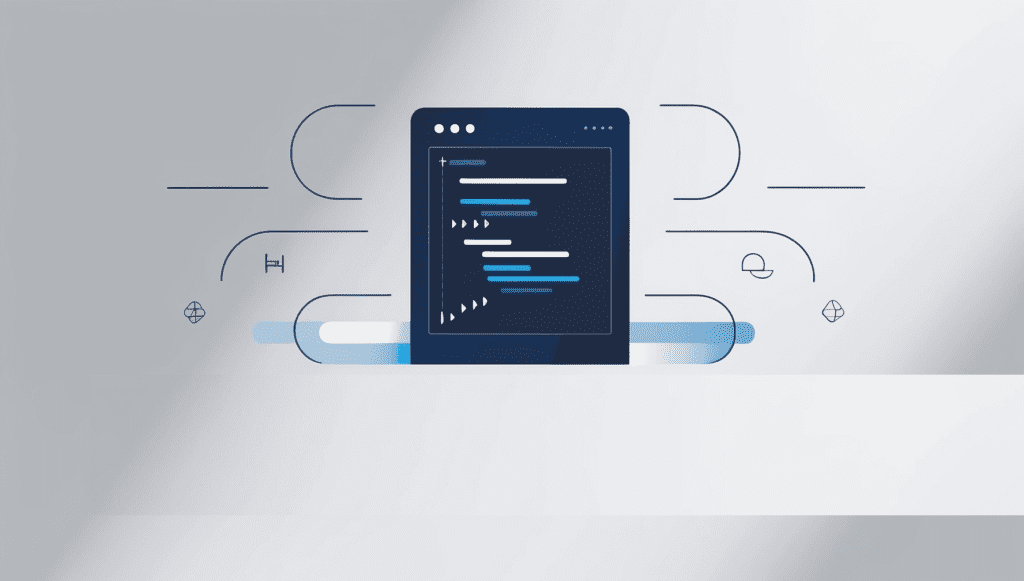
Java Exception Handling Best Practices – Effective Error Handling and Recovery
Handling exceptions effectively is essential for creating robust Java applications. Many Java developers, especially at intermediate and advanced levels, face common challenges when implementing error handling strategies, from managing unexpected behaviors to ensuring graceful recovery. This guide will walk through key best practices in Java exception handling, discussing how to avoid pitfalls, implement custom exceptions, and structure recovery strategies that improve code reliability.
Why Effective Exception Handling is Essential
In Java, exceptions signify issues that disrupt normal execution. While exceptions are unavoidable, the way we handle them defines application stability, maintainability, and user experience. Poor exception handling leads to unmanageable code, application crashes, and missed opportunities for recovery. Effective handling, on the other hand, improves error visibility and helps maintain application flow.
Understanding Java Exception Types
Java organizes exceptions into a hierarchy with checked, unchecked, and errors as the three primary types. Each serves a different purpose and affects how errors are managed.
Exception Type | Description | Examples |
---|---|---|
Checked Exception | Must be caught or declared in the method. | IOException , SQLException |
Unchecked Exception | Can be handled optionally by the developer. | NullPointerException , ArithmeticException |
Error | Critical issues that often terminate the app. | OutOfMemoryError , StackOverflowError |
Best Practices for Java Exception Handling
1. Use Specific Exception Types
Using specific exceptions helps clearly indicate the cause of an issue. Avoid Exception
and Throwable
in catch
blocks, as they capture all exception types, making it difficult to identify exact problems.
try {
// code that may throw exceptions
} catch (FileNotFoundException e) {
// Handle specific file not found case
} catch (IOException e) {
// Handle other I/O exceptions
}
Specific exception types offer more granular control, allowing distinct error messages and recovery strategies.
2. Avoid Swallowing Exceptions
Swallowing exceptions without any handling is a common anti-pattern in Java. Silent failures make debugging challenging, as there’s no feedback on what went wrong.
Anti-pattern:
try {
// Some code that might throw an exception
} catch (Exception e) {
// Do nothing
}
Best Practice:
try {
// Some code that might throw an exception
} catch (Exception e) {
System.err.println("Error occurred: " + e.getMessage());
e.printStackTrace(); // Use logging instead in production code
}
Always log exceptions at a minimum to ensure you have visibility into runtime issues.
3. Avoid Overusing Checked Exceptions
Checked exceptions force the caller to handle them, which can lead to cluttered code. Only use checked exceptions when the calling code can actually take action to recover from the failure. Otherwise, consider unchecked exceptions for programming errors or unexpected conditions.
Example:
public void loadFile(String fileName) throws IOException {
if (fileName == null) {
throw new IllegalArgumentException("Filename cannot be null"); // Unchecked exception
}
// Load file logic
}
4. Use Custom Exceptions to Improve Clarity
Custom exceptions allow you to define more meaningful error messages and track specific error cases in your application.
Creating a Custom Exception:
public class InvalidUserInputException extends RuntimeException {
public InvalidUserInputException(String message) {
super(message);
}
}
Using a Custom Exception:
public void processUserInput(String input) {
if (input == null || input.isEmpty()) {
throw new InvalidUserInputException("Input cannot be null or empty");
}
}
Custom exceptions help isolate and identify business logic errors distinct from system-level issues.
5. Leverage Finally Blocks for Resource Management
Use finally
blocks to release resources like files, network connections, or database handles, ensuring they close regardless of success or failure.
FileInputStream fileInputStream = null;
try {
fileInputStream = new FileInputStream("data.txt");
// Processing logic
} catch (IOException e) {
System.err.println("File handling error: " + e.getMessage());
} finally {
if (fileInputStream != null) {
try {
fileInputStream.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
Java 7 introduced the try-with-resources statement, making resource management simpler and less error-prone by automatically closing resources.
Advanced Exception Handling Techniques
1. Implementing Logging for Better Visibility
Logging is critical for production environments, as it provides visibility into exceptions without exposing stack traces to end-users.
import java.util.logging.Logger;
public class MyService {
private static final Logger logger = Logger.getLogger(MyService.class.getName());
public void executeService() {
try {
// Code that may throw an exception
} catch (CustomException e) {
logger.warning("Custom error encountered: " + e.getMessage());
} catch (Exception e) {
logger.severe("Unhandled exception: " + e.getMessage());
}
}
}
Effective logging captures critical information while maintaining security and performance. For large applications, structured logging frameworks like SLF4J or Logback offer extensive capabilities.
2. Using Exception Wrapping for Clarity
Exception wrapping, or chaining, enables you to catch a low-level exception and throw a higher-level exception, providing more context while preserving the original cause.
try {
// Code that might throw SQLException
} catch (SQLException e) {
throw new DataAccessException("Database access error", e);
}
Chaining exceptions with a cause parameter helps track root causes and avoid loss of context across layers.
Effective Error Recovery Strategies
1. Graceful Degradation and Fallbacks
In situations where a non-critical error occurs, allowing the application to continue in a degraded state or using a fallback can prevent total failure. This strategy is especially useful in applications where user experience should remain unaffected by certain failures.
public String fetchData() {
try {
// Attempt to fetch data from primary source
return primarySource.getData();
} catch (Exception e) {
System.err.println("Primary source failed, using fallback");
return fallbackSource.getData();
}
}
2. Retry Mechanisms for Temporary Failures
For operations prone to transient failures, such as network or database access, retrying after a delay can be effective. Implement retries with limits to avoid endless loops in case of persistent failures.
public void fetchWithRetry() {
int retryCount = 0;
while (retryCount < MAX_RETRIES) {
try {
// Code that may throw an exception
break; // Break if successful
} catch (TransientException e) {
retryCount++;
try {
Thread.sleep(1000); // Backoff before retrying
} catch (InterruptedException ie) {
Thread.currentThread().interrupt();
break;
}
}
}
}
The retry logic improves reliability but should be carefully managed to avoid excessive resource usage.
Avoiding Common Exception Handling Mistakes
Anti-Pattern | Explanation | Example |
---|---|---|
Swallowing exceptions | Ignoring exceptions without logging or notifying. | catch (Exception e) { } |
Overusing checked exceptions | Declaring checked exceptions unnecessarily, leading to verbose code. | public void myMethod() throws Exception |
Catching Throwable or Error | Handling severe system issues that should not be recovered from. | catch (Throwable t) |
Relying on error codes | Using error codes instead of exceptions, making the code harder to read and maintain. | return -1; // for error |
Logging and rethrowing without context | Logging and rethrowing exceptions without adding context, causing redundant logs and losing information. | catch (IOException e) { logger.log(e); throw e; } |
Correcting these common errors leads to cleaner, more maintainable exception handling.
Conclusion
Effective exception handling is essential for creating resilient, maintainable Java applications. Following best practices such as using specific exceptions, leveraging custom exceptions, and implementing recovery mechanisms like retries and fallbacks ensures that errors are managed gracefully without compromising application stability.
Implementing these Java exception handling best practices allows you to build applications that are both robust and maintainable, ultimately enhancing user experience and reducing downtime.
This blog provides general guidance on Java exception handling best practices. If any inaccuracies are found, please reach out so we can address them promptly.