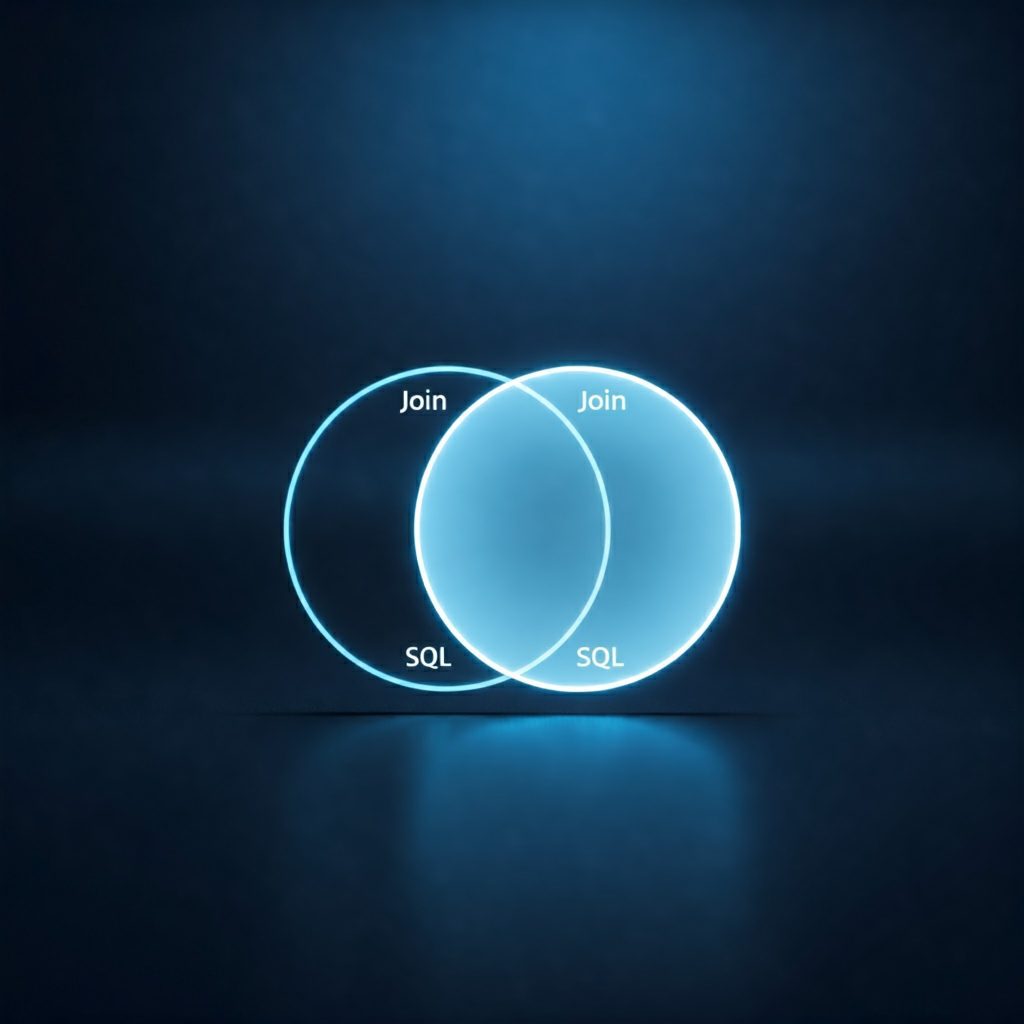
Joining Tables in SQL – A Practical Guide to Combining Data from Multiple Tables
Data is the backbone of modern organizations, and SQL (Structured Query Language) serves as one of the most powerful tools for managing and manipulating this data. In relational databases, data is often spread across multiple tables to reduce redundancy and maintain consistency. To extract meaningful insights, it’s essential to combine data from these tables. This is where SQL joins come into play.
This comprehensive guide will explore the different types of SQL joins, their use cases, and practical examples, helping you understand how to combine data from multiple tables efficiently and accurately.
What are Joins in SQL?
Joins in SQL are operations that allow you to retrieve data from multiple tables based on a related column between them. Most database systems store data in a normalized format, splitting related data into different tables. By joining tables, you can combine rows from these tables based on a condition, such as matching values in a common column.
Types of SQL Joins
SQL offers several types of joins, each serving a different purpose when merging data from multiple tables. Let’s delve into each type to understand how and when to use them.
INNER JOIN
An INNER JOIN returns only the rows that have matching values in both tables. If there is no match between the tables, the row will not be included in the result.
Syntax:
SELECT column1, column2, ...
FROM table1
INNER JOIN table2
ON table1.common_column = table2.common_column;
LEFT JOIN (LEFT OUTER JOIN)
A LEFT JOIN (or LEFT OUTER JOIN) returns all rows from the left table and the matching rows from the right table. If there is no match, NULL values will be returned for the columns from the right table.
Syntax:
SELECT column1, column2, ...
FROM table1
LEFT JOIN table2
ON table1.common_column = table2.common_column;
RIGHT JOIN (RIGHT OUTER JOIN)
A RIGHT JOIN (or RIGHT OUTER JOIN) returns all rows from the right table and the matching rows from the left table. If there is no match, NULL values will be returned for the columns from the left table.
Syntax:
SELECT column1, column2, ...
FROM table1
RIGHT JOIN table2
ON table1.common_column = table2.common_column;
FULL OUTER JOIN
A FULL OUTER JOIN returns all rows when there is a match in either the left or right table. If there is no match, NULL values will be returned for the non-matching side.
Syntax:
SELECT column1, column2, ...
FROM table1
FULL OUTER JOIN table2
ON table1.common_column = table2.common_column;
CROSS JOIN
A CROSS JOIN returns the Cartesian product of the two tables, meaning it combines all rows from the first table with all rows from the second table. This type of join does not require an ON condition.
Syntax:
SELECT column1, column2, ...
FROM table1
CROSS JOIN table2;
SELF JOIN
A SELF JOIN is a join in which a table is joined with itself. It is useful when you need to compare rows within the same table.
Syntax:
SELECT A.column1, B.column2, ...
FROM table_name A, table_name B
WHERE condition;
NATURAL JOIN
A NATURAL JOIN is an automatic join based on columns with the same names in both tables. It simplifies the query but can be risky if column names are not carefully managed.
Syntax:
SELECT column1, column2, ...
FROM table1
NATURAL JOIN table2;
SQL Join Examples with Code
Let’s now look at how to implement these joins with some practical SQL code examples.
INNER JOIN Example
Consider two tables, employees
and departments
:
employees | departments |
---|---|
emp_id | emp_name |
emp_name | department |
dept_id | dept_name |
To retrieve a list of employees along with their respective department names, you would use an INNER JOIN
:
SELECT employees.emp_name, departments.dept_name
FROM employees
INNER JOIN departments
ON employees.dept_id = departments.dept_id;
This query returns only the employees who are assigned to a department.
LEFT JOIN Example
Suppose you want to retrieve a list of all employees, including those who may not be assigned to any department. For this, you use a LEFT JOIN
:
SELECT employees.emp_name, departments.dept_name
FROM employees
LEFT JOIN departments
ON employees.dept_id = departments.dept_id;
This query will return all employees, and for those without a department, the dept_name
will display as NULL
.
RIGHT JOIN Example
If you want to list all departments and the employees assigned to them, including departments that have no employees, a RIGHT JOIN
would be suitable:
SELECT employees.emp_name, departments.dept_name
FROM employees
RIGHT JOIN departments
ON employees.dept_id = departments.dept_id;
Here, all departments will be returned, and any department without employees will show NULL
in the emp_name
column.
FULL OUTER JOIN Example
A FULL OUTER JOIN
can be used to retrieve all employees and all departments, regardless of whether they match in both tables. It combines the results of both the LEFT JOIN
and RIGHT JOIN
:
SELECT employees.emp_name, departments.dept_name
FROM employees
FULL OUTER JOIN departments
ON employees.dept_id = departments.dept_id;
This query returns all employees and departments, even if there is no match between them.
CROSS JOIN Example
A CROSS JOIN
produces a Cartesian product, so if you want to see all possible combinations of employees and departments:
SELECT employees.emp_name, departments.dept_name
FROM employees
CROSS JOIN departments;
If there are 5 employees and 4 departments, the result will have 20 rows (5 * 4).
Practical Use Cases of SQL Joins
Understanding when and why to use each type of join can significantly improve your ability to extract relevant information from your data.
- INNER JOIN Use Case:
When you need to retrieve records that exist in both tables. For example, fetching active orders that have been assigned to a customer in an e-commerce database. - LEFT JOIN Use Case:
ALEFT JOIN
is useful when you want to retrieve all records from one table and the matched records from another. This is common in reporting, where you want to see all customers regardless of whether they have placed an order. - RIGHT JOIN Use Case:
Right joins are less commonly used but are essential when you need to ensure that all records from the right table are returned, such as showing all products even if none have been sold. - FULL OUTER JOIN Use Case:
Full outer joins are helpful when you need a complete view of both datasets, such as comparing two versions of a dataset to see all records from both versions. - CROSS JOIN Use Case:
Cross joins are rare but can be useful in scenarios like generating test data where you need all combinations of multiple attributes.
Best Practices for Using SQL Joins
While SQL joins are incredibly powerful, they can be complex and resource-intensive. Here are some best practices to ensure efficient and accurate query performance:
- Optimize with Indexes:
Ensure that the columns used in theON
condition are indexed. This improves query performance by reducing the time required to match rows across tables. - Use Joins Wisely:
Don’t overuse joins; combining too many tables can lead to slower query execution times, especially in large datasets. - Be Aware of NULLs:
Be mindful ofNULL
values, especially in outer joins. Mismanagement ofNULL
s can lead to unexpected results, particularly in arithmetic operations or filters. - Test for Performance:
Always test the performance of your joins with realistic data sizes. UseEXPLAIN
statements to analyze the query execution plan and identify bottlenecks. - Avoid Cross Joins in Production:
Cross joins should generally be avoided in production environments unless absolutely necessary, as they can generate an overwhelming amount of data. - Join on Primary Keys Whenever Possible:
Joining on primary keys ensures faster lookups and maintains referential integrity.
SQL joins are a fundamental part of working with relational databases, allowing you to combine data across multiple tables based on related columns. Understanding how and when to use different types of joins—such as INNER JOIN
, LEFT JOIN
, RIGHT JOIN
, FULL OUTER JOIN
, and CROSS JOIN is critical for retrieving meaningful insights from your data. Each join type has its specific use cases and performance considerations, making it essential to choose the right join for your query needs.
By adhering to best practices and continuously optimizing your SQL queries, you can ensure accurate results and maintain optimal database performance.
Disclaimer: While we strive to provide accurate and up-to-date information, SQL implementations can vary between systems. Please consult your database documentation for specific details, and report any inaccuracies so we can correct them promptly.