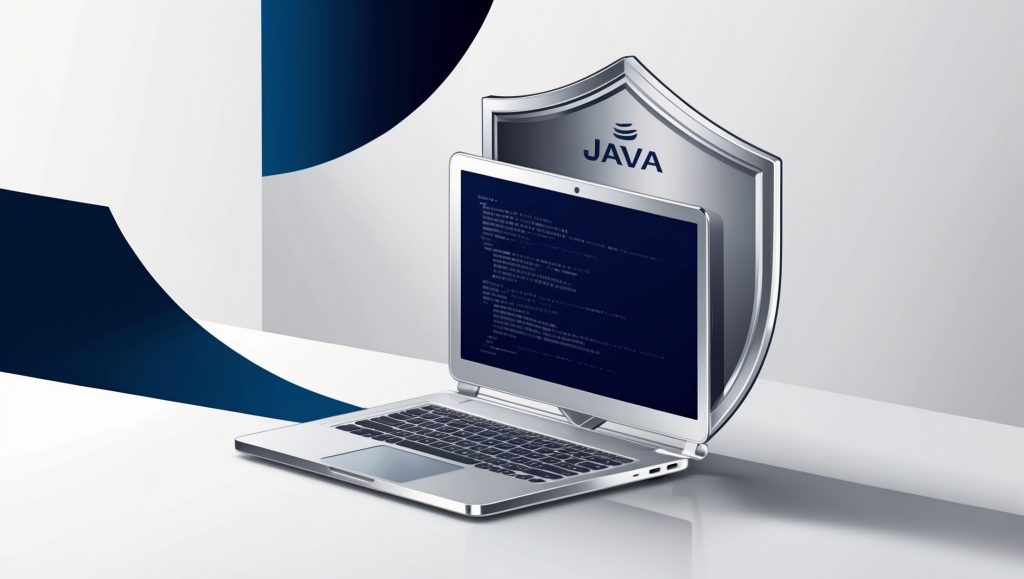
Java Security Best Practices- Protecting applications from vulnerabilities.
In today’s digital landscape, security is not just an option but a fundamental requirement for any Java application. With cyber threats becoming increasingly sophisticated, developers must implement robust security measures to protect their applications from potential vulnerabilities. This comprehensive guide explores essential Java security best practices, providing practical examples and actionable insights to help you build more secure applications.
Understanding the Importance of Java Security
Security breaches can have devastating consequences for organizations, including data theft, financial losses, and damaged reputation. Java, being one of the most widely used programming languages in enterprise applications, is often a target for malicious actors. Understanding and implementing security best practices is crucial for protecting sensitive data and maintaining the integrity of your applications. The Java Security Model provides a robust framework for implementing security measures, but developers must know how to leverage these features effectively.
Input Validation and Sanitization
The First Line of Defense
Input validation serves as the primary defense against many common security vulnerabilities, including SQL injection, cross-site scripting (XSS), and buffer overflow attacks. Every input from external sources should be treated as potentially malicious and properly validated before processing. Java provides several built-in mechanisms and libraries for input validation.
Here’s an example of implementing input validation:
public class InputValidator {
public static boolean validateUserInput(String input) {
// Check if input is null or empty
if (input == null || input.trim().isEmpty()) {
return false;
}
// Define allowed pattern (alphanumeric and some special characters)
String pattern = "^[a-zA-Z0-9@._-]{3,50}$";
// Validate input against pattern
return input.matches(pattern);
}
public static String sanitizeHtmlInput(String input) {
return input.replace("<", "<")
.replace(">", ">")
.replace("\"", """)
.replace("'", "'")
.replace("/", "/");
}
}
Secure Authentication and Authorization
Implementing Strong Authentication
Authentication and authorization form the cornerstone of application security. Implementing robust authentication mechanisms ensures that only legitimate users can access your application. Use industry-standard authentication frameworks and follow these best practices:
- Implement password hashing using strong algorithms like BCrypt
- Enforce multi-factor authentication for sensitive operations
- Implement account lockout policies
- Use secure session management
Here’s an example of password hashing using BCrypt:
public class PasswordService {
private static final int BCRYPT_ROUNDS = 12;
public static String hashPassword(String plainTextPassword) {
return BCrypt.hashpw(plainTextPassword, BCrypt.gensalt(BCRYPT_ROUNDS));
}
public static boolean verifyPassword(String plainTextPassword, String hashedPassword) {
try {
return BCrypt.checkpw(plainTextPassword, hashedPassword);
} catch (IllegalArgumentException e) {
return false;
}
}
}
Role-Based Access Control (RBAC)
Implementing RBAC ensures that users can only access resources appropriate to their role. Here’s an example using Spring Security annotations:
@Configuration
@EnableWebSecurity
public class SecurityConfig extends WebSecurityConfigurerAdapter {
@Override
protected void configure(HttpSecurity http) throws Exception {
http
.authorizeRequests()
.antMatchers("/public/**").permitAll()
.antMatchers("/admin/**").hasRole("ADMIN")
.antMatchers("/user/**").hasRole("USER")
.anyRequest().authenticated()
.and()
.formLogin()
.loginPage("/login")
.permitAll();
}
}
Secure Communication
Implementing SSL/TLS
All sensitive data transmission should be encrypted using SSL/TLS. Configure your application to use HTTPS and implement certificate pinning for additional security. Here’s an example of configuring SSL in a Spring Boot application:
@Configuration
public class ServerConfig {
@Bean
public ServletWebServerFactory servletContainer() {
TomcatServletWebServerFactory tomcat = new TomcatServletWebServerFactory();
tomcat.addAdditionalTomcatConnectors(createSslConnector());
return tomcat;
}
private Connector createSslConnector() {
Connector connector = new Connector("org.apache.coyote.http11.Http11NioProtocol");
connector.setScheme("https");
connector.setSecure(true);
connector.setPort(8443);
connector.setProperty("keystoreFile", "keystore.p12");
connector.setProperty("keystorePass", "your-keystore-password");
connector.setProperty("keystoreType", "PKCS12");
connector.setProperty("keyAlias", "tomcat");
return connector;
}
}
Secure Data Storage
Database Security
Protecting sensitive data at rest is crucial. Implement these database security measures:
- Use prepared statements to prevent SQL injection
- Encrypt sensitive data before storage
- Implement proper access controls at the database level
- Regular security audits and monitoring
Example of using prepared statements:
public class SecureDataAccess {
public User getUserByCredentials(String username, String password) {
String sql = "SELECT * FROM users WHERE username = ? AND password_hash = ?";
try (Connection conn = dataSource.getConnection();
PreparedStatement stmt = conn.prepareStatement(sql)) {
stmt.setString(1, username);
stmt.setString(2, hashPassword(password));
try (ResultSet rs = stmt.executeQuery()) {
if (rs.next()) {
return mapResultSetToUser(rs);
}
}
} catch (SQLException e) {
logger.error("Database error", e);
throw new DatabaseException("Error accessing user data");
}
return null;
}
}
Secure File Operations
File Upload Security
Implement secure file upload handling to prevent various attacks:
- Validate file types and sizes
- Use secure file storage locations
- Implement proper access controls
- Scan uploaded files for malware
public class SecureFileUploadHandler {
private static final Set<String> ALLOWED_EXTENSIONS =
Set.of("jpg", "jpeg", "png", "pdf");
private static final long MAX_FILE_SIZE = 5 * 1024 * 1024; // 5MB
public void handleFileUpload(MultipartFile file) throws SecurityException {
// Validate file size
if (file.getSize() > MAX_FILE_SIZE) {
throw new SecurityException("File size exceeds maximum limit");
}
// Validate file extension
String extension = FilenameUtils.getExtension(file.getOriginalFilename());
if (!ALLOWED_EXTENSIONS.contains(extension.toLowerCase())) {
throw new SecurityException("Invalid file type");
}
// Generate secure filename
String secureFilename = UUID.randomUUID().toString() + "." + extension;
// Save file to secure location
Path destination = Paths.get("/secure/upload/location", secureFilename);
Files.copy(file.getInputStream(), destination, StandardCopyOption.REPLACE_EXISTING);
}
}
Security Headers and Configuration
Implementing Security Headers
Proper security headers help protect against various attacks. Here’s an example of configuring security headers in Spring Security:
@Configuration
public class SecurityHeadersConfig extends WebSecurityConfigurerAdapter {
@Override
protected void configure(HttpSecurity http) throws Exception {
http
.headers()
.frameOptions().deny()
.xssProtection().block(true)
.contentSecurityPolicy("default-src 'self'")
.and()
.referrerPolicy()
.policy(ReferrerPolicy.SAME_ORIGIN)
.and()
.permissionsPolicy().policy("geolocation 'none'");
}
}
Logging and Monitoring
Implementing Secure Logging
Proper logging is essential for security monitoring and incident response. Implement these logging best practices:
- Log security-relevant events
- Protect log files from unauthorized access
- Implement log rotation
- Use structured logging formats
public class SecurityLogger {
private static final Logger logger = LoggerFactory.getLogger(SecurityLogger.class);
public void logSecurityEvent(String eventType, String username, String action) {
SecurityEvent event = new SecurityEvent(
eventType,
username,
action,
LocalDateTime.now(),
getClientIP()
);
logger.info("Security Event: {}", event);
}
private String getClientIP() {
// Implementation to get client IP securely
return ((ServletRequestAttributes) RequestContextHolder.currentRequestAttributes())
.getRequest().getRemoteAddr();
}
}
Regular Security Audits and Updates
Maintaining Security
Regular security audits and updates are crucial for maintaining application security:
- Conduct regular security assessments
- Keep dependencies updated
- Monitor security advisories
- Implement automated security testing
Here’s an example of implementing dependency vulnerability checking using OWASP Dependency-Check:
<plugin>
<groupId>org.owasp</groupId>
<artifactId>dependency-check-maven</artifactId>
<version>6.5.3</version>
<configuration>
<failBuildOnCVSS>8</failBuildOnCVSS>
<suppressionFiles>
<suppressionFile>dependency-check-suppression.xml</suppressionFile>
</suppressionFiles>
</configuration>
<executions>
<execution>
<goals>
<goal>check</goal>
</goals>
</execution>
</executions>
</plugin>
Error Handling and Security
Secure Error Handling
Implement proper error handling to prevent information leakage:
- Use custom error pages
- Avoid exposing stack traces
- Log errors securely
- Implement proper exception handling
@ControllerAdvice
public class GlobalExceptionHandler extends ResponseEntityExceptionHandler {
private static final Logger logger = LoggerFactory.getLogger(GlobalExceptionHandler.class);
@ExceptionHandler(Exception.class)
public ResponseEntity<Object> handleAllExceptions(Exception ex, WebRequest request) {
// Log the full exception for debugging
logger.error("Unhandled exception", ex);
// Return generic error message to client
ErrorResponse errorResponse = new ErrorResponse(
HttpStatus.INTERNAL_SERVER_ERROR.value(),
"An unexpected error occurred. Please try again later."
);
return new ResponseEntity<>(errorResponse, HttpStatus.INTERNAL_SERVER_ERROR);
}
}
Conclusion
Implementing robust security measures in Java applications requires a comprehensive approach that addresses multiple aspects of security. By following these best practices and regularly updating your security measures, you can significantly reduce the risk of security breaches and protect your applications from potential vulnerabilities. Remember that security is an ongoing process that requires constant vigilance and regular updates to stay ahead of emerging threats.
Disclaimer: This guide provides general security best practices for Java applications. While we strive to maintain accuracy and completeness, security requirements may vary based on specific use cases and environments. Always consult with security professionals and stay updated with the latest security guidelines and vulnerabilities. If you notice any inaccuracies in this guide, please report them to our editorial team for prompt correction.