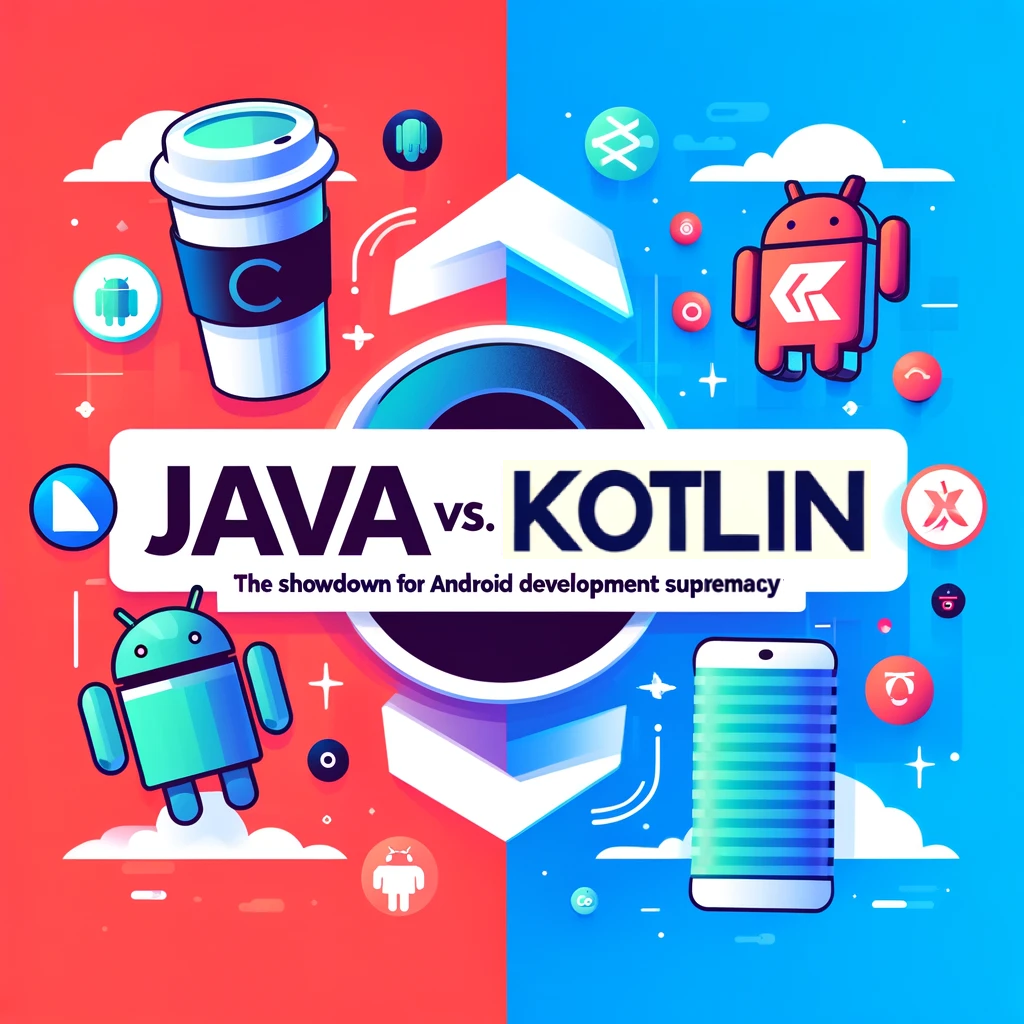
Java vs. Kotlin: The Showdown for Android Development Supremacy
Android Development: Android is the undisputed king of the mobile operating system market, with a staggering global market share of over 70%. As the demand for mobile applications continues to soar, developers are faced with a crucial decision: which programming language to choose for their Android development endeavors? The two leading contenders are Java and Kotlin, each with its own unique strengths and capabilities.
Java’s Legacy: For over a decade, Java has been the de facto standard for Android development. Its popularity stems from its cross-platform compatibility, robust ecosystem, and extensive community support. However, as the mobile landscape evolves, developers are seeking more concise, expressive, and modern languages to streamline their development processes.
Kotlin’s Rise: Introduced by JetBrains in 2011, Kotlin has swiftly gained traction within the Android development community. Designed with a focus on simplicity, safety, and interoperability with Java, Kotlin has garnered official support from Google as a first-class language for Android app development.
In this blog post, we’ll delve into the intricacies of Java and Kotlin, exploring their respective strengths, weaknesses, and suitability for Android development. We’ll examine code samples, performance considerations, and community support to help you make an informed decision about which language to embrace for your next Android project.
Java: The Tried and True
Object-Oriented Principles: Java is a pure object-oriented programming (OOP) language, adhering strictly to the principles of encapsulation, inheritance, and polymorphism. This design philosophy ensures code modularity, reusability, and maintainability, making it easier to manage complex projects.
Example: Here’s a simple Java class that demonstrates OOP principles:
public class Animal {
private String name;
private int age;
public Animal(String name, int age) {
this.name = name;
this.age = age;
}
public void makeSound() {
System.out.println("The animal makes a sound.");
}
}
public class Dog extends Animal {
public Dog(String name, int age) {
super(name, age);
}
@Override
public void makeSound() {
System.out.println("Woof! Woof!");
}
}
Extensive Libraries and Frameworks: Java boasts an extensive ecosystem of libraries and frameworks, enabling developers to leverage pre-built functionality and accelerate development. Popular libraries like Retrofit, OkHttp, and Gson simplify network communication, data parsing, and JSON handling, respectively.
Example: Using Retrofit for network requests:
public interface ApiService {
@GET("users")
Call<List<User>> getUsers();
}
Retrofit retrofit = new Retrofit.Builder()
.baseUrl("https://api.example.com/")
.build();
ApiService apiService = retrofit.create(ApiService.class);
Call<List<User>> call = apiService.getUsers();
call.enqueue(new Callback<List<User>>() {
@Override
public void onResponse(Call<List<User>> call, Response<List<User>> response) {
// Handle successful response
}
@Override
public void onFailure(Call<List<User>> call, Throwable t) {
// Handle failure
}
});
Robust Tooling and IDEs: Java benefits from a wealth of mature and feature-rich Integrated Development Environments (IDEs) like Android Studio, IntelliJ IDEA, and Eclipse. These IDEs offer advanced code editing, debugging, and refactoring capabilities, enhancing developer productivity.
Performance and Optimization: Java’s just-in-time (JIT) compilation and garbage collection mechanisms contribute to its performance and memory management efficiency. Additionally, Java’s low-level control over memory allocation and native code integration via the Native Development Kit (NDK) enable performance optimization when necessary.
Kotlin: The Modern Contender
Concise and Expressive Syntax: Kotlin’s syntax is designed to be more concise and expressive than Java, reducing boilerplate code and increasing developer productivity. Features like extension functions, lambda expressions, and null safety enhance code readability and maintainability.
Example: Kotlin’s concise syntax for defining a data class and using lambda expressions:
data class User(val name: String, val age: Int)
val users = listOf(
User("Alice", 25),
User("Bob", 30)
)
users.forEach { user ->
println("Name: ${user.name}, Age: ${user.age}")
}
Interoperability with Java: One of Kotlin’s major strengths is its seamless interoperability with Java. Kotlin code can call Java libraries and frameworks, and vice versa, allowing developers to leverage existing Java codebases and gradually migrate to Kotlin.
Example: Calling a Java method from Kotlin:
val javaClass = JavaClass()
javaClass.someJavaMethod()
Functional Programming Support: Kotlin embraces functional programming concepts, such as higher-order functions, lambdas, and immutable data structures. This paradigm promotes code reusability, testability, and concurrency, aligning with modern software development practices.
Example: Using higher-order functions and lambdas in Kotlin:
val numbers = listOf(1, 2, 3, 4, 5)
val doubledNumbers = numbers.map { it * 2 }
val evenNumbers = numbers.filter { it % 2 == 0 }
Null Safety: Kotlin’s null safety feature helps prevent null pointer exceptions, a common source of bugs in Java applications. By enforcing nullable and non-nullable types, Kotlin ensures that null values are handled explicitly, improving code robustness and reliability.
Example: Handling nullable values in Kotlin:
fun printLength(str: String?) {
val length = str?.length ?: 0
println("Length: $length")
}
Coroutines for Asynchronous Programming: Kotlin’s coroutines provide a lightweight and efficient way to handle asynchronous operations, such as network requests and background tasks. Coroutines simplify asynchronous code by eliminating the need for complex callback structures, resulting in more readable and maintainable code.
Example: Using coroutines for asynchronous network requests:
suspend fun fetchUsers(): List<User> {
return withContext(Dispatchers.IO) {
val response = apiService.getUsers().await()
response.body() ?: emptyList()
}
}
fun loadUsers() {
lifecycleScope.launch {
val users = fetchUsers()
// Update UI with users
}
}
Performance Considerations
While both Java and Kotlin are capable of delivering high-performance Android applications, there are some performance nuances to consider.
Java’s Optimizations: Java has undergone years of optimization and performance tuning, resulting in highly optimized bytecode and JIT compilation. This makes Java an excellent choice for computationally intensive tasks or applications that require low-level control over memory management.
Kotlin’s Bytecode Efficiency: Kotlin generates bytecode that is often more compact and efficient than its Java counterpart. This can lead to smaller application sizes and potentially faster execution times for certain operations. However, the performance impact varies depending on the specific use case and code complexity.
Memory Management: Both Java and Kotlin rely on automatic memory management through garbage collection. While Java’s garbage collection has been optimized over the years, Kotlin’s approach to memory management is generally considered more efficient, especially when dealing with large amounts of short-lived objects.
Startup Time: Due to its ahead-of-time (AOT) compilation approach, Kotlin applications may exhibit faster startup times compared to Java applications, which rely on just-in-time (JIT) compilation. However, this advantage diminishes as the application runs and the JIT compiler optimizes the Java bytecode.
It’s important to note that performance considerations should be evaluated within the context of your specific application requirements and use cases. Both Java and Kotlin are capable of delivering high-performance Android applications when utilized correctly.
Community and Ecosystem
Java’s Mature Ecosystem: Java has a long-standing and vibrant ecosystem, with a vast array of libraries, frameworks, and tools available. This mature ecosystem provides developers with a wealth of resources, documentation, and community support, making it easier to find solutions to common problems and leverage existing code.
Kotlin’s Growing Popularity: While Kotlin is a relatively newer language, it has gained significant traction within the Android development community. Google’s official endorsement and adoption of Kotlin have accelerated its growth, attracting a rapidly expanding community of developers and contributors. Major companies like Uber, Pinterest, and Trello have embraced Kotlin for their Android applications, further fueling its adoption.
Learning Resources: Both Java and Kotlin offer a wealth of learning resources, ranging from official documentation to online tutorials, books, and video courses. Java’s long history means there is an abundance of learning materials available, while Kotlin’s growing popularity has led to an increasing number of high-quality resources tailored specifically for Android development.
Tooling and IDE Support: As mentioned earlier, Java benefits from mature and feature-rich IDEs like Android Studio, IntelliJ IDEA, and Eclipse. Kotlin, being a first-class language for Android development, enjoys excellent tooling and IDE support within Android Studio and IntelliJ IDEA, with features like code completion, refactoring, and debugging.
The Future of Android Development
As the mobile landscape continues to evolve, the choice between Java and Kotlin for Android development will likely become more nuanced and context-dependent.
Kotlin’s Momentum: Google’s endorsement of Kotlin as a first-class language for Android development has undoubtedly given it a significant boost. Many developers and companies are embracing Kotlin for its concise syntax, null safety, and modern language features, suggesting a trend towards increased Kotlin adoption in the Android ecosystem.
Java’s Staying Power: Despite Kotlin’s rising popularity, Java’s extensive ecosystem, performance optimizations, and compatibility with existing codebases ensure its continued relevance in Android development. Particularly for legacy projects or applications with complex Java codebases, migrating to Kotlin may not always be a feasible or cost-effective option.
Hybrid Approaches: In many cases, a hybrid approach combining Java and Kotlin may be the most practical solution. Developers can leverage Kotlin’s modern features for new code while gradually migrating existing Java codebases. This incremental approach allows teams to reap the benefits of Kotlin while minimizing disruption to existing projects.
Cross-Platform Development: With the increasing demand for cross-platform mobile development, frameworks like Flutter and React Native have gained traction. While these frameworks primarily target cross-platform development, they can also be used for Android development, potentially influencing language choices in the future.
The showdown between Java and Kotlin for Android development supremacy is a compelling one, with each language offering its own unique strengths and capabilities. Java’s long-standing dominance, mature ecosystem, and proven performance make it a reliable choice for Android development. On the other hand, Kotlin’s concise syntax, null safety, and modern language features, combined with its seamless interoperability with Java, position it as a compelling alternative for new projects or developers seeking a more modern and expressive language.
Ultimately, the choice between Java and Kotlin will depend on various factors, such as project requirements, team expertise, existing codebases, and future development goals. For projects with extensive Java codebases or performance-critical requirements, Java may be the more suitable option. Conversely, for new projects or teams seeking a more modern and expressive language, Kotlin presents an attractive choice, especially given Google’s endorsement and the language’s growing popularity.
In many cases, a hybrid approach combining Java and Kotlin may be the most pragmatic solution, allowing teams to leverage the strengths of both languages while gradually migrating to Kotlin as needed. As the mobile landscape continues to evolve, it’s likely that both Java and Kotlin will continue to play important roles in Android development, with the balance shifting over time based on community adoption, language advancements, and emerging cross-platform development trends.