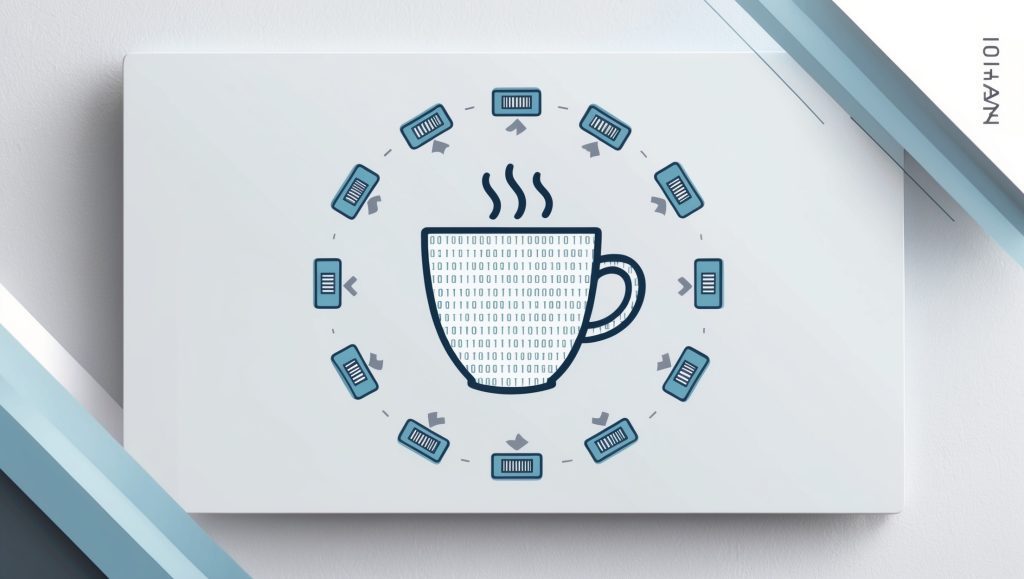
Java Serialization – Object persistence and data transfer
In today’s distributed computing landscape, the ability to convert complex objects into a format suitable for storage or transmission is crucial. Java Serialization provides a robust mechanism for transforming object states into a byte stream and vice versa, making it an essential feature for developers working with Java applications. This comprehensive guide explores the intricacies of Java Serialization, its practical applications, best practices, and potential pitfalls to avoid.
Understanding Java Serialization
Serialization in Java is the process of converting an object’s state into a byte stream, which can then be persisted to disk or transmitted over a network. This mechanism allows you to save the state of your Java objects and recreate them when needed, maintaining the object’s state across different Java Virtual Machines (JVMs) or system restarts. The reverse process, known as deserialization, reconstructs the object from the byte stream, making it a powerful tool for data persistence and inter-system communication.
Key Concepts of Serialization
- ObjectOutputStream and ObjectInputStream are the primary classes used for serialization and deserialization
- The Serializable interface marks classes as serializable
- SerialVersionUID helps maintain version compatibility
- Transient keywords exclude fields from serialization
- Custom serialization methods provide control over the process
Let’s look at a basic example of how serialization works:
import java.io.*;
public class Employee implements Serializable {
private static final long serialVersionUID = 1L;
private String name;
private int id;
private transient String tempData;
public Employee(String name, int id) {
this.name = name;
this.id = id;
this.tempData = "Non-serialized data";
}
// Getters and setters
}
// Serializing an object
public class SerializationDemo {
public static void main(String[] args) {
Employee emp = new Employee("John Doe", 1001);
// Serialization
try (FileOutputStream fileOut = new FileOutputStream("employee.ser");
ObjectOutputStream out = new ObjectOutputStream(fileOut)) {
out.writeObject(emp);
System.out.println("Employee serialized successfully");
} catch (IOException e) {
e.printStackTrace();
}
// Deserialization
try (FileInputStream fileIn = new FileInputStream("employee.ser");
ObjectInputStream in = new ObjectInputStream(fileIn)) {
Employee empDeserialized = (Employee) in.readObject();
System.out.println("Employee deserialized successfully");
} catch (IOException | ClassNotFoundException e) {
e.printStackTrace();
}
}
}
The Serialization Process in Detail
The serialization process involves several steps and considerations that developers must understand to implement it effectively. When an object is serialized, Java creates a precise binary representation of the object’s data and metadata, including information about the object’s class and the values of its non-transient fields. This process recursively handles all referenced objects, creating a complete object graph that can be reconstructed later.
Serialization Steps
- Check if the class implements Serializable
- Create an ObjectOutputStream wrapped around a FileOutputStream
- Write the object using writeObject()
- Handle any potential IOExceptions
- Close the streams properly
The following example demonstrates a more complex serialization scenario with nested objects:
public class Department implements Serializable {
private static final long serialVersionUID = 2L;
private String name;
private List<Employee> employees;
public Department(String name) {
this.name = name;
this.employees = new ArrayList<>();
}
public void addEmployee(Employee emp) {
employees.add(emp);
}
}
// Serializing nested objects
public class ComplexSerializationDemo {
public static void main(String[] args) {
Department dept = new Department("Engineering");
dept.addEmployee(new Employee("John Doe", 1001));
dept.addEmployee(new Employee("Jane Smith", 1002));
try (ObjectOutputStream out = new ObjectOutputStream(
new FileOutputStream("department.ser"))) {
out.writeObject(dept);
} catch (IOException e) {
e.printStackTrace();
}
}
}
Custom Serialization
While Java’s default serialization mechanism is sufficient for many cases, sometimes you need more control over how objects are serialized and deserialized. Custom serialization allows you to define precisely how your objects should be converted to and from byte streams, which can be particularly useful for handling complex data structures or implementing specific security measures.
Implementing Custom Serialization
To implement custom serialization, you can use the following methods:
- writeObject() – For custom serialization logic
- readObject() – For custom deserialization logic
- writeReplace() – For replacing the object before serialization
- readResolve() – For replacing the object after deserialization
Here’s an example implementing custom serialization:
public class CustomEmployee implements Serializable {
private static final long serialVersionUID = 3L;
private String name;
private int id;
private String encryptedPassword;
private void writeObject(ObjectOutputStream out) throws IOException {
out.defaultWriteObject();
// Add custom serialization logic
out.writeObject(encryptPassword(encryptedPassword));
}
private void readObject(ObjectInputStream in)
throws IOException, ClassNotFoundException {
in.defaultReadObject();
// Add custom deserialization logic
this.encryptedPassword = decryptPassword((String)in.readObject());
}
private String encryptPassword(String pwd) {
// Encryption logic here
return pwd;
}
private String decryptPassword(String encryptedPwd) {
// Decryption logic here
return encryptedPwd;
}
}
Best Practices and Performance Considerations
Implementing serialization requires careful consideration of various factors to ensure optimal performance and maintainability. Following best practices can help avoid common pitfalls and ensure your serialization implementation remains robust and efficient over time.
Serialization Best Practices
- Always declare SerialVersionUID
- Use transient for non-serializable fields
- Implement custom serialization for sensitive data
- Consider alternative serialization formats for better performance
- Handle inheritance properly
- Test serialization with different versions of your classes
Here’s a table comparing different serialization approaches:
Approach | Advantages | Disadvantages |
---|---|---|
Default Serialization | Easy to implement, Automatic handling | Less efficient, Limited control |
Custom Serialization | Full control, Better performance | More complex, Requires maintenance |
External Serialization | Maximum flexibility, Better security | Most complex, Higher development effort |
Serialization can introduce security vulnerabilities if not implemented carefully. Deserialization of untrusted data can lead to serious security issues, including remote code execution. Understanding and implementing proper security measures is crucial for any application using serialization.
Security Best Practices
- Validate all serialized data before deserialization
- Implement SecurityManager for controlled deserialization
- Use serialization filters (introduced in Java 9)
- Avoid deserializing data from untrusted sources
- Implement proper exception handling
Example of implementing serialization filters:
public class SerializationFilterDemo {
public static void main(String[] args) {
// Create a filter that only allows specific classes
ObjectInputFilter filter = FilterFactory.createFilter(
"java.base/*;com.example.trusted.*");
try (ObjectInputStream in = new ObjectInputStream(
new FileInputStream("data.ser"))) {
// Set the filter
in.setObjectInputFilter(filter);
// Safe deserialization
Object obj = in.readObject();
} catch (IOException | ClassNotFoundException e) {
e.printStackTrace();
}
}
}
Alternatives to Java Serialization
While Java Serialization is built into the language, there are several alternatives that might better suit specific use cases. These alternatives often provide better performance, cross-platform compatibility, or additional features.
Popular Serialization Alternatives
- JSON (Jackson, Gson)
- Protocol Buffers
- Apache Avro
- MessagePack
- Kryo
Here’s an example using Jackson for JSON serialization:
import com.fasterxml.jackson.databind.ObjectMapper;
public class JsonSerializationDemo {
public static void main(String[] args) {
ObjectMapper mapper = new ObjectMapper();
Employee emp = new Employee("John Doe", 1001);
try {
// Serialize to JSON
String jsonString = mapper.writeValueAsString(emp);
// Deserialize from JSON
Employee empFromJson = mapper.readValue(jsonString, Employee.class);
} catch (Exception e) {
e.printStackTrace();
}
}
}
Troubleshooting Common Serialization Issues
When working with serialization, developers often encounter various issues that can be challenging to debug. Understanding common problems and their solutions can save considerable development time and prevent potential runtime errors.
Common Issues and Solutions
- InvalidClassException – Ensure SerialVersionUID matches
- NotSerializableException – Check if all object references implement Serializable
- StreamCorruptedException – Verify the integrity of serialized data
- OutOfMemoryError – Consider implementing custom serialization for large objects
- Version compatibility issues – Implement proper versioning strategy
Future of Java Serialization
The Java serialization API has evolved since its introduction, and future Java releases continue to bring improvements and security enhancements. Understanding current developments and future directions can help developers make informed decisions about serialization strategies.
Recent Developments
- Enhanced filtering capabilities
- Improved security measures
- Better performance optimizations
- New serialization alternatives
- Integration with modern Java features
Conclusion
Java Serialization remains a fundamental feature for object persistence and data transfer in Java applications. While it has its challenges and limitations, understanding its proper implementation, security considerations, and best practices allows developers to effectively utilize this powerful mechanism. As Java continues to evolve, staying informed about serialization improvements and alternatives ensures you can choose the most appropriate solution for your specific use case.
Disclaimer: This blog post is intended for educational purposes and reflects current best practices as of November 2024. While we strive for accuracy, technology evolves rapidly, and some information may become outdated. Please verify critical information and refer to official Java documentation for the most current guidelines. If you notice any inaccuracies, please report them to our editorial team for prompt correction.