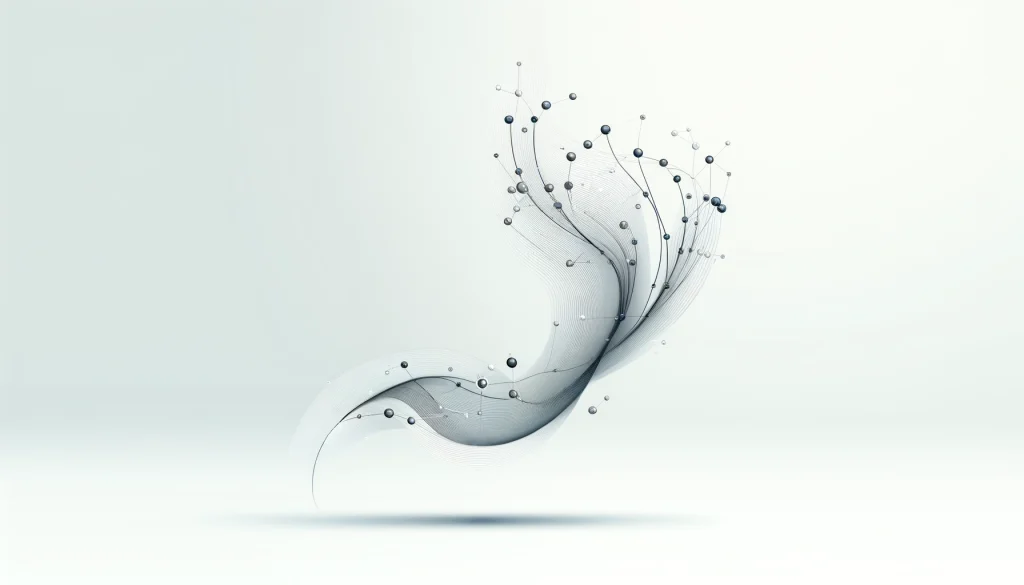
Linked Lists: Connecting the Dots of Your Data
Welcome, fellow coders and data structure enthusiasts! Today, we’re diving deep into the fascinating world of linked lists. If you’ve ever wondered how to efficiently organize and manipulate data in your programs, you’re in for a treat. Linked lists are like the unsung heroes of the programming world – they may not always grab the spotlight, but they’re incredibly versatile and powerful when used correctly. In this comprehensive guide, we’ll explore what linked lists are, why they matter, and how to implement them in Java. So, grab your favorite beverage, settle in, and let’s embark on this exciting journey through the realm of linked data structures!
What Are Linked Lists, Anyway?
Let’s start with the basics. A linked list is a linear data structure where elements are stored in nodes, and each node points to the next one in the sequence. Unlike arrays, linked lists don’t require contiguous memory allocation. Instead, they use pointers to connect the dots between data elements. This unique characteristic gives linked lists some superpowers that we’ll uncover as we go along.
Imagine you’re organizing a scavenger hunt. Instead of giving participants a map with all locations marked, you provide them with clues at each spot that lead to the next one. That’s essentially how a linked list works – each element knows where to find the next one, creating a chain of data.
There are several types of linked lists, each with its own quirks and use cases:
- Singly Linked List: The most basic form, where each node points to the next one.
- Doubly Linked List: Each node has pointers to both the next and previous nodes.
- Circular Linked List: The last node points back to the first, creating a loop.
As we progress through this blog post, we’ll focus primarily on singly linked lists, but don’t worry – we’ll touch on the other types too!
Why Should You Care About Linked Lists?
Now, you might be wondering, “Why bother with linked lists when we have arrays?” Great question! While arrays are fantastic for many scenarios, linked lists shine in situations where frequent insertions and deletions are needed, especially at the beginning or middle of the list.
Here are some compelling reasons to add linked lists to your coding toolkit:
- Dynamic Size: Unlike arrays, linked lists can grow or shrink on demand without requiring reallocation of the entire structure.
- Efficient Insertions and Deletions: Adding or removing elements from the beginning or middle of a linked list is typically an O(1) operation.
- Memory Efficiency: Linked lists only use as much memory as they need, which can be advantageous when dealing with large datasets.
- Flexibility: They can be easily reorganized and are well-suited for implementing other data structures like stacks and queues.
Understanding linked lists isn’t just about acing your computer science exams – it’s about having the right tool for the job when you’re tackling real-world programming challenges. So, let’s roll up our sleeves and dive into the nitty-gritty of implementing linked lists in Java!
Implementing a Singly Linked List in Java
Time to get our hands dirty with some code! We’ll start by implementing a basic singly linked list in Java. Don’t worry if you’re not a Java expert – the concepts we’ll cover are applicable to many programming languages.
First, let’s define our Node class:
public class Node<T> {
T data;
Node<T> next;
public Node(T data) {
this.data = data;
this.next = null;
}
}
This Node class is the building block of our linked list. It contains the data we want to store and a reference to the next node in the sequence. The use of generics (<T>
) allows our linked list to work with any data type.
Now, let’s create our LinkedList class:
public class LinkedList<T> {
private Node<T> head;
private int size;
public LinkedList() {
head = null;
size = 0;
}
// Methods for adding, removing, and accessing elements will go here
}
Our LinkedList class keeps track of the head (first node) of the list and the size (number of elements). Let’s add some basic operations to our linked list:
Adding Elements
We’ll implement two methods for adding elements: one to add at the beginning (head) of the list, and another to add at the end (tail).
public void addFirst(T data) {
Node<T> newNode = new Node<>(data);
newNode.next = head;
head = newNode;
size++;
}
public void addLast(T data) {
Node<T> newNode = new Node<>(data);
if (head == null) {
head = newNode;
} else {
Node<T> current = head;
while (current.next != null) {
current = current.next;
}
current.next = newNode;
}
size++;
}
Removing Elements
Let’s implement methods to remove elements from the beginning and end of the list:
public T removeFirst() {
if (head == null) {
throw new NoSuchElementException();
}
T data = head.data;
head = head.next;
size--;
return data;
}
public T removeLast() {
if (head == null) {
throw new NoSuchElementException();
}
if (head.next == null) {
T data = head.data;
head = null;
size--;
return data;
}
Node<T> current = head;
while (current.next.next != null) {
current = current.next;
}
T data = current.next.data;
current.next = null;
size--;
return data;
}
Accessing Elements
To access elements in our linked list, we’ll implement a method to get an element at a specific index:
public T get(int index) {
if (index < 0 || index >= size) {
throw new IndexOutOfBoundsException();
}
Node<T> current = head;
for (int i = 0; i < index; i++) {
current = current.next;
}
return current.data;
}
With these basic operations in place, we’ve created a functional singly linked list! But we’re just scratching the surface of what linked lists can do. Let’s explore some more advanced concepts and see how we can leverage the power of linked lists in real-world scenarios.
Traversing and Searching Linked Lists
One of the fundamental operations you’ll perform on linked lists is traversing through the elements. Whether you’re searching for a specific value, performing an operation on each element, or simply printing the contents of the list, traversal is key.
Here’s a method to print all elements in our linked list:
public void printList() {
Node<T> current = head;
while (current != null) {
System.out.print(current.data + " -> ");
current = current.next;
}
System.out.println("null");
}
This method starts at the head of the list and moves through each node, printing its data, until it reaches the end (null).
Searching for a specific element in a linked list is similar to traversal. Let’s implement a method to find the index of a given element:
public int indexOf(T data) {
Node<T> current = head;
int index = 0;
while (current != null) {
if (current.data.equals(data)) {
return index;
}
current = current.next;
index++;
}
return -1; // Element not found
}
This method returns the index of the first occurrence of the specified element, or -1 if the element is not found in the list.
The Power of Linked Lists: Real-World Applications
Now that we’ve covered the basics, let’s explore some real-world scenarios where linked lists truly shine. Understanding these applications will help you appreciate the versatility of this data structure and recognize opportunities to use it in your own projects.
1. Undo Functionality in Text Editors
Have you ever marveled at how effortlessly you can undo and redo actions in your favorite text editor? Linked lists often play a crucial role in implementing this feature. Each text modification can be stored as a node in a linked list, with the most recent change at the head. When you hit “undo,” the editor simply moves back one node in the list. “Redo” moves forward again. This approach allows for efficient memory usage and quick navigation through the edit history.
2. Music Playlists
Think about your favorite music streaming app. When you’re listening to a playlist, you can skip to the next song or go back to the previous one. This behavior is perfectly suited for a doubly linked list implementation. Each song is a node, with pointers to both the next and previous songs. This structure allows for seamless navigation in both directions through your playlist.
3. Browser History
Web browsers use a similar concept to music playlists for managing your browsing history. As you visit web pages, each URL can be added to a linked list. The “back” and “forward” buttons simply traverse this list. A doubly linked list is particularly useful here, allowing for efficient movement in both directions through your browsing history.
4. Memory Management
Operating systems often use linked lists to keep track of blocks of free memory. When a program requests memory, the OS can quickly scan the list to find an appropriate block. When memory is freed, it can be easily inserted back into the list. This approach allows for efficient allocation and deallocation of memory resources.
5. Polynomial Arithmetic
In mathematical applications, linked lists can be used to represent polynomials. Each term of the polynomial (coefficient and exponent) can be stored as a node. This representation makes it easy to perform operations like addition, subtraction, and multiplication of polynomials.
These examples demonstrate the versatility of linked lists in solving real-world problems. As you encounter various programming challenges, keep linked lists in mind – they might just be the perfect tool for the job!
Advanced Linked List Techniques
Now that we’ve explored some practical applications, let’s dive into some advanced techniques that can take your linked list skills to the next level.
Reversing a Linked List
Reversing a linked list is a classic interview question and a useful operation in many scenarios. Here’s an efficient method to reverse our singly linked list in-place:
public void reverse() {
Node<T> prev = null;
Node<T> current = head;
Node<T> next = null;
while (current != null) {
next = current.next;
current.next = prev;
prev = current;
current = next;
}
head = prev;
}
This method works by changing the direction of the next pointers as it traverses the list. It’s a great example of how linked lists can be manipulated efficiently.
Detecting Cycles
In some cases, you might encounter a linked list with a cycle – where the last node points back to a previous node, creating a loop. Detecting such cycles is crucial to prevent infinite loops in your code. Here’s a clever algorithm called Floyd’s Cycle-Finding Algorithm (also known as the “tortoise and hare” algorithm) to detect cycles:
public boolean hasCycle() {
if (head == null || head.next == null) {
return false;
}
Node<T> slow = head;
Node<T> fast = head.next;
while (slow != fast) {
if (fast == null || fast.next == null) {
return false;
}
slow = slow.next;
fast = fast.next.next;
}
return true;
}
This algorithm uses two pointers moving at different speeds. If there’s a cycle, the fast pointer will eventually catch up to the slow pointer, indicating the presence of a loop.
Merging Sorted Linked Lists
Merging two sorted linked lists is another common operation, especially in algorithms like merge sort. Here’s a method to merge two sorted linked lists:
public static <T extends Comparable<T>> LinkedList<T> mergeSortedLists(LinkedList<T> list1, LinkedList<T> list2) {
LinkedList<T> mergedList = new LinkedList<>();
Node<T> current1 = list1.head;
Node<T> current2 = list2.head;
while (current1 != null && current2 != null) {
if (current1.data.compareTo(current2.data) <= 0) {
mergedList.addLast(current1.data);
current1 = current1.next;
} else {
mergedList.addLast(current2.data);
current2 = current2.next;
}
}
while (current1 != null) {
mergedList.addLast(current1.data);
current1 = current1.next;
}
while (current2 != null) {
mergedList.addLast(current2.data);
current2 = current2.next;
}
return mergedList;
}
This method efficiently combines two sorted linked lists into a single sorted list, maintaining the original order.
Performance Considerations and Trade-offs
As with any data structure, it’s essential to understand the performance characteristics of linked lists to make informed decisions about when to use them. Let’s break down the time complexity of common operations:
- Accessing an element by index: O(n)
- Insertion at the beginning: O(1)
- Insertion at the end: O(n) for singly linked list, O(1) for doubly linked list with a tail pointer
- Deletion at the beginning: O(1)
- Deletion at the end: O(n) for singly linked list, O(1) for doubly linked list with a tail pointer
- Searching for an element: O(n)
Compared to arrays, linked lists excel at insertions and deletions, especially at the beginning of the list. However, they fall short when it comes to random access, as you need to traverse the list to reach a specific index.
Memory usage is another factor to consider. While linked lists don’t waste space on unused elements like arrays might, they do require extra memory for storing the next (and previous, in the case of doubly linked lists) pointers.
When deciding between linked lists and other data structures, consider the following:
- Frequency of insertions and deletions, especially at the beginning or middle of the list
- Need for random access to elements
- Memory constraints and usage patterns
- Requirement for maintaining a sorted order
By weighing these factors, you can choose the most appropriate data structure for your specific use case.
Linked Lists in the Java Collections Framework
While we’ve been implementing our own linked list from scratch, it’s worth noting that Java provides a built-in LinkedList class as part of the Collections Framework. This implementation offers both the List and Deque interfaces, making it a versatile choice for many applications.
Here’s a quick example of using Java’s LinkedList:
import java.util.LinkedList;
public class JavaLinkedListExample {
public static void main(String[] args) {
LinkedList<String> list = new LinkedList<>();
// Adding elements
list.add("Apple");
list.addFirst("Banana");
list.addLast("Cherry");
System.out.println("List: " + list);
// Removing elements
String first = list.removeFirst();
String last = list.removeLast();
System.out.println("Removed first: " + first);
System.out.println("Removed last: " + last);
System.out.println("Updated list: " + list);
// Accessing elements
String element = list.get(0);
System.out.println("First element: " + element);
// Checking if an element exists
boolean contains = list.contains("Apple");
System.out.println("Contains 'Apple': " + contains);
}
}
The Java LinkedList class provides a robust implementation with many useful methods, making it a great choice when you need a linked list in your Java projects.
Conclusion: Linking It All Together
We’ve journeyed through the world of linked lists, from basic concepts to advanced techniques and real-world applications. By now, you should have a solid understanding of how linked lists work, when to use them, and how to implement them in Java.
Remember, linked lists are more than just a theoretical concept – they’re a powerful tool in your programming arsenal. Whether you’re building a music player, implementing an undo feature, or optimizing memory management, linked lists can provide elegant and efficient solutions to a wide range of problems.
As you continue your programming journey, keep exploring and experimenting with different data structures. Each has its strengths and weaknesses, and mastering them all will make you a more versatile and effective developer.
So, the next time you’re faced with a programming challenge, ask yourself: “Could a linked list be the key to solving this problem?” You might be surprised at how often the answer is yes!
Keep practicing, keep coding, and keep connecting those data dots. Who knows? You might just find yourself becoming the go-to expert on linked lists in your development team.
Happy coding, and may your linked lists always be perfectly connected!
Disclaimer: While every effort has been made to ensure the accuracy and reliability of the information presented in this blog post, programming practices and language specifications may change over time. The code examples provided are for educational purposes and may need to be adapted for use in production environments. Always refer to the most up-to-date documentation and best practices when implementing linked lists or any data structure in your projects. If you notice any inaccuracies or have suggestions for improvement, please report them so we can correct them promptly.