton in MVC Components
In MVC architecture, the Singleton pattern finds various applications across different components. Let’s explore practical implementations for each MVC component.
Model Implementation:
class DatabaseConnection:
_instance = None
_lock = Lock()
def __new__(cls):
with cls._lock:
if cls._instance is None:
cls._instance = super().__new__(cls)
cls._instance.initialize_connection()
return cls._instance
def initialize_connection(self):
self.connection = None
self.connection_params = {
'host': 'localhost',
'port': 5432,
'database': 'myapp',
'user': 'admin'
}
def connect(self):
if not self.connection:
# Simulate database connection
self.connection = f"Connected to {self.connection_params['database']}"
return self.connection
Controller Implementation:
public class ApplicationController {
private static volatile ApplicationController instance;
private final Map<String, RequestHandler> handlers;
private ApplicationController() {
handlers = new HashMap<>();
initializeHandlers();
}
public static ApplicationController getInstance() {
if (instance == null) {
synchronized (ApplicationController.class) {
if (instance == null) {
instance = new ApplicationController();
}
}
}
return instance;
}
private void initializeHandlers() {
handlers.put("user", new UserRequestHandler());
handlers.put("product", new ProductRequestHandler());
}
public RequestHandler getHandler(String type) {
return handlers.get(type);
}
}
Managing Shared Resources
One of the primary use cases for the Singleton pattern in MVC applications is managing shared resources. Let’s examine a comprehensive implementation of a cache manager.
class CacheManager:
_instance = None
_lock = Lock()
def __new__(cls):
with cls._lock:
if cls._instance is None:
cls._instance = super().__new__(cls)
cls._instance.initialize_cache()
return cls._instance
def initialize_cache(self):
self._cache = {}
self._cache_timeout = {}
self._default_timeout = 3600 # 1 hour
def set(self, key, value, timeout=None):
with self._lock:
self._cache[key] = value
self._cache_timeout[key] = time.time() + (timeout or self._default_timeout)
def get(self, key):
with self._lock:
if key in self._cache:
if time.time() < self._cache_timeout[key]:
return self._cache[key]
else:
del self._cache[key]
del self._cache_timeout[key]
return None
Testing Singleton Implementations
Testing Singleton implementations requires special consideration to ensure thread safety and proper instance management. Here’s a comprehensive test suite:
import unittest
import threading
class TestSingleton(unittest.TestCase):
def test_singleton_instance(self):
instance1 = CacheManager()
instance2 = CacheManager()
self.assertEqual(id(instance1), id(instance2))
def test_thread_safety(self):
instances = []
def create_instance():
instances.append(id(CacheManager()))
threads = []
for _ in range(10):
thread = threading.Thread(target=create_instance)
threads.append(thread)
thread.start()
for thread in threads:
thread.join()
self.assertEqual(len(set(instances)), 1)
if __name__ == '__main__':
unittest.main()
Best Practices and Common Pitfalls
When implementing the Singleton pattern in MVC applications, several best practices should be followed:
1. Initialization Timing
- Lazy initialization for resource-heavy instances
- Eager initialization for lightweight, frequently-used instances
- Always implement proper synchronization mechanisms
- Use double-checked locking in Java implementations
- Consider using atomic operations when possible
- Implement proper cleanup methods
- Handle resource release in application shutdown
- Monitor resource usage and implement limits
2. Thread Safety Considerations
3. Resource Management
Here’s a table summarizing common pitfalls and their solutions:
Pitfall | Impact | Solution |
---|---|---|
Missing Thread Safety | Race conditions and multiple instances | Implement proper synchronization |
Memory Leaks | Resource exhaustion | Implement cleanup mechanisms |
Tight Coupling | Reduced maintainability | Use dependency injection when possible |
Excessive Global State | Complex testing and debugging | Limit singleton usage to necessary cases |
Advanced Implementation Patterns
For more complex applications, consider these advanced implementation patterns:
public class Registry {
private static final Registry INSTANCE = new Registry();
private final Map<Class<?>, Object> instances = new ConcurrentHashMap<>();
private Registry() {}
public static Registry getInstance() {
return INSTANCE;
}
public <T> void register(Class<T> type, T instance) {
instances.put(type, instance);
}
@SuppressWarnings("unchecked")
public <T> T get(Class<T> type) {
return (T) instances.get(type);
}
public void reset() {
instances.clear();
}
}
Performance Considerations
The Singleton pattern can significantly impact application performance. Here are key metrics to consider:
Memory Usage:
- Single instance reduces memory overhead
- Shared resources are efficiently utilized
- Careful management of cached data is essential
- Global access reduces lookup time
- Synchronized access may cause contention
- Proper caching strategies can improve performance
Response Time:
Security Implications
When implementing Singleton patterns in MVC applications, security considerations are crucial:
1. Access Control
class SecureSingleton:
_instance = None
_lock = Lock()
_access_token = None
@classmethod
def getInstance(cls, token):
if not cls._validate_token(token):
raise SecurityException("Invalid access token")
with cls._lock:
if cls._instance is None:
cls._instance = super().__new__(cls)
cls._instance._initialize()
return cls._instance
@staticmethod
def _validate_token(token):
# Implement token validation logic
return token == "valid_token"
Integration with Modern Frameworks
Modern frameworks often provide their own dependency injection and singleton management. Here’s how to integrate custom singletons:
Spring Framework (Java):
@Component
@Scope("singleton")
public class ApplicationCache {
private final Map<String, Object> cache = new ConcurrentHashMap<>();
@PostConstruct
private void initialize() {
// Initialization logic
}
public void put(String key, Object value) {
cache.put(key, value);
}
public Object get(String key) {
return cache.get(key);
}
}
Conclusion
The Singleton pattern, when properly implemented in MVC applications, provides an efficient solution for managing shared resources and maintaining application state. By following the best practices and implementation patterns discussed in this guide, developers can create robust, maintainable applications that effectively handle resource management while avoiding common pitfalls. Remember to carefully consider the specific needs of your application when deciding to implement the Singleton pattern, as its global state characteristic can impact testing and maintenance if not properly managed.
Disclaimer: The code examples and implementations provided in this blog post are for educational purposes and may need to be adapted based on specific requirements and constraints of your application. While we strive for accuracy, technology evolves rapidly, and best practices may change. Please report any inaccuracies or outdated information to our editorial team for prompt correction. Always thoroughly test implementations in your specific environment before deploying to production.