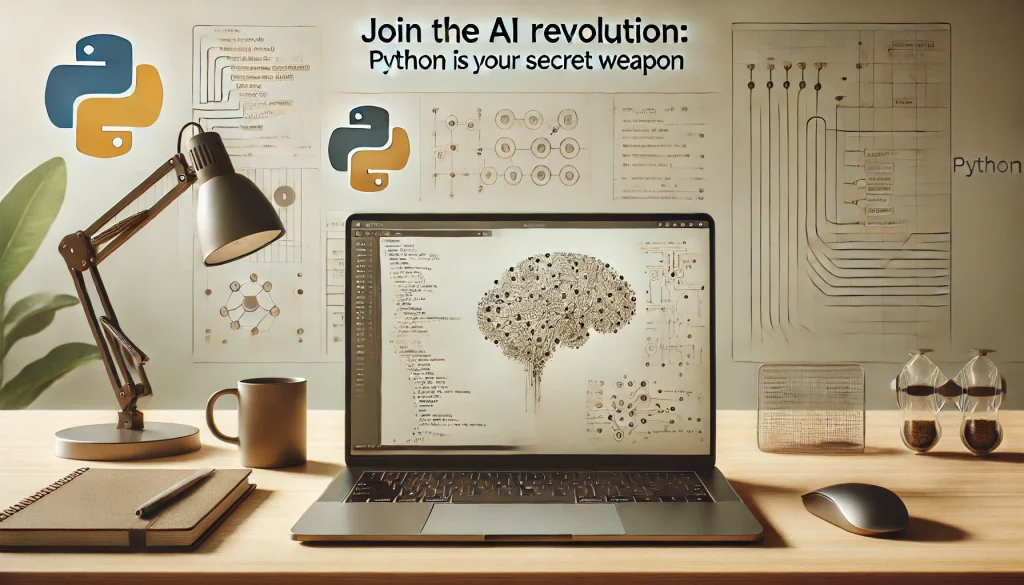
Join the AI Revolution: Python is Your Secret Weapon
Artificial Intelligence (AI) is reshaping the world as we know it. From self-driving cars to personalized recommendations, AI is the driving force behind many of today’s innovations. If you’re a college student or young professional eager to dive into this exciting field, there’s one language you need in your toolkit: Python. Why Python, you ask? Let’s explore how Python is your secret weapon for mastering AI.
Why Python is Perfect for AI
Python has become the go-to language for AI development, and for good reasons:
1. Easy to Learn and Use: Python’s syntax is clear and concise, making it accessible even for beginners.
2. Extensive Libraries and Frameworks: Python boasts a rich ecosystem of libraries and frameworks that simplify AI development.
3. Strong Community Support: With a vast community of developers, finding help and resources is a breeze.
4. Versatility: Python is versatile, allowing you to build a variety of applications, from web development to data analysis.
Getting Started with Python for AI
Setting Up Your Environment
Before diving into AI projects, you need to set up your Python environment. Here’s a step-by-step guide:
1. Install Python: Download the latest version of Python from python.org and follow the installation instructions.
2. Set Up a Virtual Environment: Creating a virtual environment helps manage dependencies. Use the following commands:
pip install virtualenv
virtualenv ai_env
source ai_env/bin/activate
3. Install Essential Libraries: Start by installing popular AI libraries like NumPy, pandas, and scikit-learn:
pip install numpy pandas scikit-learn
Understanding the Basics of AI
Before we jump into coding, let’s understand some fundamental concepts of AI:
1. Machine Learning (ML): A subset of AI, ML involves training algorithms to make predictions or decisions based on data.
2. Deep Learning: A subset of ML, deep learning uses neural networks with multiple layers to analyze complex patterns in data.
3. Natural Language Processing (NLP): NLP focuses on the interaction between computers and human language, enabling tasks like translation and sentiment analysis.
Building Your First AI Model
Let’s start with a simple machine learning model using Python. We’ll build a linear regression model to predict housing prices.
1. Import Libraries:
import numpy as np
import pandas as pd
from sklearn.model_selection import train_test_split
from sklearn.linear_model import LinearRegression
from sklearn.metrics import mean_squared_error
2. Load the Dataset:
For this example, we’ll use a sample dataset from scikit-learn:
from sklearn.datasets import load_boston
boston = load_boston()
data = pd.DataFrame(boston.data, columns=boston.feature_names)
data['PRICE'] = boston.target
3. Prepare the Data:
X = data.drop('PRICE', axis=1)
y = data['PRICE']
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
4. Train the Model:
model = LinearRegression()
model.fit(X_train, y_train)
5. Make Predictions and Evaluate:
y_pred = model.predict(X_test)
mse = mean_squared_error(y_test, y_pred)
print(f"Mean Squared Error: {mse}")
Exploring Deep Learning with Python
Deep learning can be intimidating, but Python’s libraries make it approachable. Let’s create a simple neural network using TensorFlow and Keras.
1. Install TensorFlow and Keras:
pip install tensorflow keras
2. Import Libraries:
import tensorflow as tf
from tensorflow.keras.models import Sequential
from tensorflow.keras.layers import Dense
3. Prepare the Data:
We’ll use the MNIST dataset, a collection of handwritten digits:
mnist = tf.keras.datasets.mnist
(X_train, y_train), (X_test, y_test) = mnist.load_data()
X_train, X_test = X_train / 255.0, X_test / 255.0
4. Build the Model:
model = Sequential([
Dense(128, activation='relu', input_shape=(784,)),
Dense(10, activation='softmax')
])
5. Compile and Train:
model.compile(optimizer='adam', loss='sparse_categorical_crossentropy', metrics=['accuracy'])
model.fit(X_train.reshape(-1, 784), y_train, epochs=5)
6. Evaluate the Model:
loss, accuracy = model.evaluate(X_test.reshape(-1, 784), y_test)
print(f"Accuracy: {accuracy}")
Natural Language Processing with Python
NLP is another fascinating area of AI. Let’s create a simple sentiment analysis model using Python and NLTK.
1. Install NLTK:
pip install nltk
2. Import Libraries:
import nltk
from nltk.sentiment.vader import SentimentIntensityAnalyzer
3. Analyze Sentiments:
nltk.download('vader_lexicon')
sia = SentimentIntensityAnalyzer()
text = "Python is an amazing language for AI!"
score = sia.polarity_scores(text)
print(score)
Advanced AI Projects with Python
Once you’re comfortable with the basics, you can move on to more advanced projects. Here are a few ideas:
1. Image Recognition: Use convolutional neural networks (CNNs) to classify images.
2. Chatbots: Build intelligent chatbots using NLP techniques.
3. Recommendation Systems: Create recommendation engines for personalized content.
Sample Project: Building a Simple Chatbot
Let’s build a basic chatbot using Python and the ChatterBot library.
1. Install ChatterBot:
pip install chatterbot
pip install chatterbot_corpus
2. Create and Train the Chatbot:
from chatterbot import ChatBot
from chatterbot.trainers import ChatterBotCorpusTrainer
bot = ChatBot('AI Bot')
trainer = ChatterBotCorpusTrainer(bot)
trainer.train('chatterbot.corpus.english')
3. Interact with the Chatbot:
while True:
user_input = input("You: ")
if user_input.lower() == 'exit':
break
response = bot.get_response(user_input)
print(f"Bot: {response}")
The Future of AI with Python
The potential of AI is limitless, and Python continues to evolve alongside it. As you advance in your AI journey, you’ll discover more sophisticated techniques and tools. Here are a few trends to watch:
1. Explainable AI (XAI): As AI systems become more complex, understanding their decisions is crucial.
2. Edge AI: Running AI models on devices like smartphones and IoT gadgets.
3. AI Ethics: Ensuring AI is used responsibly and ethically.
Conclusion
Joining the AI revolution is an exciting journey, and Python is your key to unlocking its potential. With its simplicity, powerful libraries, and strong community support, Python makes AI accessible to everyone. Whether you’re building your first machine learning model or diving into deep learning, Python provides the tools you need to succeed.
So, what are you waiting for? Grab your laptop, install Python, and start building the AI solutions of tomorrow. The future is yours to create.
Disclaimer: The information provided in this blog is for educational purposes only. While we strive for accuracy, some details may change over time. Please report any inaccuracies so we can correct them promptly.