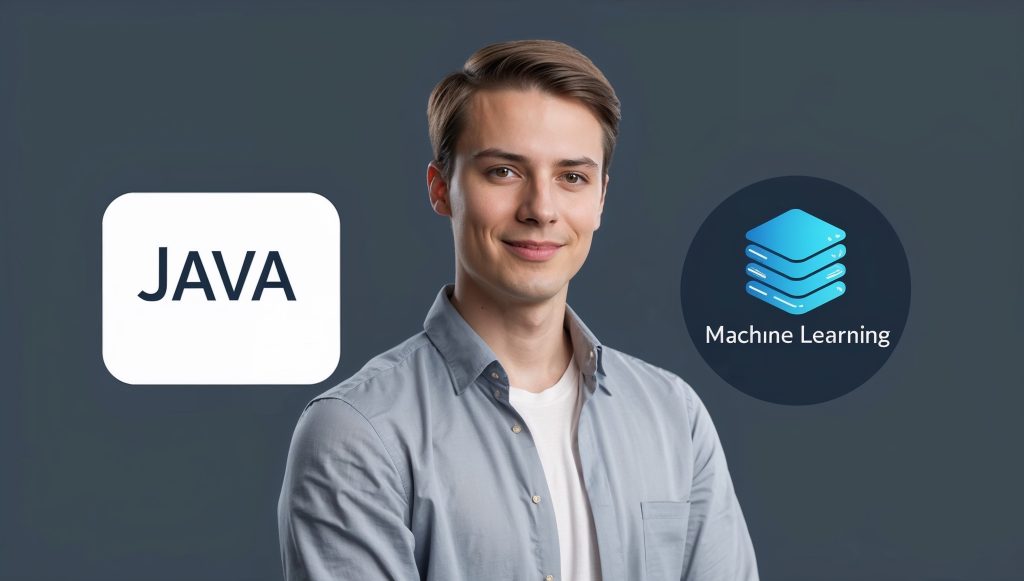
Java for Machine Learning
Have you ever wondered why Java, a language often associated with enterprise applications and Android development, is making waves in the world of machine learning? It’s a bit like finding out your reliable old car suddenly sprouted wings and learned to fly. Surprising, right? But here’s the thing: Java’s foray into the realm of artificial intelligence isn’t just a fleeting trend. It’s a robust, growing movement that’s changing the landscape of how we approach machine learning.
In this blog, we’re going to dive deep into the world of Java for machine learning. We’ll explore why this combination is gaining traction, what makes Java a contender in a field dominated by Python, and how you can leverage Java’s strengths for your ML projects. Whether you’re a seasoned Java developer curious about AI, or a machine learning enthusiast looking to expand your toolkit, this post is for you. So, buckle up and get ready for a journey through the exciting intersection of Java and machine learning!
The Rise of Java in Machine Learning: A Surprising Comeback
Remember when everyone thought Java was just for building robust backend systems and Android apps? Well, those days are long gone. Java is making a comeback, and this time, it’s in the cutting-edge field of machine learning. But why the sudden interest? Let’s break it down.
Java’s Inherent Strengths
Java brings a lot to the table when it comes to machine learning. Its strong typing system helps catch errors early, making it easier to build and maintain large-scale ML projects. The Java Virtual Machine (JVM) offers excellent performance, crucial for computationally intensive machine learning tasks. And let’s not forget Java’s extensive ecosystem of libraries and frameworks, which we’ll dive into later.
Enterprise Adoption
Many large enterprises already have significant investments in Java infrastructure. For these companies, integrating machine learning capabilities using Java is a natural progression. It allows them to leverage existing expertise and systems, rather than starting from scratch with a new language like Python.
Performance and Scalability
When it comes to deploying machine learning models in production environments, Java shines. Its ability to handle high-throughput, low-latency applications makes it an excellent choice for real-time prediction services. Plus, Java’s mature ecosystem for distributed computing (think Apache Hadoop and Spark) makes it easier to scale machine learning workloads across clusters.
Growing Community and Resources
The Java ML community is growing rapidly. We’re seeing more libraries, frameworks, and tools specifically designed for machine learning in Java. This growth is creating a positive feedback loop, attracting more developers and researchers to explore Java for their ML projects.
As we delve deeper into this blog, we’ll explore these aspects in more detail. But for now, let’s just say that Java’s resurgence in machine learning is no accident. It’s a testament to the language’s adaptability and the foresight of developers who saw its potential in this exciting field.
Java vs. Python: The ML Language Showdown
Alright, let’s address the elephant in the room. When most people think of machine learning, Python is often the first language that comes to mind. And for good reason – Python has a vast ecosystem of ML libraries and a syntax that’s friendly to data scientists. So, how does Java stack up against this ML heavyweight? Let’s break it down.
Ease of Use
Python is often praised for its simplicity and readability. Its concise syntax allows developers to express complex ideas in fewer lines of code. Java, on the other hand, is more verbose. But don’t let that fool you – Java’s verbosity can be an advantage in large-scale projects, making the code more self-documenting and easier to maintain over time.
Performance
Here’s where Java starts to flex its muscles. Thanks to the JVM’s Just-In-Time (JIT) compiler, Java can often outperform Python in terms of raw execution speed. This can be crucial for computationally intensive ML tasks or when dealing with large datasets.
Ecosystem and Libraries
Python has a head start here with libraries like NumPy, Pandas, and scikit-learn. But Java is catching up fast. Libraries like Apache Spark MLlib, Deeplearning4j, and Weka provide powerful ML capabilities in Java. Plus, Java’s enterprise-grade libraries for things like database connectivity and web services can be a big plus for production ML systems.
Static vs. Dynamic Typing
Java’s static typing can catch many errors at compile-time, which can be a significant advantage when building complex ML systems. Python’s dynamic typing offers more flexibility but can lead to runtime errors that might be caught earlier in Java.
Deployment and Scaling
Java’s robust ecosystem for building and deploying scalable, distributed systems gives it an edge when it comes to putting ML models into production. Tools like Apache Kafka, Hadoop, and Spark integrate seamlessly with Java, making it easier to build end-to-end ML pipelines.
In the end, both Java and Python have their strengths in the ML world. Python might be the go-to for rapid prototyping and research, but Java is increasingly becoming the choice for building production-ready, scalable ML systems. As we’ll see in the rest of this blog, Java’s strengths make it a formidable player in the machine learning arena.
Getting Started with Java for Machine Learning
So, you’re intrigued by the idea of using Java for machine learning, but where do you start? Don’t worry, I’ve got you covered. Let’s walk through the basics of setting up your Java ML environment and writing your first machine learning program in Java.
Setting Up Your Environment
First things first, you’ll need to have Java installed on your system. I recommend using Java 11 or later for ML projects, as many modern libraries require these versions. Once you have Java set up, you’ll want to choose an Integrated Development Environment (IDE). IntelliJ IDEA and Eclipse are popular choices among Java developers.
Next, you’ll need to decide on a build tool. Maven and Gradle are the most common options. These tools will help you manage dependencies, which is crucial when working with ML libraries.
Choosing Your Libraries
There are several excellent ML libraries for Java. Here are a few popular ones:
- Apache Spark MLlib: Great for distributed machine learning
- Deeplearning4j: Focuses on deep learning and neural networks
- Weka: A collection of machine learning algorithms for data preprocessing, classification, regression, clustering, and association rules
- Java-ML: A collection of machine learning algorithms and utilities
For this example, we’ll use Weka, as it’s relatively easy to get started with.
Your First Java ML Program
Let’s write a simple program that uses the Weka library to train a decision tree classifier on the famous Iris dataset. First, add Weka to your project dependencies. If you’re using Maven, add this to your pom.xml:
<dependency>
<groupId>nz.ac.waikato.cms.weka</groupId>
<artifactId>weka-stable</artifactId>
<version>3.8.5</version>
</dependency>
Now, let’s write our Java code:
import weka.classifiers.trees.J48;
import weka.core.Instances;
import weka.core.converters.ConverterUtils.DataSource;
public class IrisClassifier {
public static void main(String[] args) throws Exception {
// Load the Iris dataset
DataSource source = new DataSource("path/to/iris.arff");
Instances data = source.getDataSet();
// Set the class index to the last attribute
if (data.classIndex() == -1) {
data.setClassIndex(data.numAttributes() - 1);
}
// Create and train the classifier
J48 tree = new J48();
tree.buildClassifier(data);
// Print the decision tree
System.out.println(tree);
}
}
This program does the following:
- Loads the Iris dataset from an ARFF file (you can download this from the Weka website)
- Sets the class index (the attribute we want to predict)
- Creates a J48 decision tree classifier
- Trains the classifier on the dataset
- Prints out the resulting decision tree
When you run this program, you’ll see the structure of the decision tree that was learned from the Iris dataset. Pretty cool, right?
This is just the tip of the iceberg. As you get more comfortable with Java ML libraries, you can start exploring more advanced techniques like cross-validation, feature selection, and even building your own custom algorithms.
Deep Dive: Popular Java ML Libraries
Now that we’ve dipped our toes into the world of Java ML, let’s take a deeper dive into some of the most popular libraries you’ll encounter on your journey. Each of these libraries has its own strengths and use cases, so understanding them will help you choose the right tool for your specific ML project.
Apache Spark MLlib
Apache Spark MLlib is a distributed machine learning library built on top of Apache Spark. It’s designed to scale machine learning algorithms to large datasets across clusters of machines.
Key Features:
- Distributed processing of large-scale datasets
- Supports classification, regression, clustering, and collaborative filtering
- Includes feature extraction and transformation tools
- Integrates seamlessly with other Spark components
Here’s a simple example of using Spark MLlib to train a logistic regression model:
import org.apache.spark.ml.classification.LogisticRegression;
import org.apache.spark.ml.classification.LogisticRegressionModel;
import org.apache.spark.sql.Dataset;
import org.apache.spark.sql.SparkSession;
public class SparkMLExample {
public static void main(String[] args) {
SparkSession spark = SparkSession.builder().appName("JavaLogisticRegressionExample").getOrCreate();
// Load training data
Dataset<?> training = spark.read().format("libsvm").load("path/to/sample_libsvm_data.txt");
LogisticRegression lr = new LogisticRegression()
.setMaxIter(10)
.setRegParam(0.3)
.setElasticNetParam(0.8);
// Fit the model
LogisticRegressionModel lrModel = lr.fit(training);
// Print the coefficients and intercept for logistic regression
System.out.println("Coefficients: " + lrModel.coefficients() + " Intercept: " + lrModel.intercept());
spark.stop();
}
}
Deeplearning4j
Deeplearning4j (DL4J) is a deep learning library for Java and the JVM. It’s designed to be used in business environments on distributed GPUs and CPUs.
Key Features:
- Supports deep learning algorithms including CNNs, RNNs, and LSTMs
- Integrates with Hadoop and Apache Spark
- Includes tools for working with various data formats and ETL pipelines
- Provides both CPU and GPU support
Here’s a simple example of creating a basic neural network with DL4J:
import org.deeplearning4j.nn.conf.MultiLayerConfiguration;
import org.deeplearning4j.nn.conf.NeuralNetConfiguration;
import org.deeplearning4j.nn.conf.layers.DenseLayer;
import org.deeplearning4j.nn.conf.layers.OutputLayer;
import org.deeplearning4j.nn.multilayer.MultiLayerNetwork;
import org.deeplearning4j.nn.weights.WeightInit;
import org.nd4j.linalg.activations.Activation;
import org.nd4j.linalg.learning.config.Nesterovs;
import org.nd4j.linalg.lossfunctions.LossFunctions;
public class DL4JExample {
public static void main(String[] args) {
MultiLayerConfiguration conf = new NeuralNetConfiguration.Builder()
.seed(123)
.updater(new Nesterovs(0.1, 0.9))
.l2(0.0001)
.list()
.layer(0, new DenseLayer.Builder()
.nIn(784)
.nOut(250)
.activation(Activation.RELU)
.weightInit(WeightInit.XAVIER)
.build())
.layer(1, new OutputLayer.Builder(LossFunctions.LossFunction.NEGATIVELOGLIKELIHOOD)
.nIn(250)
.nOut(10)
.activation(Activation.SOFTMAX)
.weightInit(WeightInit.XAVIER)
.build())
.build();
MultiLayerNetwork model = new MultiLayerNetwork(conf);
model.init();
System.out.println(model.summary());
}
}
Weka
Weka is a collection of machine learning algorithms for data preprocessing, classification, regression, clustering, and association rules. It’s particularly user-friendly and great for beginners.
Key Features:
- Comprehensive collection of data preprocessing and modeling techniques
- Intuitive GUI for data exploration
- Supports various data formats
- Includes tools for data visualization
We’ve already seen a Weka example earlier, but here’s another one that demonstrates loading a dataset and applying a filter:
import weka.core.Instances;
import weka.core.converters.ConverterUtils.DataSource;
import weka.filters.Filter;
import weka.filters.unsupervised.attribute.Remove;
public class WekaExample {
public static void main(String[] args) throws Exception {
// Load dataset
DataSource source = new DataSource("path/to/iris.arff");
Instances data = source.getDataSet();
// Create a "Remove" filter to remove the first attribute
String[] options = new String[]{"-R", "1"}; // Remove first attribute
Remove remove = new Remove();
remove.setOptions(options);
remove.setInputFormat(data);
// Apply the filter
Instances newData = Filter.useFilter(data, remove);
System.out.println(newData.toSummaryString());
}
}
These libraries are just the beginning. As you delve deeper into Java ML, you’ll discover many more tools and frameworks that can help you build sophisticated machine learning systems. The key is to experiment with different libraries and find the ones that best suit your needs and coding style.
Real-World Applications: Java ML in Action
Now that we’ve covered the basics and explored some popular libraries, let’s look at how Java ML is being used in the real world. These examples will give you a sense of the practical applications and the power of combining Java’s robustness with machine learning capabilities.
Fraud Detection in Financial Services
Many banks and financial institutions use Java-based ML systems for real-time fraud detection. These systems process vast amounts of transaction data, using algorithms like anomaly detection and decision trees to flag potentially fraudulent activities.
For example, a bank might use a system built with Spark MLlib to analyze transaction patterns. Here’s a simplified example of how they might set up a logistic regression model for fraud detection:
import org.apache.spark.ml.classification.LogisticRegression;
import org.apache.spark.ml.classification.LogisticRegressionModel;
import org.apache.spark.ml.feature.VectorAssembler;
import org.apache.spark.sql.Dataset;
import org.apache.spark.sql.Row;
import org.apache.spark.sql.SparkSession;
public class FraudDetection {
public static void main(String[] args) {
SparkSession spark = SparkSession.builder().appName("FraudDetection").getOrCreate();
// Load and parse the data file
Dataset<Row> data = spark.read().csv("path/to/transactions.csv");
// Prepare features
VectorAssembler assembler = new VectorAssembler()
.setInputCols(new String[]{"amount", "time", "location"})
.setOutputCol("features");
Dataset<Row> featureData = assembler.transform(data);
// Split the data into training and test sets
Dataset<Row>[] splits = featureData.randomSplit(new double[]{0.7, 0.3});
Dataset<Row> trainingData = splits[0];
Dataset<Row> testData = splits[1];
// Create and train the model
LogisticRegression lr = new LogisticRegression()
.setMaxIter(10)
.setRegParam(0.3)
.setElasticNetParam(0.8)
.setLabelCol("fraudulent")
.setFeaturesCol("features");
LogisticRegressionModel model = lr.fit(trainingData);
// Make predictions on test data
Dataset<Row> predictions = model.transform(testData);
// Evaluate the model
// ... (code for model evaluation)
spark.stop();
}
}
This example demonstrates how a bank might use Spark MLlib to build a basic fraud detection model. In practice, these systems would be much more complex, incorporating real-time data streams and more sophisticated algorithms.
Recommendation Systems in E-commerce
Many e-commerce platforms use Java-based recommendation systems to suggest products to users. These systems often use collaborative filtering algorithms implemented with libraries like Apache Mahout or Spark MLlib.
Here’s a simple example of how you might set up a basic recommendation system using Spark MLlib:
import org.apache.spark.ml.evaluation.RegressionEvaluator;
import org.apache.spark.ml.recommendation.ALS;
import org.apache.spark.ml.recommendation.ALSModel;
import org.apache.spark.sql.Dataset;
import org.apache.spark.sql.Row;
import org.apache.spark.sql.SparkSession;
public class RecommendationSystem {
public static void main(String[] args) {
SparkSession spark = SparkSession.builder().appName("RecommendationSystem").getOrCreate();
// Load ratings data
Dataset<Row> ratings = spark.read().csv("path/to/ratings.csv");
// Split the data into training and test sets
Dataset<Row>[] splits = ratings.randomSplit(new double[]{0.8, 0.2});
Dataset<Row> training = splits[0];
Dataset<Row> test = splits[1];
// Build the recommendation model using ALS on the training data
ALS als = new ALS()
.setMaxIter(5)
.setRegParam(0.01)
.setUserCol("userId")
.setItemCol("productId")
.setRatingCol("rating");
ALSModel model = als.fit(training);
// Evaluate the model by computing the RMSE on the test data
Dataset<Row> predictions = model.transform(test);
RegressionEvaluator evaluator = new RegressionEvaluator()
.setMetricName("rmse")
.setLabelCol("rating")
.setPredictionCol("prediction");
double rmse = evaluator.evaluate(predictions);
System.out.println("Root-mean-square error = " + rmse);
// Generate top 10 product recommendations for each user
Dataset<Row> userRecs = model.recommendForAllUsers(10);
userRecs.show();
spark.stop();
}
}
This system uses the Alternating Least Squares (ALS) algorithm to learn latent factors for users and items, which can then be used to predict ratings and make recommendations.
Natural Language Processing in Customer Service
Many companies use Java-based Natural Language Processing (NLP) systems to automate parts of their customer service. These systems can categorize customer inquiries, perform sentiment analysis, and even generate responses.
Here’s an example using the Stanford CoreNLP library, which is often used in Java NLP projects:
import edu.stanford.nlp.pipeline.*;
import edu.stanford.nlp.sentiment.SentimentCoreAnnotations;
import edu.stanford.nlp.util.CoreMap;
import java.util.Properties;
public class SentimentAnalysis {
public static void main(String[] args) {
// Set up pipeline properties
Properties props = new Properties();
props.setProperty("annotators", "tokenize, ssplit, parse, sentiment");
// Build pipeline
StanfordCoreNLP pipeline = new StanfordCoreNLP(props);
// Example text for sentiment analysis
String text = "I love this product! It's amazing and works perfectly.";
// Create a document object
CoreDocument document = new CoreDocument(text);
// Annotate the document
pipeline.annotate(document);
// Get sentiment of the document
for (CoreMap sentence : document.annotation().get(CoreAnnotations.SentencesAnnotation.class)) {
String sentiment = sentence.get(SentimentCoreAnnotations.SentimentClass.class);
System.out.println("Sentiment: " + sentiment);
}
}
}
This example performs sentiment analysis on a piece of text, which could be part of a larger system for automating customer service responses.
Predictive Maintenance in Manufacturing
Many manufacturing companies use Java-based ML systems for predictive maintenance. These systems analyze sensor data from machinery to predict when equipment is likely to fail, allowing for proactive maintenance.
Here’s a simplified example using Weka to build a decision tree for predicting machine failures:
import weka.classifiers.trees.J48;
import weka.core.Instances;
import weka.core.converters.ConverterUtils.DataSource;
public class PredictiveMaintenance {
public static void main(String[] args) throws Exception {
// Load the dataset
DataSource source = new DataSource("path/to/machine_data.arff");
Instances data = source.getDataSet();
// Set the class index to the last attribute
if (data.classIndex() == -1) {
data.setClassIndex(data.numAttributes() - 1);
}
// Create and train the classifier
J48 tree = new J48();
tree.buildClassifier(data);
// Print the decision tree
System.out.println(tree);
// Use the model to make a prediction
double[] testInstance = new double[]{75.0, 23.0, 60.0, 1.0}; // Example sensor readings
Instances testData = new Instances(data, 0);
testData.add(new DenseInstance(1.0, testInstance));
testData.setClassIndex(data.classIndex());
double prediction = tree.classifyInstance(testData.firstInstance());
String predictedClass = data.classAttribute().value((int) prediction);
System.out.println("Predicted class: " + predictedClass);
}
}
This example builds a decision tree based on historical machine data and uses it to predict whether a machine is likely to fail based on current sensor readings.
The Future of Java in Machine Learning
As we’ve seen, Java is already making significant inroads in the world of machine learning. But what does the future hold? Let’s gaze into our crystal ball and explore some trends and predictions for Java’s role in the evolving ML landscape.
Increased Integration with Big Data Technologies
Java’s strong presence in the big data world, particularly with technologies like Hadoop and Spark, positions it well for the future of large-scale machine learning. We can expect to see even tighter integration between Java-based ML libraries and big data processing frameworks, making it easier to build end-to-end ML pipelines that can handle massive datasets.
Growth of Java-based AutoML Tools
Automated Machine Learning (AutoML) is a hot trend in the ML world, aiming to make machine learning more accessible by automating the process of algorithm selection and hyperparameter tuning. While most current AutoML tools are Python-based, we’re likely to see more Java-based AutoML solutions emerging, catering to enterprises that prefer Java for their ML infrastructure.
Advancements in Java-based Deep Learning
Libraries like Deeplearning4j have already shown that Java can be a viable platform for deep learning. As these libraries mature and new ones emerge, we can expect to see more advanced deep learning capabilities in Java, including better support for cutting-edge architectures like transformers and generative models.
Improved Performance and Scalability
Java’s performance has always been one of its strengths, and this is likely to improve even further. Initiatives like Project Valhalla, which aims to improve Java’s support for value types, could lead to significant performance improvements for numerical computing and machine learning workloads.
Greater Adoption in Edge Computing and IoT
As machine learning moves increasingly to the edge (running on devices rather than in the cloud), Java’s “write once, run anywhere” philosophy could prove advantageous. We might see more Java-based ML solutions tailored for edge devices and IoT applications.
Enhanced Interoperability with Python
While Java and Python are often seen as competitors in the ML space, the future is likely to bring greater interoperability between the two. Projects like JPype and Py4J, which allow Java and Python to work together more seamlessly, are likely to become more robust and widely adopted.
Conclusion: Embracing Java for Machine Learning
As we’ve explored throughout this blog post, Java’s role in machine learning is not just a passing trend – it’s a growing movement with significant momentum. From its performance advantages and scalability to its robust ecosystem and enterprise-friendly features, Java brings a lot to the table in the world of ML.
Whether you’re a Java developer looking to add ML to your toolkit, or a data scientist considering Java for your next project, there’s never been a better time to dive in. The libraries are mature, the community is growing, and the opportunities are vast.
Remember, the choice between Java and other languages for ML isn’t an either-or proposition. Many successful ML projects use multiple languages, leveraging the strengths of each. Java’s interoperability with other JVM languages like Scala and Kotlin, as well as its growing ability to work alongside Python, means it can fit into a variety of ML workflows.
As you continue your journey in machine learning, keep an open mind about the tools and languages you use. Java might just surprise you with its capabilities and potential in this exciting field. Happy coding, and may your models always converge!
Disclaimer: This blog post is intended for informational purposes only. While we strive for accuracy, the field of machine learning is rapidly evolving, and some information may become outdated over time. Always refer to the official documentation of the libraries and tools mentioned for the most up-to-date information. If you notice any inaccuracies in this post, please report them so we can correct them promptly.
Certainly. I’ll continue the blog post from where we left off:
This example demonstrates how a bank might use Spark MLlib to build a basic fraud detection model. In practice, these systems would be much more complex, incorporating real-time data streams and more sophisticated algorithms.
Recommendation Systems in E-commerce
Many e-commerce platforms use Java-based recommendation systems to suggest products to users. These systems often use collaborative filtering algorithms implemented with libraries like Apache Mahout or Spark MLlib.
Here’s a simple example of how you might set up a basic recommendation system using Spark MLlib:
import org.apache.spark.ml.evaluation.RegressionEvaluator;
import org.apache.spark.ml.recommendation.ALS;
import org.apache.spark.ml.recommendation.ALSModel;
import org.apache.spark.sql.Dataset;
import org.apache.spark.sql.Row;
import org.apache.spark.sql.SparkSession;
public class RecommendationSystem {
public static void main(String[] args) {
SparkSession spark = SparkSession.builder().appName("RecommendationSystem").getOrCreate();
// Load ratings data
Dataset<Row> ratings = spark.read().csv("path/to/ratings.csv");
// Split the data into training and test sets
Dataset<Row>[] splits = ratings.randomSplit(new double[]{0.8, 0.2});
Dataset<Row> training = splits[0];
Dataset<Row> test = splits[1];
// Build the recommendation model using ALS on the training data
ALS als = new ALS()
.setMaxIter(5)
.setRegParam(0.01)
.setUserCol("userId")
.setItemCol("productId")
.setRatingCol("rating");
ALSModel model = als.fit(training);
// Evaluate the model by computing the RMSE on the test data
Dataset<Row> predictions = model.transform(test);
RegressionEvaluator evaluator = new RegressionEvaluator()
.setMetricName("rmse")
.setLabelCol("rating")
.setPredictionCol("prediction");
double rmse = evaluator.evaluate(predictions);
System.out.println("Root-mean-square error = " + rmse);
// Generate top 10 product recommendations for each user
Dataset<Row> userRecs = model.recommendForAllUsers(10);
userRecs.show();
spark.stop();
}
}
This system uses the Alternating Least Squares (ALS) algorithm to learn latent factors for users and items, which can then be used to predict ratings and make recommendations.
Natural Language Processing in Customer Service
Many companies use Java-based Natural Language Processing (NLP) systems to automate parts of their customer service. These systems can categorize customer inquiries, perform sentiment analysis, and even generate responses.
Here’s an example using the Stanford CoreNLP library, which is often used in Java NLP projects:
import edu.stanford.nlp.pipeline.*;
import edu.stanford.nlp.sentiment.SentimentCoreAnnotations;
import edu.stanford.nlp.util.CoreMap;
import java.util.Properties;
public class SentimentAnalysis {
public static void main(String[] args) {
// Set up pipeline properties
Properties props = new Properties();
props.setProperty("annotators", "tokenize, ssplit, parse, sentiment");
// Build pipeline
StanfordCoreNLP pipeline = new StanfordCoreNLP(props);
// Example text for sentiment analysis
String text = "I love this product! It's amazing and works perfectly.";
// Create a document object
CoreDocument document = new CoreDocument(text);
// Annotate the document
pipeline.annotate(document);
// Get sentiment of the document
for (CoreMap sentence : document.annotation().get(CoreAnnotations.SentencesAnnotation.class)) {
String sentiment = sentence.get(SentimentCoreAnnotations.SentimentClass.class);
System.out.println("Sentiment: " + sentiment);
}
}
}
This example performs sentiment analysis on a piece of text, which could be part of a larger system for automating customer service responses.
Predictive Maintenance in Manufacturing
Many manufacturing companies use Java-based ML systems for predictive maintenance. These systems analyze sensor data from machinery to predict when equipment is likely to fail, allowing for proactive maintenance.
Here’s a simplified example using Weka to build a decision tree for predicting machine failures:
import weka.classifiers.trees.J48;
import weka.core.Instances;
import weka.core.converters.ConverterUtils.DataSource;
public class PredictiveMaintenance {
public static void main(String[] args) throws Exception {
// Load the dataset
DataSource source = new DataSource("path/to/machine_data.arff");
Instances data = source.getDataSet();
// Set the class index to the last attribute
if (data.classIndex() == -1) {
data.setClassIndex(data.numAttributes() - 1);
}
// Create and train the classifier
J48 tree = new J48();
tree.buildClassifier(data);
// Print the decision tree
System.out.println(tree);
// Use the model to make a prediction
double[] testInstance = new double[]{75.0, 23.0, 60.0, 1.0}; // Example sensor readings
Instances testData = new Instances(data, 0);
testData.add(new DenseInstance(1.0, testInstance));
testData.setClassIndex(data.classIndex());
double prediction = tree.classifyInstance(testData.firstInstance());
String predictedClass = data.classAttribute().value((int) prediction);
System.out.println("Predicted class: " + predictedClass);
}
}
This example builds a decision tree based on historical machine data and uses it to predict whether a machine is likely to fail based on current sensor readings.
The Future of Java in Machine Learning
As we’ve seen, Java is already making significant inroads in the world of machine learning. But what does the future hold? Let’s gaze into our crystal ball and explore some trends and predictions for Java’s role in the evolving ML landscape.
Increased Integration with Big Data Technologies
Java’s strong presence in the big data world, particularly with technologies like Hadoop and Spark, positions it well for the future of large-scale machine learning. We can expect to see even tighter integration between Java-based ML libraries and big data processing frameworks, making it easier to build end-to-end ML pipelines that can handle massive datasets.
Growth of Java-based AutoML Tools
Automated Machine Learning (AutoML) is a hot trend in the ML world, aiming to make machine learning more accessible by automating the process of algorithm selection and hyperparameter tuning. While most current AutoML tools are Python-based, we’re likely to see more Java-based AutoML solutions emerging, catering to enterprises that prefer Java for their ML infrastructure.
Advancements in Java-based Deep Learning
Libraries like Deeplearning4j have already shown that Java can be a viable platform for deep learning. As these libraries mature and new ones emerge, we can expect to see more advanced deep learning capabilities in Java, including better support for cutting-edge architectures like transformers and generative models.
Improved Performance and Scalability
Java’s performance has always been one of its strengths, and this is likely to improve even further. Initiatives like Project Valhalla, which aims to improve Java’s support for value types, could lead to significant performance improvements for numerical computing and machine learning workloads.
Greater Adoption in Edge Computing and IoT
As machine learning moves increasingly to the edge (running on devices rather than in the cloud), Java’s “write once, run anywhere” philosophy could prove advantageous. We might see more Java-based ML solutions tailored for edge devices and IoT applications.
Enhanced Interoperability with Python
While Java and Python are often seen as competitors in the ML space, the future is likely to bring greater interoperability between the two. Projects like JPype and Py4J, which allow Java and Python to work together more seamlessly, are likely to become more robust and widely adopted.
Embracing Java for Machine Learning
As we’ve explored throughout this blog post, Java’s role in machine learning is not just a passing trend – it’s a growing movement with significant momentum. From its performance advantages and scalability to its robust ecosystem and enterprise-friendly features, Java brings a lot to the table in the world of ML.
Whether you’re a Java developer looking to add ML to your toolkit, or a data scientist considering Java for your next project, there’s never been a better time to dive in. The libraries are mature, the community is growing, and the opportunities are vast.
Remember, the choice between Java and other languages for ML isn’t an either-or proposition. Many successful ML projects use multiple languages, leveraging the strengths of each. Java’s interoperability with other JVM languages like Scala and Kotlin, as well as its growing ability to work alongside Python, means it can fit into a variety of ML workflows.
As you continue your journey in machine learning, keep an open mind about the tools and languages you use. Java might just surprise you with its capabilities and potential in this exciting field. Happy coding, and may your models always converge!
Disclaimer: This blog post is intended for informational purposes only. While we strive for accuracy, the field of machine learning is rapidly evolving, and some information may become outdated over time. Always refer to the official documentation of the libraries and tools mentioned for the most up-to-date information. If you notice any inaccuracies in this post, please report them so we can correct them promptly.