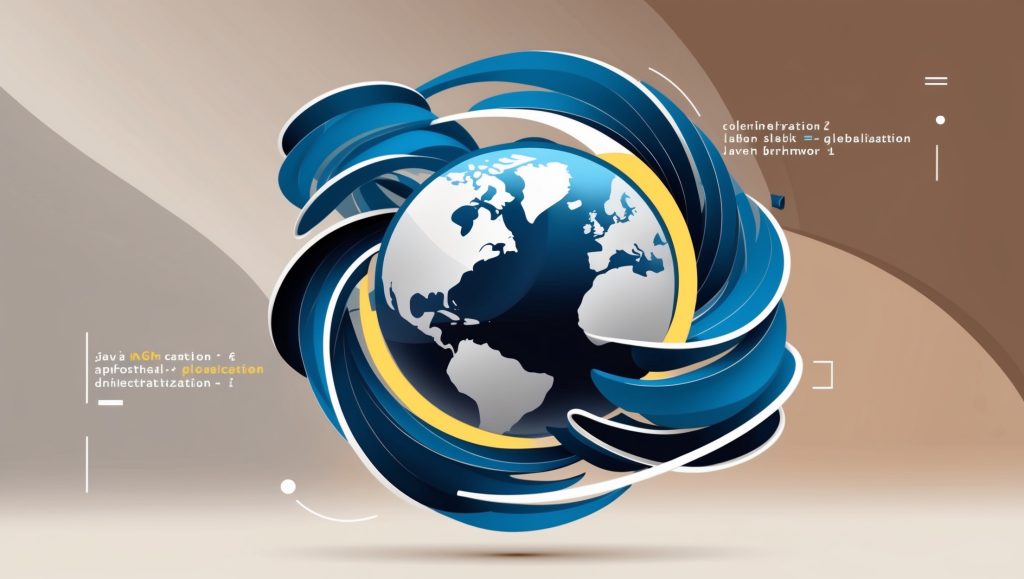
Java Internationalization – Building applications for global audiences
In today’s interconnected world, developing applications that can seamlessly adapt to different languages, regions, and cultural preferences is no longer optional – it’s a necessity. Java Internationalization, often abbreviated as i18n (where 18 represents the number of letters between ‘i’ and ‘n’), provides developers with robust tools and frameworks to create globally accessible applications. This comprehensive guide will explore the fundamental concepts, best practices, and practical implementations of Java Internationalization, helping you build applications that can reach users worldwide. We’ll dive deep into various aspects of i18n, from basic locale handling to complex date and number formatting, ensuring your applications are truly global-ready.
Understanding Java Internationalization Basics
What is Internationalization?
Internationalization is the process of designing and developing applications that can be adapted to various languages and regions without requiring engineering changes to the source code. It involves separating locale-specific elements from your code, such as text strings, date and time formats, currency notations, and number formats. Java provides a comprehensive framework through the java.util package, offering classes like Locale, ResourceBundle, and various formatters to handle these requirements efficiently. The goal is to create applications that can be easily localized for different regions while maintaining a single codebase.
Key Components of Java i18n
Java’s internationalization framework consists of several essential components that work together to provide a complete solution for building global applications. The Locale class serves as the foundation, representing a specific geographical, political, or cultural region. ResourceBundle manages locale-specific resources, while various formatter classes handle the proper display of numbers, dates, and currencies according to local conventions. These components are designed to work seamlessly together, providing a robust framework for creating globally accessible applications that respect local preferences and conventions.
Setting Up Locale in Java Applications
Understanding Locale Class
The Locale class is the cornerstone of Java internationalization. Here’s how to work with Locale:
// Creating Locale objects
Locale usLocale = new Locale("en", "US");
Locale frLocale = new Locale("fr", "FR");
Locale jpLocale = new Locale("ja", "JP");
// Getting default locale
Locale defaultLocale = Locale.getDefault();
// Getting available locales
Locale[] availableLocales = Locale.getAvailableLocales();
// Setting default locale
Locale.setDefault(new Locale("es", "ES"));
Locale Selection Strategy
Implementing a proper locale selection strategy is crucial for your application’s user experience. Here’s a common approach:
public class LocaleResolver {
public static Locale resolveLocale(String userPreference, String browserLocale) {
// Priority 1: User's explicit preference
if (userPreference != null && !userPreference.isEmpty()) {
return new Locale(userPreference);
}
// Priority 2: Browser locale
if (browserLocale != null && !browserLocale.isEmpty()) {
return new Locale(browserLocale);
}
// Priority 3: System default
return Locale.getDefault();
}
}
Resource Bundle Implementation
Creating and Managing Resource Bundles
Resource bundles are essential for managing locale-specific strings and resources. Here’s how to implement them effectively:
// messages_en_US.properties
welcome=Welcome to our application
greeting=Hello, {0}!
// messages_fr_FR.properties
welcome=Bienvenue dans notre application
greeting=Bonjour, {0}!
// Java implementation
public class ResourceBundleExample {
public static void main(String[] args) {
Locale locale = new Locale("fr", "FR");
ResourceBundle messages = ResourceBundle.getBundle("messages", locale);
String welcome = messages.getString("welcome");
String greeting = MessageFormat.format(
messages.getString("greeting"),
"John"
);
System.out.println(welcome);
System.out.println(greeting);
}
}
Best Practices for Resource Bundle Organization
Resource bundles should be organized in a clear and maintainable structure. Here’s a recommended approach:
src/
main/
resources/
i18n/
messages/
general_en_US.properties
general_fr_FR.properties
errors/
errors_en_US.properties
errors_fr_FR.properties
labels/
labels_en_US.properties
labels_fr_FR.properties
Date and Time Formatting
Using DateTimeFormatter
Java’s DateTimeFormatter provides powerful tools for handling date and time formatting across different locales:
public class DateTimeFormattingExample {
public static void main(String[] args) {
LocalDateTime now = LocalDateTime.now();
// Format date for different locales
Locale[] locales = {
new Locale("en", "US"),
new Locale("fr", "FR"),
new Locale("ja", "JP")
};
for (Locale locale : locales) {
DateTimeFormatter formatter = DateTimeFormatter
.ofLocalizedDateTime(FormatStyle.FULL)
.withLocale(locale);
System.out.println(locale.getDisplayName() + ": "
+ now.format(formatter));
}
}
}
Custom Date Patterns
Sometimes you need custom date patterns while maintaining locale sensitivity:
public class CustomDateFormatExample {
public static String formatDate(LocalDateTime date,
String pattern,
Locale locale) {
DateTimeFormatter formatter = DateTimeFormatter
.ofPattern(pattern, locale);
return date.format(formatter);
}
public static void main(String[] args) {
LocalDateTime now = LocalDateTime.now();
String pattern = "EEEE, MMMM d, yyyy 'at' HH:mm:ss";
System.out.println(formatDate(now, pattern,
new Locale("en", "US")));
System.out.println(formatDate(now, pattern,
new Locale("fr", "FR")));
}
}
Number and Currency Formatting
NumberFormat Implementation
Proper number formatting is crucial for international applications:
public class NumberFormatExample {
public static void main(String[] args) {
double number = 1234567.89;
Locale[] locales = {
new Locale("en", "US"),
new Locale("fr", "FR"),
new Locale("de", "DE")
};
for (Locale locale : locales) {
NumberFormat numberFormat =
NumberFormat.getNumberInstance(locale);
NumberFormat currencyFormat =
NumberFormat.getCurrencyInstance(locale);
System.out.println(locale.getDisplayName());
System.out.println("Number: " +
numberFormat.format(number));
System.out.println("Currency: " +
currencyFormat.format(number));
System.out.println();
}
}
}
Custom Number Formatting Patterns
For specific formatting requirements:
public class CustomNumberFormatExample {
public static void main(String[] args) {
double number = 1234567.89;
Locale locale = new Locale("fr", "FR");
DecimalFormat customFormat = (DecimalFormat)
NumberFormat.getNumberInstance(locale);
customFormat.applyPattern("#,###.## '€'");
System.out.println(customFormat.format(number));
}
}
Handling Text Direction and Bidirectional Text
Supporting RTL Languages
When supporting right-to-left (RTL) languages, consider the following practices:
public class BidiTextExample {
public static String getTextDirection(Locale locale) {
ComponentOrientation orientation =
ComponentOrientation.getOrientation(locale);
return orientation.isLeftToRight() ? "ltr" : "rtl";
}
public static void main(String[] args) {
Locale[] locales = {
new Locale("en", "US"), // LTR
new Locale("ar", "SA"), // RTL
new Locale("he", "IL") // RTL
};
for (Locale locale : locales) {
System.out.println(locale.getDisplayName() +
": " + getTextDirection(locale));
}
}
}
Testing Internationalization
Unit Testing i18n Implementation
Here’s an example of testing internationalization features:
public class I18nTest {
@Test
public void testResourceBundle() {
Locale.setDefault(new Locale("en", "US"));
ResourceBundle bundle =
ResourceBundle.getBundle("messages");
assertEquals("Welcome", bundle.getString("welcome"));
Locale.setDefault(new Locale("fr", "FR"));
bundle = ResourceBundle.getBundle("messages");
assertEquals("Bienvenue", bundle.getString("welcome"));
}
@Test
public void testDateFormatting() {
LocalDate date = LocalDate.of(2024, 1, 1);
DateTimeFormatter formatter =
DateTimeFormatter.ofLocalizedDate(FormatStyle.LONG);
assertEquals("January 1, 2024",
date.format(formatter.withLocale(
new Locale("en", "US"))));
assertEquals("1 janvier 2024",
date.format(formatter.withLocale(
new Locale("fr", "FR"))));
}
}
Best Practices and Common Pitfalls
Internationalization Guidelines
- Always externalize strings in resource bundles
- Use proper encoding (UTF-8) for all resource files
- Implement a fallback strategy for missing translations
- Consider cultural differences in colors, images, and symbols
- Test with various locales throughout development
-
Use appropriate data types for storing international text
Common Mistakes to Avoid
- Hardcoding strings in source code
- Assuming all languages read left-to-right
- Concatenating translated strings
- Ignoring cultural conventions for date and number formats
- Not considering text expansion in translations
- Using inappropriate character encodings
Performance Considerations
Resource Bundle Caching
Implement efficient resource bundle caching:
public class ResourceBundleCache {
private static final Map<Locale, ResourceBundle> cache =
new ConcurrentHashMap<>();
public static ResourceBundle getBundle(
String baseName, Locale locale) {
return cache.computeIfAbsent(locale, l ->
ResourceBundle.getBundle(baseName, l));
}
public static void clearCache() {
cache.clear();
}
}
Lazy Loading Strategies
Implement lazy loading for resource bundles:
public class LazyResourceLoader {
private static final Map<String, String> resources =
new ConcurrentHashMap<>();
public static String getString(
String key, Locale locale) {
String cacheKey = locale.toString() + "_" + key;
return resources.computeIfAbsent(cacheKey, k ->
loadString(key, locale));
}
private static String loadString(
String key, Locale locale) {
ResourceBundle bundle =
ResourceBundle.getBundle("messages", locale);
return bundle.getString(key);
}
}
Conclusion
Java Internationalization is a powerful framework that enables developers to create truly global applications. By following the best practices and implementations outlined in this guide, you can ensure your applications are accessible and user-friendly for audiences worldwide. Remember that internationalization should be considered from the project’s inception rather than as an afterthought. With proper planning and implementation, you can create applications that seamlessly adapt to various languages and cultural preferences while maintaining a single, manageable codebase.
Disclaimer: This blog post is intended for educational purposes and represents best practices as of November 2024. While we strive for accuracy, technologies and best practices evolve. Please verify critical information and test thoroughly in your specific environment. Report any inaccuracies to our technical team for prompt correction.