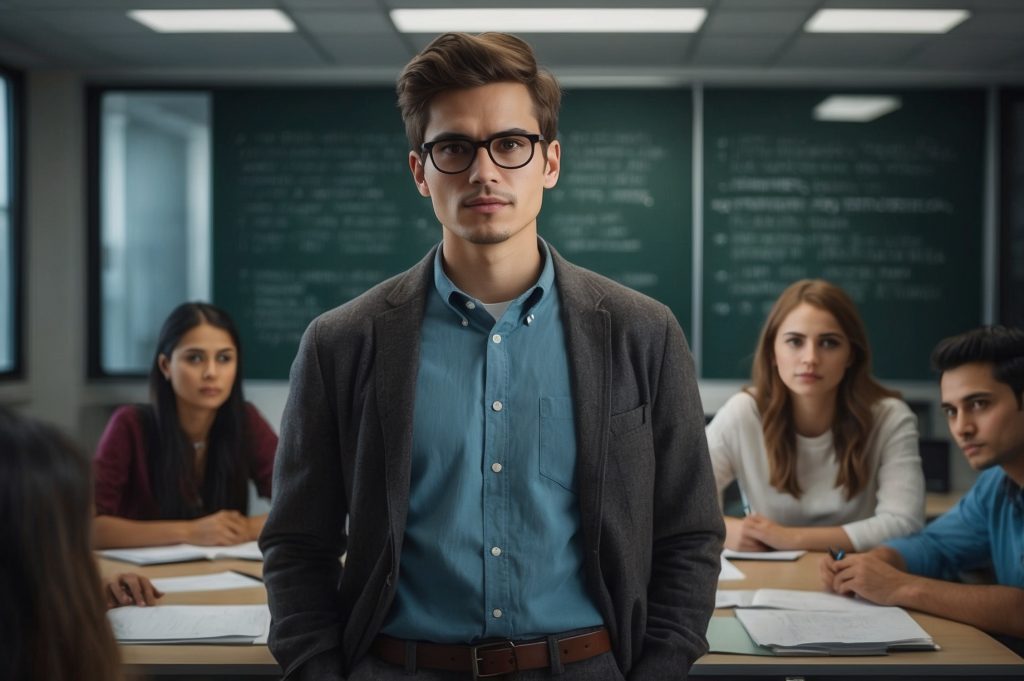
Introduction to Object-Oriented Programming
Object-Oriented Programming (OOP) is a paradigm that has transformed the way software is developed. Unlike procedural programming, which revolves around functions and procedures, OOP organizes software design around data, or objects, and the methods that operate on these objects. This approach mirrors the way we think about real-world entities, making it easier to model complex systems. In this blog, we’ll explore the basics of OOP, its core principles, and how it enhances software development. We’ll also provide plenty of code snippets to illustrate these concepts.
What is Object-Oriented Programming?
OOP is a programming paradigm that uses objects and classes. An object is an instance of a class, and a class is a blueprint that defines the properties and behaviors (methods) of the objects created from it. This approach provides a modular structure to programs, making them easier to manage and extend. By encapsulating data and behavior within objects, OOP helps in creating more flexible and reusable code.
Sample Code: Basic Class and Object
class Dog:
def __init__(self, name, age):
self.name = name
self.age = age
def bark(self):
return f"{self.name} says Woof!"
# Creating an object of the Dog class
my_dog = Dog("Buddy", 5)
print(my_dog.bark())
In this example, Dog
is a class with an initializer method (__init__
) that sets the name and age of the dog. The bark
method defines the behavior of the dog object. my_dog
is an instance of the Dog
class.
Core Principles of OOP
OOP is built on four main principles: Encapsulation, Abstraction, Inheritance, and Polymorphism. Understanding these principles is crucial for mastering OOP.
Encapsulation
Encapsulation refers to bundling the data (attributes) and methods (functions) that operate on the data into a single unit, or class. It restricts direct access to some of an object’s components, which is a means of preventing accidental interference and misuse of the methods and data. Access to these components is typically controlled through public methods.
Sample Code: Encapsulation
class BankAccount:
def __init__(self, account_number, balance):
self.account_number = account_number
self.__balance = balance # Private attribute
def deposit(self, amount):
if amount > 0:
self.__balance += amount
def withdraw(self, amount):
if 0 < amount <= self.__balance:
self.__balance -= amount
def get_balance(self):
return self.__balance
# Creating an object of the BankAccount class
account = BankAccount("12345678", 1000)
account.deposit(500)
account.withdraw(200)
print(account.get_balance())
In this example, the BankAccount
class encapsulates the account number and balance. The balance is a private attribute, meaning it cannot be accessed directly from outside the class. Instead, the deposit
, withdraw
, and get_balance
methods control access to the balance.
Abstraction
Abstraction involves hiding complex implementation details and showing only the necessary features of an object. It helps in reducing programming complexity and effort. Through abstraction, a programmer can manage complexity by omitting unnecessary details.
Sample Code: Abstraction
from abc import ABC, abstractmethod
class Animal(ABC):
@abstractmethod
def make_sound(self):
pass
class Dog(Animal):
def make_sound(self):
return "Woof!"
class Cat(Animal):
def make_sound(self):
return "Meow!"
# Creating objects of Dog and Cat classes
dog = Dog()
cat = Cat()
print(dog.make_sound())
print(cat.make_sound())
In this example, Animal
is an abstract class with an abstract method make_sound
. The Dog
and Cat
classes inherit from Animal
and provide concrete implementations of the make_sound
method. This abstraction hides the implementation details and shows only the necessary functionality.
Inheritance
Inheritance allows a new class to inherit properties and behavior from an existing class. This promotes code reuse and establishes a natural hierarchy between classes. The class that inherits is called a subclass, and the class being inherited from is called a superclass.
Sample Code: Inheritance
class Vehicle:
def __init__(self, brand, model):
self.brand = brand
self.model = model
def start(self):
return f"{self.brand} {self.model} is starting."
class Car(Vehicle):
def __init__(self, brand, model, doors):
super().__init__(brand, model)
self.doors = doors
def open_doors(self):
return f"Opening {self.doors} doors of the {self.brand} {self.model}."
# Creating an object of the Car class
my_car = Car("Toyota", "Camry", 4)
print(my_car.start())
print(my_car.open_doors())
In this example, the Car
class inherits from the Vehicle
class. The Car
class can use the properties and methods of the Vehicle
class and also define its own.
Polymorphism
Polymorphism allows methods to do different things based on the object it is acting upon, even though they share the same name. This is achieved through method overriding and interfaces, promoting flexibility and integration in OOP.
Sample Code: Polymorphism
class Bird:
def fly(self):
return "Most birds can fly."
class Penguin(Bird):
def fly(self):
return "Penguins cannot fly."
def show_flying_ability(bird):
print(bird.fly())
# Creating objects of Bird and Penguin classes
sparrow = Bird()
penguin = Penguin()
show_flying_ability(sparrow)
show_flying_ability(penguin)
In this example, both Bird
and Penguin
classes have a fly
method. However, the Penguin
class overrides the fly
method to indicate that penguins cannot fly. The show_flying_ability
function demonstrates polymorphism by calling the appropriate fly
method based on the object passed to it.
Advantages of Object-Oriented Programming
OOP offers several advantages that make it a preferred programming paradigm for many developers:
- Modularity: OOP allows breaking down a program into smaller, manageable pieces. Each piece, or class, can be developed, tested, and debugged independently.
- Reusability: Once a class is written, it can be used multiple times in different programs. Inheritance allows extending existing classes to create new functionality without modifying the original class.
- Maintainability: OOP makes it easier to manage and update code. Changes in one part of the system can be made with minimal impact on other parts, thanks to encapsulation.
- Scalability: OOP provides a clear structure for programs, making it easier to manage large codebases and scale applications.
Practical Applications of OOP
OOP is widely used in various fields of software development, including:
- Web Development: Frameworks like Django (Python), Ruby on Rails (Ruby), and Laravel (PHP) use OOP principles to create scalable web applications.
- Game Development: Game engines like Unity and Unreal Engine are heavily based on OOP, allowing developers to create complex game systems with reusable components.
- Desktop Applications: GUI frameworks like PyQt (Python) and Swing (Java) use OOP to create interactive desktop applications.
- Data Science: Libraries like Pandas and TensorFlow use OOP to provide powerful tools for data manipulation and machine learning.
Getting Started with OOP
To get started with OOP, it’s essential to choose a programming language that supports OOP concepts. Popular OOP languages include Python, Java, C++, and Ruby. Each of these languages has extensive documentation and community support, making it easier to learn and apply OOP principles.
Sample Code: A Complete Example
class Person:
def __init__(self, name, age):
self.name = name
self.age = age
def greet(self):
return f"Hello, my name is {self.name} and I am {self.age} years old."
class Student(Person):
def __init__(self, name, age, student_id):
super().__init__(name, age)
self.student_id = student_id
def study(self):
return f"{self.name} is studying."
class Teacher(Person):
def __init__(self, name, age, subject):
super().__init__(name, age)
self.subject = subject
def teach(self):
return f"{self.name} is teaching {self.subject}."
# Creating objects of Student and Teacher classes
student = Student("Alice", 20, "S12345")
teacher = Teacher("Mr. Smith", 40, "Mathematics")
print(student.greet())
print(student.study())
print(teacher.greet())
print(teacher.teach())
In this complete example, the Person
class is the base class, while Student
and Teacher
are subclasses that inherit from Person
. This demonstrates how inheritance and polymorphism can be used to create a flexible and reusable code structure.
Object-Oriented Programming is a powerful paradigm that provides a structured approach to software development. By organizing code into objects and classes, OOP promotes modularity, reusability, and maintainability. Understanding and applying the core principles of encapsulation, abstraction, inheritance, and polymorphism can significantly enhance your programming skills. Whether you’re developing web applications, games