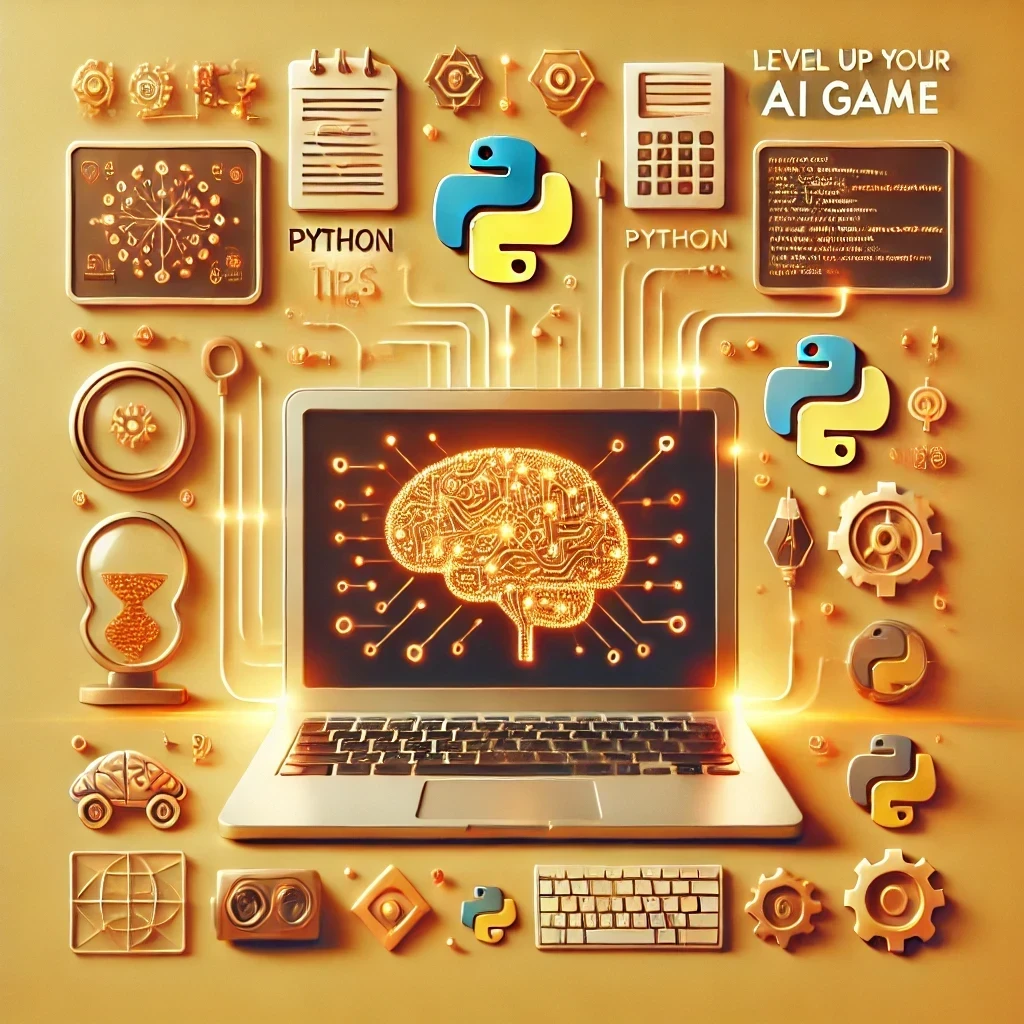
Level Up Your AI Game: Python Tips & Tricks
Python has become the go-to language for artificial intelligence (AI) and machine learning (ML) development. Whether you’re a college student or a young professional looking to dive into the world of AI, Python offers an extensive library ecosystem, a vibrant community, and a straightforward syntax. This blog will provide tips and tricks to help you level up your AI game with Python. We’ll cover everything from optimizing your code to utilizing powerful libraries. Let’s get started!
Why Python for AI?
Python is popular for AI for several reasons:
- Ease of Learning: Python’s simple and readable syntax makes it accessible to beginners.
- Extensive Libraries: Libraries like TensorFlow, Keras, and PyTorch make developing AI models easier.
- Community Support: A large, active community means plenty of resources and support.
- Flexibility: Python is versatile and can be used across different stages of AI development.
Getting Started with Python
Before diving into advanced tips and tricks, ensure you have Python installed on your machine. You can download it from Python’s official website. Additionally, you might want to install an Integrated Development Environment (IDE) like PyCharm or use Jupyter Notebooks for interactive coding.
Tip 1: Mastering Data Preprocessing
Data preprocessing is crucial in AI as it ensures your data is clean and ready for modeling.
Example: Handling Missing Data
import pandas as pd
import numpy as np
# Load dataset
df = pd.read_csv('data.csv')
# Fill missing values with the mean
df.fillna(df.mean(), inplace=True)
# Alternatively, drop rows with missing values
df.dropna(inplace=True)
# Check the changes
print(df.head())
Tip: Use libraries like pandas for data manipulation and numpy for numerical operations to streamline your preprocessing tasks.
Tip 2: Leveraging Built-in Python Libraries
Python’s standard library is powerful and often overlooked. Functions in libraries like itertools
, collections
, and functools
can significantly enhance your code efficiency.
Example: Using itertools
for Efficient Iteration
import itertools
# Create an iterator that returns accumulated sums
accumulated_sums = itertools.accumulate([1, 2, 3, 4, 5])
print(list(accumulated_sums))
# Create an iterator that returns the Cartesian product of input iterables
cartesian_product = itertools.product('AB', '12')
print(list(cartesian_product))
Tip: Familiarize yourself with the Python standard library documentation to discover more such useful functions.
Tip 3: Utilizing Advanced Libraries for AI
To build sophisticated AI models, leverage advanced libraries like TensorFlow, Keras, and PyTorch.
Example: Building a Simple Neural Network with Keras
import keras
from keras.models import Sequential
from keras.layers import Dense
# Create a simple neural network model
model = Sequential()
model.add(Dense(12, input_dim=8, activation='relu'))
model.add(Dense(8, activation='relu'))
model.add(Dense(1, activation='sigmoid'))
# Compile the model
model.compile(loss='binary_crossentropy', optimizer='adam', metrics=['accuracy'])
# Fit the model
model.fit(X, y, epochs=150, batch_size=10)
# Evaluate the model
scores = model.evaluate(X, y)
print(f"\nAccuracy: {scores[1]*100}")
Tip: When working with neural networks, always experiment with different architectures and hyperparameters to find the best model for your data.
Tip 4: Effective Use of Jupyter Notebooks
Jupyter Notebooks are a fantastic tool for interactive coding, especially for data science and AI.
Example: Magic Commands in Jupyter
# Time the execution of a single statement
%timeit sum(range(100))
# Time the execution of a cell
%%timeit
total = 0
for i in range(100):
total += i
Tip: Use markdown cells to document your code and results effectively, making your notebooks more readable and shareable.
Tip 5: Version Control with Git
Version control is essential for managing code changes, especially in collaborative projects.
Example: Basic Git Commands
# Initialize a new Git repository
git init
# Add files to the staging area
git add .
# Commit changes
git commit -m "Initial commit"
# Push changes to a remote repository
git remote add origin <remote_repository_URL>
git push -u origin master
Tip: Regularly commit your changes and write meaningful commit messages to keep track of your project’s progress.
Tip 6: Optimize Your Code with Numba
Numba is a Just-In-Time (JIT) compiler that translates a subset of Python and NumPy code into fast machine code.
Example: Using Numba to Speed Up a Function
from numba import jit
import numpy as np
# Define a function to compute the sum of an array
@jit(nopython=True)
def sum_array(arr):
total = 0
for i in arr:
total += i
return total
# Create a large array
arr = np.random.rand(1000000)
# Use the optimized function
print(sum_array(arr))
Tip: Use Numba for numerical functions to achieve significant speedups with minimal code changes.
Tip 7: Visualizing Data with Matplotlib and Seaborn
Visualization is key to understanding your data and model performance.
Example: Creating a Line Plot with Matplotlib
import matplotlib.pyplot as plt
# Sample data
x = [1, 2, 3, 4, 5]
y = [2, 3, 5, 7, 11]
# Create a line plot
plt.plot(x, y, label='Prime Numbers')
plt.xlabel('X-axis')
plt.ylabel('Y-axis')
plt.title('Line Plot Example')
plt.legend()
plt.show()
Example: Creating a Heatmap with Seaborn
import seaborn as sns
import numpy as np
# Create a sample matrix
data = np.random.rand(10, 12)
# Create a heatmap
sns.heatmap(data, annot=True, cmap='coolwarm')
plt.title('Heatmap Example')
plt.show()
Tip: Combine different types of plots to gain deeper insights into your data.
Tip 8: Enhancing Your Workflow with Virtual Environments
Using virtual environments helps you manage dependencies and avoid conflicts between projects.
Example: Creating and Activating a Virtual Environment
# Create a virtual environment
python -m venv myenv
# Activate the virtual environment (Windows)
myenv\Scripts\activate
# Activate the virtual environment (macOS/Linux)
source myenv/bin/activate
Tip: Always use a virtual environment for your projects to ensure reproducibility and dependency management.
Tip 9: Debugging and Profiling Your Code
Debugging and profiling help you identify and fix issues and optimize performance.
Example: Using PDB for Debugging
import pdb
def faulty_function(x, y):
result = x / y
return result
# Set a breakpoint
pdb.set_trace()
faulty_function(10, 0)
Example: Using cProfile for Profiling
import cProfile
def some_function():
# Some time-consuming operations
pass
# Profile the function
cProfile.run('some_function()')
Tip: Regularly debug and profile your code to catch errors early and improve efficiency.
Tip 10: Automating Tasks with Python Scripts
Automation can save you a lot of time and effort. Use Python scripts to automate repetitive tasks.
Example: Automating File Renaming
import os
def rename_files(directory, prefix):
for count, filename in enumerate(os.listdir(directory)):
dst = f"{prefix}_{str(count)}.txt"
src = f"{directory}/{filename}"
dst = f"{directory}/{dst}"
os.rename(src, dst)
# Call the function
rename_files('path/to/your/directory', 'new_name')
Tip: Write reusable scripts for common tasks to enhance your productivity.
Conclusion
Mastering Python for AI involves understanding its powerful libraries, optimizing your code, and adopting best practices. By following these tips and tricks, you’ll be well on your way to becoming proficient in Python for AI. Remember, practice is key, and don’t hesitate to explore and experiment with different techniques.
Disclaimer: The content provided in this blog is for informational purposes only. While we strive for accuracy, there may be errors or outdated information. Please report any inaccuracies so we can correct them promptly.