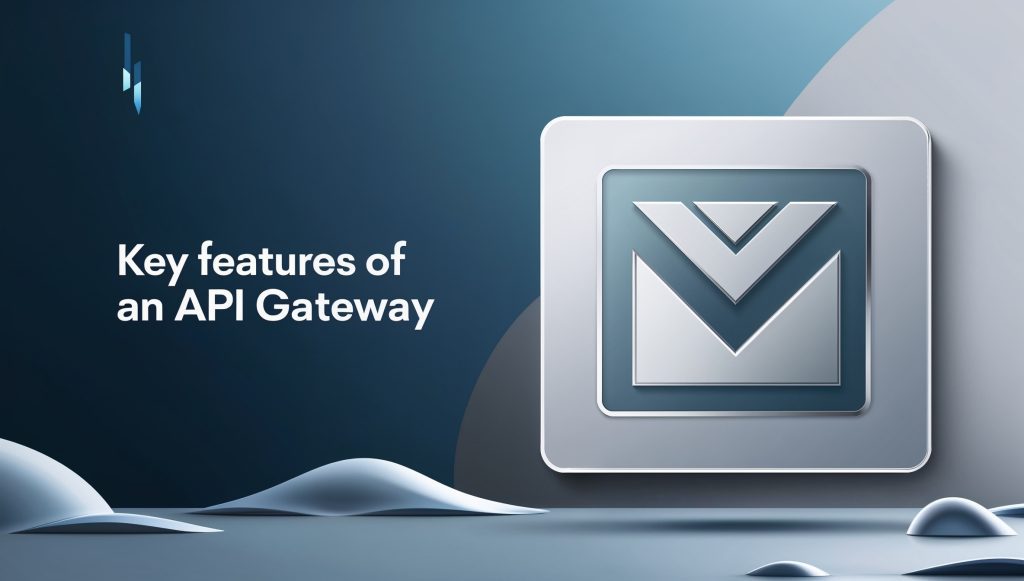
Key Features of API Gateways for Modern Software Architecture
In today’s interconnected digital landscape, APIs (Application Programming Interfaces) have become the lifeblood of modern software systems. They enable seamless communication between different applications, services, and platforms, powering everything from mobile apps to complex enterprise systems. But as the number of APIs grows, so does the need for efficient management and control. Enter the API gateway – a crucial component in any robust API strategy. In this comprehensive guide, we’ll dive deep into the key features of an API gateway, exploring how they can revolutionize your approach to API management and enhance your overall software architecture.
What is an API Gateway?
Before we delve into the nitty-gritty details, let’s start with the basics. An API gateway acts as a central point of entry for all API calls within your system. Think of it as a sophisticated traffic controller for your APIs, managing requests, routing them to the appropriate services, and handling various critical functions along the way. But why is this so important? Well, in a world where microservices architecture and distributed systems are becoming the norm, having a reliable and feature-rich API gateway can make the difference between a smooth, scalable operation and a tangled mess of API chaos.
Now, you might be wondering, “Isn’t an API gateway just another layer of complexity?” That’s a fair question, and the answer is both yes and no. While it does add another component to your architecture, the benefits it brings in terms of security, performance, and manageability far outweigh any potential drawbacks. In fact, as we explore the key features of API gateways, you’ll see how they can actually simplify your overall system design and operations.
Key Features of an API Gateway
Let’s roll up our sleeves and dive into the essential features that make API gateways such powerful tools in modern software architecture. Each of these features addresses specific challenges in API management and contributes to creating a more robust, secure, and efficient API ecosystem.
1. Request Routing and Load Balancing
The traffic director of your API ecosystem
One of the primary functions of an API gateway is to act as a sophisticated router for incoming API requests. Imagine you’re running a bustling restaurant with multiple specialized kitchens. The API gateway is like the head waiter, expertly directing each order to the right kitchen based on the type of dish requested. This routing capability is crucial in microservices architectures, where different services handle different functionalities.
But it doesn’t stop at simple routing. API gateways often come equipped with load balancing capabilities, ensuring that requests are distributed evenly across multiple instances of a service. This is like having a team of efficient waiters who know exactly how busy each kitchen is, making sure no single chef gets overwhelmed while others stand idle. Load balancing is essential for maintaining high availability and preventing individual services from becoming bottlenecks.
Let’s look at a simple Java example of how routing might be implemented in an API gateway:
public class APIGateway {
private Map<String, String> routingTable = new HashMap<>();
public APIGateway() {
// Initialize routing table
routingTable.put("/users", "http://user-service:8080");
routingTable.put("/orders", "http://order-service:8080");
routingTable.put("/products", "http://product-service:8080");
}
public String routeRequest(String path) {
for (Map.Entry<String, String> entry : routingTable.entrySet()) {
if (path.startsWith(entry.getKey())) {
return entry.getValue();
}
}
throw new IllegalArgumentException("No route found for path: " + path);
}
}
In this example, the APIGateway
class maintains a simple routing table and provides a method to determine the appropriate backend service for a given request path. Of course, real-world implementations would be much more sophisticated, incorporating load balancing algorithms and dynamic service discovery.
The beauty of centralized routing is that it abstracts the complexity of your backend services from the client. Clients don’t need to know about the intricacies of your microservices architecture; they just need to know how to talk to the API gateway. This simplification can lead to more maintainable and flexible systems, allowing you to evolve your backend architecture without impacting client applications.
2. Authentication and Authorization
The bouncer and VIP list manager of your API club
Security is paramount in any API ecosystem, and API gateways play a crucial role in ensuring that only authorized users and applications can access your services. Think of your API gateway as the discerning bouncer at an exclusive club. It’s not just about keeping the bad actors out; it’s about ensuring that each guest (or in this case, each API request) has the right level of access.
Authentication is the process of verifying the identity of a user or application making an API request. This could involve checking API keys, validating JWT tokens, or integrating with more complex identity providers. An API gateway centralizes this process, relieving individual services from having to implement their own authentication mechanisms.
Authorization, on the other hand, is about determining what an authenticated user is allowed to do. This might involve checking roles, permissions, or other attributes associated with the user’s identity. API gateways can implement fine-grained access control policies, ensuring that users only have access to the resources and operations they’re entitled to.
Here’s a simplified Java example of how an API gateway might handle authentication and authorization:
public class SecurityManager {
private Map<String, User> users = new HashMap<>();
public SecurityManager() {
// Initialize user database
users.put("api_key_1", new User("Alice", new String[]{"read", "write"}));
users.put("api_key_2", new User("Bob", new String[]{"read"}));
}
public boolean authenticate(String apiKey) {
return users.containsKey(apiKey);
}
public boolean authorize(String apiKey, String requiredPermission) {
User user = users.get(apiKey);
if (user == null) {
return false;
}
return Arrays.asList(user.permissions).contains(requiredPermission);
}
private static class User {
String name;
String[] permissions;
User(String name, String[] permissions) {
this.name = name;
this.permissions = permissions;
}
}
}
This example demonstrates a basic security manager that could be part of an API gateway. It handles both authentication (checking if an API key is valid) and authorization (checking if a user has the required permission for an operation).
By centralizing authentication and authorization at the API gateway level, you can enforce consistent security policies across all your services. This not only enhances security but also simplifies the development of individual services, as they can focus on their core functionality rather than reimplementing security measures.
3. Rate Limiting and Throttling
The traffic cop of your API highway
Imagine your API as a bustling highway. Without proper traffic control, it could quickly become congested, leading to slowdowns or even complete gridlock. This is where rate limiting and throttling come into play, acting as the vigilant traffic cops of your API ecosystem.
Rate limiting is the practice of controlling the number of requests a client can make to your API within a specified time frame. For example, you might allow a client to make 100 requests per minute. Once that limit is reached, subsequent requests are either delayed or rejected until the next time window begins. This helps prevent any single client from overwhelming your system, either intentionally (in the case of a malicious attack) or unintentionally (due to a bug in the client application).
Throttling, while similar, often refers to the practice of slowing down the rate of requests rather than outright rejecting them. It’s like a traffic cop directing cars to a slower lane during rush hour, ensuring that traffic keeps moving, albeit at a reduced pace.
Let’s look at a simple Java implementation of a rate limiter:
public class RateLimiter {
private final int maxRequests;
private final long timeWindow;
private final Map<String, Queue<Long>> clientRequests = new HashMap<>();
public RateLimiter(int maxRequests, long timeWindow) {
this.maxRequests = maxRequests;
this.timeWindow = timeWindow;
}
public synchronized boolean allowRequest(String clientId) {
long now = System.currentTimeMillis();
Queue<Long> requests = clientRequests.computeIfAbsent(clientId, k -> new LinkedList<>());
// Remove old requests
while (!requests.isEmpty() && now - requests.peek() > timeWindow) {
requests.poll();
}
if (requests.size() < maxRequests) {
requests.offer(now);
return true;
} else {
return false;
}
}
}
This rate limiter allows a specified number of requests within a given time window for each client. If a client exceeds the limit, their requests are rejected until the time window resets.
Implementing rate limiting and throttling at the API gateway level offers several advantages:
- Protection against abuse: It helps prevent intentional or accidental DoS (Denial of Service) attacks by limiting the rate at which any single client can access your API.
- Fair resource allocation: By enforcing limits, you ensure that resources are fairly distributed among all clients, preventing any one client from monopolizing your system.
- Improved overall performance: By controlling the inflow of requests, you can maintain consistent performance even during peak usage times.
- Flexible policies: API gateways often allow for sophisticated rate limiting policies, such as different limits for different endpoints, user tiers, or time of day.
Remember, the goal of rate limiting and throttling isn’t to restrict your API’s usage unnecessarily, but to ensure its stability and availability for all users. It’s about creating a smooth, predictable experience for everyone on your API highway.
4. Request and Response Transformation
The universal translator of your API universe
In an ideal world, all your services would speak the same language, use the same data formats, and adhere to the same conventions. But in reality, especially in large or evolving systems, you often have to deal with a variety of formats, protocols, and standards. This is where the request and response transformation capabilities of an API gateway come into play.
Think of your API gateway as a universal translator, capable of understanding and converting between different dialects of API communication. This feature allows you to present a consistent, clean API to your clients, even if your backend services are a motley crew of different technologies and standards.
Here are some common transformations an API gateway might perform:
- Protocol transformation: Converting between different protocols, such as translating a REST request to a SOAP request for a legacy backend service.
- Data format conversion: Transforming data between formats like JSON, XML, or even proprietary formats.
- Field renaming or restructuring: Modifying the structure of requests or responses to match the expected format of clients or backend services.
- Enrichment: Adding additional information to requests or responses, such as appending user context or metadata.
Let’s look at a simple Java example of how an API gateway might transform a request:
public class RequestTransformer {
public String transformRequest(String originalRequest) {
// Parse the original JSON request
JSONObject request = new JSONObject(originalRequest);
// Create a new transformed request
JSONObject transformedRequest = new JSONObject();
// Rename fields
transformedRequest.put("user_id", request.getString("id"));
transformedRequest.put("user_name", request.getString("name"));
// Add a new field
transformedRequest.put("request_timestamp", System.currentTimeMillis());
// Convert back to string
return transformedRequest.toString();
}
}
In this example, the RequestTransformer
takes an original request, renames some fields, and adds a new field before passing it on to the backend service.
The ability to transform requests and responses offers several benefits:
- Backward compatibility: You can evolve your API without breaking existing clients by transforming requests and responses to match the expected format.
- Frontend-backend decoupling: Your client applications can work with a consistent API format, even if backend services use different formats or protocols.
- Legacy system integration: You can expose modern, RESTful APIs even if some of your backend services use older protocols or formats.
- Simplification: Complex operations that might require multiple API calls can be combined into a single call from the client’s perspective, with the API gateway handling the complexity of multiple backend requests.
By centralizing these transformations at the API gateway level, you maintain a clean separation of concerns. Your backend services can focus on their core functionality without worrying about the specifics of how they’re exposed to the outside world. Meanwhile, your client applications get to work with a consistent, well-designed API regardless of the underlying complexities.
5. Caching
The memory wizard of your API realm
In the quest for API performance, caching is your secret weapon. It’s like having a brilliant assistant with a photographic memory, capable of instantly recalling information that would otherwise require time-consuming computations or database lookups. An API gateway with robust caching capabilities can significantly reduce the load on your backend services and dramatically improve response times for your clients.
The basic principle of caching is simple: store the results of expensive operations so that future requests for the same information can be served faster. In the context of an API gateway, this often means caching the responses from backend services. When a request comes in, the gateway first checks if it has a cached response. If it does, it can return that response immediately without needing to forward the request to the backend service.
Here’s a simple Java implementation of a cache that could be used in an API gateway:
public class APICache {
private Map<String, CacheEntry> cache = new ConcurrentHashMap<>();
public void put(String key, String value, long ttlMillis) {
cache.put(key, new CacheEntry(value, System.currentTimeMillis() + ttlMillis));
}
public String get(String key) {
CacheEntry entry = cache.get(key);
if (entry != null && System.currentTimeMillis() < entry.expirationTime) {
return entry.value;
}
cache.remove(key);
return null;
}
private static class CacheEntry {
String value;
long expirationTime;
CacheEntry(String value, long expirationTime) {
this.value = value;
this.expirationTime = expirationTime;
}
}
}
This cache implementation allows storing values with a Time-To-Live (TTL), automatically expiring entries after a specified duration.
Effective caching in an API gateway can provide several benefits:
- Improved performance: Cached responses can be returned much faster than making a round trip to the backend service.
- Reduced backend load: By serving cached responses, the API gateway reduces the number of requests that need to be processed by your backend services.
- Increased reliability: Even if a backend service is temporarily unavailable, the API gateway can continue to serve cached responses for some requests.
- Cost savings: By reducing the load on backend services, caching can lead to lower infrastructure costs.
However, caching also comes with its challenges. The main one is cache invalidation – ensuring that the cached data doesn’t become stale. API gateways often provide mechanisms for setting cache expiration times, as well as ways to manually invalidate cache entries when the underlying data changes.
It’s also worth noting that not all data is suitable for caching. Frequently changing data or data that must always be up-to-the-second accurate may not be good candidates for caching. The key is to carefully consider your caching strategy, balancing the benefits of improved performance against the need for data freshness.
6. Monitoring and Analytics
The all-seeing eye of your API kingdom
In the complex world of API management, knowledge truly is power. And that’s where the monitoring and analytics capabilities of an API gateway come into play. Think of it as having an all-seeing eye watching over your API kingdom, providing you with valuable insights into how your APIs are being used, performing, and potentially being misused.
A good API gateway should offer comprehensive monitoring and analytics features that allow you to:
- Track usage: Understand which APIs are being called, how often, and by whom. This information is crucial for capacity planning and identifying your most valuable APIs.
- Monitor performance: Keep an eye on response times, error rates, and other key performance indicators. This helps you identify and address issues before they impact your users.
- Detect anomalies: Spot unusual patterns of activity that could indicate problems or potential security threats.
- Generate reports: Create detailed reports on API usage, performance, and trends over time.
Here’s a simple Java class that could be used to log API calls for later analysis:
Certainly. I’ll continue the blog post from where we left off:
public class APILogger {
private static final Logger logger = Logger.getLogger(APILogger.class.getName());
public void logAPICall(String endpoint, String method, int statusCode, long responseTime) {
String logMessage = String.format("API Call: %s %s, Status: %d, Response Time: %d ms",
method, endpoint, statusCode, responseTime);
logger.info(logMessage);
}
public void logError(String endpoint, String method, String errorMessage) {
String logMessage = String.format("API Error: %s %s, Error: %s",
method, endpoint, errorMessage);
logger.severe(logMessage);
}
}
This simple logger class could be used within an API gateway to record information about each API call, including successful calls and errors. In a real-world scenario, this data would likely be sent to a more sophisticated analytics system for processing and visualization.
The benefits of robust monitoring and analytics in an API gateway include:
- Proactive problem solving: By monitoring key metrics in real-time, you can identify and address issues before they escalate into major problems.
- Informed decision making: Analytics provide valuable insights that can guide decisions about API design, infrastructure scaling, and feature prioritization.
- Security enhancement: Monitoring can help detect unusual patterns that might indicate security threats, allowing you to respond quickly to potential attacks.
- SLA compliance: Detailed performance data makes it easier to ensure you’re meeting your Service Level Agreements (SLAs) and to provide evidence of compliance.
- User behavior insights: Understanding how your APIs are being used can inform product development decisions and help you better serve your users’ needs.
Remember, the goal of monitoring and analytics isn’t just to collect data – it’s to turn that data into actionable insights. Many API gateways offer customizable dashboards and alerting systems that can help you quickly make sense of the wealth of data being collected.
7. Version Management
The time-traveler of your API world
In the ever-evolving landscape of software development, change is the only constant. But when it comes to APIs, changes can be disruptive, potentially breaking client applications that depend on specific API behaviors. This is where version management in API gateways becomes crucial, acting as a time machine that allows different versions of your API to coexist peacefully.
Effective version management in an API gateway allows you to:
- Introduce new API versions: Roll out new features or make significant changes without disrupting existing clients.
- Support multiple versions simultaneously: Allow clients to continue using older versions while encouraging migration to newer ones.
- Deprecate old versions gracefully: Phase out outdated versions over time, giving clients ample opportunity to update their integrations.
Here’s a simple Java example of how version routing might be implemented in an API gateway:
public class VersionRouter {
private Map<String, Map<String, String>> versionRoutes = new HashMap<>();
public VersionRouter() {
// Initialize version routes
Map<String, String> v1Routes = new HashMap<>();
v1Routes.put("/users", "http://user-service-v1:8080");
v1Routes.put("/orders", "http://order-service-v1:8080");
versionRoutes.put("v1", v1Routes);
Map<String, String> v2Routes = new HashMap<>();
v2Routes.put("/users", "http://user-service-v2:8080");
v2Routes.put("/orders", "http://order-service-v2:8080");
versionRoutes.put("v2", v2Routes);
}
public String routeRequest(String version, String path) {
Map<String, String> routes = versionRoutes.get(version);
if (routes == null) {
throw new IllegalArgumentException("Unknown API version: " + version);
}
String route = routes.get(path);
if (route == null) {
throw new IllegalArgumentException("No route found for path: " + path);
}
return route;
}
}
This VersionRouter
class demonstrates a simple way to route requests to different backend services based on the API version specified in the request.
The benefits of robust version management in an API gateway include:
- Backward compatibility: You can maintain support for older clients while still evolving your API.
- Smoother transitions: Clients can migrate to new versions at their own pace, reducing the risk of disruptions.
- Innovation enablement: You can experiment with new API designs and features without fear of breaking existing integrations.
- Better developer experience: Clear versioning makes it easier for developers to understand which features are available in each API version.
When implementing version management, consider the following best practices:
- Use semantic versioning (e.g., v1, v2) to clearly communicate the scale of changes between versions.
- Provide clear documentation for each API version, including any differences or deprecations.
- Use HTTP headers or URL paths to specify API versions, making it easy for clients to request specific versions.
- Implement a deprecation policy that gives clients ample time to migrate to newer versions before old ones are retired.
8. Service Discovery
The GPS of your microservices universe
In the world of microservices, where services can be dynamically created, destroyed, or scaled, keeping track of where everything is located can be a challenge. This is where service discovery comes into play, acting as a GPS for your API gateway to locate and route requests to the appropriate services.
Service discovery allows your API gateway to:
- Dynamically locate services: Find the current network location (IP address and port) of a service at runtime.
- Handle service changes: Adapt to services being added, removed, or scaled without manual reconfiguration.
- Load balance effectively: Distribute requests across multiple instances of a service.
Here’s a simplified Java example of how service discovery might be implemented:
public class ServiceDiscovery {
private Map<String, List<String>> serviceRegistry = new HashMap<>();
public void registerService(String serviceName, String serviceUrl) {
serviceRegistry.computeIfAbsent(serviceName, k -> new ArrayList<>()).add(serviceUrl);
}
public void deregisterService(String serviceName, String serviceUrl) {
List<String> urls = serviceRegistry.get(serviceName);
if (urls != null) {
urls.remove(serviceUrl);
}
}
public String discoverService(String serviceName) {
List<String> urls = serviceRegistry.get(serviceName);
if (urls == null || urls.isEmpty()) {
throw new IllegalArgumentException("No instances found for service: " + serviceName);
}
// Simple round-robin load balancing
return urls.get(ThreadLocalRandom.current().nextInt(urls.size()));
}
}
This ServiceDiscovery
class provides basic functionality for registering and discovering services, including simple load balancing across multiple instances of a service.
The benefits of incorporating service discovery in your API gateway include:
- Increased flexibility: Services can be added, removed, or scaled dynamically without reconfiguring the API gateway.
- Improved reliability: The API gateway can route around failed service instances, improving overall system reliability.
- Simplified deployment: New service instances can automatically register themselves, simplifying the deployment process.
- Enhanced scalability: The system can more easily adapt to changes in load by adding or removing service instances.
Many API gateways integrate with popular service discovery tools like Consul, Etcd, or Kubernetes’ built-in service discovery, providing robust, production-ready solutions for managing service locations in complex, dynamic environments.
Empowering Your API Strategy with a Feature-Rich Gateway
As we’ve explored in this comprehensive guide, API gateways are far more than simple proxies or routers. They are powerful tools that can significantly enhance your API strategy, providing a wealth of features that improve security, performance, manageability, and developer experience.
From request routing and authentication to caching and service discovery, each feature of an API gateway addresses specific challenges in modern software architecture. By centralizing these functions, API gateways simplify the development and management of individual services, allowing teams to focus on core business logic rather than reimplementing common API management features.
However, it’s important to remember that implementing an API gateway is not a one-size-fits-all solution. The specific features you need and how you implement them will depend on your particular use case, architecture, and requirements. Some organizations might prioritize security features like robust authentication and rate limiting, while others might focus on performance optimizations through caching and efficient routing.
As you consider implementing or upgrading your API gateway, take the time to evaluate your needs carefully. Think about your current pain points in API management, your future scalability requirements, and the developer experience you want to provide to both internal teams and external partners.
Remember, an API gateway is a powerful tool, but it’s not a magic solution to all API-related challenges. It requires thoughtful implementation, ongoing management, and a clear strategy aligned with your overall architectural goals. When done right, however, an API gateway can be a game-changer, providing a solid foundation for building scalable, secure, and efficient API-driven systems.
So, whether you’re just starting your API journey or looking to take your existing API management to the next level, consider how a feature-rich API gateway could empower your strategy. In the fast-paced world of modern software development, having a robust, flexible API infrastructure isn’t just nice to have – it’s a necessity for staying competitive and delivering the best possible experience to your users and partners.
Disclaimer: This blog post is intended for informational purposes only. While we strive to provide accurate and up-to-date information, the field of API management is rapidly evolving. Always consult official documentation and expert advice when implementing API gateways in production environments. If you notice any inaccuracies in this post, please report them so we can correct them promptly.